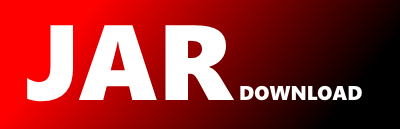
io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envoy-scala-control-plane_2.13 Show documentation
Show all versions of envoy-scala-control-plane_2.13 Show documentation
ScalaPB generated bindings for Envoy
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package io.envoyproxy.envoy.api.v2.cluster
/** :ref:`Circuit breaking<arch_overview_circuit_break>` settings can be
* specified individually for each defined priority.
*
* @param thresholds
* If multiple :ref:`Thresholds<envoy_api_msg_cluster.CircuitBreakers.Thresholds>`
* are defined with the same :ref:`RoutingPriority<envoy_api_enum_core.RoutingPriority>`,
* the first one in the list is used. If no Thresholds is defined for a given
* :ref:`RoutingPriority<envoy_api_enum_core.RoutingPriority>`, the default values
* are used.
*/
@SerialVersionUID(0L)
final case class CircuitBreakers(
thresholds: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds] = _root_.scala.Seq.empty,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[CircuitBreakers] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
thresholds.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
thresholds.foreach { __v =>
val __m = __v
_output__.writeTag(1, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def clearThresholds = copy(thresholds = _root_.scala.Seq.empty)
def addThresholds(__vs: io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds *): CircuitBreakers = addAllThresholds(__vs)
def addAllThresholds(__vs: Iterable[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds]): CircuitBreakers = copy(thresholds = thresholds ++ __vs)
def withThresholds(__v: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds]): CircuitBreakers = copy(thresholds = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => thresholds
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PRepeated(thresholds.iterator.map(_.toPMessage).toVector)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.type = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers
// @@protoc_insertion_point(GeneratedMessage[envoy.api.v2.cluster.CircuitBreakers])
}
object CircuitBreakers extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers = {
val __thresholds: _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds] = new _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds]
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__thresholds += _root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds](_input__)
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers(
thresholds = __thresholds.result(),
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers(
thresholds = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds]]).getOrElse(_root_.scala.Seq.empty)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = CircuitBreakerProto.javaDescriptor.getMessageTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = CircuitBreakerProto.scalaDescriptor.messages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 1 => __out = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers(
thresholds = _root_.scala.Seq.empty
)
/** A Thresholds defines CircuitBreaker settings for a
* :ref:`RoutingPriority<envoy_api_enum_core.RoutingPriority>`.
* [#next-free-field: 9]
*
* @param priority
* The :ref:`RoutingPriority<envoy_api_enum_core.RoutingPriority>`
* the specified CircuitBreaker settings apply to.
* @param maxConnections
* The maximum number of connections that Envoy will make to the upstream
* cluster. If not specified, the default is 1024.
* @param maxPendingRequests
* The maximum number of pending requests that Envoy will allow to the
* upstream cluster. If not specified, the default is 1024.
* @param maxRequests
* The maximum number of parallel requests that Envoy will make to the
* upstream cluster. If not specified, the default is 1024.
* @param maxRetries
* The maximum number of parallel retries that Envoy will allow to the
* upstream cluster. If not specified, the default is 3.
* @param retryBudget
* Specifies a limit on concurrent retries in relation to the number of active requests. This
* parameter is optional.
*
* .. note::
*
* If this field is set, the retry budget will override any configured retry circuit
* breaker.
* @param trackRemaining
* If track_remaining is true, then stats will be published that expose
* the number of resources remaining until the circuit breakers open. If
* not specified, the default is false.
*
* .. note::
*
* If a retry budget is used in lieu of the max_retries circuit breaker,
* the remaining retry resources remaining will not be tracked.
* @param maxConnectionPools
* The maximum number of connection pools per cluster that Envoy will concurrently support at
* once. If not specified, the default is unlimited. Set this for clusters which create a
* large number of connection pools. See
* :ref:`Circuit Breaking <arch_overview_circuit_break_cluster_maximum_connection_pools>` for
* more details.
*/
@SerialVersionUID(0L)
final case class Thresholds(
priority: io.envoyproxy.envoy.api.v2.core.RoutingPriority = io.envoyproxy.envoy.api.v2.core.RoutingPriority.DEFAULT,
maxConnections: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None,
maxPendingRequests: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None,
maxRequests: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None,
maxRetries: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None,
retryBudget: _root_.scala.Option[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget] = _root_.scala.None,
trackRemaining: _root_.scala.Boolean = false,
maxConnectionPools: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Thresholds] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = priority.value
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeEnumSize(1, __value)
}
};
if (maxConnections.isDefined) {
val __value = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnections.toBase(maxConnections.get)
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (maxPendingRequests.isDefined) {
val __value = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxPendingRequests.toBase(maxPendingRequests.get)
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (maxRequests.isDefined) {
val __value = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRequests.toBase(maxRequests.get)
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (maxRetries.isDefined) {
val __value = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRetries.toBase(maxRetries.get)
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (retryBudget.isDefined) {
val __value = retryBudget.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
{
val __value = trackRemaining
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(6, __value)
}
};
if (maxConnectionPools.isDefined) {
val __value = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnectionPools.toBase(maxConnectionPools.get)
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = priority.value
if (__v != 0) {
_output__.writeEnum(1, __v)
}
};
maxConnections.foreach { __v =>
val __m = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnections.toBase(__v)
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
maxPendingRequests.foreach { __v =>
val __m = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxPendingRequests.toBase(__v)
_output__.writeTag(3, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
maxRequests.foreach { __v =>
val __m = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRequests.toBase(__v)
_output__.writeTag(4, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
maxRetries.foreach { __v =>
val __m = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRetries.toBase(__v)
_output__.writeTag(5, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = trackRemaining
if (__v != false) {
_output__.writeBool(6, __v)
}
};
maxConnectionPools.foreach { __v =>
val __m = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnectionPools.toBase(__v)
_output__.writeTag(7, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
retryBudget.foreach { __v =>
val __m = __v
_output__.writeTag(8, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withPriority(__v: io.envoyproxy.envoy.api.v2.core.RoutingPriority): Thresholds = copy(priority = __v)
def getMaxConnections: _root_.scala.Int = maxConnections.getOrElse(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnections.toCustom(com.google.protobuf.wrappers.UInt32Value.defaultInstance))
def clearMaxConnections: Thresholds = copy(maxConnections = _root_.scala.None)
def withMaxConnections(__v: _root_.scala.Int): Thresholds = copy(maxConnections = Option(__v))
def getMaxPendingRequests: _root_.scala.Int = maxPendingRequests.getOrElse(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxPendingRequests.toCustom(com.google.protobuf.wrappers.UInt32Value.defaultInstance))
def clearMaxPendingRequests: Thresholds = copy(maxPendingRequests = _root_.scala.None)
def withMaxPendingRequests(__v: _root_.scala.Int): Thresholds = copy(maxPendingRequests = Option(__v))
def getMaxRequests: _root_.scala.Int = maxRequests.getOrElse(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRequests.toCustom(com.google.protobuf.wrappers.UInt32Value.defaultInstance))
def clearMaxRequests: Thresholds = copy(maxRequests = _root_.scala.None)
def withMaxRequests(__v: _root_.scala.Int): Thresholds = copy(maxRequests = Option(__v))
def getMaxRetries: _root_.scala.Int = maxRetries.getOrElse(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRetries.toCustom(com.google.protobuf.wrappers.UInt32Value.defaultInstance))
def clearMaxRetries: Thresholds = copy(maxRetries = _root_.scala.None)
def withMaxRetries(__v: _root_.scala.Int): Thresholds = copy(maxRetries = Option(__v))
def getRetryBudget: io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget = retryBudget.getOrElse(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget.defaultInstance)
def clearRetryBudget: Thresholds = copy(retryBudget = _root_.scala.None)
def withRetryBudget(__v: io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget): Thresholds = copy(retryBudget = Option(__v))
def withTrackRemaining(__v: _root_.scala.Boolean): Thresholds = copy(trackRemaining = __v)
def getMaxConnectionPools: _root_.scala.Int = maxConnectionPools.getOrElse(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnectionPools.toCustom(com.google.protobuf.wrappers.UInt32Value.defaultInstance))
def clearMaxConnectionPools: Thresholds = copy(maxConnectionPools = _root_.scala.None)
def withMaxConnectionPools(__v: _root_.scala.Int): Thresholds = copy(maxConnectionPools = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = priority.javaValueDescriptor
if (__t.getNumber() != 0) __t else null
}
case 2 => maxConnections.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnections.toBase(_)).orNull
case 3 => maxPendingRequests.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxPendingRequests.toBase(_)).orNull
case 4 => maxRequests.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRequests.toBase(_)).orNull
case 5 => maxRetries.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRetries.toBase(_)).orNull
case 8 => retryBudget.orNull
case 6 => {
val __t = trackRemaining
if (__t != false) __t else null
}
case 7 => maxConnectionPools.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnectionPools.toBase(_)).orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PEnum(priority.scalaValueDescriptor)
case 2 => maxConnections.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnections.toBase(_).toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 3 => maxPendingRequests.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxPendingRequests.toBase(_).toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 4 => maxRequests.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRequests.toBase(_).toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 5 => maxRetries.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRetries.toBase(_).toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 8 => retryBudget.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 6 => _root_.scalapb.descriptors.PBoolean(trackRemaining)
case 7 => maxConnectionPools.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnectionPools.toBase(_).toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.type = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds
// @@protoc_insertion_point(GeneratedMessage[envoy.api.v2.cluster.CircuitBreakers.Thresholds])
}
object Thresholds extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds = {
var __priority: io.envoyproxy.envoy.api.v2.core.RoutingPriority = io.envoyproxy.envoy.api.v2.core.RoutingPriority.DEFAULT
var __maxConnections: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None
var __maxPendingRequests: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None
var __maxRequests: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None
var __maxRetries: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None
var __retryBudget: _root_.scala.Option[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget] = _root_.scala.None
var __trackRemaining: _root_.scala.Boolean = false
var __maxConnectionPools: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 8 =>
__priority = io.envoyproxy.envoy.api.v2.core.RoutingPriority.fromValue(_input__.readEnum())
case 18 =>
__maxConnections = Option(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnections.toCustom(__maxConnections.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnections.toBase(_)).fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.wrappers.UInt32Value](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _))))
case 26 =>
__maxPendingRequests = Option(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxPendingRequests.toCustom(__maxPendingRequests.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxPendingRequests.toBase(_)).fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.wrappers.UInt32Value](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _))))
case 34 =>
__maxRequests = Option(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRequests.toCustom(__maxRequests.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRequests.toBase(_)).fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.wrappers.UInt32Value](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _))))
case 42 =>
__maxRetries = Option(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRetries.toCustom(__maxRetries.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRetries.toBase(_)).fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.wrappers.UInt32Value](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _))))
case 66 =>
__retryBudget = Option(__retryBudget.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 48 =>
__trackRemaining = _input__.readBool()
case 58 =>
__maxConnectionPools = Option(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnectionPools.toCustom(__maxConnectionPools.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnectionPools.toBase(_)).fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.wrappers.UInt32Value](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _))))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds(
priority = __priority,
maxConnections = __maxConnections,
maxPendingRequests = __maxPendingRequests,
maxRequests = __maxRequests,
maxRetries = __maxRetries,
retryBudget = __retryBudget,
trackRemaining = __trackRemaining,
maxConnectionPools = __maxConnectionPools,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds(
priority = io.envoyproxy.envoy.api.v2.core.RoutingPriority.fromValue(__fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scalapb.descriptors.EnumValueDescriptor]).getOrElse(io.envoyproxy.envoy.api.v2.core.RoutingPriority.DEFAULT.scalaValueDescriptor).number),
maxConnections = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.wrappers.UInt32Value]]).map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnections.toCustom(_)),
maxPendingRequests = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.wrappers.UInt32Value]]).map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxPendingRequests.toCustom(_)),
maxRequests = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.wrappers.UInt32Value]]).map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRequests.toCustom(_)),
maxRetries = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.wrappers.UInt32Value]]).map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxRetries.toCustom(_)),
retryBudget = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget]]),
trackRemaining = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Boolean]).getOrElse(false),
maxConnectionPools = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.wrappers.UInt32Value]]).map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds._typemapper_maxConnectionPools.toCustom(_))
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 2 => __out = com.google.protobuf.wrappers.UInt32Value
case 3 => __out = com.google.protobuf.wrappers.UInt32Value
case 4 => __out = com.google.protobuf.wrappers.UInt32Value
case 5 => __out = com.google.protobuf.wrappers.UInt32Value
case 8 => __out = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget
case 7 => __out = com.google.protobuf.wrappers.UInt32Value
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => io.envoyproxy.envoy.api.v2.core.RoutingPriority
}
}
lazy val defaultInstance = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds(
priority = io.envoyproxy.envoy.api.v2.core.RoutingPriority.DEFAULT,
maxConnections = _root_.scala.None,
maxPendingRequests = _root_.scala.None,
maxRequests = _root_.scala.None,
maxRetries = _root_.scala.None,
retryBudget = _root_.scala.None,
trackRemaining = false,
maxConnectionPools = _root_.scala.None
)
/** @param budgetPercent
* Specifies the limit on concurrent retries as a percentage of the sum of active requests and
* active pending requests. For example, if there are 100 active requests and the
* budget_percent is set to 25, there may be 25 active retries.
*
* This parameter is optional. Defaults to 20%.
* @param minRetryConcurrency
* Specifies the minimum retry concurrency allowed for the retry budget. The limit on the
* number of active retries may never go below this number.
*
* This parameter is optional. Defaults to 3.
*/
@SerialVersionUID(0L)
final case class RetryBudget(
budgetPercent: _root_.scala.Option[io.envoyproxy.envoy.`type`.Percent] = _root_.scala.None,
minRetryConcurrency: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[RetryBudget] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
if (budgetPercent.isDefined) {
val __value = budgetPercent.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (minRetryConcurrency.isDefined) {
val __value = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget._typemapper_minRetryConcurrency.toBase(minRetryConcurrency.get)
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
budgetPercent.foreach { __v =>
val __m = __v
_output__.writeTag(1, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
minRetryConcurrency.foreach { __v =>
val __m = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget._typemapper_minRetryConcurrency.toBase(__v)
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def getBudgetPercent: io.envoyproxy.envoy.`type`.Percent = budgetPercent.getOrElse(io.envoyproxy.envoy.`type`.Percent.defaultInstance)
def clearBudgetPercent: RetryBudget = copy(budgetPercent = _root_.scala.None)
def withBudgetPercent(__v: io.envoyproxy.envoy.`type`.Percent): RetryBudget = copy(budgetPercent = Option(__v))
def getMinRetryConcurrency: _root_.scala.Int = minRetryConcurrency.getOrElse(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget._typemapper_minRetryConcurrency.toCustom(com.google.protobuf.wrappers.UInt32Value.defaultInstance))
def clearMinRetryConcurrency: RetryBudget = copy(minRetryConcurrency = _root_.scala.None)
def withMinRetryConcurrency(__v: _root_.scala.Int): RetryBudget = copy(minRetryConcurrency = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => budgetPercent.orNull
case 2 => minRetryConcurrency.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget._typemapper_minRetryConcurrency.toBase(_)).orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => budgetPercent.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 2 => minRetryConcurrency.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget._typemapper_minRetryConcurrency.toBase(_).toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget.type = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget
// @@protoc_insertion_point(GeneratedMessage[envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget])
}
object RetryBudget extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget = {
var __budgetPercent: _root_.scala.Option[io.envoyproxy.envoy.`type`.Percent] = _root_.scala.None
var __minRetryConcurrency: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__budgetPercent = Option(__budgetPercent.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.`type`.Percent](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 18 =>
__minRetryConcurrency = Option(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget._typemapper_minRetryConcurrency.toCustom(__minRetryConcurrency.map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget._typemapper_minRetryConcurrency.toBase(_)).fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.wrappers.UInt32Value](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _))))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget(
budgetPercent = __budgetPercent,
minRetryConcurrency = __minRetryConcurrency,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget(
budgetPercent = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.`type`.Percent]]),
minRetryConcurrency = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.wrappers.UInt32Value]]).map(io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget._typemapper_minRetryConcurrency.toCustom(_))
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 1 => __out = io.envoyproxy.envoy.`type`.Percent
case 2 => __out = com.google.protobuf.wrappers.UInt32Value
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget(
budgetPercent = _root_.scala.None,
minRetryConcurrency = _root_.scala.None
)
implicit class RetryBudgetLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget](_l) {
def budgetPercent: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.`type`.Percent] = field(_.getBudgetPercent)((c_, f_) => c_.copy(budgetPercent = Option(f_)))
def optionalBudgetPercent: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[io.envoyproxy.envoy.`type`.Percent]] = field(_.budgetPercent)((c_, f_) => c_.copy(budgetPercent = f_))
def minRetryConcurrency: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.getMinRetryConcurrency)((c_, f_) => c_.copy(minRetryConcurrency = Option(f_)))
def optionalMinRetryConcurrency: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[_root_.scala.Int]] = field(_.minRetryConcurrency)((c_, f_) => c_.copy(minRetryConcurrency = f_))
}
final val BUDGET_PERCENT_FIELD_NUMBER = 1
final val MIN_RETRY_CONCURRENCY_FIELD_NUMBER = 2
@transient
private[cluster] val _typemapper_minRetryConcurrency: _root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int] = implicitly[_root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int]]
def of(
budgetPercent: _root_.scala.Option[io.envoyproxy.envoy.`type`.Percent],
minRetryConcurrency: _root_.scala.Option[_root_.scala.Int]
): _root_.io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget = _root_.io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget(
budgetPercent,
minRetryConcurrency
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget])
}
implicit class ThresholdsLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds](_l) {
def priority: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.core.RoutingPriority] = field(_.priority)((c_, f_) => c_.copy(priority = f_))
def maxConnections: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.getMaxConnections)((c_, f_) => c_.copy(maxConnections = Option(f_)))
def optionalMaxConnections: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[_root_.scala.Int]] = field(_.maxConnections)((c_, f_) => c_.copy(maxConnections = f_))
def maxPendingRequests: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.getMaxPendingRequests)((c_, f_) => c_.copy(maxPendingRequests = Option(f_)))
def optionalMaxPendingRequests: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[_root_.scala.Int]] = field(_.maxPendingRequests)((c_, f_) => c_.copy(maxPendingRequests = f_))
def maxRequests: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.getMaxRequests)((c_, f_) => c_.copy(maxRequests = Option(f_)))
def optionalMaxRequests: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[_root_.scala.Int]] = field(_.maxRequests)((c_, f_) => c_.copy(maxRequests = f_))
def maxRetries: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.getMaxRetries)((c_, f_) => c_.copy(maxRetries = Option(f_)))
def optionalMaxRetries: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[_root_.scala.Int]] = field(_.maxRetries)((c_, f_) => c_.copy(maxRetries = f_))
def retryBudget: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget] = field(_.getRetryBudget)((c_, f_) => c_.copy(retryBudget = Option(f_)))
def optionalRetryBudget: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget]] = field(_.retryBudget)((c_, f_) => c_.copy(retryBudget = f_))
def trackRemaining: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.trackRemaining)((c_, f_) => c_.copy(trackRemaining = f_))
def maxConnectionPools: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.getMaxConnectionPools)((c_, f_) => c_.copy(maxConnectionPools = Option(f_)))
def optionalMaxConnectionPools: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[_root_.scala.Int]] = field(_.maxConnectionPools)((c_, f_) => c_.copy(maxConnectionPools = f_))
}
final val PRIORITY_FIELD_NUMBER = 1
final val MAX_CONNECTIONS_FIELD_NUMBER = 2
final val MAX_PENDING_REQUESTS_FIELD_NUMBER = 3
final val MAX_REQUESTS_FIELD_NUMBER = 4
final val MAX_RETRIES_FIELD_NUMBER = 5
final val RETRY_BUDGET_FIELD_NUMBER = 8
final val TRACK_REMAINING_FIELD_NUMBER = 6
final val MAX_CONNECTION_POOLS_FIELD_NUMBER = 7
@transient
private[cluster] val _typemapper_maxConnections: _root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int] = implicitly[_root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int]]
@transient
private[cluster] val _typemapper_maxPendingRequests: _root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int] = implicitly[_root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int]]
@transient
private[cluster] val _typemapper_maxRequests: _root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int] = implicitly[_root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int]]
@transient
private[cluster] val _typemapper_maxRetries: _root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int] = implicitly[_root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int]]
@transient
private[cluster] val _typemapper_maxConnectionPools: _root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int] = implicitly[_root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int]]
def of(
priority: io.envoyproxy.envoy.api.v2.core.RoutingPriority,
maxConnections: _root_.scala.Option[_root_.scala.Int],
maxPendingRequests: _root_.scala.Option[_root_.scala.Int],
maxRequests: _root_.scala.Option[_root_.scala.Int],
maxRetries: _root_.scala.Option[_root_.scala.Int],
retryBudget: _root_.scala.Option[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds.RetryBudget],
trackRemaining: _root_.scala.Boolean,
maxConnectionPools: _root_.scala.Option[_root_.scala.Int]
): _root_.io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds = _root_.io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds(
priority,
maxConnections,
maxPendingRequests,
maxRequests,
maxRetries,
retryBudget,
trackRemaining,
maxConnectionPools
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.api.v2.cluster.CircuitBreakers.Thresholds])
}
implicit class CircuitBreakersLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers](_l) {
def thresholds: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds]] = field(_.thresholds)((c_, f_) => c_.copy(thresholds = f_))
}
final val THRESHOLDS_FIELD_NUMBER = 1
def of(
thresholds: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers.Thresholds]
): _root_.io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers = _root_.io.envoyproxy.envoy.api.v2.cluster.CircuitBreakers(
thresholds
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.api.v2.cluster.CircuitBreakers])
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy