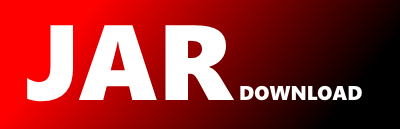
io.envoyproxy.envoy.api.v2.route.WeightedCluster.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envoy-scala-control-plane_2.13 Show documentation
Show all versions of envoy-scala-control-plane_2.13 Show documentation
ScalaPB generated bindings for Envoy
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package io.envoyproxy.envoy.api.v2.route
/** Compared to the :ref:`cluster <envoy_api_field_route.RouteAction.cluster>` field that specifies a
* single upstream cluster as the target of a request, the :ref:`weighted_clusters
* <envoy_api_field_route.RouteAction.weighted_clusters>` option allows for specification of
* multiple upstream clusters along with weights that indicate the percentage of
* traffic to be forwarded to each cluster. The router selects an upstream cluster based on the
* weights.
*
* @param clusters
* Specifies one or more upstream clusters associated with the route.
* @param totalWeight
* Specifies the total weight across all clusters. The sum of all cluster weights must equal this
* value, which must be greater than 0. Defaults to 100.
* @param runtimeKeyPrefix
* Specifies the runtime key prefix that should be used to construct the
* runtime keys associated with each cluster. When the *runtime_key_prefix* is
* specified, the router will look for weights associated with each upstream
* cluster under the key *runtime_key_prefix* + "." + *cluster[i].name* where
* *cluster[i]* denotes an entry in the clusters array field. If the runtime
* key for the cluster does not exist, the value specified in the
* configuration file will be used as the default weight. See the :ref:`runtime documentation
* <operations_runtime>` for how key names map to the underlying implementation.
*/
@SerialVersionUID(0L)
final case class WeightedCluster(
clusters: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight] = _root_.scala.Seq.empty,
totalWeight: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None,
runtimeKeyPrefix: _root_.scala.Predef.String = "",
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[WeightedCluster] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
clusters.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
if (totalWeight.isDefined) {
val __value = io.envoyproxy.envoy.api.v2.route.WeightedCluster._typemapper_totalWeight.toBase(totalWeight.get)
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
{
val __value = runtimeKeyPrefix
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(2, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
clusters.foreach { __v =>
val __m = __v
_output__.writeTag(1, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = runtimeKeyPrefix
if (!__v.isEmpty) {
_output__.writeString(2, __v)
}
};
totalWeight.foreach { __v =>
val __m = io.envoyproxy.envoy.api.v2.route.WeightedCluster._typemapper_totalWeight.toBase(__v)
_output__.writeTag(3, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def clearClusters = copy(clusters = _root_.scala.Seq.empty)
def addClusters(__vs: io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight *): WeightedCluster = addAllClusters(__vs)
def addAllClusters(__vs: Iterable[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight]): WeightedCluster = copy(clusters = clusters ++ __vs)
def withClusters(__v: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight]): WeightedCluster = copy(clusters = __v)
def getTotalWeight: _root_.scala.Int = totalWeight.getOrElse(io.envoyproxy.envoy.api.v2.route.WeightedCluster._typemapper_totalWeight.toCustom(com.google.protobuf.wrappers.UInt32Value.defaultInstance))
def clearTotalWeight: WeightedCluster = copy(totalWeight = _root_.scala.None)
def withTotalWeight(__v: _root_.scala.Int): WeightedCluster = copy(totalWeight = Option(__v))
def withRuntimeKeyPrefix(__v: _root_.scala.Predef.String): WeightedCluster = copy(runtimeKeyPrefix = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => clusters
case 3 => totalWeight.map(io.envoyproxy.envoy.api.v2.route.WeightedCluster._typemapper_totalWeight.toBase(_)).orNull
case 2 => {
val __t = runtimeKeyPrefix
if (__t != "") __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PRepeated(clusters.iterator.map(_.toPMessage).toVector)
case 3 => totalWeight.map(io.envoyproxy.envoy.api.v2.route.WeightedCluster._typemapper_totalWeight.toBase(_).toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 2 => _root_.scalapb.descriptors.PString(runtimeKeyPrefix)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.api.v2.route.WeightedCluster.type = io.envoyproxy.envoy.api.v2.route.WeightedCluster
// @@protoc_insertion_point(GeneratedMessage[envoy.api.v2.route.WeightedCluster])
}
object WeightedCluster extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.route.WeightedCluster] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.route.WeightedCluster] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.api.v2.route.WeightedCluster = {
val __clusters: _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight] = new _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight]
var __totalWeight: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None
var __runtimeKeyPrefix: _root_.scala.Predef.String = ""
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__clusters += _root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight](_input__)
case 26 =>
__totalWeight = Option(io.envoyproxy.envoy.api.v2.route.WeightedCluster._typemapper_totalWeight.toCustom(__totalWeight.map(io.envoyproxy.envoy.api.v2.route.WeightedCluster._typemapper_totalWeight.toBase(_)).fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.wrappers.UInt32Value](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _))))
case 18 =>
__runtimeKeyPrefix = _input__.readStringRequireUtf8()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.api.v2.route.WeightedCluster(
clusters = __clusters.result(),
totalWeight = __totalWeight,
runtimeKeyPrefix = __runtimeKeyPrefix,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.api.v2.route.WeightedCluster] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.api.v2.route.WeightedCluster(
clusters = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight]]).getOrElse(_root_.scala.Seq.empty),
totalWeight = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.wrappers.UInt32Value]]).map(io.envoyproxy.envoy.api.v2.route.WeightedCluster._typemapper_totalWeight.toCustom(_)),
runtimeKeyPrefix = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Predef.String]).getOrElse("")
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = RouteComponentsProto.javaDescriptor.getMessageTypes().get(3)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = RouteComponentsProto.scalaDescriptor.messages(3)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 1 => __out = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight
case 3 => __out = com.google.protobuf.wrappers.UInt32Value
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.envoyproxy.envoy.api.v2.route.WeightedCluster(
clusters = _root_.scala.Seq.empty,
totalWeight = _root_.scala.None,
runtimeKeyPrefix = ""
)
/** [#next-free-field: 11]
*
* @param name
* Name of the upstream cluster. The cluster must exist in the
* :ref:`cluster manager configuration <config_cluster_manager>`.
* @param weight
* An integer between 0 and :ref:`total_weight
* <envoy_api_field_route.WeightedCluster.total_weight>`. When a request matches the route,
* the choice of an upstream cluster is determined by its weight. The sum of weights across all
* entries in the clusters array must add up to the total_weight, which defaults to 100.
* @param metadataMatch
* Optional endpoint metadata match criteria used by the subset load balancer. Only endpoints in
* the upstream cluster with metadata matching what is set in this field will be considered for
* load balancing. Note that this will be merged with what's provided in
* :ref:`RouteAction.metadata_match <envoy_api_field_route.RouteAction.metadata_match>`, with
* values here taking precedence. The filter name should be specified as *envoy.lb*.
* @param requestHeadersToAdd
* Specifies a list of headers to be added to requests when this cluster is selected
* through the enclosing :ref:`envoy_api_msg_route.RouteAction`.
* Headers specified at this level are applied before headers from the enclosing
* :ref:`envoy_api_msg_route.Route`, :ref:`envoy_api_msg_route.VirtualHost`, and
* :ref:`envoy_api_msg_RouteConfiguration`. For more information, including details on
* header value syntax, see the documentation on :ref:`custom request headers
* <config_http_conn_man_headers_custom_request_headers>`.
* @param requestHeadersToRemove
* Specifies a list of HTTP headers that should be removed from each request when
* this cluster is selected through the enclosing :ref:`envoy_api_msg_route.RouteAction`.
* @param responseHeadersToAdd
* Specifies a list of headers to be added to responses when this cluster is selected
* through the enclosing :ref:`envoy_api_msg_route.RouteAction`.
* Headers specified at this level are applied before headers from the enclosing
* :ref:`envoy_api_msg_route.Route`, :ref:`envoy_api_msg_route.VirtualHost`, and
* :ref:`envoy_api_msg_RouteConfiguration`. For more information, including details on
* header value syntax, see the documentation on :ref:`custom request headers
* <config_http_conn_man_headers_custom_request_headers>`.
* @param responseHeadersToRemove
* Specifies a list of headers to be removed from responses when this cluster is selected
* through the enclosing :ref:`envoy_api_msg_route.RouteAction`.
* @param perFilterConfig
* The per_filter_config field can be used to provide weighted cluster-specific
* configurations for filters. The key should match the filter name, such as
* *envoy.filters.http.buffer* for the HTTP buffer filter. Use of this field is filter
* specific; see the :ref:`HTTP filter documentation <config_http_filters>`
* for if and how it is utilized.
* @param typedPerFilterConfig
* The per_filter_config field can be used to provide weighted cluster-specific
* configurations for filters. The key should match the filter name, such as
* *envoy.filters.http.buffer* for the HTTP buffer filter. Use of this field is filter
* specific; see the :ref:`HTTP filter documentation <config_http_filters>`
* for if and how it is utilized.
*/
@SerialVersionUID(0L)
final case class ClusterWeight(
name: _root_.scala.Predef.String = "",
weight: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None,
metadataMatch: _root_.scala.Option[io.envoyproxy.envoy.api.v2.core.Metadata] = _root_.scala.None,
requestHeadersToAdd: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.core.HeaderValueOption] = _root_.scala.Seq.empty,
requestHeadersToRemove: _root_.scala.Seq[_root_.scala.Predef.String] = _root_.scala.Seq.empty,
responseHeadersToAdd: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.core.HeaderValueOption] = _root_.scala.Seq.empty,
responseHeadersToRemove: _root_.scala.Seq[_root_.scala.Predef.String] = _root_.scala.Seq.empty,
@scala.deprecated(message="Marked as deprecated in proto file", "") perFilterConfig: _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, com.google.protobuf.struct.Struct] = _root_.scala.collection.immutable.Map.empty,
typedPerFilterConfig: _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, com.google.protobuf.any.Any] = _root_.scala.collection.immutable.Map.empty,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[ClusterWeight] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = name
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
}
};
if (weight.isDefined) {
val __value = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_weight.toBase(weight.get)
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (metadataMatch.isDefined) {
val __value = metadataMatch.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
requestHeadersToAdd.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
requestHeadersToRemove.foreach { __item =>
val __value = __item
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(9, __value)
}
responseHeadersToAdd.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
responseHeadersToRemove.foreach { __item =>
val __value = __item
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(6, __value)
}
perFilterConfig.foreach { __item =>
val __value = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_perFilterConfig.toBase(__item)
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
typedPerFilterConfig.foreach { __item =>
val __value = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_typedPerFilterConfig.toBase(__item)
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = name
if (!__v.isEmpty) {
_output__.writeString(1, __v)
}
};
weight.foreach { __v =>
val __m = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_weight.toBase(__v)
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
metadataMatch.foreach { __v =>
val __m = __v
_output__.writeTag(3, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
requestHeadersToAdd.foreach { __v =>
val __m = __v
_output__.writeTag(4, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
responseHeadersToAdd.foreach { __v =>
val __m = __v
_output__.writeTag(5, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
responseHeadersToRemove.foreach { __v =>
val __m = __v
_output__.writeString(6, __m)
};
perFilterConfig.foreach { __v =>
val __m = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_perFilterConfig.toBase(__v)
_output__.writeTag(8, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
requestHeadersToRemove.foreach { __v =>
val __m = __v
_output__.writeString(9, __m)
};
typedPerFilterConfig.foreach { __v =>
val __m = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_typedPerFilterConfig.toBase(__v)
_output__.writeTag(10, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withName(__v: _root_.scala.Predef.String): ClusterWeight = copy(name = __v)
def getWeight: _root_.scala.Int = weight.getOrElse(io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_weight.toCustom(com.google.protobuf.wrappers.UInt32Value.defaultInstance))
def clearWeight: ClusterWeight = copy(weight = _root_.scala.None)
def withWeight(__v: _root_.scala.Int): ClusterWeight = copy(weight = Option(__v))
def getMetadataMatch: io.envoyproxy.envoy.api.v2.core.Metadata = metadataMatch.getOrElse(io.envoyproxy.envoy.api.v2.core.Metadata.defaultInstance)
def clearMetadataMatch: ClusterWeight = copy(metadataMatch = _root_.scala.None)
def withMetadataMatch(__v: io.envoyproxy.envoy.api.v2.core.Metadata): ClusterWeight = copy(metadataMatch = Option(__v))
def clearRequestHeadersToAdd = copy(requestHeadersToAdd = _root_.scala.Seq.empty)
def addRequestHeadersToAdd(__vs: io.envoyproxy.envoy.api.v2.core.HeaderValueOption *): ClusterWeight = addAllRequestHeadersToAdd(__vs)
def addAllRequestHeadersToAdd(__vs: Iterable[io.envoyproxy.envoy.api.v2.core.HeaderValueOption]): ClusterWeight = copy(requestHeadersToAdd = requestHeadersToAdd ++ __vs)
def withRequestHeadersToAdd(__v: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.core.HeaderValueOption]): ClusterWeight = copy(requestHeadersToAdd = __v)
def clearRequestHeadersToRemove = copy(requestHeadersToRemove = _root_.scala.Seq.empty)
def addRequestHeadersToRemove(__vs: _root_.scala.Predef.String *): ClusterWeight = addAllRequestHeadersToRemove(__vs)
def addAllRequestHeadersToRemove(__vs: Iterable[_root_.scala.Predef.String]): ClusterWeight = copy(requestHeadersToRemove = requestHeadersToRemove ++ __vs)
def withRequestHeadersToRemove(__v: _root_.scala.Seq[_root_.scala.Predef.String]): ClusterWeight = copy(requestHeadersToRemove = __v)
def clearResponseHeadersToAdd = copy(responseHeadersToAdd = _root_.scala.Seq.empty)
def addResponseHeadersToAdd(__vs: io.envoyproxy.envoy.api.v2.core.HeaderValueOption *): ClusterWeight = addAllResponseHeadersToAdd(__vs)
def addAllResponseHeadersToAdd(__vs: Iterable[io.envoyproxy.envoy.api.v2.core.HeaderValueOption]): ClusterWeight = copy(responseHeadersToAdd = responseHeadersToAdd ++ __vs)
def withResponseHeadersToAdd(__v: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.core.HeaderValueOption]): ClusterWeight = copy(responseHeadersToAdd = __v)
def clearResponseHeadersToRemove = copy(responseHeadersToRemove = _root_.scala.Seq.empty)
def addResponseHeadersToRemove(__vs: _root_.scala.Predef.String *): ClusterWeight = addAllResponseHeadersToRemove(__vs)
def addAllResponseHeadersToRemove(__vs: Iterable[_root_.scala.Predef.String]): ClusterWeight = copy(responseHeadersToRemove = responseHeadersToRemove ++ __vs)
def withResponseHeadersToRemove(__v: _root_.scala.Seq[_root_.scala.Predef.String]): ClusterWeight = copy(responseHeadersToRemove = __v)
def clearPerFilterConfig = copy(perFilterConfig = _root_.scala.collection.immutable.Map.empty)
def addPerFilterConfig(__vs: (_root_.scala.Predef.String, com.google.protobuf.struct.Struct) *): ClusterWeight = addAllPerFilterConfig(__vs)
def addAllPerFilterConfig(__vs: Iterable[(_root_.scala.Predef.String, com.google.protobuf.struct.Struct)]): ClusterWeight = copy(perFilterConfig = perFilterConfig ++ __vs)
def withPerFilterConfig(__v: _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, com.google.protobuf.struct.Struct]): ClusterWeight = copy(perFilterConfig = __v)
def clearTypedPerFilterConfig = copy(typedPerFilterConfig = _root_.scala.collection.immutable.Map.empty)
def addTypedPerFilterConfig(__vs: (_root_.scala.Predef.String, com.google.protobuf.any.Any) *): ClusterWeight = addAllTypedPerFilterConfig(__vs)
def addAllTypedPerFilterConfig(__vs: Iterable[(_root_.scala.Predef.String, com.google.protobuf.any.Any)]): ClusterWeight = copy(typedPerFilterConfig = typedPerFilterConfig ++ __vs)
def withTypedPerFilterConfig(__v: _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, com.google.protobuf.any.Any]): ClusterWeight = copy(typedPerFilterConfig = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = name
if (__t != "") __t else null
}
case 2 => weight.map(io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_weight.toBase(_)).orNull
case 3 => metadataMatch.orNull
case 4 => requestHeadersToAdd
case 9 => requestHeadersToRemove
case 5 => responseHeadersToAdd
case 6 => responseHeadersToRemove
case 8 => perFilterConfig.iterator.map(io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_perFilterConfig.toBase(_)).toSeq
case 10 => typedPerFilterConfig.iterator.map(io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_typedPerFilterConfig.toBase(_)).toSeq
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PString(name)
case 2 => weight.map(io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_weight.toBase(_).toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 3 => metadataMatch.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 4 => _root_.scalapb.descriptors.PRepeated(requestHeadersToAdd.iterator.map(_.toPMessage).toVector)
case 9 => _root_.scalapb.descriptors.PRepeated(requestHeadersToRemove.iterator.map(_root_.scalapb.descriptors.PString(_)).toVector)
case 5 => _root_.scalapb.descriptors.PRepeated(responseHeadersToAdd.iterator.map(_.toPMessage).toVector)
case 6 => _root_.scalapb.descriptors.PRepeated(responseHeadersToRemove.iterator.map(_root_.scalapb.descriptors.PString(_)).toVector)
case 8 => _root_.scalapb.descriptors.PRepeated(perFilterConfig.iterator.map(io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_perFilterConfig.toBase(_).toPMessage).toVector)
case 10 => _root_.scalapb.descriptors.PRepeated(typedPerFilterConfig.iterator.map(io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_typedPerFilterConfig.toBase(_).toPMessage).toVector)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.type = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight
// @@protoc_insertion_point(GeneratedMessage[envoy.api.v2.route.WeightedCluster.ClusterWeight])
}
object ClusterWeight extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight = {
var __name: _root_.scala.Predef.String = ""
var __weight: _root_.scala.Option[_root_.scala.Int] = _root_.scala.None
var __metadataMatch: _root_.scala.Option[io.envoyproxy.envoy.api.v2.core.Metadata] = _root_.scala.None
val __requestHeadersToAdd: _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.api.v2.core.HeaderValueOption] = new _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.api.v2.core.HeaderValueOption]
val __requestHeadersToRemove: _root_.scala.collection.immutable.VectorBuilder[_root_.scala.Predef.String] = new _root_.scala.collection.immutable.VectorBuilder[_root_.scala.Predef.String]
val __responseHeadersToAdd: _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.api.v2.core.HeaderValueOption] = new _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.api.v2.core.HeaderValueOption]
val __responseHeadersToRemove: _root_.scala.collection.immutable.VectorBuilder[_root_.scala.Predef.String] = new _root_.scala.collection.immutable.VectorBuilder[_root_.scala.Predef.String]
val __perFilterConfig: _root_.scala.collection.mutable.Builder[(_root_.scala.Predef.String, com.google.protobuf.struct.Struct), _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, com.google.protobuf.struct.Struct]] = _root_.scala.collection.immutable.Map.newBuilder[_root_.scala.Predef.String, com.google.protobuf.struct.Struct]
val __typedPerFilterConfig: _root_.scala.collection.mutable.Builder[(_root_.scala.Predef.String, com.google.protobuf.any.Any), _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, com.google.protobuf.any.Any]] = _root_.scala.collection.immutable.Map.newBuilder[_root_.scala.Predef.String, com.google.protobuf.any.Any]
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__name = _input__.readStringRequireUtf8()
case 18 =>
__weight = Option(io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_weight.toCustom(__weight.map(io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_weight.toBase(_)).fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.wrappers.UInt32Value](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _))))
case 26 =>
__metadataMatch = Option(__metadataMatch.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.core.Metadata](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 34 =>
__requestHeadersToAdd += _root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.core.HeaderValueOption](_input__)
case 74 =>
__requestHeadersToRemove += _input__.readStringRequireUtf8()
case 42 =>
__responseHeadersToAdd += _root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.core.HeaderValueOption](_input__)
case 50 =>
__responseHeadersToRemove += _input__.readStringRequireUtf8()
case 66 =>
__perFilterConfig += io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_perFilterConfig.toCustom(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry](_input__))
case 82 =>
__typedPerFilterConfig += io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_typedPerFilterConfig.toCustom(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry](_input__))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight(
name = __name,
weight = __weight,
metadataMatch = __metadataMatch,
requestHeadersToAdd = __requestHeadersToAdd.result(),
requestHeadersToRemove = __requestHeadersToRemove.result(),
responseHeadersToAdd = __responseHeadersToAdd.result(),
responseHeadersToRemove = __responseHeadersToRemove.result(),
perFilterConfig = __perFilterConfig.result(),
typedPerFilterConfig = __typedPerFilterConfig.result(),
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight(
name = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
weight = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.wrappers.UInt32Value]]).map(io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_weight.toCustom(_)),
metadataMatch = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.api.v2.core.Metadata]]),
requestHeadersToAdd = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.api.v2.core.HeaderValueOption]]).getOrElse(_root_.scala.Seq.empty),
requestHeadersToRemove = __fieldsMap.get(scalaDescriptor.findFieldByNumber(9).get).map(_.as[_root_.scala.Seq[_root_.scala.Predef.String]]).getOrElse(_root_.scala.Seq.empty),
responseHeadersToAdd = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.api.v2.core.HeaderValueOption]]).getOrElse(_root_.scala.Seq.empty),
responseHeadersToRemove = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Seq[_root_.scala.Predef.String]]).getOrElse(_root_.scala.Seq.empty),
perFilterConfig = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry]]).getOrElse(_root_.scala.Seq.empty).iterator.map(io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_perFilterConfig.toCustom(_)).toMap,
typedPerFilterConfig = __fieldsMap.get(scalaDescriptor.findFieldByNumber(10).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry]]).getOrElse(_root_.scala.Seq.empty).iterator.map(io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight._typemapper_typedPerFilterConfig.toCustom(_)).toMap
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = io.envoyproxy.envoy.api.v2.route.WeightedCluster.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = io.envoyproxy.envoy.api.v2.route.WeightedCluster.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 2 => __out = com.google.protobuf.wrappers.UInt32Value
case 3 => __out = io.envoyproxy.envoy.api.v2.core.Metadata
case 4 => __out = io.envoyproxy.envoy.api.v2.core.HeaderValueOption
case 5 => __out = io.envoyproxy.envoy.api.v2.core.HeaderValueOption
case 8 => __out = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry
case 10 => __out = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry,
_root_.io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight(
name = "",
weight = _root_.scala.None,
metadataMatch = _root_.scala.None,
requestHeadersToAdd = _root_.scala.Seq.empty,
requestHeadersToRemove = _root_.scala.Seq.empty,
responseHeadersToAdd = _root_.scala.Seq.empty,
responseHeadersToRemove = _root_.scala.Seq.empty,
perFilterConfig = _root_.scala.collection.immutable.Map.empty,
typedPerFilterConfig = _root_.scala.collection.immutable.Map.empty
)
@SerialVersionUID(0L)
final case class PerFilterConfigEntry(
key: _root_.scala.Predef.String = "",
value: _root_.scala.Option[com.google.protobuf.struct.Struct] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[PerFilterConfigEntry] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = key
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
}
};
if (value.isDefined) {
val __value = value.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = key
if (!__v.isEmpty) {
_output__.writeString(1, __v)
}
};
value.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withKey(__v: _root_.scala.Predef.String): PerFilterConfigEntry = copy(key = __v)
def getValue: com.google.protobuf.struct.Struct = value.getOrElse(com.google.protobuf.struct.Struct.defaultInstance)
def clearValue: PerFilterConfigEntry = copy(value = _root_.scala.None)
def withValue(__v: com.google.protobuf.struct.Struct): PerFilterConfigEntry = copy(value = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = key
if (__t != "") __t else null
}
case 2 => value.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PString(key)
case 2 => value.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry.type = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry
// @@protoc_insertion_point(GeneratedMessage[envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry])
}
object PerFilterConfigEntry extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry = {
var __key: _root_.scala.Predef.String = ""
var __value: _root_.scala.Option[com.google.protobuf.struct.Struct] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__key = _input__.readStringRequireUtf8()
case 18 =>
__value = Option(__value.fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.struct.Struct](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry(
key = __key,
value = __value,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry(
key = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
value = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.struct.Struct]])
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 2 => __out = com.google.protobuf.struct.Struct
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry(
key = "",
value = _root_.scala.None
)
implicit class PerFilterConfigEntryLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry](_l) {
def key: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.key)((c_, f_) => c_.copy(key = f_))
def value: _root_.scalapb.lenses.Lens[UpperPB, com.google.protobuf.struct.Struct] = field(_.getValue)((c_, f_) => c_.copy(value = Option(f_)))
def optionalValue: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.protobuf.struct.Struct]] = field(_.value)((c_, f_) => c_.copy(value = f_))
}
final val KEY_FIELD_NUMBER = 1
final val VALUE_FIELD_NUMBER = 2
@transient
implicit val keyValueMapper: _root_.scalapb.TypeMapper[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry, (_root_.scala.Predef.String, com.google.protobuf.struct.Struct)] =
_root_.scalapb.TypeMapper[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry, (_root_.scala.Predef.String, com.google.protobuf.struct.Struct)](__m => (__m.key, __m.getValue))(__p => io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry(__p._1, Some(__p._2)))
def of(
key: _root_.scala.Predef.String,
value: _root_.scala.Option[com.google.protobuf.struct.Struct]
): _root_.io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry = _root_.io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry(
key,
value
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry])
}
@SerialVersionUID(0L)
final case class TypedPerFilterConfigEntry(
key: _root_.scala.Predef.String = "",
value: _root_.scala.Option[com.google.protobuf.any.Any] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[TypedPerFilterConfigEntry] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = key
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
}
};
if (value.isDefined) {
val __value = value.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = key
if (!__v.isEmpty) {
_output__.writeString(1, __v)
}
};
value.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withKey(__v: _root_.scala.Predef.String): TypedPerFilterConfigEntry = copy(key = __v)
def getValue: com.google.protobuf.any.Any = value.getOrElse(com.google.protobuf.any.Any.defaultInstance)
def clearValue: TypedPerFilterConfigEntry = copy(value = _root_.scala.None)
def withValue(__v: com.google.protobuf.any.Any): TypedPerFilterConfigEntry = copy(value = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = key
if (__t != "") __t else null
}
case 2 => value.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PString(key)
case 2 => value.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry.type = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry
// @@protoc_insertion_point(GeneratedMessage[envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry])
}
object TypedPerFilterConfigEntry extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry = {
var __key: _root_.scala.Predef.String = ""
var __value: _root_.scala.Option[com.google.protobuf.any.Any] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__key = _input__.readStringRequireUtf8()
case 18 =>
__value = Option(__value.fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.any.Any](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry(
key = __key,
value = __value,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry(
key = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
value = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.any.Any]])
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.javaDescriptor.getNestedTypes().get(1)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.scalaDescriptor.nestedMessages(1)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 2 => __out = com.google.protobuf.any.Any
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry(
key = "",
value = _root_.scala.None
)
implicit class TypedPerFilterConfigEntryLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry](_l) {
def key: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.key)((c_, f_) => c_.copy(key = f_))
def value: _root_.scalapb.lenses.Lens[UpperPB, com.google.protobuf.any.Any] = field(_.getValue)((c_, f_) => c_.copy(value = Option(f_)))
def optionalValue: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.protobuf.any.Any]] = field(_.value)((c_, f_) => c_.copy(value = f_))
}
final val KEY_FIELD_NUMBER = 1
final val VALUE_FIELD_NUMBER = 2
@transient
implicit val keyValueMapper: _root_.scalapb.TypeMapper[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry, (_root_.scala.Predef.String, com.google.protobuf.any.Any)] =
_root_.scalapb.TypeMapper[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry, (_root_.scala.Predef.String, com.google.protobuf.any.Any)](__m => (__m.key, __m.getValue))(__p => io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry(__p._1, Some(__p._2)))
def of(
key: _root_.scala.Predef.String,
value: _root_.scala.Option[com.google.protobuf.any.Any]
): _root_.io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry = _root_.io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry(
key,
value
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry])
}
implicit class ClusterWeightLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight](_l) {
def name: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.name)((c_, f_) => c_.copy(name = f_))
def weight: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.getWeight)((c_, f_) => c_.copy(weight = Option(f_)))
def optionalWeight: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[_root_.scala.Int]] = field(_.weight)((c_, f_) => c_.copy(weight = f_))
def metadataMatch: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.core.Metadata] = field(_.getMetadataMatch)((c_, f_) => c_.copy(metadataMatch = Option(f_)))
def optionalMetadataMatch: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[io.envoyproxy.envoy.api.v2.core.Metadata]] = field(_.metadataMatch)((c_, f_) => c_.copy(metadataMatch = f_))
def requestHeadersToAdd: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.envoyproxy.envoy.api.v2.core.HeaderValueOption]] = field(_.requestHeadersToAdd)((c_, f_) => c_.copy(requestHeadersToAdd = f_))
def requestHeadersToRemove: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[_root_.scala.Predef.String]] = field(_.requestHeadersToRemove)((c_, f_) => c_.copy(requestHeadersToRemove = f_))
def responseHeadersToAdd: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.envoyproxy.envoy.api.v2.core.HeaderValueOption]] = field(_.responseHeadersToAdd)((c_, f_) => c_.copy(responseHeadersToAdd = f_))
def responseHeadersToRemove: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[_root_.scala.Predef.String]] = field(_.responseHeadersToRemove)((c_, f_) => c_.copy(responseHeadersToRemove = f_))
def perFilterConfig: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, com.google.protobuf.struct.Struct]] = field(_.perFilterConfig)((c_, f_) => c_.copy(perFilterConfig = f_))
def typedPerFilterConfig: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, com.google.protobuf.any.Any]] = field(_.typedPerFilterConfig)((c_, f_) => c_.copy(typedPerFilterConfig = f_))
}
final val NAME_FIELD_NUMBER = 1
final val WEIGHT_FIELD_NUMBER = 2
final val METADATA_MATCH_FIELD_NUMBER = 3
final val REQUEST_HEADERS_TO_ADD_FIELD_NUMBER = 4
final val REQUEST_HEADERS_TO_REMOVE_FIELD_NUMBER = 9
final val RESPONSE_HEADERS_TO_ADD_FIELD_NUMBER = 5
final val RESPONSE_HEADERS_TO_REMOVE_FIELD_NUMBER = 6
final val PER_FILTER_CONFIG_FIELD_NUMBER = 8
final val TYPED_PER_FILTER_CONFIG_FIELD_NUMBER = 10
@transient
private[route] val _typemapper_weight: _root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int] = implicitly[_root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int]]
@transient
private[route] val _typemapper_perFilterConfig: _root_.scalapb.TypeMapper[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry, (_root_.scala.Predef.String, com.google.protobuf.struct.Struct)] = implicitly[_root_.scalapb.TypeMapper[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.PerFilterConfigEntry, (_root_.scala.Predef.String, com.google.protobuf.struct.Struct)]]
@transient
private[route] val _typemapper_typedPerFilterConfig: _root_.scalapb.TypeMapper[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry, (_root_.scala.Predef.String, com.google.protobuf.any.Any)] = implicitly[_root_.scalapb.TypeMapper[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight.TypedPerFilterConfigEntry, (_root_.scala.Predef.String, com.google.protobuf.any.Any)]]
def of(
name: _root_.scala.Predef.String,
weight: _root_.scala.Option[_root_.scala.Int],
metadataMatch: _root_.scala.Option[io.envoyproxy.envoy.api.v2.core.Metadata],
requestHeadersToAdd: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.core.HeaderValueOption],
requestHeadersToRemove: _root_.scala.Seq[_root_.scala.Predef.String],
responseHeadersToAdd: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.core.HeaderValueOption],
responseHeadersToRemove: _root_.scala.Seq[_root_.scala.Predef.String],
perFilterConfig: _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, com.google.protobuf.struct.Struct],
typedPerFilterConfig: _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, com.google.protobuf.any.Any]
): _root_.io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight = _root_.io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight(
name,
weight,
metadataMatch,
requestHeadersToAdd,
requestHeadersToRemove,
responseHeadersToAdd,
responseHeadersToRemove,
perFilterConfig,
typedPerFilterConfig
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.api.v2.route.WeightedCluster.ClusterWeight])
}
implicit class WeightedClusterLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.api.v2.route.WeightedCluster]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.api.v2.route.WeightedCluster](_l) {
def clusters: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight]] = field(_.clusters)((c_, f_) => c_.copy(clusters = f_))
def totalWeight: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.getTotalWeight)((c_, f_) => c_.copy(totalWeight = Option(f_)))
def optionalTotalWeight: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[_root_.scala.Int]] = field(_.totalWeight)((c_, f_) => c_.copy(totalWeight = f_))
def runtimeKeyPrefix: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.runtimeKeyPrefix)((c_, f_) => c_.copy(runtimeKeyPrefix = f_))
}
final val CLUSTERS_FIELD_NUMBER = 1
final val TOTAL_WEIGHT_FIELD_NUMBER = 3
final val RUNTIME_KEY_PREFIX_FIELD_NUMBER = 2
@transient
private[route] val _typemapper_totalWeight: _root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int] = implicitly[_root_.scalapb.TypeMapper[com.google.protobuf.wrappers.UInt32Value, _root_.scala.Int]]
def of(
clusters: _root_.scala.Seq[io.envoyproxy.envoy.api.v2.route.WeightedCluster.ClusterWeight],
totalWeight: _root_.scala.Option[_root_.scala.Int],
runtimeKeyPrefix: _root_.scala.Predef.String
): _root_.io.envoyproxy.envoy.api.v2.route.WeightedCluster = _root_.io.envoyproxy.envoy.api.v2.route.WeightedCluster(
clusters,
totalWeight,
runtimeKeyPrefix
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.api.v2.route.WeightedCluster])
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy