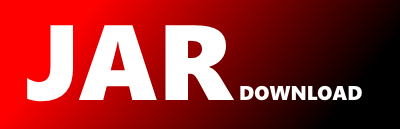
io.envoyproxy.envoy.config.bootstrap.v3.Admin.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envoy-scala-control-plane_2.13 Show documentation
Show all versions of envoy-scala-control-plane_2.13 Show documentation
ScalaPB generated bindings for Envoy
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package io.envoyproxy.envoy.config.bootstrap.v3
/** Administration interface :ref:`operations documentation
* <operations_admin_interface>`.
* [#next-free-field: 7]
*
* @param accessLog
* Configuration for :ref:`access logs <arch_overview_access_logs>`
* emitted by the administration server.
* @param accessLogPath
* The path to write the access log for the administration server. If no
* access log is desired specify ‘/dev/null’. This is only required if
* :ref:`address <envoy_v3_api_field_config.bootstrap.v3.Admin.address>` is set.
* Deprecated in favor of *access_log* which offers more options.
* @param profilePath
* The cpu profiler output path for the administration server. If no profile
* path is specified, the default is ‘/var/log/envoy/envoy.prof’.
* @param address
* The TCP address that the administration server will listen on.
* If not specified, Envoy will not start an administration server.
* @param socketOptions
* Additional socket options that may not be present in Envoy source code or
* precompiled binaries.
* @param ignoreGlobalConnLimit
* Indicates whether :ref:`global_downstream_max_connections <config_overload_manager_limiting_connections>`
* should apply to the admin interface or not.
*/
@SerialVersionUID(0L)
final case class Admin(
accessLog: _root_.scala.Seq[io.envoyproxy.envoy.config.accesslog.v3.AccessLog] = _root_.scala.Seq.empty,
@scala.deprecated(message="Marked as deprecated in proto file", "") accessLogPath: _root_.scala.Predef.String = "",
profilePath: _root_.scala.Predef.String = "",
address: _root_.scala.Option[io.envoyproxy.envoy.config.core.v3.Address] = _root_.scala.None,
socketOptions: _root_.scala.Seq[io.envoyproxy.envoy.config.core.v3.SocketOption] = _root_.scala.Seq.empty,
ignoreGlobalConnLimit: _root_.scala.Boolean = false,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Admin] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
accessLog.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = accessLogPath
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
}
};
{
val __value = profilePath
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(2, __value)
}
};
if (address.isDefined) {
val __value = address.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
socketOptions.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = ignoreGlobalConnLimit
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(6, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = accessLogPath
if (!__v.isEmpty) {
_output__.writeString(1, __v)
}
};
{
val __v = profilePath
if (!__v.isEmpty) {
_output__.writeString(2, __v)
}
};
address.foreach { __v =>
val __m = __v
_output__.writeTag(3, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
socketOptions.foreach { __v =>
val __m = __v
_output__.writeTag(4, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
accessLog.foreach { __v =>
val __m = __v
_output__.writeTag(5, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = ignoreGlobalConnLimit
if (__v != false) {
_output__.writeBool(6, __v)
}
};
unknownFields.writeTo(_output__)
}
def clearAccessLog = copy(accessLog = _root_.scala.Seq.empty)
def addAccessLog(__vs: io.envoyproxy.envoy.config.accesslog.v3.AccessLog *): Admin = addAllAccessLog(__vs)
def addAllAccessLog(__vs: Iterable[io.envoyproxy.envoy.config.accesslog.v3.AccessLog]): Admin = copy(accessLog = accessLog ++ __vs)
def withAccessLog(__v: _root_.scala.Seq[io.envoyproxy.envoy.config.accesslog.v3.AccessLog]): Admin = copy(accessLog = __v)
def withAccessLogPath(__v: _root_.scala.Predef.String): Admin = copy(accessLogPath = __v)
def withProfilePath(__v: _root_.scala.Predef.String): Admin = copy(profilePath = __v)
def getAddress: io.envoyproxy.envoy.config.core.v3.Address = address.getOrElse(io.envoyproxy.envoy.config.core.v3.Address.defaultInstance)
def clearAddress: Admin = copy(address = _root_.scala.None)
def withAddress(__v: io.envoyproxy.envoy.config.core.v3.Address): Admin = copy(address = Option(__v))
def clearSocketOptions = copy(socketOptions = _root_.scala.Seq.empty)
def addSocketOptions(__vs: io.envoyproxy.envoy.config.core.v3.SocketOption *): Admin = addAllSocketOptions(__vs)
def addAllSocketOptions(__vs: Iterable[io.envoyproxy.envoy.config.core.v3.SocketOption]): Admin = copy(socketOptions = socketOptions ++ __vs)
def withSocketOptions(__v: _root_.scala.Seq[io.envoyproxy.envoy.config.core.v3.SocketOption]): Admin = copy(socketOptions = __v)
def withIgnoreGlobalConnLimit(__v: _root_.scala.Boolean): Admin = copy(ignoreGlobalConnLimit = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 5 => accessLog
case 1 => {
val __t = accessLogPath
if (__t != "") __t else null
}
case 2 => {
val __t = profilePath
if (__t != "") __t else null
}
case 3 => address.orNull
case 4 => socketOptions
case 6 => {
val __t = ignoreGlobalConnLimit
if (__t != false) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 5 => _root_.scalapb.descriptors.PRepeated(accessLog.iterator.map(_.toPMessage).toVector)
case 1 => _root_.scalapb.descriptors.PString(accessLogPath)
case 2 => _root_.scalapb.descriptors.PString(profilePath)
case 3 => address.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 4 => _root_.scalapb.descriptors.PRepeated(socketOptions.iterator.map(_.toPMessage).toVector)
case 6 => _root_.scalapb.descriptors.PBoolean(ignoreGlobalConnLimit)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.config.bootstrap.v3.Admin.type = io.envoyproxy.envoy.config.bootstrap.v3.Admin
// @@protoc_insertion_point(GeneratedMessage[envoy.config.bootstrap.v3.Admin])
}
object Admin extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.config.bootstrap.v3.Admin] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.config.bootstrap.v3.Admin] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.config.bootstrap.v3.Admin = {
val __accessLog: _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.config.accesslog.v3.AccessLog] = new _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.config.accesslog.v3.AccessLog]
var __accessLogPath: _root_.scala.Predef.String = ""
var __profilePath: _root_.scala.Predef.String = ""
var __address: _root_.scala.Option[io.envoyproxy.envoy.config.core.v3.Address] = _root_.scala.None
val __socketOptions: _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.config.core.v3.SocketOption] = new _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.config.core.v3.SocketOption]
var __ignoreGlobalConnLimit: _root_.scala.Boolean = false
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 42 =>
__accessLog += _root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.config.accesslog.v3.AccessLog](_input__)
case 10 =>
__accessLogPath = _input__.readStringRequireUtf8()
case 18 =>
__profilePath = _input__.readStringRequireUtf8()
case 26 =>
__address = Option(__address.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.config.core.v3.Address](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 34 =>
__socketOptions += _root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.config.core.v3.SocketOption](_input__)
case 48 =>
__ignoreGlobalConnLimit = _input__.readBool()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.config.bootstrap.v3.Admin(
accessLog = __accessLog.result(),
accessLogPath = __accessLogPath,
profilePath = __profilePath,
address = __address,
socketOptions = __socketOptions.result(),
ignoreGlobalConnLimit = __ignoreGlobalConnLimit,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.config.bootstrap.v3.Admin] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.config.bootstrap.v3.Admin(
accessLog = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.config.accesslog.v3.AccessLog]]).getOrElse(_root_.scala.Seq.empty),
accessLogPath = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
profilePath = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
address = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.config.core.v3.Address]]),
socketOptions = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.config.core.v3.SocketOption]]).getOrElse(_root_.scala.Seq.empty),
ignoreGlobalConnLimit = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Boolean]).getOrElse(false)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = BootstrapProto.javaDescriptor.getMessageTypes().get(1)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = BootstrapProto.scalaDescriptor.messages(1)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 5 => __out = io.envoyproxy.envoy.config.accesslog.v3.AccessLog
case 3 => __out = io.envoyproxy.envoy.config.core.v3.Address
case 4 => __out = io.envoyproxy.envoy.config.core.v3.SocketOption
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.envoyproxy.envoy.config.bootstrap.v3.Admin(
accessLog = _root_.scala.Seq.empty,
accessLogPath = "",
profilePath = "",
address = _root_.scala.None,
socketOptions = _root_.scala.Seq.empty,
ignoreGlobalConnLimit = false
)
implicit class AdminLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.config.bootstrap.v3.Admin]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.config.bootstrap.v3.Admin](_l) {
def accessLog: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.envoyproxy.envoy.config.accesslog.v3.AccessLog]] = field(_.accessLog)((c_, f_) => c_.copy(accessLog = f_))
def accessLogPath: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.accessLogPath)((c_, f_) => c_.copy(accessLogPath = f_))
def profilePath: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.profilePath)((c_, f_) => c_.copy(profilePath = f_))
def address: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.config.core.v3.Address] = field(_.getAddress)((c_, f_) => c_.copy(address = Option(f_)))
def optionalAddress: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[io.envoyproxy.envoy.config.core.v3.Address]] = field(_.address)((c_, f_) => c_.copy(address = f_))
def socketOptions: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.envoyproxy.envoy.config.core.v3.SocketOption]] = field(_.socketOptions)((c_, f_) => c_.copy(socketOptions = f_))
def ignoreGlobalConnLimit: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.ignoreGlobalConnLimit)((c_, f_) => c_.copy(ignoreGlobalConnLimit = f_))
}
final val ACCESS_LOG_FIELD_NUMBER = 5
final val ACCESS_LOG_PATH_FIELD_NUMBER = 1
final val PROFILE_PATH_FIELD_NUMBER = 2
final val ADDRESS_FIELD_NUMBER = 3
final val SOCKET_OPTIONS_FIELD_NUMBER = 4
final val IGNORE_GLOBAL_CONN_LIMIT_FIELD_NUMBER = 6
def of(
accessLog: _root_.scala.Seq[io.envoyproxy.envoy.config.accesslog.v3.AccessLog],
accessLogPath: _root_.scala.Predef.String,
profilePath: _root_.scala.Predef.String,
address: _root_.scala.Option[io.envoyproxy.envoy.config.core.v3.Address],
socketOptions: _root_.scala.Seq[io.envoyproxy.envoy.config.core.v3.SocketOption],
ignoreGlobalConnLimit: _root_.scala.Boolean
): _root_.io.envoyproxy.envoy.config.bootstrap.v3.Admin = _root_.io.envoyproxy.envoy.config.bootstrap.v3.Admin(
accessLog,
accessLogPath,
profilePath,
address,
socketOptions,
ignoreGlobalConnLimit
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.config.bootstrap.v3.Admin])
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy