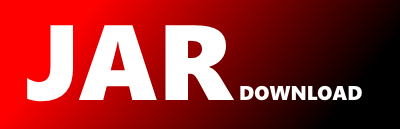
io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envoy-scala-control-plane_2.13 Show documentation
Show all versions of envoy-scala-control-plane_2.13 Show documentation
ScalaPB generated bindings for Envoy
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package io.envoyproxy.envoy.config.bootstrap.v3
/** Envoy process watchdog configuration. When configured, this monitors for
* nonresponsive threads and kills the process after the configured thresholds.
* See the :ref:`watchdog documentation <operations_performance_watchdog>` for more information.
* [#next-free-field: 8]
*
* @param actions
* Register actions that will fire on given WatchDog events.
* See *WatchDogAction* for priority of events.
* @param missTimeout
* The duration after which Envoy counts a nonresponsive thread in the
* *watchdog_miss* statistic. If not specified the default is 200ms.
* @param megamissTimeout
* The duration after which Envoy counts a nonresponsive thread in the
* *watchdog_mega_miss* statistic. If not specified the default is
* 1000ms.
* @param killTimeout
* If a watched thread has been nonresponsive for this duration, assume a
* programming error and kill the entire Envoy process. Set to 0 to disable
* kill behavior. If not specified the default is 0 (disabled).
* @param maxKillTimeoutJitter
* Defines the maximum jitter used to adjust the *kill_timeout* if *kill_timeout* is
* enabled. Enabling this feature would help to reduce risk of synchronized
* watchdog kill events across proxies due to external triggers. Set to 0 to
* disable. If not specified the default is 0 (disabled).
* @param multikillTimeout
* If max(2, ceil(registered_threads * Fraction(*multikill_threshold*)))
* threads have been nonresponsive for at least this duration kill the entire
* Envoy process. Set to 0 to disable this behavior. If not specified the
* default is 0 (disabled).
* @param multikillThreshold
* Sets the threshold for *multikill_timeout* in terms of the percentage of
* nonresponsive threads required for the *multikill_timeout*.
* If not specified the default is 0.
*/
@SerialVersionUID(0L)
final case class Watchdog(
actions: _root_.scala.Seq[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction] = _root_.scala.Seq.empty,
missTimeout: _root_.scala.Option[com.google.protobuf.duration.Duration] = _root_.scala.None,
megamissTimeout: _root_.scala.Option[com.google.protobuf.duration.Duration] = _root_.scala.None,
killTimeout: _root_.scala.Option[com.google.protobuf.duration.Duration] = _root_.scala.None,
maxKillTimeoutJitter: _root_.scala.Option[com.google.protobuf.duration.Duration] = _root_.scala.None,
multikillTimeout: _root_.scala.Option[com.google.protobuf.duration.Duration] = _root_.scala.None,
multikillThreshold: _root_.scala.Option[io.envoyproxy.envoy.`type`.v3.Percent] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Watchdog] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
actions.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
if (missTimeout.isDefined) {
val __value = missTimeout.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (megamissTimeout.isDefined) {
val __value = megamissTimeout.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (killTimeout.isDefined) {
val __value = killTimeout.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (maxKillTimeoutJitter.isDefined) {
val __value = maxKillTimeoutJitter.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (multikillTimeout.isDefined) {
val __value = multikillTimeout.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (multikillThreshold.isDefined) {
val __value = multikillThreshold.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
missTimeout.foreach { __v =>
val __m = __v
_output__.writeTag(1, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
megamissTimeout.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
killTimeout.foreach { __v =>
val __m = __v
_output__.writeTag(3, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
multikillTimeout.foreach { __v =>
val __m = __v
_output__.writeTag(4, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
multikillThreshold.foreach { __v =>
val __m = __v
_output__.writeTag(5, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
maxKillTimeoutJitter.foreach { __v =>
val __m = __v
_output__.writeTag(6, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
actions.foreach { __v =>
val __m = __v
_output__.writeTag(7, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def clearActions = copy(actions = _root_.scala.Seq.empty)
def addActions(__vs: io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction *): Watchdog = addAllActions(__vs)
def addAllActions(__vs: Iterable[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction]): Watchdog = copy(actions = actions ++ __vs)
def withActions(__v: _root_.scala.Seq[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction]): Watchdog = copy(actions = __v)
def getMissTimeout: com.google.protobuf.duration.Duration = missTimeout.getOrElse(com.google.protobuf.duration.Duration.defaultInstance)
def clearMissTimeout: Watchdog = copy(missTimeout = _root_.scala.None)
def withMissTimeout(__v: com.google.protobuf.duration.Duration): Watchdog = copy(missTimeout = Option(__v))
def getMegamissTimeout: com.google.protobuf.duration.Duration = megamissTimeout.getOrElse(com.google.protobuf.duration.Duration.defaultInstance)
def clearMegamissTimeout: Watchdog = copy(megamissTimeout = _root_.scala.None)
def withMegamissTimeout(__v: com.google.protobuf.duration.Duration): Watchdog = copy(megamissTimeout = Option(__v))
def getKillTimeout: com.google.protobuf.duration.Duration = killTimeout.getOrElse(com.google.protobuf.duration.Duration.defaultInstance)
def clearKillTimeout: Watchdog = copy(killTimeout = _root_.scala.None)
def withKillTimeout(__v: com.google.protobuf.duration.Duration): Watchdog = copy(killTimeout = Option(__v))
def getMaxKillTimeoutJitter: com.google.protobuf.duration.Duration = maxKillTimeoutJitter.getOrElse(com.google.protobuf.duration.Duration.defaultInstance)
def clearMaxKillTimeoutJitter: Watchdog = copy(maxKillTimeoutJitter = _root_.scala.None)
def withMaxKillTimeoutJitter(__v: com.google.protobuf.duration.Duration): Watchdog = copy(maxKillTimeoutJitter = Option(__v))
def getMultikillTimeout: com.google.protobuf.duration.Duration = multikillTimeout.getOrElse(com.google.protobuf.duration.Duration.defaultInstance)
def clearMultikillTimeout: Watchdog = copy(multikillTimeout = _root_.scala.None)
def withMultikillTimeout(__v: com.google.protobuf.duration.Duration): Watchdog = copy(multikillTimeout = Option(__v))
def getMultikillThreshold: io.envoyproxy.envoy.`type`.v3.Percent = multikillThreshold.getOrElse(io.envoyproxy.envoy.`type`.v3.Percent.defaultInstance)
def clearMultikillThreshold: Watchdog = copy(multikillThreshold = _root_.scala.None)
def withMultikillThreshold(__v: io.envoyproxy.envoy.`type`.v3.Percent): Watchdog = copy(multikillThreshold = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 7 => actions
case 1 => missTimeout.orNull
case 2 => megamissTimeout.orNull
case 3 => killTimeout.orNull
case 6 => maxKillTimeoutJitter.orNull
case 4 => multikillTimeout.orNull
case 5 => multikillThreshold.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 7 => _root_.scalapb.descriptors.PRepeated(actions.iterator.map(_.toPMessage).toVector)
case 1 => missTimeout.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 2 => megamissTimeout.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 3 => killTimeout.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 6 => maxKillTimeoutJitter.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 4 => multikillTimeout.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 5 => multikillThreshold.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.type = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog
// @@protoc_insertion_point(GeneratedMessage[envoy.config.bootstrap.v3.Watchdog])
}
object Watchdog extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.config.bootstrap.v3.Watchdog = {
val __actions: _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction] = new _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction]
var __missTimeout: _root_.scala.Option[com.google.protobuf.duration.Duration] = _root_.scala.None
var __megamissTimeout: _root_.scala.Option[com.google.protobuf.duration.Duration] = _root_.scala.None
var __killTimeout: _root_.scala.Option[com.google.protobuf.duration.Duration] = _root_.scala.None
var __maxKillTimeoutJitter: _root_.scala.Option[com.google.protobuf.duration.Duration] = _root_.scala.None
var __multikillTimeout: _root_.scala.Option[com.google.protobuf.duration.Duration] = _root_.scala.None
var __multikillThreshold: _root_.scala.Option[io.envoyproxy.envoy.`type`.v3.Percent] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 58 =>
__actions += _root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction](_input__)
case 10 =>
__missTimeout = Option(__missTimeout.fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.duration.Duration](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 18 =>
__megamissTimeout = Option(__megamissTimeout.fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.duration.Duration](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 26 =>
__killTimeout = Option(__killTimeout.fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.duration.Duration](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 50 =>
__maxKillTimeoutJitter = Option(__maxKillTimeoutJitter.fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.duration.Duration](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 34 =>
__multikillTimeout = Option(__multikillTimeout.fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.duration.Duration](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 42 =>
__multikillThreshold = Option(__multikillThreshold.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.`type`.v3.Percent](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.config.bootstrap.v3.Watchdog(
actions = __actions.result(),
missTimeout = __missTimeout,
megamissTimeout = __megamissTimeout,
killTimeout = __killTimeout,
maxKillTimeoutJitter = __maxKillTimeoutJitter,
multikillTimeout = __multikillTimeout,
multikillThreshold = __multikillThreshold,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.config.bootstrap.v3.Watchdog(
actions = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction]]).getOrElse(_root_.scala.Seq.empty),
missTimeout = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.duration.Duration]]),
megamissTimeout = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.duration.Duration]]),
killTimeout = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.duration.Duration]]),
maxKillTimeoutJitter = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.duration.Duration]]),
multikillTimeout = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.duration.Duration]]),
multikillThreshold = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.`type`.v3.Percent]])
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = BootstrapProto.javaDescriptor.getMessageTypes().get(4)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = BootstrapProto.scalaDescriptor.messages(4)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 7 => __out = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction
case 1 => __out = com.google.protobuf.duration.Duration
case 2 => __out = com.google.protobuf.duration.Duration
case 3 => __out = com.google.protobuf.duration.Duration
case 6 => __out = com.google.protobuf.duration.Duration
case 4 => __out = com.google.protobuf.duration.Duration
case 5 => __out = io.envoyproxy.envoy.`type`.v3.Percent
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog(
actions = _root_.scala.Seq.empty,
missTimeout = _root_.scala.None,
megamissTimeout = _root_.scala.None,
killTimeout = _root_.scala.None,
maxKillTimeoutJitter = _root_.scala.None,
multikillTimeout = _root_.scala.None,
multikillThreshold = _root_.scala.None
)
/** @param config
* Extension specific configuration for the action.
*/
@SerialVersionUID(0L)
final case class WatchdogAction(
config: _root_.scala.Option[io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig] = _root_.scala.None,
event: io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent.UNKNOWN,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[WatchdogAction] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
if (config.isDefined) {
val __value = config.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
{
val __value = event.value
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeEnumSize(2, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
config.foreach { __v =>
val __m = __v
_output__.writeTag(1, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = event.value
if (__v != 0) {
_output__.writeEnum(2, __v)
}
};
unknownFields.writeTo(_output__)
}
def getConfig: io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig = config.getOrElse(io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig.defaultInstance)
def clearConfig: WatchdogAction = copy(config = _root_.scala.None)
def withConfig(__v: io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig): WatchdogAction = copy(config = Option(__v))
def withEvent(__v: io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent): WatchdogAction = copy(event = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => config.orNull
case 2 => {
val __t = event.javaValueDescriptor
if (__t.getNumber() != 0) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => config.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 2 => _root_.scalapb.descriptors.PEnum(event.scalaValueDescriptor)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.type = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction
// @@protoc_insertion_point(GeneratedMessage[envoy.config.bootstrap.v3.Watchdog.WatchdogAction])
}
object WatchdogAction extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction = {
var __config: _root_.scala.Option[io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig] = _root_.scala.None
var __event: io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent.UNKNOWN
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__config = Option(__config.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 16 =>
__event = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent.fromValue(_input__.readEnum())
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction(
config = __config,
event = __event,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction(
config = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig]]),
event = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent.fromValue(__fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scalapb.descriptors.EnumValueDescriptor]).getOrElse(io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent.UNKNOWN.scalaValueDescriptor).number)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 1 => __out = io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 2 => io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent
}
}
lazy val defaultInstance = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction(
config = _root_.scala.None,
event = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent.UNKNOWN
)
/** The events are fired in this order: KILL, MULTIKILL, MEGAMISS, MISS.
* Within an event type, actions execute in the order they are configured.
* For KILL/MULTIKILL there is a default PANIC that will run after the
* registered actions and kills the process if it wasn't already killed.
* It might be useful to specify several debug actions, and possibly an
* alternate FATAL action.
*/
sealed abstract class WatchdogEvent(val value: _root_.scala.Int) extends _root_.scalapb.GeneratedEnum {
type EnumType = WatchdogEvent
def isUnknown: _root_.scala.Boolean = false
def isKill: _root_.scala.Boolean = false
def isMultikill: _root_.scala.Boolean = false
def isMegamiss: _root_.scala.Boolean = false
def isMiss: _root_.scala.Boolean = false
def companion: _root_.scalapb.GeneratedEnumCompanion[WatchdogEvent] = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent
final def asRecognized: _root_.scala.Option[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent.Recognized] = if (isUnrecognized) _root_.scala.None else _root_.scala.Some(this.asInstanceOf[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent.Recognized])
}
object WatchdogEvent extends _root_.scalapb.GeneratedEnumCompanion[WatchdogEvent] {
sealed trait Recognized extends WatchdogEvent
implicit def enumCompanion: _root_.scalapb.GeneratedEnumCompanion[WatchdogEvent] = this
@SerialVersionUID(0L)
case object UNKNOWN extends WatchdogEvent(0) with WatchdogEvent.Recognized {
val index = 0
val name = "UNKNOWN"
override def isUnknown: _root_.scala.Boolean = true
}
@SerialVersionUID(0L)
case object KILL extends WatchdogEvent(1) with WatchdogEvent.Recognized {
val index = 1
val name = "KILL"
override def isKill: _root_.scala.Boolean = true
}
@SerialVersionUID(0L)
case object MULTIKILL extends WatchdogEvent(2) with WatchdogEvent.Recognized {
val index = 2
val name = "MULTIKILL"
override def isMultikill: _root_.scala.Boolean = true
}
@SerialVersionUID(0L)
case object MEGAMISS extends WatchdogEvent(3) with WatchdogEvent.Recognized {
val index = 3
val name = "MEGAMISS"
override def isMegamiss: _root_.scala.Boolean = true
}
@SerialVersionUID(0L)
case object MISS extends WatchdogEvent(4) with WatchdogEvent.Recognized {
val index = 4
val name = "MISS"
override def isMiss: _root_.scala.Boolean = true
}
@SerialVersionUID(0L)
final case class Unrecognized(unrecognizedValue: _root_.scala.Int) extends WatchdogEvent(unrecognizedValue) with _root_.scalapb.UnrecognizedEnum
lazy val values = scala.collection.immutable.Seq(UNKNOWN, KILL, MULTIKILL, MEGAMISS, MISS)
def fromValue(__value: _root_.scala.Int): WatchdogEvent = __value match {
case 0 => UNKNOWN
case 1 => KILL
case 2 => MULTIKILL
case 3 => MEGAMISS
case 4 => MISS
case __other => Unrecognized(__other)
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.EnumDescriptor = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.javaDescriptor.getEnumTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.EnumDescriptor = io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.scalaDescriptor.enums(0)
}
implicit class WatchdogActionLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction](_l) {
def config: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig] = field(_.getConfig)((c_, f_) => c_.copy(config = Option(f_)))
def optionalConfig: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig]] = field(_.config)((c_, f_) => c_.copy(config = f_))
def event: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent] = field(_.event)((c_, f_) => c_.copy(event = f_))
}
final val CONFIG_FIELD_NUMBER = 1
final val EVENT_FIELD_NUMBER = 2
def of(
config: _root_.scala.Option[io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig],
event: io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction.WatchdogEvent
): _root_.io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction = _root_.io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction(
config,
event
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.config.bootstrap.v3.Watchdog.WatchdogAction])
}
implicit class WatchdogLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.config.bootstrap.v3.Watchdog]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.config.bootstrap.v3.Watchdog](_l) {
def actions: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction]] = field(_.actions)((c_, f_) => c_.copy(actions = f_))
def missTimeout: _root_.scalapb.lenses.Lens[UpperPB, com.google.protobuf.duration.Duration] = field(_.getMissTimeout)((c_, f_) => c_.copy(missTimeout = Option(f_)))
def optionalMissTimeout: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.protobuf.duration.Duration]] = field(_.missTimeout)((c_, f_) => c_.copy(missTimeout = f_))
def megamissTimeout: _root_.scalapb.lenses.Lens[UpperPB, com.google.protobuf.duration.Duration] = field(_.getMegamissTimeout)((c_, f_) => c_.copy(megamissTimeout = Option(f_)))
def optionalMegamissTimeout: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.protobuf.duration.Duration]] = field(_.megamissTimeout)((c_, f_) => c_.copy(megamissTimeout = f_))
def killTimeout: _root_.scalapb.lenses.Lens[UpperPB, com.google.protobuf.duration.Duration] = field(_.getKillTimeout)((c_, f_) => c_.copy(killTimeout = Option(f_)))
def optionalKillTimeout: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.protobuf.duration.Duration]] = field(_.killTimeout)((c_, f_) => c_.copy(killTimeout = f_))
def maxKillTimeoutJitter: _root_.scalapb.lenses.Lens[UpperPB, com.google.protobuf.duration.Duration] = field(_.getMaxKillTimeoutJitter)((c_, f_) => c_.copy(maxKillTimeoutJitter = Option(f_)))
def optionalMaxKillTimeoutJitter: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.protobuf.duration.Duration]] = field(_.maxKillTimeoutJitter)((c_, f_) => c_.copy(maxKillTimeoutJitter = f_))
def multikillTimeout: _root_.scalapb.lenses.Lens[UpperPB, com.google.protobuf.duration.Duration] = field(_.getMultikillTimeout)((c_, f_) => c_.copy(multikillTimeout = Option(f_)))
def optionalMultikillTimeout: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.protobuf.duration.Duration]] = field(_.multikillTimeout)((c_, f_) => c_.copy(multikillTimeout = f_))
def multikillThreshold: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.`type`.v3.Percent] = field(_.getMultikillThreshold)((c_, f_) => c_.copy(multikillThreshold = Option(f_)))
def optionalMultikillThreshold: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[io.envoyproxy.envoy.`type`.v3.Percent]] = field(_.multikillThreshold)((c_, f_) => c_.copy(multikillThreshold = f_))
}
final val ACTIONS_FIELD_NUMBER = 7
final val MISS_TIMEOUT_FIELD_NUMBER = 1
final val MEGAMISS_TIMEOUT_FIELD_NUMBER = 2
final val KILL_TIMEOUT_FIELD_NUMBER = 3
final val MAX_KILL_TIMEOUT_JITTER_FIELD_NUMBER = 6
final val MULTIKILL_TIMEOUT_FIELD_NUMBER = 4
final val MULTIKILL_THRESHOLD_FIELD_NUMBER = 5
def of(
actions: _root_.scala.Seq[io.envoyproxy.envoy.config.bootstrap.v3.Watchdog.WatchdogAction],
missTimeout: _root_.scala.Option[com.google.protobuf.duration.Duration],
megamissTimeout: _root_.scala.Option[com.google.protobuf.duration.Duration],
killTimeout: _root_.scala.Option[com.google.protobuf.duration.Duration],
maxKillTimeoutJitter: _root_.scala.Option[com.google.protobuf.duration.Duration],
multikillTimeout: _root_.scala.Option[com.google.protobuf.duration.Duration],
multikillThreshold: _root_.scala.Option[io.envoyproxy.envoy.`type`.v3.Percent]
): _root_.io.envoyproxy.envoy.config.bootstrap.v3.Watchdog = _root_.io.envoyproxy.envoy.config.bootstrap.v3.Watchdog(
actions,
missTimeout,
megamissTimeout,
killTimeout,
maxKillTimeoutJitter,
multikillTimeout,
multikillThreshold
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.config.bootstrap.v3.Watchdog])
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy