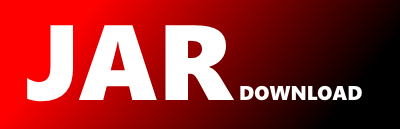
io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envoy-scala-control-plane_2.13 Show documentation
Show all versions of envoy-scala-control-plane_2.13 Show documentation
ScalaPB generated bindings for Envoy
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package io.envoyproxy.envoy.config.tap.v3
/** HTTP generic body match configuration.
* List of text strings and hex strings to be located in HTTP body.
* All specified strings must be found in the HTTP body for positive match.
* The search may be limited to specified number of bytes from the body start.
*
* .. attention::
*
* Searching for patterns in HTTP body is potentially cpu intensive. For each specified pattern, http body is scanned byte by byte to find a match.
* If multiple patterns are specified, the process is repeated for each pattern. If location of a pattern is known, ``bytes_limit`` should be specified
* to scan only part of the http body.
*
* @param bytesLimit
* Limits search to specified number of bytes - default zero (no limit - match entire captured buffer).
* @param patterns
* List of patterns to match.
*/
@SerialVersionUID(0L)
final case class HttpGenericBodyMatch(
bytesLimit: _root_.scala.Int = 0,
patterns: _root_.scala.Seq[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch] = _root_.scala.Seq.empty,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[HttpGenericBodyMatch] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = bytesLimit
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt32Size(1, __value)
}
};
patterns.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = bytesLimit
if (__v != 0) {
_output__.writeUInt32(1, __v)
}
};
patterns.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withBytesLimit(__v: _root_.scala.Int): HttpGenericBodyMatch = copy(bytesLimit = __v)
def clearPatterns = copy(patterns = _root_.scala.Seq.empty)
def addPatterns(__vs: io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch *): HttpGenericBodyMatch = addAllPatterns(__vs)
def addAllPatterns(__vs: Iterable[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch]): HttpGenericBodyMatch = copy(patterns = patterns ++ __vs)
def withPatterns(__v: _root_.scala.Seq[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch]): HttpGenericBodyMatch = copy(patterns = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = bytesLimit
if (__t != 0) __t else null
}
case 2 => patterns
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PInt(bytesLimit)
case 2 => _root_.scalapb.descriptors.PRepeated(patterns.iterator.map(_.toPMessage).toVector)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.type = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch
// @@protoc_insertion_point(GeneratedMessage[envoy.config.tap.v3.HttpGenericBodyMatch])
}
object HttpGenericBodyMatch extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch = {
var __bytesLimit: _root_.scala.Int = 0
val __patterns: _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch] = new _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch]
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 8 =>
__bytesLimit = _input__.readUInt32()
case 18 =>
__patterns += _root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch](_input__)
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch(
bytesLimit = __bytesLimit,
patterns = __patterns.result(),
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch(
bytesLimit = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Int]).getOrElse(0),
patterns = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch]]).getOrElse(_root_.scala.Seq.empty)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = CommonProto.javaDescriptor.getMessageTypes().get(3)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = CommonProto.scalaDescriptor.messages(3)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 2 => __out = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch(
bytesLimit = 0,
patterns = _root_.scala.Seq.empty
)
@SerialVersionUID(0L)
final case class GenericTextMatch(
rule: io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule.Empty,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[GenericTextMatch] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
if (rule.stringMatch.isDefined) {
val __value = rule.stringMatch.get
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
};
if (rule.binaryMatch.isDefined) {
val __value = rule.binaryMatch.get
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(2, __value)
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
rule.stringMatch.foreach { __v =>
val __m = __v
_output__.writeString(1, __m)
};
rule.binaryMatch.foreach { __v =>
val __m = __v
_output__.writeBytes(2, __m)
};
unknownFields.writeTo(_output__)
}
def getStringMatch: _root_.scala.Predef.String = rule.stringMatch.getOrElse("")
def withStringMatch(__v: _root_.scala.Predef.String): GenericTextMatch = copy(rule = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule.StringMatch(__v))
def getBinaryMatch: _root_.com.google.protobuf.ByteString = rule.binaryMatch.getOrElse(_root_.com.google.protobuf.ByteString.EMPTY)
def withBinaryMatch(__v: _root_.com.google.protobuf.ByteString): GenericTextMatch = copy(rule = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule.BinaryMatch(__v))
def clearRule: GenericTextMatch = copy(rule = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule.Empty)
def withRule(__v: io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule): GenericTextMatch = copy(rule = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => rule.stringMatch.orNull
case 2 => rule.binaryMatch.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => rule.stringMatch.map(_root_.scalapb.descriptors.PString(_)).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 2 => rule.binaryMatch.map(_root_.scalapb.descriptors.PByteString(_)).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.type = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch
// @@protoc_insertion_point(GeneratedMessage[envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch])
}
object GenericTextMatch extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch = {
var __rule: io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule.Empty
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__rule = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule.StringMatch(_input__.readStringRequireUtf8())
case 18 =>
__rule = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule.BinaryMatch(_input__.readBytes())
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch(
rule = __rule,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch(
rule = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).flatMap(_.as[_root_.scala.Option[_root_.scala.Predef.String]]).map(io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule.StringMatch(_))
.orElse[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule](__fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[_root_.com.google.protobuf.ByteString]]).map(io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule.BinaryMatch(_)))
.getOrElse(io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule.Empty)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch(
rule = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule.Empty
)
sealed trait Rule extends _root_.scalapb.GeneratedOneof {
def isEmpty: _root_.scala.Boolean = false
def isDefined: _root_.scala.Boolean = true
def isStringMatch: _root_.scala.Boolean = false
def isBinaryMatch: _root_.scala.Boolean = false
def stringMatch: _root_.scala.Option[_root_.scala.Predef.String] = _root_.scala.None
def binaryMatch: _root_.scala.Option[_root_.com.google.protobuf.ByteString] = _root_.scala.None
}
object Rule {
@SerialVersionUID(0L)
case object Empty extends io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule {
type ValueType = _root_.scala.Nothing
override def isEmpty: _root_.scala.Boolean = true
override def isDefined: _root_.scala.Boolean = false
override def number: _root_.scala.Int = 0
override def value: _root_.scala.Nothing = throw new java.util.NoSuchElementException("Empty.value")
}
@SerialVersionUID(0L)
final case class StringMatch(value: _root_.scala.Predef.String) extends io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule {
type ValueType = _root_.scala.Predef.String
override def isStringMatch: _root_.scala.Boolean = true
override def stringMatch: _root_.scala.Option[_root_.scala.Predef.String] = Some(value)
override def number: _root_.scala.Int = 1
}
@SerialVersionUID(0L)
final case class BinaryMatch(value: _root_.com.google.protobuf.ByteString) extends io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule {
type ValueType = _root_.com.google.protobuf.ByteString
override def isBinaryMatch: _root_.scala.Boolean = true
override def binaryMatch: _root_.scala.Option[_root_.com.google.protobuf.ByteString] = Some(value)
override def number: _root_.scala.Int = 2
}
}
implicit class GenericTextMatchLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch](_l) {
def stringMatch: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.getStringMatch)((c_, f_) => c_.copy(rule = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule.StringMatch(f_)))
def binaryMatch: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.getBinaryMatch)((c_, f_) => c_.copy(rule = io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule.BinaryMatch(f_)))
def rule: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule] = field(_.rule)((c_, f_) => c_.copy(rule = f_))
}
final val STRING_MATCH_FIELD_NUMBER = 1
final val BINARY_MATCH_FIELD_NUMBER = 2
def of(
rule: io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch.Rule
): _root_.io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch = _root_.io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch(
rule
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch])
}
implicit class HttpGenericBodyMatchLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch](_l) {
def bytesLimit: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.bytesLimit)((c_, f_) => c_.copy(bytesLimit = f_))
def patterns: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch]] = field(_.patterns)((c_, f_) => c_.copy(patterns = f_))
}
final val BYTES_LIMIT_FIELD_NUMBER = 1
final val PATTERNS_FIELD_NUMBER = 2
def of(
bytesLimit: _root_.scala.Int,
patterns: _root_.scala.Seq[io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch.GenericTextMatch]
): _root_.io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch = _root_.io.envoyproxy.envoy.config.tap.v3.HttpGenericBodyMatch(
bytesLimit,
patterns
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.config.tap.v3.HttpGenericBodyMatch])
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy