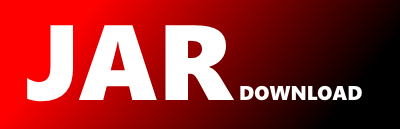
io.envoyproxy.envoy.service.auth.v2alpha.AuthorizationGrpc.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envoy-scala-control-plane_2.13 Show documentation
Show all versions of envoy-scala-control-plane_2.13 Show documentation
ScalaPB generated bindings for Envoy
package io.envoyproxy.envoy.service.auth.v2alpha
object AuthorizationGrpc {
val METHOD_CHECK: _root_.io.grpc.MethodDescriptor[io.envoyproxy.envoy.service.auth.v2.CheckRequest, io.envoyproxy.envoy.service.auth.v2.CheckResponse] =
_root_.io.grpc.MethodDescriptor.newBuilder()
.setType(_root_.io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(_root_.io.grpc.MethodDescriptor.generateFullMethodName("envoy.service.auth.v2alpha.Authorization", "Check"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.service.auth.v2.CheckRequest])
.setResponseMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.service.auth.v2.CheckResponse])
.setSchemaDescriptor(_root_.scalapb.grpc.ConcreteProtoMethodDescriptorSupplier.fromMethodDescriptor(io.envoyproxy.envoy.service.auth.v2alpha.ExternalAuthProto.javaDescriptor.getServices().get(0).getMethods().get(0)))
.build()
val SERVICE: _root_.io.grpc.ServiceDescriptor =
_root_.io.grpc.ServiceDescriptor.newBuilder("envoy.service.auth.v2alpha.Authorization")
.setSchemaDescriptor(new _root_.scalapb.grpc.ConcreteProtoFileDescriptorSupplier(io.envoyproxy.envoy.service.auth.v2alpha.ExternalAuthProto.javaDescriptor))
.addMethod(METHOD_CHECK)
.build()
/** A generic interface for performing authorization check on incoming
* requests to a networked service.
*/
trait Authorization extends _root_.scalapb.grpc.AbstractService {
override def serviceCompanion = Authorization
/** Performs authorization check based on the attributes associated with the
* incoming request, and returns status `OK` or not `OK`.
*/
def check(request: io.envoyproxy.envoy.service.auth.v2.CheckRequest): scala.concurrent.Future[io.envoyproxy.envoy.service.auth.v2.CheckResponse]
}
object Authorization extends _root_.scalapb.grpc.ServiceCompanion[Authorization] {
implicit def serviceCompanion: _root_.scalapb.grpc.ServiceCompanion[Authorization] = this
def javaDescriptor: _root_.com.google.protobuf.Descriptors.ServiceDescriptor = io.envoyproxy.envoy.service.auth.v2alpha.ExternalAuthProto.javaDescriptor.getServices().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.ServiceDescriptor = io.envoyproxy.envoy.service.auth.v2alpha.ExternalAuthProto.scalaDescriptor.services(0)
def bindService(serviceImpl: Authorization, executionContext: scala.concurrent.ExecutionContext): _root_.io.grpc.ServerServiceDefinition =
_root_.io.grpc.ServerServiceDefinition.builder(SERVICE)
.addMethod(
METHOD_CHECK,
_root_.io.grpc.stub.ServerCalls.asyncUnaryCall(new _root_.io.grpc.stub.ServerCalls.UnaryMethod[io.envoyproxy.envoy.service.auth.v2.CheckRequest, io.envoyproxy.envoy.service.auth.v2.CheckResponse] {
override def invoke(request: io.envoyproxy.envoy.service.auth.v2.CheckRequest, observer: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.service.auth.v2.CheckResponse]): _root_.scala.Unit =
serviceImpl.check(request).onComplete(scalapb.grpc.Grpc.completeObserver(observer))(
executionContext)
}))
.build()
}
/** A generic interface for performing authorization check on incoming
* requests to a networked service.
*/
trait AuthorizationBlockingClient {
def serviceCompanion = Authorization
/** Performs authorization check based on the attributes associated with the
* incoming request, and returns status `OK` or not `OK`.
*/
def check(request: io.envoyproxy.envoy.service.auth.v2.CheckRequest): io.envoyproxy.envoy.service.auth.v2.CheckResponse
}
class AuthorizationBlockingStub(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions = _root_.io.grpc.CallOptions.DEFAULT) extends _root_.io.grpc.stub.AbstractStub[AuthorizationBlockingStub](channel, options) with AuthorizationBlockingClient {
/** Performs authorization check based on the attributes associated with the
* incoming request, and returns status `OK` or not `OK`.
*/
override def check(request: io.envoyproxy.envoy.service.auth.v2.CheckRequest): io.envoyproxy.envoy.service.auth.v2.CheckResponse = {
_root_.scalapb.grpc.ClientCalls.blockingUnaryCall(channel, METHOD_CHECK, options, request)
}
override def build(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions): AuthorizationBlockingStub = new AuthorizationBlockingStub(channel, options)
}
class AuthorizationStub(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions = _root_.io.grpc.CallOptions.DEFAULT) extends _root_.io.grpc.stub.AbstractStub[AuthorizationStub](channel, options) with Authorization {
/** Performs authorization check based on the attributes associated with the
* incoming request, and returns status `OK` or not `OK`.
*/
override def check(request: io.envoyproxy.envoy.service.auth.v2.CheckRequest): scala.concurrent.Future[io.envoyproxy.envoy.service.auth.v2.CheckResponse] = {
_root_.scalapb.grpc.ClientCalls.asyncUnaryCall(channel, METHOD_CHECK, options, request)
}
override def build(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions): AuthorizationStub = new AuthorizationStub(channel, options)
}
def bindService(serviceImpl: Authorization, executionContext: scala.concurrent.ExecutionContext): _root_.io.grpc.ServerServiceDefinition = Authorization.bindService(serviceImpl, executionContext)
def blockingStub(channel: _root_.io.grpc.Channel): AuthorizationBlockingStub = new AuthorizationBlockingStub(channel)
def stub(channel: _root_.io.grpc.Channel): AuthorizationStub = new AuthorizationStub(channel)
def javaDescriptor: _root_.com.google.protobuf.Descriptors.ServiceDescriptor = io.envoyproxy.envoy.service.auth.v2alpha.ExternalAuthProto.javaDescriptor.getServices().get(0)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy