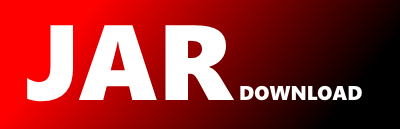
io.envoyproxy.envoy.service.discovery.v2.HealthDiscoveryServiceGrpc.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envoy-scala-control-plane_2.13 Show documentation
Show all versions of envoy-scala-control-plane_2.13 Show documentation
ScalaPB generated bindings for Envoy
package io.envoyproxy.envoy.service.discovery.v2
object HealthDiscoveryServiceGrpc {
val METHOD_STREAM_HEALTH_CHECK: _root_.io.grpc.MethodDescriptor[io.envoyproxy.envoy.service.discovery.v2.HealthCheckRequestOrEndpointHealthResponse, io.envoyproxy.envoy.service.discovery.v2.HealthCheckSpecifier] =
_root_.io.grpc.MethodDescriptor.newBuilder()
.setType(_root_.io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(_root_.io.grpc.MethodDescriptor.generateFullMethodName("envoy.service.discovery.v2.HealthDiscoveryService", "StreamHealthCheck"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.service.discovery.v2.HealthCheckRequestOrEndpointHealthResponse])
.setResponseMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.service.discovery.v2.HealthCheckSpecifier])
.setSchemaDescriptor(_root_.scalapb.grpc.ConcreteProtoMethodDescriptorSupplier.fromMethodDescriptor(io.envoyproxy.envoy.service.discovery.v2.HdsProto.javaDescriptor.getServices().get(0).getMethods().get(0)))
.build()
val METHOD_FETCH_HEALTH_CHECK: _root_.io.grpc.MethodDescriptor[io.envoyproxy.envoy.service.discovery.v2.HealthCheckRequestOrEndpointHealthResponse, io.envoyproxy.envoy.service.discovery.v2.HealthCheckSpecifier] =
_root_.io.grpc.MethodDescriptor.newBuilder()
.setType(_root_.io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(_root_.io.grpc.MethodDescriptor.generateFullMethodName("envoy.service.discovery.v2.HealthDiscoveryService", "FetchHealthCheck"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.service.discovery.v2.HealthCheckRequestOrEndpointHealthResponse])
.setResponseMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.service.discovery.v2.HealthCheckSpecifier])
.setSchemaDescriptor(_root_.scalapb.grpc.ConcreteProtoMethodDescriptorSupplier.fromMethodDescriptor(io.envoyproxy.envoy.service.discovery.v2.HdsProto.javaDescriptor.getServices().get(0).getMethods().get(1)))
.build()
val SERVICE: _root_.io.grpc.ServiceDescriptor =
_root_.io.grpc.ServiceDescriptor.newBuilder("envoy.service.discovery.v2.HealthDiscoveryService")
.setSchemaDescriptor(new _root_.scalapb.grpc.ConcreteProtoFileDescriptorSupplier(io.envoyproxy.envoy.service.discovery.v2.HdsProto.javaDescriptor))
.addMethod(METHOD_STREAM_HEALTH_CHECK)
.addMethod(METHOD_FETCH_HEALTH_CHECK)
.build()
/** HDS is Health Discovery Service. It compliments Envoy’s health checking
* service by designating this Envoy to be a healthchecker for a subset of hosts
* in the cluster. The status of these health checks will be reported to the
* management server, where it can be aggregated etc and redistributed back to
* Envoy through EDS.
*/
trait HealthDiscoveryService extends _root_.scalapb.grpc.AbstractService {
override def serviceCompanion = HealthDiscoveryService
/** 1. Envoy starts up and if its can_healthcheck option in the static
* bootstrap config is enabled, sends HealthCheckRequest to the management
* server. It supplies its capabilities (which protocol it can health check
* with, what zone it resides in, etc.).
* 2. In response to (1), the management server designates this Envoy as a
* healthchecker to health check a subset of all upstream hosts for a given
* cluster (for example upstream Host 1 and Host 2). It streams
* HealthCheckSpecifier messages with cluster related configuration for all
* clusters this Envoy is designated to health check. Subsequent
* HealthCheckSpecifier message will be sent on changes to:
* a. Endpoints to health checks
* b. Per cluster configuration change
* 3. Envoy creates a health probe based on the HealthCheck config and sends
* it to endpoint(ip:port) of Host 1 and 2. Based on the HealthCheck
* configuration Envoy waits upon the arrival of the probe response and
* looks at the content of the response to decide whether the endpoint is
* healthy or not. If a response hasn't been received within the timeout
* interval, the endpoint health status is considered TIMEOUT.
* 4. Envoy reports results back in an EndpointHealthResponse message.
* Envoy streams responses as often as the interval configured by the
* management server in HealthCheckSpecifier.
* 5. The management Server collects health statuses for all endpoints in the
* cluster (for all clusters) and uses this information to construct
* EndpointDiscoveryResponse messages.
* 6. Once Envoy has a list of upstream endpoints to send traffic to, it load
* balances traffic to them without additional health checking. It may
* use inline healthcheck (i.e. consider endpoint UNHEALTHY if connection
* failed to a particular endpoint to account for health status propagation
* delay between HDS and EDS).
* By default, can_healthcheck is true. If can_healthcheck is false, Cluster
* configuration may not contain HealthCheck message.
* TODO(htuch): How is can_healthcheck communicated to CDS to ensure the above
* invariant?
* TODO(htuch): Add @amb67's diagram.
*/
def streamHealthCheck(responseObserver: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.service.discovery.v2.HealthCheckSpecifier]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.service.discovery.v2.HealthCheckRequestOrEndpointHealthResponse]
/** TODO(htuch): Unlike the gRPC version, there is no stream-based binding of
* request/response. Should we add an identifier to the HealthCheckSpecifier
* to bind with the response?
*/
def fetchHealthCheck(request: io.envoyproxy.envoy.service.discovery.v2.HealthCheckRequestOrEndpointHealthResponse): scala.concurrent.Future[io.envoyproxy.envoy.service.discovery.v2.HealthCheckSpecifier]
}
object HealthDiscoveryService extends _root_.scalapb.grpc.ServiceCompanion[HealthDiscoveryService] {
implicit def serviceCompanion: _root_.scalapb.grpc.ServiceCompanion[HealthDiscoveryService] = this
def javaDescriptor: _root_.com.google.protobuf.Descriptors.ServiceDescriptor = io.envoyproxy.envoy.service.discovery.v2.HdsProto.javaDescriptor.getServices().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.ServiceDescriptor = io.envoyproxy.envoy.service.discovery.v2.HdsProto.scalaDescriptor.services(0)
def bindService(serviceImpl: HealthDiscoveryService, executionContext: scala.concurrent.ExecutionContext): _root_.io.grpc.ServerServiceDefinition =
_root_.io.grpc.ServerServiceDefinition.builder(SERVICE)
.addMethod(
METHOD_STREAM_HEALTH_CHECK,
_root_.io.grpc.stub.ServerCalls.asyncBidiStreamingCall(new _root_.io.grpc.stub.ServerCalls.BidiStreamingMethod[io.envoyproxy.envoy.service.discovery.v2.HealthCheckRequestOrEndpointHealthResponse, io.envoyproxy.envoy.service.discovery.v2.HealthCheckSpecifier] {
override def invoke(observer: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.service.discovery.v2.HealthCheckSpecifier]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.service.discovery.v2.HealthCheckRequestOrEndpointHealthResponse] =
serviceImpl.streamHealthCheck(observer)
}))
.addMethod(
METHOD_FETCH_HEALTH_CHECK,
_root_.io.grpc.stub.ServerCalls.asyncUnaryCall(new _root_.io.grpc.stub.ServerCalls.UnaryMethod[io.envoyproxy.envoy.service.discovery.v2.HealthCheckRequestOrEndpointHealthResponse, io.envoyproxy.envoy.service.discovery.v2.HealthCheckSpecifier] {
override def invoke(request: io.envoyproxy.envoy.service.discovery.v2.HealthCheckRequestOrEndpointHealthResponse, observer: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.service.discovery.v2.HealthCheckSpecifier]): _root_.scala.Unit =
serviceImpl.fetchHealthCheck(request).onComplete(scalapb.grpc.Grpc.completeObserver(observer))(
executionContext)
}))
.build()
}
/** HDS is Health Discovery Service. It compliments Envoy’s health checking
* service by designating this Envoy to be a healthchecker for a subset of hosts
* in the cluster. The status of these health checks will be reported to the
* management server, where it can be aggregated etc and redistributed back to
* Envoy through EDS.
*/
trait HealthDiscoveryServiceBlockingClient {
def serviceCompanion = HealthDiscoveryService
/** TODO(htuch): Unlike the gRPC version, there is no stream-based binding of
* request/response. Should we add an identifier to the HealthCheckSpecifier
* to bind with the response?
*/
def fetchHealthCheck(request: io.envoyproxy.envoy.service.discovery.v2.HealthCheckRequestOrEndpointHealthResponse): io.envoyproxy.envoy.service.discovery.v2.HealthCheckSpecifier
}
class HealthDiscoveryServiceBlockingStub(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions = _root_.io.grpc.CallOptions.DEFAULT) extends _root_.io.grpc.stub.AbstractStub[HealthDiscoveryServiceBlockingStub](channel, options) with HealthDiscoveryServiceBlockingClient {
/** TODO(htuch): Unlike the gRPC version, there is no stream-based binding of
* request/response. Should we add an identifier to the HealthCheckSpecifier
* to bind with the response?
*/
override def fetchHealthCheck(request: io.envoyproxy.envoy.service.discovery.v2.HealthCheckRequestOrEndpointHealthResponse): io.envoyproxy.envoy.service.discovery.v2.HealthCheckSpecifier = {
_root_.scalapb.grpc.ClientCalls.blockingUnaryCall(channel, METHOD_FETCH_HEALTH_CHECK, options, request)
}
override def build(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions): HealthDiscoveryServiceBlockingStub = new HealthDiscoveryServiceBlockingStub(channel, options)
}
class HealthDiscoveryServiceStub(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions = _root_.io.grpc.CallOptions.DEFAULT) extends _root_.io.grpc.stub.AbstractStub[HealthDiscoveryServiceStub](channel, options) with HealthDiscoveryService {
/** 1. Envoy starts up and if its can_healthcheck option in the static
* bootstrap config is enabled, sends HealthCheckRequest to the management
* server. It supplies its capabilities (which protocol it can health check
* with, what zone it resides in, etc.).
* 2. In response to (1), the management server designates this Envoy as a
* healthchecker to health check a subset of all upstream hosts for a given
* cluster (for example upstream Host 1 and Host 2). It streams
* HealthCheckSpecifier messages with cluster related configuration for all
* clusters this Envoy is designated to health check. Subsequent
* HealthCheckSpecifier message will be sent on changes to:
* a. Endpoints to health checks
* b. Per cluster configuration change
* 3. Envoy creates a health probe based on the HealthCheck config and sends
* it to endpoint(ip:port) of Host 1 and 2. Based on the HealthCheck
* configuration Envoy waits upon the arrival of the probe response and
* looks at the content of the response to decide whether the endpoint is
* healthy or not. If a response hasn't been received within the timeout
* interval, the endpoint health status is considered TIMEOUT.
* 4. Envoy reports results back in an EndpointHealthResponse message.
* Envoy streams responses as often as the interval configured by the
* management server in HealthCheckSpecifier.
* 5. The management Server collects health statuses for all endpoints in the
* cluster (for all clusters) and uses this information to construct
* EndpointDiscoveryResponse messages.
* 6. Once Envoy has a list of upstream endpoints to send traffic to, it load
* balances traffic to them without additional health checking. It may
* use inline healthcheck (i.e. consider endpoint UNHEALTHY if connection
* failed to a particular endpoint to account for health status propagation
* delay between HDS and EDS).
* By default, can_healthcheck is true. If can_healthcheck is false, Cluster
* configuration may not contain HealthCheck message.
* TODO(htuch): How is can_healthcheck communicated to CDS to ensure the above
* invariant?
* TODO(htuch): Add @amb67's diagram.
*/
override def streamHealthCheck(responseObserver: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.service.discovery.v2.HealthCheckSpecifier]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.service.discovery.v2.HealthCheckRequestOrEndpointHealthResponse] = {
_root_.scalapb.grpc.ClientCalls.asyncBidiStreamingCall(channel, METHOD_STREAM_HEALTH_CHECK, options, responseObserver)
}
/** TODO(htuch): Unlike the gRPC version, there is no stream-based binding of
* request/response. Should we add an identifier to the HealthCheckSpecifier
* to bind with the response?
*/
override def fetchHealthCheck(request: io.envoyproxy.envoy.service.discovery.v2.HealthCheckRequestOrEndpointHealthResponse): scala.concurrent.Future[io.envoyproxy.envoy.service.discovery.v2.HealthCheckSpecifier] = {
_root_.scalapb.grpc.ClientCalls.asyncUnaryCall(channel, METHOD_FETCH_HEALTH_CHECK, options, request)
}
override def build(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions): HealthDiscoveryServiceStub = new HealthDiscoveryServiceStub(channel, options)
}
def bindService(serviceImpl: HealthDiscoveryService, executionContext: scala.concurrent.ExecutionContext): _root_.io.grpc.ServerServiceDefinition = HealthDiscoveryService.bindService(serviceImpl, executionContext)
def blockingStub(channel: _root_.io.grpc.Channel): HealthDiscoveryServiceBlockingStub = new HealthDiscoveryServiceBlockingStub(channel)
def stub(channel: _root_.io.grpc.Channel): HealthDiscoveryServiceStub = new HealthDiscoveryServiceStub(channel)
def javaDescriptor: _root_.com.google.protobuf.Descriptors.ServiceDescriptor = io.envoyproxy.envoy.service.discovery.v2.HdsProto.javaDescriptor.getServices().get(0)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy