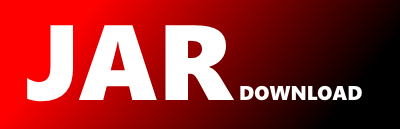
io.envoyproxy.envoy.service.discovery.v2.RuntimeDiscoveryServiceGrpc.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envoy-scala-control-plane_2.13 Show documentation
Show all versions of envoy-scala-control-plane_2.13 Show documentation
ScalaPB generated bindings for Envoy
package io.envoyproxy.envoy.service.discovery.v2
object RuntimeDiscoveryServiceGrpc {
val METHOD_STREAM_RUNTIME: _root_.io.grpc.MethodDescriptor[io.envoyproxy.envoy.api.v2.DiscoveryRequest, io.envoyproxy.envoy.api.v2.DiscoveryResponse] =
_root_.io.grpc.MethodDescriptor.newBuilder()
.setType(_root_.io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(_root_.io.grpc.MethodDescriptor.generateFullMethodName("envoy.service.discovery.v2.RuntimeDiscoveryService", "StreamRuntime"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.api.v2.DiscoveryRequest])
.setResponseMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.api.v2.DiscoveryResponse])
.setSchemaDescriptor(_root_.scalapb.grpc.ConcreteProtoMethodDescriptorSupplier.fromMethodDescriptor(io.envoyproxy.envoy.service.discovery.v2.RtdsProto.javaDescriptor.getServices().get(0).getMethods().get(0)))
.build()
val METHOD_DELTA_RUNTIME: _root_.io.grpc.MethodDescriptor[io.envoyproxy.envoy.api.v2.DeltaDiscoveryRequest, io.envoyproxy.envoy.api.v2.DeltaDiscoveryResponse] =
_root_.io.grpc.MethodDescriptor.newBuilder()
.setType(_root_.io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(_root_.io.grpc.MethodDescriptor.generateFullMethodName("envoy.service.discovery.v2.RuntimeDiscoveryService", "DeltaRuntime"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.api.v2.DeltaDiscoveryRequest])
.setResponseMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.api.v2.DeltaDiscoveryResponse])
.setSchemaDescriptor(_root_.scalapb.grpc.ConcreteProtoMethodDescriptorSupplier.fromMethodDescriptor(io.envoyproxy.envoy.service.discovery.v2.RtdsProto.javaDescriptor.getServices().get(0).getMethods().get(1)))
.build()
val METHOD_FETCH_RUNTIME: _root_.io.grpc.MethodDescriptor[io.envoyproxy.envoy.api.v2.DiscoveryRequest, io.envoyproxy.envoy.api.v2.DiscoveryResponse] =
_root_.io.grpc.MethodDescriptor.newBuilder()
.setType(_root_.io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(_root_.io.grpc.MethodDescriptor.generateFullMethodName("envoy.service.discovery.v2.RuntimeDiscoveryService", "FetchRuntime"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.api.v2.DiscoveryRequest])
.setResponseMarshaller(_root_.scalapb.grpc.Marshaller.forMessage[io.envoyproxy.envoy.api.v2.DiscoveryResponse])
.setSchemaDescriptor(_root_.scalapb.grpc.ConcreteProtoMethodDescriptorSupplier.fromMethodDescriptor(io.envoyproxy.envoy.service.discovery.v2.RtdsProto.javaDescriptor.getServices().get(0).getMethods().get(2)))
.build()
val SERVICE: _root_.io.grpc.ServiceDescriptor =
_root_.io.grpc.ServiceDescriptor.newBuilder("envoy.service.discovery.v2.RuntimeDiscoveryService")
.setSchemaDescriptor(new _root_.scalapb.grpc.ConcreteProtoFileDescriptorSupplier(io.envoyproxy.envoy.service.discovery.v2.RtdsProto.javaDescriptor))
.addMethod(METHOD_STREAM_RUNTIME)
.addMethod(METHOD_DELTA_RUNTIME)
.addMethod(METHOD_FETCH_RUNTIME)
.build()
/** Discovery service for Runtime resources.
*/
trait RuntimeDiscoveryService extends _root_.scalapb.grpc.AbstractService {
override def serviceCompanion = RuntimeDiscoveryService
def streamRuntime(responseObserver: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DiscoveryResponse]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DiscoveryRequest]
def deltaRuntime(responseObserver: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DeltaDiscoveryResponse]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DeltaDiscoveryRequest]
def fetchRuntime(request: io.envoyproxy.envoy.api.v2.DiscoveryRequest): scala.concurrent.Future[io.envoyproxy.envoy.api.v2.DiscoveryResponse]
}
object RuntimeDiscoveryService extends _root_.scalapb.grpc.ServiceCompanion[RuntimeDiscoveryService] {
implicit def serviceCompanion: _root_.scalapb.grpc.ServiceCompanion[RuntimeDiscoveryService] = this
def javaDescriptor: _root_.com.google.protobuf.Descriptors.ServiceDescriptor = io.envoyproxy.envoy.service.discovery.v2.RtdsProto.javaDescriptor.getServices().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.ServiceDescriptor = io.envoyproxy.envoy.service.discovery.v2.RtdsProto.scalaDescriptor.services(0)
def bindService(serviceImpl: RuntimeDiscoveryService, executionContext: scala.concurrent.ExecutionContext): _root_.io.grpc.ServerServiceDefinition =
_root_.io.grpc.ServerServiceDefinition.builder(SERVICE)
.addMethod(
METHOD_STREAM_RUNTIME,
_root_.io.grpc.stub.ServerCalls.asyncBidiStreamingCall(new _root_.io.grpc.stub.ServerCalls.BidiStreamingMethod[io.envoyproxy.envoy.api.v2.DiscoveryRequest, io.envoyproxy.envoy.api.v2.DiscoveryResponse] {
override def invoke(observer: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DiscoveryResponse]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DiscoveryRequest] =
serviceImpl.streamRuntime(observer)
}))
.addMethod(
METHOD_DELTA_RUNTIME,
_root_.io.grpc.stub.ServerCalls.asyncBidiStreamingCall(new _root_.io.grpc.stub.ServerCalls.BidiStreamingMethod[io.envoyproxy.envoy.api.v2.DeltaDiscoveryRequest, io.envoyproxy.envoy.api.v2.DeltaDiscoveryResponse] {
override def invoke(observer: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DeltaDiscoveryResponse]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DeltaDiscoveryRequest] =
serviceImpl.deltaRuntime(observer)
}))
.addMethod(
METHOD_FETCH_RUNTIME,
_root_.io.grpc.stub.ServerCalls.asyncUnaryCall(new _root_.io.grpc.stub.ServerCalls.UnaryMethod[io.envoyproxy.envoy.api.v2.DiscoveryRequest, io.envoyproxy.envoy.api.v2.DiscoveryResponse] {
override def invoke(request: io.envoyproxy.envoy.api.v2.DiscoveryRequest, observer: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DiscoveryResponse]): _root_.scala.Unit =
serviceImpl.fetchRuntime(request).onComplete(scalapb.grpc.Grpc.completeObserver(observer))(
executionContext)
}))
.build()
}
/** Discovery service for Runtime resources.
*/
trait RuntimeDiscoveryServiceBlockingClient {
def serviceCompanion = RuntimeDiscoveryService
def fetchRuntime(request: io.envoyproxy.envoy.api.v2.DiscoveryRequest): io.envoyproxy.envoy.api.v2.DiscoveryResponse
}
class RuntimeDiscoveryServiceBlockingStub(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions = _root_.io.grpc.CallOptions.DEFAULT) extends _root_.io.grpc.stub.AbstractStub[RuntimeDiscoveryServiceBlockingStub](channel, options) with RuntimeDiscoveryServiceBlockingClient {
override def fetchRuntime(request: io.envoyproxy.envoy.api.v2.DiscoveryRequest): io.envoyproxy.envoy.api.v2.DiscoveryResponse = {
_root_.scalapb.grpc.ClientCalls.blockingUnaryCall(channel, METHOD_FETCH_RUNTIME, options, request)
}
override def build(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions): RuntimeDiscoveryServiceBlockingStub = new RuntimeDiscoveryServiceBlockingStub(channel, options)
}
class RuntimeDiscoveryServiceStub(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions = _root_.io.grpc.CallOptions.DEFAULT) extends _root_.io.grpc.stub.AbstractStub[RuntimeDiscoveryServiceStub](channel, options) with RuntimeDiscoveryService {
override def streamRuntime(responseObserver: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DiscoveryResponse]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DiscoveryRequest] = {
_root_.scalapb.grpc.ClientCalls.asyncBidiStreamingCall(channel, METHOD_STREAM_RUNTIME, options, responseObserver)
}
override def deltaRuntime(responseObserver: _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DeltaDiscoveryResponse]): _root_.io.grpc.stub.StreamObserver[io.envoyproxy.envoy.api.v2.DeltaDiscoveryRequest] = {
_root_.scalapb.grpc.ClientCalls.asyncBidiStreamingCall(channel, METHOD_DELTA_RUNTIME, options, responseObserver)
}
override def fetchRuntime(request: io.envoyproxy.envoy.api.v2.DiscoveryRequest): scala.concurrent.Future[io.envoyproxy.envoy.api.v2.DiscoveryResponse] = {
_root_.scalapb.grpc.ClientCalls.asyncUnaryCall(channel, METHOD_FETCH_RUNTIME, options, request)
}
override def build(channel: _root_.io.grpc.Channel, options: _root_.io.grpc.CallOptions): RuntimeDiscoveryServiceStub = new RuntimeDiscoveryServiceStub(channel, options)
}
def bindService(serviceImpl: RuntimeDiscoveryService, executionContext: scala.concurrent.ExecutionContext): _root_.io.grpc.ServerServiceDefinition = RuntimeDiscoveryService.bindService(serviceImpl, executionContext)
def blockingStub(channel: _root_.io.grpc.Channel): RuntimeDiscoveryServiceBlockingStub = new RuntimeDiscoveryServiceBlockingStub(channel)
def stub(channel: _root_.io.grpc.Channel): RuntimeDiscoveryServiceStub = new RuntimeDiscoveryServiceStub(channel)
def javaDescriptor: _root_.com.google.protobuf.Descriptors.ServiceDescriptor = io.envoyproxy.envoy.service.discovery.v2.RtdsProto.javaDescriptor.getServices().get(0)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy