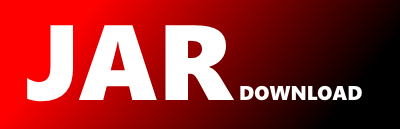
io.envoyproxy.envoy.service.status.v3.ClientConfig.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envoy-scala-control-plane_2.13 Show documentation
Show all versions of envoy-scala-control-plane_2.13 Show documentation
ScalaPB generated bindings for Envoy
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package io.envoyproxy.envoy.service.status.v3
/** All xds configs for a particular client.
*
* @param node
* Node for a particular client.
* @param xdsConfig
* This field is deprecated in favor of generic_xds_configs which is
* much simpler and uniform in structure.
* @param genericXdsConfigs
* Represents generic xDS config and the exact config structure depends on
* the type URL (like Cluster if it is CDS)
*/
@SerialVersionUID(0L)
final case class ClientConfig(
node: _root_.scala.Option[io.envoyproxy.envoy.config.core.v3.Node] = _root_.scala.None,
@scala.deprecated(message="Marked as deprecated in proto file", "") xdsConfig: _root_.scala.Seq[io.envoyproxy.envoy.service.status.v3.PerXdsConfig] = _root_.scala.Seq.empty,
genericXdsConfigs: _root_.scala.Seq[io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig] = _root_.scala.Seq.empty,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[ClientConfig] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
if (node.isDefined) {
val __value = node.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
xdsConfig.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
genericXdsConfigs.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
node.foreach { __v =>
val __m = __v
_output__.writeTag(1, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
xdsConfig.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
genericXdsConfigs.foreach { __v =>
val __m = __v
_output__.writeTag(3, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def getNode: io.envoyproxy.envoy.config.core.v3.Node = node.getOrElse(io.envoyproxy.envoy.config.core.v3.Node.defaultInstance)
def clearNode: ClientConfig = copy(node = _root_.scala.None)
def withNode(__v: io.envoyproxy.envoy.config.core.v3.Node): ClientConfig = copy(node = Option(__v))
def clearXdsConfig = copy(xdsConfig = _root_.scala.Seq.empty)
def addXdsConfig(__vs: io.envoyproxy.envoy.service.status.v3.PerXdsConfig *): ClientConfig = addAllXdsConfig(__vs)
def addAllXdsConfig(__vs: Iterable[io.envoyproxy.envoy.service.status.v3.PerXdsConfig]): ClientConfig = copy(xdsConfig = xdsConfig ++ __vs)
def withXdsConfig(__v: _root_.scala.Seq[io.envoyproxy.envoy.service.status.v3.PerXdsConfig]): ClientConfig = copy(xdsConfig = __v)
def clearGenericXdsConfigs = copy(genericXdsConfigs = _root_.scala.Seq.empty)
def addGenericXdsConfigs(__vs: io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig *): ClientConfig = addAllGenericXdsConfigs(__vs)
def addAllGenericXdsConfigs(__vs: Iterable[io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig]): ClientConfig = copy(genericXdsConfigs = genericXdsConfigs ++ __vs)
def withGenericXdsConfigs(__v: _root_.scala.Seq[io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig]): ClientConfig = copy(genericXdsConfigs = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => node.orNull
case 2 => xdsConfig
case 3 => genericXdsConfigs
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => node.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 2 => _root_.scalapb.descriptors.PRepeated(xdsConfig.iterator.map(_.toPMessage).toVector)
case 3 => _root_.scalapb.descriptors.PRepeated(genericXdsConfigs.iterator.map(_.toPMessage).toVector)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.service.status.v3.ClientConfig.type = io.envoyproxy.envoy.service.status.v3.ClientConfig
// @@protoc_insertion_point(GeneratedMessage[envoy.service.status.v3.ClientConfig])
}
object ClientConfig extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.service.status.v3.ClientConfig] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.service.status.v3.ClientConfig] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.service.status.v3.ClientConfig = {
var __node: _root_.scala.Option[io.envoyproxy.envoy.config.core.v3.Node] = _root_.scala.None
val __xdsConfig: _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.service.status.v3.PerXdsConfig] = new _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.service.status.v3.PerXdsConfig]
val __genericXdsConfigs: _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig] = new _root_.scala.collection.immutable.VectorBuilder[io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig]
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__node = Option(__node.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.config.core.v3.Node](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 18 =>
__xdsConfig += _root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.service.status.v3.PerXdsConfig](_input__)
case 26 =>
__genericXdsConfigs += _root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig](_input__)
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.service.status.v3.ClientConfig(
node = __node,
xdsConfig = __xdsConfig.result(),
genericXdsConfigs = __genericXdsConfigs.result(),
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.service.status.v3.ClientConfig] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.service.status.v3.ClientConfig(
node = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.config.core.v3.Node]]),
xdsConfig = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.service.status.v3.PerXdsConfig]]).getOrElse(_root_.scala.Seq.empty),
genericXdsConfigs = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Seq[io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig]]).getOrElse(_root_.scala.Seq.empty)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = CsdsProto.javaDescriptor.getMessageTypes().get(2)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = CsdsProto.scalaDescriptor.messages(2)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 1 => __out = io.envoyproxy.envoy.config.core.v3.Node
case 2 => __out = io.envoyproxy.envoy.service.status.v3.PerXdsConfig
case 3 => __out = io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.envoyproxy.envoy.service.status.v3.ClientConfig(
node = _root_.scala.None,
xdsConfig = _root_.scala.Seq.empty,
genericXdsConfigs = _root_.scala.Seq.empty
)
/** GenericXdsConfig is used to specify the config status and the dump
* of any xDS resource identified by their type URL. It is the generalized
* version of the now deprecated ListenersConfigDump, ClustersConfigDump etc
* [#next-free-field: 10]
*
* @param typeUrl
* Type_url represents the fully qualified name of xDS resource type
* like envoy.v3.Cluster, envoy.v3.ClusterLoadAssignment etc.
* @param name
* Name of the xDS resource
* @param versionInfo
* This is the :ref:`version_info <envoy_v3_api_field_service.discovery.v3.DiscoveryResponse.version_info>`
* in the last processed xDS discovery response. If there are only
* static bootstrap listeners, this field will be ""
* @param xdsConfig
* The xDS resource config. Actual content depends on the type
* @param lastUpdated
* Timestamp when the xDS resource was last updated
* @param configStatus
* Per xDS resource config status. It is generated by management servers.
* It will not be present if the CSDS server is an xDS client.
* @param clientStatus
* Per xDS resource status from the view of a xDS client
* @param errorState
* Set if the last update failed, cleared after the next successful
* update. The *error_state* field contains the rejected version of
* this particular resource along with the reason and timestamp. For
* successfully updated or acknowledged resource, this field should
* be empty.
* [#not-implemented-hide:]
* @param isStaticResource
* Is static resource is true if it is specified in the config supplied
* through the file at the startup.
*/
@SerialVersionUID(0L)
final case class GenericXdsConfig(
typeUrl: _root_.scala.Predef.String = "",
name: _root_.scala.Predef.String = "",
versionInfo: _root_.scala.Predef.String = "",
xdsConfig: _root_.scala.Option[com.google.protobuf.any.Any] = _root_.scala.None,
lastUpdated: _root_.scala.Option[com.google.protobuf.timestamp.Timestamp] = _root_.scala.None,
configStatus: io.envoyproxy.envoy.service.status.v3.ConfigStatus = io.envoyproxy.envoy.service.status.v3.ConfigStatus.UNKNOWN,
clientStatus: io.envoyproxy.envoy.admin.v3.ClientResourceStatus = io.envoyproxy.envoy.admin.v3.ClientResourceStatus.UNKNOWN,
errorState: _root_.scala.Option[io.envoyproxy.envoy.admin.v3.UpdateFailureState] = _root_.scala.None,
isStaticResource: _root_.scala.Boolean = false,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[GenericXdsConfig] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = typeUrl
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
}
};
{
val __value = name
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(2, __value)
}
};
{
val __value = versionInfo
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(3, __value)
}
};
if (xdsConfig.isDefined) {
val __value = xdsConfig.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
if (lastUpdated.isDefined) {
val __value = lastUpdated.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
{
val __value = configStatus.value
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeEnumSize(6, __value)
}
};
{
val __value = clientStatus.value
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeEnumSize(7, __value)
}
};
if (errorState.isDefined) {
val __value = errorState.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
{
val __value = isStaticResource
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(9, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = typeUrl
if (!__v.isEmpty) {
_output__.writeString(1, __v)
}
};
{
val __v = name
if (!__v.isEmpty) {
_output__.writeString(2, __v)
}
};
{
val __v = versionInfo
if (!__v.isEmpty) {
_output__.writeString(3, __v)
}
};
xdsConfig.foreach { __v =>
val __m = __v
_output__.writeTag(4, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
lastUpdated.foreach { __v =>
val __m = __v
_output__.writeTag(5, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = configStatus.value
if (__v != 0) {
_output__.writeEnum(6, __v)
}
};
{
val __v = clientStatus.value
if (__v != 0) {
_output__.writeEnum(7, __v)
}
};
errorState.foreach { __v =>
val __m = __v
_output__.writeTag(8, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = isStaticResource
if (__v != false) {
_output__.writeBool(9, __v)
}
};
unknownFields.writeTo(_output__)
}
def withTypeUrl(__v: _root_.scala.Predef.String): GenericXdsConfig = copy(typeUrl = __v)
def withName(__v: _root_.scala.Predef.String): GenericXdsConfig = copy(name = __v)
def withVersionInfo(__v: _root_.scala.Predef.String): GenericXdsConfig = copy(versionInfo = __v)
def getXdsConfig: com.google.protobuf.any.Any = xdsConfig.getOrElse(com.google.protobuf.any.Any.defaultInstance)
def clearXdsConfig: GenericXdsConfig = copy(xdsConfig = _root_.scala.None)
def withXdsConfig(__v: com.google.protobuf.any.Any): GenericXdsConfig = copy(xdsConfig = Option(__v))
def getLastUpdated: com.google.protobuf.timestamp.Timestamp = lastUpdated.getOrElse(com.google.protobuf.timestamp.Timestamp.defaultInstance)
def clearLastUpdated: GenericXdsConfig = copy(lastUpdated = _root_.scala.None)
def withLastUpdated(__v: com.google.protobuf.timestamp.Timestamp): GenericXdsConfig = copy(lastUpdated = Option(__v))
def withConfigStatus(__v: io.envoyproxy.envoy.service.status.v3.ConfigStatus): GenericXdsConfig = copy(configStatus = __v)
def withClientStatus(__v: io.envoyproxy.envoy.admin.v3.ClientResourceStatus): GenericXdsConfig = copy(clientStatus = __v)
def getErrorState: io.envoyproxy.envoy.admin.v3.UpdateFailureState = errorState.getOrElse(io.envoyproxy.envoy.admin.v3.UpdateFailureState.defaultInstance)
def clearErrorState: GenericXdsConfig = copy(errorState = _root_.scala.None)
def withErrorState(__v: io.envoyproxy.envoy.admin.v3.UpdateFailureState): GenericXdsConfig = copy(errorState = Option(__v))
def withIsStaticResource(__v: _root_.scala.Boolean): GenericXdsConfig = copy(isStaticResource = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = typeUrl
if (__t != "") __t else null
}
case 2 => {
val __t = name
if (__t != "") __t else null
}
case 3 => {
val __t = versionInfo
if (__t != "") __t else null
}
case 4 => xdsConfig.orNull
case 5 => lastUpdated.orNull
case 6 => {
val __t = configStatus.javaValueDescriptor
if (__t.getNumber() != 0) __t else null
}
case 7 => {
val __t = clientStatus.javaValueDescriptor
if (__t.getNumber() != 0) __t else null
}
case 8 => errorState.orNull
case 9 => {
val __t = isStaticResource
if (__t != false) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PString(typeUrl)
case 2 => _root_.scalapb.descriptors.PString(name)
case 3 => _root_.scalapb.descriptors.PString(versionInfo)
case 4 => xdsConfig.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 5 => lastUpdated.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 6 => _root_.scalapb.descriptors.PEnum(configStatus.scalaValueDescriptor)
case 7 => _root_.scalapb.descriptors.PEnum(clientStatus.scalaValueDescriptor)
case 8 => errorState.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 9 => _root_.scalapb.descriptors.PBoolean(isStaticResource)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig.type = io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig
// @@protoc_insertion_point(GeneratedMessage[envoy.service.status.v3.ClientConfig.GenericXdsConfig])
}
object GenericXdsConfig extends scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig = {
var __typeUrl: _root_.scala.Predef.String = ""
var __name: _root_.scala.Predef.String = ""
var __versionInfo: _root_.scala.Predef.String = ""
var __xdsConfig: _root_.scala.Option[com.google.protobuf.any.Any] = _root_.scala.None
var __lastUpdated: _root_.scala.Option[com.google.protobuf.timestamp.Timestamp] = _root_.scala.None
var __configStatus: io.envoyproxy.envoy.service.status.v3.ConfigStatus = io.envoyproxy.envoy.service.status.v3.ConfigStatus.UNKNOWN
var __clientStatus: io.envoyproxy.envoy.admin.v3.ClientResourceStatus = io.envoyproxy.envoy.admin.v3.ClientResourceStatus.UNKNOWN
var __errorState: _root_.scala.Option[io.envoyproxy.envoy.admin.v3.UpdateFailureState] = _root_.scala.None
var __isStaticResource: _root_.scala.Boolean = false
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__typeUrl = _input__.readStringRequireUtf8()
case 18 =>
__name = _input__.readStringRequireUtf8()
case 26 =>
__versionInfo = _input__.readStringRequireUtf8()
case 34 =>
__xdsConfig = Option(__xdsConfig.fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.any.Any](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 42 =>
__lastUpdated = Option(__lastUpdated.fold(_root_.scalapb.LiteParser.readMessage[com.google.protobuf.timestamp.Timestamp](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 48 =>
__configStatus = io.envoyproxy.envoy.service.status.v3.ConfigStatus.fromValue(_input__.readEnum())
case 56 =>
__clientStatus = io.envoyproxy.envoy.admin.v3.ClientResourceStatus.fromValue(_input__.readEnum())
case 66 =>
__errorState = Option(__errorState.fold(_root_.scalapb.LiteParser.readMessage[io.envoyproxy.envoy.admin.v3.UpdateFailureState](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 72 =>
__isStaticResource = _input__.readBool()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig(
typeUrl = __typeUrl,
name = __name,
versionInfo = __versionInfo,
xdsConfig = __xdsConfig,
lastUpdated = __lastUpdated,
configStatus = __configStatus,
clientStatus = __clientStatus,
errorState = __errorState,
isStaticResource = __isStaticResource,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig(
typeUrl = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
name = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
versionInfo = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
xdsConfig = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.any.Any]]),
lastUpdated = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).flatMap(_.as[_root_.scala.Option[com.google.protobuf.timestamp.Timestamp]]),
configStatus = io.envoyproxy.envoy.service.status.v3.ConfigStatus.fromValue(__fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scalapb.descriptors.EnumValueDescriptor]).getOrElse(io.envoyproxy.envoy.service.status.v3.ConfigStatus.UNKNOWN.scalaValueDescriptor).number),
clientStatus = io.envoyproxy.envoy.admin.v3.ClientResourceStatus.fromValue(__fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.scalapb.descriptors.EnumValueDescriptor]).getOrElse(io.envoyproxy.envoy.admin.v3.ClientResourceStatus.UNKNOWN.scalaValueDescriptor).number),
errorState = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).flatMap(_.as[_root_.scala.Option[io.envoyproxy.envoy.admin.v3.UpdateFailureState]]),
isStaticResource = __fieldsMap.get(scalaDescriptor.findFieldByNumber(9).get).map(_.as[_root_.scala.Boolean]).getOrElse(false)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = io.envoyproxy.envoy.service.status.v3.ClientConfig.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = io.envoyproxy.envoy.service.status.v3.ClientConfig.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 4 => __out = com.google.protobuf.any.Any
case 5 => __out = com.google.protobuf.timestamp.Timestamp
case 8 => __out = io.envoyproxy.envoy.admin.v3.UpdateFailureState
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 6 => io.envoyproxy.envoy.service.status.v3.ConfigStatus
case 7 => io.envoyproxy.envoy.admin.v3.ClientResourceStatus
}
}
lazy val defaultInstance = io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig(
typeUrl = "",
name = "",
versionInfo = "",
xdsConfig = _root_.scala.None,
lastUpdated = _root_.scala.None,
configStatus = io.envoyproxy.envoy.service.status.v3.ConfigStatus.UNKNOWN,
clientStatus = io.envoyproxy.envoy.admin.v3.ClientResourceStatus.UNKNOWN,
errorState = _root_.scala.None,
isStaticResource = false
)
implicit class GenericXdsConfigLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig](_l) {
def typeUrl: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.typeUrl)((c_, f_) => c_.copy(typeUrl = f_))
def name: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.name)((c_, f_) => c_.copy(name = f_))
def versionInfo: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.versionInfo)((c_, f_) => c_.copy(versionInfo = f_))
def xdsConfig: _root_.scalapb.lenses.Lens[UpperPB, com.google.protobuf.any.Any] = field(_.getXdsConfig)((c_, f_) => c_.copy(xdsConfig = Option(f_)))
def optionalXdsConfig: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.protobuf.any.Any]] = field(_.xdsConfig)((c_, f_) => c_.copy(xdsConfig = f_))
def lastUpdated: _root_.scalapb.lenses.Lens[UpperPB, com.google.protobuf.timestamp.Timestamp] = field(_.getLastUpdated)((c_, f_) => c_.copy(lastUpdated = Option(f_)))
def optionalLastUpdated: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[com.google.protobuf.timestamp.Timestamp]] = field(_.lastUpdated)((c_, f_) => c_.copy(lastUpdated = f_))
def configStatus: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.service.status.v3.ConfigStatus] = field(_.configStatus)((c_, f_) => c_.copy(configStatus = f_))
def clientStatus: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.admin.v3.ClientResourceStatus] = field(_.clientStatus)((c_, f_) => c_.copy(clientStatus = f_))
def errorState: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.admin.v3.UpdateFailureState] = field(_.getErrorState)((c_, f_) => c_.copy(errorState = Option(f_)))
def optionalErrorState: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[io.envoyproxy.envoy.admin.v3.UpdateFailureState]] = field(_.errorState)((c_, f_) => c_.copy(errorState = f_))
def isStaticResource: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.isStaticResource)((c_, f_) => c_.copy(isStaticResource = f_))
}
final val TYPE_URL_FIELD_NUMBER = 1
final val NAME_FIELD_NUMBER = 2
final val VERSION_INFO_FIELD_NUMBER = 3
final val XDS_CONFIG_FIELD_NUMBER = 4
final val LAST_UPDATED_FIELD_NUMBER = 5
final val CONFIG_STATUS_FIELD_NUMBER = 6
final val CLIENT_STATUS_FIELD_NUMBER = 7
final val ERROR_STATE_FIELD_NUMBER = 8
final val IS_STATIC_RESOURCE_FIELD_NUMBER = 9
def of(
typeUrl: _root_.scala.Predef.String,
name: _root_.scala.Predef.String,
versionInfo: _root_.scala.Predef.String,
xdsConfig: _root_.scala.Option[com.google.protobuf.any.Any],
lastUpdated: _root_.scala.Option[com.google.protobuf.timestamp.Timestamp],
configStatus: io.envoyproxy.envoy.service.status.v3.ConfigStatus,
clientStatus: io.envoyproxy.envoy.admin.v3.ClientResourceStatus,
errorState: _root_.scala.Option[io.envoyproxy.envoy.admin.v3.UpdateFailureState],
isStaticResource: _root_.scala.Boolean
): _root_.io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig = _root_.io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig(
typeUrl,
name,
versionInfo,
xdsConfig,
lastUpdated,
configStatus,
clientStatus,
errorState,
isStaticResource
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.service.status.v3.ClientConfig.GenericXdsConfig])
}
implicit class ClientConfigLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.service.status.v3.ClientConfig]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.envoyproxy.envoy.service.status.v3.ClientConfig](_l) {
def node: _root_.scalapb.lenses.Lens[UpperPB, io.envoyproxy.envoy.config.core.v3.Node] = field(_.getNode)((c_, f_) => c_.copy(node = Option(f_)))
def optionalNode: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[io.envoyproxy.envoy.config.core.v3.Node]] = field(_.node)((c_, f_) => c_.copy(node = f_))
def xdsConfig: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.envoyproxy.envoy.service.status.v3.PerXdsConfig]] = field(_.xdsConfig)((c_, f_) => c_.copy(xdsConfig = f_))
def genericXdsConfigs: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig]] = field(_.genericXdsConfigs)((c_, f_) => c_.copy(genericXdsConfigs = f_))
}
final val NODE_FIELD_NUMBER = 1
final val XDS_CONFIG_FIELD_NUMBER = 2
final val GENERIC_XDS_CONFIGS_FIELD_NUMBER = 3
def of(
node: _root_.scala.Option[io.envoyproxy.envoy.config.core.v3.Node],
xdsConfig: _root_.scala.Seq[io.envoyproxy.envoy.service.status.v3.PerXdsConfig],
genericXdsConfigs: _root_.scala.Seq[io.envoyproxy.envoy.service.status.v3.ClientConfig.GenericXdsConfig]
): _root_.io.envoyproxy.envoy.service.status.v3.ClientConfig = _root_.io.envoyproxy.envoy.service.status.v3.ClientConfig(
node,
xdsConfig,
genericXdsConfigs
)
// @@protoc_insertion_point(GeneratedMessageCompanion[envoy.service.status.v3.ClientConfig])
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy