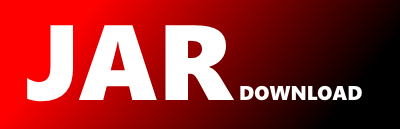
io.opentelemetry.proto.metrics.v1.SummaryDataPoint.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envoy-scala-control-plane_2.13 Show documentation
Show all versions of envoy-scala-control-plane_2.13 Show documentation
ScalaPB generated bindings for Envoy
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package io.opentelemetry.proto.metrics.v1
/** SummaryDataPoint is a single data point in a timeseries that describes the
* time-varying values of a Summary metric.
*
* @param attributes
* The set of key/value pairs that uniquely identify the timeseries from
* where this point belongs. The list may be empty (may contain 0 elements).
* Attribute keys MUST be unique (it is not allowed to have more than one
* attribute with the same key).
* @param startTimeUnixNano
* StartTimeUnixNano is optional but strongly encouraged, see the
* the detailed comments above Metric.
*
* Value is UNIX Epoch time in nanoseconds since 00:00:00 UTC on 1 January
* 1970.
* @param timeUnixNano
* TimeUnixNano is required, see the detailed comments above Metric.
*
* Value is UNIX Epoch time in nanoseconds since 00:00:00 UTC on 1 January
* 1970.
* @param count
* count is the number of values in the population. Must be non-negative.
* @param sum
* sum of the values in the population. If count is zero then this field
* must be zero.
*
* Note: Sum should only be filled out when measuring non-negative discrete
* events, and is assumed to be monotonic over the values of these events.
* Negative events *can* be recorded, but sum should not be filled out when
* doing so. This is specifically to enforce compatibility w/ OpenMetrics,
* see: https://github.com/OpenObservability/OpenMetrics/blob/main/specification/OpenMetrics.md#summary
* @param quantileValues
* (Optional) list of values at different quantiles of the distribution calculated
* from the current snapshot. The quantiles must be strictly increasing.
* @param flags
* Flags that apply to this specific data point. See DataPointFlags
* for the available flags and their meaning.
*/
@SerialVersionUID(0L)
final case class SummaryDataPoint(
attributes: _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue] = _root_.scala.Seq.empty,
startTimeUnixNano: _root_.scala.Long = 0L,
timeUnixNano: _root_.scala.Long = 0L,
count: _root_.scala.Long = 0L,
sum: _root_.scala.Double = 0.0,
quantileValues: _root_.scala.Seq[io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile] = _root_.scala.Seq.empty,
flags: _root_.scala.Int = 0,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[SummaryDataPoint] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
attributes.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = startTimeUnixNano
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeFixed64Size(2, __value)
}
};
{
val __value = timeUnixNano
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeFixed64Size(3, __value)
}
};
{
val __value = count
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeFixed64Size(4, __value)
}
};
{
val __value = sum
if (__value != 0.0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeDoubleSize(5, __value)
}
};
quantileValues.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = flags
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt32Size(8, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = startTimeUnixNano
if (__v != 0L) {
_output__.writeFixed64(2, __v)
}
};
{
val __v = timeUnixNano
if (__v != 0L) {
_output__.writeFixed64(3, __v)
}
};
{
val __v = count
if (__v != 0L) {
_output__.writeFixed64(4, __v)
}
};
{
val __v = sum
if (__v != 0.0) {
_output__.writeDouble(5, __v)
}
};
quantileValues.foreach { __v =>
val __m = __v
_output__.writeTag(6, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
attributes.foreach { __v =>
val __m = __v
_output__.writeTag(7, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = flags
if (__v != 0) {
_output__.writeUInt32(8, __v)
}
};
unknownFields.writeTo(_output__)
}
def clearAttributes = copy(attributes = _root_.scala.Seq.empty)
def addAttributes(__vs: io.opentelemetry.proto.common.v1.KeyValue *): SummaryDataPoint = addAllAttributes(__vs)
def addAllAttributes(__vs: Iterable[io.opentelemetry.proto.common.v1.KeyValue]): SummaryDataPoint = copy(attributes = attributes ++ __vs)
def withAttributes(__v: _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue]): SummaryDataPoint = copy(attributes = __v)
def withStartTimeUnixNano(__v: _root_.scala.Long): SummaryDataPoint = copy(startTimeUnixNano = __v)
def withTimeUnixNano(__v: _root_.scala.Long): SummaryDataPoint = copy(timeUnixNano = __v)
def withCount(__v: _root_.scala.Long): SummaryDataPoint = copy(count = __v)
def withSum(__v: _root_.scala.Double): SummaryDataPoint = copy(sum = __v)
def clearQuantileValues = copy(quantileValues = _root_.scala.Seq.empty)
def addQuantileValues(__vs: io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile *): SummaryDataPoint = addAllQuantileValues(__vs)
def addAllQuantileValues(__vs: Iterable[io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile]): SummaryDataPoint = copy(quantileValues = quantileValues ++ __vs)
def withQuantileValues(__v: _root_.scala.Seq[io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile]): SummaryDataPoint = copy(quantileValues = __v)
def withFlags(__v: _root_.scala.Int): SummaryDataPoint = copy(flags = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 7 => attributes
case 2 => {
val __t = startTimeUnixNano
if (__t != 0L) __t else null
}
case 3 => {
val __t = timeUnixNano
if (__t != 0L) __t else null
}
case 4 => {
val __t = count
if (__t != 0L) __t else null
}
case 5 => {
val __t = sum
if (__t != 0.0) __t else null
}
case 6 => quantileValues
case 8 => {
val __t = flags
if (__t != 0) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 7 => _root_.scalapb.descriptors.PRepeated(attributes.iterator.map(_.toPMessage).toVector)
case 2 => _root_.scalapb.descriptors.PLong(startTimeUnixNano)
case 3 => _root_.scalapb.descriptors.PLong(timeUnixNano)
case 4 => _root_.scalapb.descriptors.PLong(count)
case 5 => _root_.scalapb.descriptors.PDouble(sum)
case 6 => _root_.scalapb.descriptors.PRepeated(quantileValues.iterator.map(_.toPMessage).toVector)
case 8 => _root_.scalapb.descriptors.PInt(flags)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.opentelemetry.proto.metrics.v1.SummaryDataPoint.type = io.opentelemetry.proto.metrics.v1.SummaryDataPoint
// @@protoc_insertion_point(GeneratedMessage[opentelemetry.proto.metrics.v1.SummaryDataPoint])
}
object SummaryDataPoint extends scalapb.GeneratedMessageCompanion[io.opentelemetry.proto.metrics.v1.SummaryDataPoint] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.opentelemetry.proto.metrics.v1.SummaryDataPoint] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.opentelemetry.proto.metrics.v1.SummaryDataPoint = {
val __attributes: _root_.scala.collection.immutable.VectorBuilder[io.opentelemetry.proto.common.v1.KeyValue] = new _root_.scala.collection.immutable.VectorBuilder[io.opentelemetry.proto.common.v1.KeyValue]
var __startTimeUnixNano: _root_.scala.Long = 0L
var __timeUnixNano: _root_.scala.Long = 0L
var __count: _root_.scala.Long = 0L
var __sum: _root_.scala.Double = 0.0
val __quantileValues: _root_.scala.collection.immutable.VectorBuilder[io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile] = new _root_.scala.collection.immutable.VectorBuilder[io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile]
var __flags: _root_.scala.Int = 0
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 58 =>
__attributes += _root_.scalapb.LiteParser.readMessage[io.opentelemetry.proto.common.v1.KeyValue](_input__)
case 17 =>
__startTimeUnixNano = _input__.readFixed64()
case 25 =>
__timeUnixNano = _input__.readFixed64()
case 33 =>
__count = _input__.readFixed64()
case 41 =>
__sum = _input__.readDouble()
case 50 =>
__quantileValues += _root_.scalapb.LiteParser.readMessage[io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile](_input__)
case 64 =>
__flags = _input__.readUInt32()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.opentelemetry.proto.metrics.v1.SummaryDataPoint(
attributes = __attributes.result(),
startTimeUnixNano = __startTimeUnixNano,
timeUnixNano = __timeUnixNano,
count = __count,
sum = __sum,
quantileValues = __quantileValues.result(),
flags = __flags,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.opentelemetry.proto.metrics.v1.SummaryDataPoint] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.opentelemetry.proto.metrics.v1.SummaryDataPoint(
attributes = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue]]).getOrElse(_root_.scala.Seq.empty),
startTimeUnixNano = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
timeUnixNano = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
count = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
sum = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Double]).getOrElse(0.0),
quantileValues = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Seq[io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile]]).getOrElse(_root_.scala.Seq.empty),
flags = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).map(_.as[_root_.scala.Int]).getOrElse(0)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = MetricsProto.javaDescriptor.getMessageTypes().get(13)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = MetricsProto.scalaDescriptor.messages(13)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 7 => __out = io.opentelemetry.proto.common.v1.KeyValue
case 6 => __out = io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.opentelemetry.proto.metrics.v1.SummaryDataPoint(
attributes = _root_.scala.Seq.empty,
startTimeUnixNano = 0L,
timeUnixNano = 0L,
count = 0L,
sum = 0.0,
quantileValues = _root_.scala.Seq.empty,
flags = 0
)
/** Represents the value at a given quantile of a distribution.
*
* To record Min and Max values following conventions are used:
* - The 1.0 quantile is equivalent to the maximum value observed.
* - The 0.0 quantile is equivalent to the minimum value observed.
*
* See the following issue for more context:
* https://github.com/open-telemetry/opentelemetry-proto/issues/125
*
* @param quantile
* The quantile of a distribution. Must be in the interval
* [0.0, 1.0].
* @param value
* The value at the given quantile of a distribution.
*
* Quantile values must NOT be negative.
*/
@SerialVersionUID(0L)
final case class ValueAtQuantile(
quantile: _root_.scala.Double = 0.0,
value: _root_.scala.Double = 0.0,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[ValueAtQuantile] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = quantile
if (__value != 0.0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeDoubleSize(1, __value)
}
};
{
val __value = value
if (__value != 0.0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeDoubleSize(2, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = quantile
if (__v != 0.0) {
_output__.writeDouble(1, __v)
}
};
{
val __v = value
if (__v != 0.0) {
_output__.writeDouble(2, __v)
}
};
unknownFields.writeTo(_output__)
}
def withQuantile(__v: _root_.scala.Double): ValueAtQuantile = copy(quantile = __v)
def withValue(__v: _root_.scala.Double): ValueAtQuantile = copy(value = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = quantile
if (__t != 0.0) __t else null
}
case 2 => {
val __t = value
if (__t != 0.0) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PDouble(quantile)
case 2 => _root_.scalapb.descriptors.PDouble(value)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile.type = io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile
// @@protoc_insertion_point(GeneratedMessage[opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile])
}
object ValueAtQuantile extends scalapb.GeneratedMessageCompanion[io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile = {
var __quantile: _root_.scala.Double = 0.0
var __value: _root_.scala.Double = 0.0
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 9 =>
__quantile = _input__.readDouble()
case 17 =>
__value = _input__.readDouble()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile(
quantile = __quantile,
value = __value,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile(
quantile = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Double]).getOrElse(0.0),
value = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Double]).getOrElse(0.0)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = io.opentelemetry.proto.metrics.v1.SummaryDataPoint.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = io.opentelemetry.proto.metrics.v1.SummaryDataPoint.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile(
quantile = 0.0,
value = 0.0
)
implicit class ValueAtQuantileLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile](_l) {
def quantile: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Double] = field(_.quantile)((c_, f_) => c_.copy(quantile = f_))
def value: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Double] = field(_.value)((c_, f_) => c_.copy(value = f_))
}
final val QUANTILE_FIELD_NUMBER = 1
final val VALUE_FIELD_NUMBER = 2
def of(
quantile: _root_.scala.Double,
value: _root_.scala.Double
): _root_.io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile = _root_.io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile(
quantile,
value
)
// @@protoc_insertion_point(GeneratedMessageCompanion[opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile])
}
implicit class SummaryDataPointLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.opentelemetry.proto.metrics.v1.SummaryDataPoint]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.opentelemetry.proto.metrics.v1.SummaryDataPoint](_l) {
def attributes: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue]] = field(_.attributes)((c_, f_) => c_.copy(attributes = f_))
def startTimeUnixNano: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.startTimeUnixNano)((c_, f_) => c_.copy(startTimeUnixNano = f_))
def timeUnixNano: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.timeUnixNano)((c_, f_) => c_.copy(timeUnixNano = f_))
def count: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.count)((c_, f_) => c_.copy(count = f_))
def sum: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Double] = field(_.sum)((c_, f_) => c_.copy(sum = f_))
def quantileValues: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile]] = field(_.quantileValues)((c_, f_) => c_.copy(quantileValues = f_))
def flags: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.flags)((c_, f_) => c_.copy(flags = f_))
}
final val ATTRIBUTES_FIELD_NUMBER = 7
final val START_TIME_UNIX_NANO_FIELD_NUMBER = 2
final val TIME_UNIX_NANO_FIELD_NUMBER = 3
final val COUNT_FIELD_NUMBER = 4
final val SUM_FIELD_NUMBER = 5
final val QUANTILE_VALUES_FIELD_NUMBER = 6
final val FLAGS_FIELD_NUMBER = 8
def of(
attributes: _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue],
startTimeUnixNano: _root_.scala.Long,
timeUnixNano: _root_.scala.Long,
count: _root_.scala.Long,
sum: _root_.scala.Double,
quantileValues: _root_.scala.Seq[io.opentelemetry.proto.metrics.v1.SummaryDataPoint.ValueAtQuantile],
flags: _root_.scala.Int
): _root_.io.opentelemetry.proto.metrics.v1.SummaryDataPoint = _root_.io.opentelemetry.proto.metrics.v1.SummaryDataPoint(
attributes,
startTimeUnixNano,
timeUnixNano,
count,
sum,
quantileValues,
flags
)
// @@protoc_insertion_point(GeneratedMessageCompanion[opentelemetry.proto.metrics.v1.SummaryDataPoint])
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy