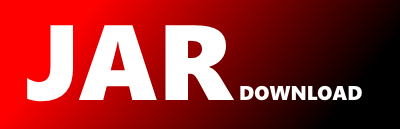
io.opentelemetry.proto.trace.v1.Span.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envoy-scala-control-plane_2.13 Show documentation
Show all versions of envoy-scala-control-plane_2.13 Show documentation
ScalaPB generated bindings for Envoy
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package io.opentelemetry.proto.trace.v1
/** Span represents a single operation within a trace. Spans can be
* nested to form a trace tree. Spans may also be linked to other spans
* from the same or different trace and form graphs. Often, a trace
* contains a root span that describes the end-to-end latency, and one
* or more subspans for its sub-operations. A trace can also contain
* multiple root spans, or none at all. Spans do not need to be
* contiguous - there may be gaps or overlaps between spans in a trace.
*
* The next available field id is 17.
*
* @param traceId
* A unique identifier for a trace. All spans from the same trace share
* the same `trace_id`. The ID is a 16-byte array. An ID with all zeroes
* is considered invalid.
*
* This field is semantically required. Receiver should generate new
* random trace_id if empty or invalid trace_id was received.
*
* This field is required.
* @param spanId
* A unique identifier for a span within a trace, assigned when the span
* is created. The ID is an 8-byte array. An ID with all zeroes is considered
* invalid.
*
* This field is semantically required. Receiver should generate new
* random span_id if empty or invalid span_id was received.
*
* This field is required.
* @param traceState
* trace_state conveys information about request position in multiple distributed tracing graphs.
* It is a trace_state in w3c-trace-context format: https://www.w3.org/TR/trace-context/#tracestate-header
* See also https://github.com/w3c/distributed-tracing for more details about this field.
* @param parentSpanId
* The `span_id` of this span's parent span. If this is a root span, then this
* field must be empty. The ID is an 8-byte array.
* @param name
* A description of the span's operation.
*
* For example, the name can be a qualified method name or a file name
* and a line number where the operation is called. A best practice is to use
* the same display name at the same call point in an application.
* This makes it easier to correlate spans in different traces.
*
* This field is semantically required to be set to non-empty string.
* Empty value is equivalent to an unknown span name.
*
* This field is required.
* @param kind
* Distinguishes between spans generated in a particular context. For example,
* two spans with the same name may be distinguished using `CLIENT` (caller)
* and `SERVER` (callee) to identify queueing latency associated with the span.
* @param startTimeUnixNano
* start_time_unix_nano is the start time of the span. On the client side, this is the time
* kept by the local machine where the span execution starts. On the server side, this
* is the time when the server's application handler starts running.
* Value is UNIX Epoch time in nanoseconds since 00:00:00 UTC on 1 January 1970.
*
* This field is semantically required and it is expected that end_time >= start_time.
* @param endTimeUnixNano
* end_time_unix_nano is the end time of the span. On the client side, this is the time
* kept by the local machine where the span execution ends. On the server side, this
* is the time when the server application handler stops running.
* Value is UNIX Epoch time in nanoseconds since 00:00:00 UTC on 1 January 1970.
*
* This field is semantically required and it is expected that end_time >= start_time.
* @param attributes
* attributes is a collection of key/value pairs. Note, global attributes
* like server name can be set using the resource API. Examples of attributes:
*
* "/http/user_agent": "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_14_2) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/71.0.3578.98 Safari/537.36"
* "/http/server_latency": 300
* "abc.com/myattribute": true
* "abc.com/score": 10.239
*
* The OpenTelemetry API specification further restricts the allowed value types:
* https://github.com/open-telemetry/opentelemetry-specification/blob/main/specification/common/common.md#attributes
* Attribute keys MUST be unique (it is not allowed to have more than one
* attribute with the same key).
* @param droppedAttributesCount
* dropped_attributes_count is the number of attributes that were discarded. Attributes
* can be discarded because their keys are too long or because there are too many
* attributes. If this value is 0, then no attributes were dropped.
* @param events
* events is a collection of Event items.
* @param droppedEventsCount
* dropped_events_count is the number of dropped events. If the value is 0, then no
* events were dropped.
* @param links
* links is a collection of Links, which are references from this span to a span
* in the same or different trace.
* @param droppedLinksCount
* dropped_links_count is the number of dropped links after the maximum size was
* enforced. If this value is 0, then no links were dropped.
* @param status
* An optional final status for this span. Semantically when Status isn't set, it means
* span's status code is unset, i.e. assume STATUS_CODE_UNSET (code = 0).
*/
@SerialVersionUID(0L)
final case class Span(
traceId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
spanId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
traceState: _root_.scala.Predef.String = "",
parentSpanId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
name: _root_.scala.Predef.String = "",
kind: io.opentelemetry.proto.trace.v1.Span.SpanKind = io.opentelemetry.proto.trace.v1.Span.SpanKind.SPAN_KIND_UNSPECIFIED,
startTimeUnixNano: _root_.scala.Long = 0L,
endTimeUnixNano: _root_.scala.Long = 0L,
attributes: _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue] = _root_.scala.Seq.empty,
droppedAttributesCount: _root_.scala.Int = 0,
events: _root_.scala.Seq[io.opentelemetry.proto.trace.v1.Span.Event] = _root_.scala.Seq.empty,
droppedEventsCount: _root_.scala.Int = 0,
links: _root_.scala.Seq[io.opentelemetry.proto.trace.v1.Span.Link] = _root_.scala.Seq.empty,
droppedLinksCount: _root_.scala.Int = 0,
status: _root_.scala.Option[io.opentelemetry.proto.trace.v1.Status] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Span] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = traceId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = spanId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(2, __value)
}
};
{
val __value = traceState
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(3, __value)
}
};
{
val __value = parentSpanId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(4, __value)
}
};
{
val __value = name
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(5, __value)
}
};
{
val __value = kind.value
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeEnumSize(6, __value)
}
};
{
val __value = startTimeUnixNano
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeFixed64Size(7, __value)
}
};
{
val __value = endTimeUnixNano
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeFixed64Size(8, __value)
}
};
attributes.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = droppedAttributesCount
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt32Size(10, __value)
}
};
events.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = droppedEventsCount
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt32Size(12, __value)
}
};
links.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = droppedLinksCount
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt32Size(14, __value)
}
};
if (status.isDefined) {
val __value = status.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = traceId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = spanId
if (!__v.isEmpty) {
_output__.writeBytes(2, __v)
}
};
{
val __v = traceState
if (!__v.isEmpty) {
_output__.writeString(3, __v)
}
};
{
val __v = parentSpanId
if (!__v.isEmpty) {
_output__.writeBytes(4, __v)
}
};
{
val __v = name
if (!__v.isEmpty) {
_output__.writeString(5, __v)
}
};
{
val __v = kind.value
if (__v != 0) {
_output__.writeEnum(6, __v)
}
};
{
val __v = startTimeUnixNano
if (__v != 0L) {
_output__.writeFixed64(7, __v)
}
};
{
val __v = endTimeUnixNano
if (__v != 0L) {
_output__.writeFixed64(8, __v)
}
};
attributes.foreach { __v =>
val __m = __v
_output__.writeTag(9, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = droppedAttributesCount
if (__v != 0) {
_output__.writeUInt32(10, __v)
}
};
events.foreach { __v =>
val __m = __v
_output__.writeTag(11, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = droppedEventsCount
if (__v != 0) {
_output__.writeUInt32(12, __v)
}
};
links.foreach { __v =>
val __m = __v
_output__.writeTag(13, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = droppedLinksCount
if (__v != 0) {
_output__.writeUInt32(14, __v)
}
};
status.foreach { __v =>
val __m = __v
_output__.writeTag(15, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withTraceId(__v: _root_.com.google.protobuf.ByteString): Span = copy(traceId = __v)
def withSpanId(__v: _root_.com.google.protobuf.ByteString): Span = copy(spanId = __v)
def withTraceState(__v: _root_.scala.Predef.String): Span = copy(traceState = __v)
def withParentSpanId(__v: _root_.com.google.protobuf.ByteString): Span = copy(parentSpanId = __v)
def withName(__v: _root_.scala.Predef.String): Span = copy(name = __v)
def withKind(__v: io.opentelemetry.proto.trace.v1.Span.SpanKind): Span = copy(kind = __v)
def withStartTimeUnixNano(__v: _root_.scala.Long): Span = copy(startTimeUnixNano = __v)
def withEndTimeUnixNano(__v: _root_.scala.Long): Span = copy(endTimeUnixNano = __v)
def clearAttributes = copy(attributes = _root_.scala.Seq.empty)
def addAttributes(__vs: io.opentelemetry.proto.common.v1.KeyValue *): Span = addAllAttributes(__vs)
def addAllAttributes(__vs: Iterable[io.opentelemetry.proto.common.v1.KeyValue]): Span = copy(attributes = attributes ++ __vs)
def withAttributes(__v: _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue]): Span = copy(attributes = __v)
def withDroppedAttributesCount(__v: _root_.scala.Int): Span = copy(droppedAttributesCount = __v)
def clearEvents = copy(events = _root_.scala.Seq.empty)
def addEvents(__vs: io.opentelemetry.proto.trace.v1.Span.Event *): Span = addAllEvents(__vs)
def addAllEvents(__vs: Iterable[io.opentelemetry.proto.trace.v1.Span.Event]): Span = copy(events = events ++ __vs)
def withEvents(__v: _root_.scala.Seq[io.opentelemetry.proto.trace.v1.Span.Event]): Span = copy(events = __v)
def withDroppedEventsCount(__v: _root_.scala.Int): Span = copy(droppedEventsCount = __v)
def clearLinks = copy(links = _root_.scala.Seq.empty)
def addLinks(__vs: io.opentelemetry.proto.trace.v1.Span.Link *): Span = addAllLinks(__vs)
def addAllLinks(__vs: Iterable[io.opentelemetry.proto.trace.v1.Span.Link]): Span = copy(links = links ++ __vs)
def withLinks(__v: _root_.scala.Seq[io.opentelemetry.proto.trace.v1.Span.Link]): Span = copy(links = __v)
def withDroppedLinksCount(__v: _root_.scala.Int): Span = copy(droppedLinksCount = __v)
def getStatus: io.opentelemetry.proto.trace.v1.Status = status.getOrElse(io.opentelemetry.proto.trace.v1.Status.defaultInstance)
def clearStatus: Span = copy(status = _root_.scala.None)
def withStatus(__v: io.opentelemetry.proto.trace.v1.Status): Span = copy(status = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = traceId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = spanId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 3 => {
val __t = traceState
if (__t != "") __t else null
}
case 4 => {
val __t = parentSpanId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 5 => {
val __t = name
if (__t != "") __t else null
}
case 6 => {
val __t = kind.javaValueDescriptor
if (__t.getNumber() != 0) __t else null
}
case 7 => {
val __t = startTimeUnixNano
if (__t != 0L) __t else null
}
case 8 => {
val __t = endTimeUnixNano
if (__t != 0L) __t else null
}
case 9 => attributes
case 10 => {
val __t = droppedAttributesCount
if (__t != 0) __t else null
}
case 11 => events
case 12 => {
val __t = droppedEventsCount
if (__t != 0) __t else null
}
case 13 => links
case 14 => {
val __t = droppedLinksCount
if (__t != 0) __t else null
}
case 15 => status.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(traceId)
case 2 => _root_.scalapb.descriptors.PByteString(spanId)
case 3 => _root_.scalapb.descriptors.PString(traceState)
case 4 => _root_.scalapb.descriptors.PByteString(parentSpanId)
case 5 => _root_.scalapb.descriptors.PString(name)
case 6 => _root_.scalapb.descriptors.PEnum(kind.scalaValueDescriptor)
case 7 => _root_.scalapb.descriptors.PLong(startTimeUnixNano)
case 8 => _root_.scalapb.descriptors.PLong(endTimeUnixNano)
case 9 => _root_.scalapb.descriptors.PRepeated(attributes.iterator.map(_.toPMessage).toVector)
case 10 => _root_.scalapb.descriptors.PInt(droppedAttributesCount)
case 11 => _root_.scalapb.descriptors.PRepeated(events.iterator.map(_.toPMessage).toVector)
case 12 => _root_.scalapb.descriptors.PInt(droppedEventsCount)
case 13 => _root_.scalapb.descriptors.PRepeated(links.iterator.map(_.toPMessage).toVector)
case 14 => _root_.scalapb.descriptors.PInt(droppedLinksCount)
case 15 => status.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.opentelemetry.proto.trace.v1.Span.type = io.opentelemetry.proto.trace.v1.Span
// @@protoc_insertion_point(GeneratedMessage[opentelemetry.proto.trace.v1.Span])
}
object Span extends scalapb.GeneratedMessageCompanion[io.opentelemetry.proto.trace.v1.Span] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.opentelemetry.proto.trace.v1.Span] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.opentelemetry.proto.trace.v1.Span = {
var __traceId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __spanId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __traceState: _root_.scala.Predef.String = ""
var __parentSpanId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __name: _root_.scala.Predef.String = ""
var __kind: io.opentelemetry.proto.trace.v1.Span.SpanKind = io.opentelemetry.proto.trace.v1.Span.SpanKind.SPAN_KIND_UNSPECIFIED
var __startTimeUnixNano: _root_.scala.Long = 0L
var __endTimeUnixNano: _root_.scala.Long = 0L
val __attributes: _root_.scala.collection.immutable.VectorBuilder[io.opentelemetry.proto.common.v1.KeyValue] = new _root_.scala.collection.immutable.VectorBuilder[io.opentelemetry.proto.common.v1.KeyValue]
var __droppedAttributesCount: _root_.scala.Int = 0
val __events: _root_.scala.collection.immutable.VectorBuilder[io.opentelemetry.proto.trace.v1.Span.Event] = new _root_.scala.collection.immutable.VectorBuilder[io.opentelemetry.proto.trace.v1.Span.Event]
var __droppedEventsCount: _root_.scala.Int = 0
val __links: _root_.scala.collection.immutable.VectorBuilder[io.opentelemetry.proto.trace.v1.Span.Link] = new _root_.scala.collection.immutable.VectorBuilder[io.opentelemetry.proto.trace.v1.Span.Link]
var __droppedLinksCount: _root_.scala.Int = 0
var __status: _root_.scala.Option[io.opentelemetry.proto.trace.v1.Status] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__traceId = _input__.readBytes()
case 18 =>
__spanId = _input__.readBytes()
case 26 =>
__traceState = _input__.readStringRequireUtf8()
case 34 =>
__parentSpanId = _input__.readBytes()
case 42 =>
__name = _input__.readStringRequireUtf8()
case 48 =>
__kind = io.opentelemetry.proto.trace.v1.Span.SpanKind.fromValue(_input__.readEnum())
case 57 =>
__startTimeUnixNano = _input__.readFixed64()
case 65 =>
__endTimeUnixNano = _input__.readFixed64()
case 74 =>
__attributes += _root_.scalapb.LiteParser.readMessage[io.opentelemetry.proto.common.v1.KeyValue](_input__)
case 80 =>
__droppedAttributesCount = _input__.readUInt32()
case 90 =>
__events += _root_.scalapb.LiteParser.readMessage[io.opentelemetry.proto.trace.v1.Span.Event](_input__)
case 96 =>
__droppedEventsCount = _input__.readUInt32()
case 106 =>
__links += _root_.scalapb.LiteParser.readMessage[io.opentelemetry.proto.trace.v1.Span.Link](_input__)
case 112 =>
__droppedLinksCount = _input__.readUInt32()
case 122 =>
__status = Option(__status.fold(_root_.scalapb.LiteParser.readMessage[io.opentelemetry.proto.trace.v1.Status](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.opentelemetry.proto.trace.v1.Span(
traceId = __traceId,
spanId = __spanId,
traceState = __traceState,
parentSpanId = __parentSpanId,
name = __name,
kind = __kind,
startTimeUnixNano = __startTimeUnixNano,
endTimeUnixNano = __endTimeUnixNano,
attributes = __attributes.result(),
droppedAttributesCount = __droppedAttributesCount,
events = __events.result(),
droppedEventsCount = __droppedEventsCount,
links = __links.result(),
droppedLinksCount = __droppedLinksCount,
status = __status,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.opentelemetry.proto.trace.v1.Span] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.opentelemetry.proto.trace.v1.Span(
traceId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
spanId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
traceState = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
parentSpanId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
name = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
kind = io.opentelemetry.proto.trace.v1.Span.SpanKind.fromValue(__fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scalapb.descriptors.EnumValueDescriptor]).getOrElse(io.opentelemetry.proto.trace.v1.Span.SpanKind.SPAN_KIND_UNSPECIFIED.scalaValueDescriptor).number),
startTimeUnixNano = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
endTimeUnixNano = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
attributes = __fieldsMap.get(scalaDescriptor.findFieldByNumber(9).get).map(_.as[_root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue]]).getOrElse(_root_.scala.Seq.empty),
droppedAttributesCount = __fieldsMap.get(scalaDescriptor.findFieldByNumber(10).get).map(_.as[_root_.scala.Int]).getOrElse(0),
events = __fieldsMap.get(scalaDescriptor.findFieldByNumber(11).get).map(_.as[_root_.scala.Seq[io.opentelemetry.proto.trace.v1.Span.Event]]).getOrElse(_root_.scala.Seq.empty),
droppedEventsCount = __fieldsMap.get(scalaDescriptor.findFieldByNumber(12).get).map(_.as[_root_.scala.Int]).getOrElse(0),
links = __fieldsMap.get(scalaDescriptor.findFieldByNumber(13).get).map(_.as[_root_.scala.Seq[io.opentelemetry.proto.trace.v1.Span.Link]]).getOrElse(_root_.scala.Seq.empty),
droppedLinksCount = __fieldsMap.get(scalaDescriptor.findFieldByNumber(14).get).map(_.as[_root_.scala.Int]).getOrElse(0),
status = __fieldsMap.get(scalaDescriptor.findFieldByNumber(15).get).flatMap(_.as[_root_.scala.Option[io.opentelemetry.proto.trace.v1.Status]])
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = TraceProto.javaDescriptor.getMessageTypes().get(4)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = TraceProto.scalaDescriptor.messages(4)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 9 => __out = io.opentelemetry.proto.common.v1.KeyValue
case 11 => __out = io.opentelemetry.proto.trace.v1.Span.Event
case 13 => __out = io.opentelemetry.proto.trace.v1.Span.Link
case 15 => __out = io.opentelemetry.proto.trace.v1.Status
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.io.opentelemetry.proto.trace.v1.Span.Event,
_root_.io.opentelemetry.proto.trace.v1.Span.Link
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 6 => io.opentelemetry.proto.trace.v1.Span.SpanKind
}
}
lazy val defaultInstance = io.opentelemetry.proto.trace.v1.Span(
traceId = _root_.com.google.protobuf.ByteString.EMPTY,
spanId = _root_.com.google.protobuf.ByteString.EMPTY,
traceState = "",
parentSpanId = _root_.com.google.protobuf.ByteString.EMPTY,
name = "",
kind = io.opentelemetry.proto.trace.v1.Span.SpanKind.SPAN_KIND_UNSPECIFIED,
startTimeUnixNano = 0L,
endTimeUnixNano = 0L,
attributes = _root_.scala.Seq.empty,
droppedAttributesCount = 0,
events = _root_.scala.Seq.empty,
droppedEventsCount = 0,
links = _root_.scala.Seq.empty,
droppedLinksCount = 0,
status = _root_.scala.None
)
/** SpanKind is the type of span. Can be used to specify additional relationships between spans
* in addition to a parent/child relationship.
*/
sealed abstract class SpanKind(val value: _root_.scala.Int) extends _root_.scalapb.GeneratedEnum {
type EnumType = SpanKind
def isSpanKindUnspecified: _root_.scala.Boolean = false
def isSpanKindInternal: _root_.scala.Boolean = false
def isSpanKindServer: _root_.scala.Boolean = false
def isSpanKindClient: _root_.scala.Boolean = false
def isSpanKindProducer: _root_.scala.Boolean = false
def isSpanKindConsumer: _root_.scala.Boolean = false
def companion: _root_.scalapb.GeneratedEnumCompanion[SpanKind] = io.opentelemetry.proto.trace.v1.Span.SpanKind
final def asRecognized: _root_.scala.Option[io.opentelemetry.proto.trace.v1.Span.SpanKind.Recognized] = if (isUnrecognized) _root_.scala.None else _root_.scala.Some(this.asInstanceOf[io.opentelemetry.proto.trace.v1.Span.SpanKind.Recognized])
}
object SpanKind extends _root_.scalapb.GeneratedEnumCompanion[SpanKind] {
sealed trait Recognized extends SpanKind
implicit def enumCompanion: _root_.scalapb.GeneratedEnumCompanion[SpanKind] = this
/** Unspecified. Do NOT use as default.
* Implementations MAY assume SpanKind to be INTERNAL when receiving UNSPECIFIED.
*/
@SerialVersionUID(0L)
case object SPAN_KIND_UNSPECIFIED extends SpanKind(0) with SpanKind.Recognized {
val index = 0
val name = "SPAN_KIND_UNSPECIFIED"
override def isSpanKindUnspecified: _root_.scala.Boolean = true
}
/** Indicates that the span represents an internal operation within an application,
* as opposed to an operation happening at the boundaries. Default value.
*/
@SerialVersionUID(0L)
case object SPAN_KIND_INTERNAL extends SpanKind(1) with SpanKind.Recognized {
val index = 1
val name = "SPAN_KIND_INTERNAL"
override def isSpanKindInternal: _root_.scala.Boolean = true
}
/** Indicates that the span covers server-side handling of an RPC or other
* remote network request.
*/
@SerialVersionUID(0L)
case object SPAN_KIND_SERVER extends SpanKind(2) with SpanKind.Recognized {
val index = 2
val name = "SPAN_KIND_SERVER"
override def isSpanKindServer: _root_.scala.Boolean = true
}
/** Indicates that the span describes a request to some remote service.
*/
@SerialVersionUID(0L)
case object SPAN_KIND_CLIENT extends SpanKind(3) with SpanKind.Recognized {
val index = 3
val name = "SPAN_KIND_CLIENT"
override def isSpanKindClient: _root_.scala.Boolean = true
}
/** Indicates that the span describes a producer sending a message to a broker.
* Unlike CLIENT and SERVER, there is often no direct critical path latency relationship
* between producer and consumer spans. A PRODUCER span ends when the message was accepted
* by the broker while the logical processing of the message might span a much longer time.
*/
@SerialVersionUID(0L)
case object SPAN_KIND_PRODUCER extends SpanKind(4) with SpanKind.Recognized {
val index = 4
val name = "SPAN_KIND_PRODUCER"
override def isSpanKindProducer: _root_.scala.Boolean = true
}
/** Indicates that the span describes consumer receiving a message from a broker.
* Like the PRODUCER kind, there is often no direct critical path latency relationship
* between producer and consumer spans.
*/
@SerialVersionUID(0L)
case object SPAN_KIND_CONSUMER extends SpanKind(5) with SpanKind.Recognized {
val index = 5
val name = "SPAN_KIND_CONSUMER"
override def isSpanKindConsumer: _root_.scala.Boolean = true
}
@SerialVersionUID(0L)
final case class Unrecognized(unrecognizedValue: _root_.scala.Int) extends SpanKind(unrecognizedValue) with _root_.scalapb.UnrecognizedEnum
lazy val values = scala.collection.immutable.Seq(SPAN_KIND_UNSPECIFIED, SPAN_KIND_INTERNAL, SPAN_KIND_SERVER, SPAN_KIND_CLIENT, SPAN_KIND_PRODUCER, SPAN_KIND_CONSUMER)
def fromValue(__value: _root_.scala.Int): SpanKind = __value match {
case 0 => SPAN_KIND_UNSPECIFIED
case 1 => SPAN_KIND_INTERNAL
case 2 => SPAN_KIND_SERVER
case 3 => SPAN_KIND_CLIENT
case 4 => SPAN_KIND_PRODUCER
case 5 => SPAN_KIND_CONSUMER
case __other => Unrecognized(__other)
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.EnumDescriptor = io.opentelemetry.proto.trace.v1.Span.javaDescriptor.getEnumTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.EnumDescriptor = io.opentelemetry.proto.trace.v1.Span.scalaDescriptor.enums(0)
}
/** Event is a time-stamped annotation of the span, consisting of user-supplied
* text description and key-value pairs.
*
* @param timeUnixNano
* time_unix_nano is the time the event occurred.
* @param name
* name of the event.
* This field is semantically required to be set to non-empty string.
* @param attributes
* attributes is a collection of attribute key/value pairs on the event.
* Attribute keys MUST be unique (it is not allowed to have more than one
* attribute with the same key).
* @param droppedAttributesCount
* dropped_attributes_count is the number of dropped attributes. If the value is 0,
* then no attributes were dropped.
*/
@SerialVersionUID(0L)
final case class Event(
timeUnixNano: _root_.scala.Long = 0L,
name: _root_.scala.Predef.String = "",
attributes: _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue] = _root_.scala.Seq.empty,
droppedAttributesCount: _root_.scala.Int = 0,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Event] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = timeUnixNano
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeFixed64Size(1, __value)
}
};
{
val __value = name
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(2, __value)
}
};
attributes.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = droppedAttributesCount
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt32Size(4, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = timeUnixNano
if (__v != 0L) {
_output__.writeFixed64(1, __v)
}
};
{
val __v = name
if (!__v.isEmpty) {
_output__.writeString(2, __v)
}
};
attributes.foreach { __v =>
val __m = __v
_output__.writeTag(3, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = droppedAttributesCount
if (__v != 0) {
_output__.writeUInt32(4, __v)
}
};
unknownFields.writeTo(_output__)
}
def withTimeUnixNano(__v: _root_.scala.Long): Event = copy(timeUnixNano = __v)
def withName(__v: _root_.scala.Predef.String): Event = copy(name = __v)
def clearAttributes = copy(attributes = _root_.scala.Seq.empty)
def addAttributes(__vs: io.opentelemetry.proto.common.v1.KeyValue *): Event = addAllAttributes(__vs)
def addAllAttributes(__vs: Iterable[io.opentelemetry.proto.common.v1.KeyValue]): Event = copy(attributes = attributes ++ __vs)
def withAttributes(__v: _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue]): Event = copy(attributes = __v)
def withDroppedAttributesCount(__v: _root_.scala.Int): Event = copy(droppedAttributesCount = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = timeUnixNano
if (__t != 0L) __t else null
}
case 2 => {
val __t = name
if (__t != "") __t else null
}
case 3 => attributes
case 4 => {
val __t = droppedAttributesCount
if (__t != 0) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PLong(timeUnixNano)
case 2 => _root_.scalapb.descriptors.PString(name)
case 3 => _root_.scalapb.descriptors.PRepeated(attributes.iterator.map(_.toPMessage).toVector)
case 4 => _root_.scalapb.descriptors.PInt(droppedAttributesCount)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.opentelemetry.proto.trace.v1.Span.Event.type = io.opentelemetry.proto.trace.v1.Span.Event
// @@protoc_insertion_point(GeneratedMessage[opentelemetry.proto.trace.v1.Span.Event])
}
object Event extends scalapb.GeneratedMessageCompanion[io.opentelemetry.proto.trace.v1.Span.Event] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.opentelemetry.proto.trace.v1.Span.Event] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.opentelemetry.proto.trace.v1.Span.Event = {
var __timeUnixNano: _root_.scala.Long = 0L
var __name: _root_.scala.Predef.String = ""
val __attributes: _root_.scala.collection.immutable.VectorBuilder[io.opentelemetry.proto.common.v1.KeyValue] = new _root_.scala.collection.immutable.VectorBuilder[io.opentelemetry.proto.common.v1.KeyValue]
var __droppedAttributesCount: _root_.scala.Int = 0
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 9 =>
__timeUnixNano = _input__.readFixed64()
case 18 =>
__name = _input__.readStringRequireUtf8()
case 26 =>
__attributes += _root_.scalapb.LiteParser.readMessage[io.opentelemetry.proto.common.v1.KeyValue](_input__)
case 32 =>
__droppedAttributesCount = _input__.readUInt32()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.opentelemetry.proto.trace.v1.Span.Event(
timeUnixNano = __timeUnixNano,
name = __name,
attributes = __attributes.result(),
droppedAttributesCount = __droppedAttributesCount,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.opentelemetry.proto.trace.v1.Span.Event] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.opentelemetry.proto.trace.v1.Span.Event(
timeUnixNano = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
name = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
attributes = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue]]).getOrElse(_root_.scala.Seq.empty),
droppedAttributesCount = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Int]).getOrElse(0)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = io.opentelemetry.proto.trace.v1.Span.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = io.opentelemetry.proto.trace.v1.Span.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 3 => __out = io.opentelemetry.proto.common.v1.KeyValue
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.opentelemetry.proto.trace.v1.Span.Event(
timeUnixNano = 0L,
name = "",
attributes = _root_.scala.Seq.empty,
droppedAttributesCount = 0
)
implicit class EventLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.opentelemetry.proto.trace.v1.Span.Event]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.opentelemetry.proto.trace.v1.Span.Event](_l) {
def timeUnixNano: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.timeUnixNano)((c_, f_) => c_.copy(timeUnixNano = f_))
def name: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.name)((c_, f_) => c_.copy(name = f_))
def attributes: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue]] = field(_.attributes)((c_, f_) => c_.copy(attributes = f_))
def droppedAttributesCount: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.droppedAttributesCount)((c_, f_) => c_.copy(droppedAttributesCount = f_))
}
final val TIME_UNIX_NANO_FIELD_NUMBER = 1
final val NAME_FIELD_NUMBER = 2
final val ATTRIBUTES_FIELD_NUMBER = 3
final val DROPPED_ATTRIBUTES_COUNT_FIELD_NUMBER = 4
def of(
timeUnixNano: _root_.scala.Long,
name: _root_.scala.Predef.String,
attributes: _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue],
droppedAttributesCount: _root_.scala.Int
): _root_.io.opentelemetry.proto.trace.v1.Span.Event = _root_.io.opentelemetry.proto.trace.v1.Span.Event(
timeUnixNano,
name,
attributes,
droppedAttributesCount
)
// @@protoc_insertion_point(GeneratedMessageCompanion[opentelemetry.proto.trace.v1.Span.Event])
}
/** A pointer from the current span to another span in the same trace or in a
* different trace. For example, this can be used in batching operations,
* where a single batch handler processes multiple requests from different
* traces or when the handler receives a request from a different project.
*
* @param traceId
* A unique identifier of a trace that this linked span is part of. The ID is a
* 16-byte array.
* @param spanId
* A unique identifier for the linked span. The ID is an 8-byte array.
* @param traceState
* The trace_state associated with the link.
* @param attributes
* attributes is a collection of attribute key/value pairs on the link.
* Attribute keys MUST be unique (it is not allowed to have more than one
* attribute with the same key).
* @param droppedAttributesCount
* dropped_attributes_count is the number of dropped attributes. If the value is 0,
* then no attributes were dropped.
*/
@SerialVersionUID(0L)
final case class Link(
traceId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
spanId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
traceState: _root_.scala.Predef.String = "",
attributes: _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue] = _root_.scala.Seq.empty,
droppedAttributesCount: _root_.scala.Int = 0,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Link] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = traceId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = spanId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(2, __value)
}
};
{
val __value = traceState
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(3, __value)
}
};
attributes.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = droppedAttributesCount
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt32Size(5, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = traceId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = spanId
if (!__v.isEmpty) {
_output__.writeBytes(2, __v)
}
};
{
val __v = traceState
if (!__v.isEmpty) {
_output__.writeString(3, __v)
}
};
attributes.foreach { __v =>
val __m = __v
_output__.writeTag(4, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = droppedAttributesCount
if (__v != 0) {
_output__.writeUInt32(5, __v)
}
};
unknownFields.writeTo(_output__)
}
def withTraceId(__v: _root_.com.google.protobuf.ByteString): Link = copy(traceId = __v)
def withSpanId(__v: _root_.com.google.protobuf.ByteString): Link = copy(spanId = __v)
def withTraceState(__v: _root_.scala.Predef.String): Link = copy(traceState = __v)
def clearAttributes = copy(attributes = _root_.scala.Seq.empty)
def addAttributes(__vs: io.opentelemetry.proto.common.v1.KeyValue *): Link = addAllAttributes(__vs)
def addAllAttributes(__vs: Iterable[io.opentelemetry.proto.common.v1.KeyValue]): Link = copy(attributes = attributes ++ __vs)
def withAttributes(__v: _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue]): Link = copy(attributes = __v)
def withDroppedAttributesCount(__v: _root_.scala.Int): Link = copy(droppedAttributesCount = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = traceId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = spanId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 3 => {
val __t = traceState
if (__t != "") __t else null
}
case 4 => attributes
case 5 => {
val __t = droppedAttributesCount
if (__t != 0) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(traceId)
case 2 => _root_.scalapb.descriptors.PByteString(spanId)
case 3 => _root_.scalapb.descriptors.PString(traceState)
case 4 => _root_.scalapb.descriptors.PRepeated(attributes.iterator.map(_.toPMessage).toVector)
case 5 => _root_.scalapb.descriptors.PInt(droppedAttributesCount)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: io.opentelemetry.proto.trace.v1.Span.Link.type = io.opentelemetry.proto.trace.v1.Span.Link
// @@protoc_insertion_point(GeneratedMessage[opentelemetry.proto.trace.v1.Span.Link])
}
object Link extends scalapb.GeneratedMessageCompanion[io.opentelemetry.proto.trace.v1.Span.Link] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[io.opentelemetry.proto.trace.v1.Span.Link] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): io.opentelemetry.proto.trace.v1.Span.Link = {
var __traceId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __spanId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __traceState: _root_.scala.Predef.String = ""
val __attributes: _root_.scala.collection.immutable.VectorBuilder[io.opentelemetry.proto.common.v1.KeyValue] = new _root_.scala.collection.immutable.VectorBuilder[io.opentelemetry.proto.common.v1.KeyValue]
var __droppedAttributesCount: _root_.scala.Int = 0
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__traceId = _input__.readBytes()
case 18 =>
__spanId = _input__.readBytes()
case 26 =>
__traceState = _input__.readStringRequireUtf8()
case 34 =>
__attributes += _root_.scalapb.LiteParser.readMessage[io.opentelemetry.proto.common.v1.KeyValue](_input__)
case 40 =>
__droppedAttributesCount = _input__.readUInt32()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
io.opentelemetry.proto.trace.v1.Span.Link(
traceId = __traceId,
spanId = __spanId,
traceState = __traceState,
attributes = __attributes.result(),
droppedAttributesCount = __droppedAttributesCount,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[io.opentelemetry.proto.trace.v1.Span.Link] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
io.opentelemetry.proto.trace.v1.Span.Link(
traceId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
spanId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
traceState = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
attributes = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue]]).getOrElse(_root_.scala.Seq.empty),
droppedAttributesCount = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Int]).getOrElse(0)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = io.opentelemetry.proto.trace.v1.Span.javaDescriptor.getNestedTypes().get(1)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = io.opentelemetry.proto.trace.v1.Span.scalaDescriptor.nestedMessages(1)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 4 => __out = io.opentelemetry.proto.common.v1.KeyValue
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = io.opentelemetry.proto.trace.v1.Span.Link(
traceId = _root_.com.google.protobuf.ByteString.EMPTY,
spanId = _root_.com.google.protobuf.ByteString.EMPTY,
traceState = "",
attributes = _root_.scala.Seq.empty,
droppedAttributesCount = 0
)
implicit class LinkLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.opentelemetry.proto.trace.v1.Span.Link]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.opentelemetry.proto.trace.v1.Span.Link](_l) {
def traceId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.traceId)((c_, f_) => c_.copy(traceId = f_))
def spanId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.spanId)((c_, f_) => c_.copy(spanId = f_))
def traceState: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.traceState)((c_, f_) => c_.copy(traceState = f_))
def attributes: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue]] = field(_.attributes)((c_, f_) => c_.copy(attributes = f_))
def droppedAttributesCount: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.droppedAttributesCount)((c_, f_) => c_.copy(droppedAttributesCount = f_))
}
final val TRACE_ID_FIELD_NUMBER = 1
final val SPAN_ID_FIELD_NUMBER = 2
final val TRACE_STATE_FIELD_NUMBER = 3
final val ATTRIBUTES_FIELD_NUMBER = 4
final val DROPPED_ATTRIBUTES_COUNT_FIELD_NUMBER = 5
def of(
traceId: _root_.com.google.protobuf.ByteString,
spanId: _root_.com.google.protobuf.ByteString,
traceState: _root_.scala.Predef.String,
attributes: _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue],
droppedAttributesCount: _root_.scala.Int
): _root_.io.opentelemetry.proto.trace.v1.Span.Link = _root_.io.opentelemetry.proto.trace.v1.Span.Link(
traceId,
spanId,
traceState,
attributes,
droppedAttributesCount
)
// @@protoc_insertion_point(GeneratedMessageCompanion[opentelemetry.proto.trace.v1.Span.Link])
}
implicit class SpanLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, io.opentelemetry.proto.trace.v1.Span]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, io.opentelemetry.proto.trace.v1.Span](_l) {
def traceId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.traceId)((c_, f_) => c_.copy(traceId = f_))
def spanId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.spanId)((c_, f_) => c_.copy(spanId = f_))
def traceState: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.traceState)((c_, f_) => c_.copy(traceState = f_))
def parentSpanId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.parentSpanId)((c_, f_) => c_.copy(parentSpanId = f_))
def name: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.name)((c_, f_) => c_.copy(name = f_))
def kind: _root_.scalapb.lenses.Lens[UpperPB, io.opentelemetry.proto.trace.v1.Span.SpanKind] = field(_.kind)((c_, f_) => c_.copy(kind = f_))
def startTimeUnixNano: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.startTimeUnixNano)((c_, f_) => c_.copy(startTimeUnixNano = f_))
def endTimeUnixNano: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.endTimeUnixNano)((c_, f_) => c_.copy(endTimeUnixNano = f_))
def attributes: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue]] = field(_.attributes)((c_, f_) => c_.copy(attributes = f_))
def droppedAttributesCount: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.droppedAttributesCount)((c_, f_) => c_.copy(droppedAttributesCount = f_))
def events: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.opentelemetry.proto.trace.v1.Span.Event]] = field(_.events)((c_, f_) => c_.copy(events = f_))
def droppedEventsCount: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.droppedEventsCount)((c_, f_) => c_.copy(droppedEventsCount = f_))
def links: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[io.opentelemetry.proto.trace.v1.Span.Link]] = field(_.links)((c_, f_) => c_.copy(links = f_))
def droppedLinksCount: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.droppedLinksCount)((c_, f_) => c_.copy(droppedLinksCount = f_))
def status: _root_.scalapb.lenses.Lens[UpperPB, io.opentelemetry.proto.trace.v1.Status] = field(_.getStatus)((c_, f_) => c_.copy(status = Option(f_)))
def optionalStatus: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[io.opentelemetry.proto.trace.v1.Status]] = field(_.status)((c_, f_) => c_.copy(status = f_))
}
final val TRACE_ID_FIELD_NUMBER = 1
final val SPAN_ID_FIELD_NUMBER = 2
final val TRACE_STATE_FIELD_NUMBER = 3
final val PARENT_SPAN_ID_FIELD_NUMBER = 4
final val NAME_FIELD_NUMBER = 5
final val KIND_FIELD_NUMBER = 6
final val START_TIME_UNIX_NANO_FIELD_NUMBER = 7
final val END_TIME_UNIX_NANO_FIELD_NUMBER = 8
final val ATTRIBUTES_FIELD_NUMBER = 9
final val DROPPED_ATTRIBUTES_COUNT_FIELD_NUMBER = 10
final val EVENTS_FIELD_NUMBER = 11
final val DROPPED_EVENTS_COUNT_FIELD_NUMBER = 12
final val LINKS_FIELD_NUMBER = 13
final val DROPPED_LINKS_COUNT_FIELD_NUMBER = 14
final val STATUS_FIELD_NUMBER = 15
def of(
traceId: _root_.com.google.protobuf.ByteString,
spanId: _root_.com.google.protobuf.ByteString,
traceState: _root_.scala.Predef.String,
parentSpanId: _root_.com.google.protobuf.ByteString,
name: _root_.scala.Predef.String,
kind: io.opentelemetry.proto.trace.v1.Span.SpanKind,
startTimeUnixNano: _root_.scala.Long,
endTimeUnixNano: _root_.scala.Long,
attributes: _root_.scala.Seq[io.opentelemetry.proto.common.v1.KeyValue],
droppedAttributesCount: _root_.scala.Int,
events: _root_.scala.Seq[io.opentelemetry.proto.trace.v1.Span.Event],
droppedEventsCount: _root_.scala.Int,
links: _root_.scala.Seq[io.opentelemetry.proto.trace.v1.Span.Link],
droppedLinksCount: _root_.scala.Int,
status: _root_.scala.Option[io.opentelemetry.proto.trace.v1.Status]
): _root_.io.opentelemetry.proto.trace.v1.Span = _root_.io.opentelemetry.proto.trace.v1.Span(
traceId,
spanId,
traceState,
parentSpanId,
name,
kind,
startTimeUnixNano,
endTimeUnixNano,
attributes,
droppedAttributesCount,
events,
droppedEventsCount,
links,
droppedLinksCount,
status
)
// @@protoc_insertion_point(GeneratedMessageCompanion[opentelemetry.proto.trace.v1.Span])
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy