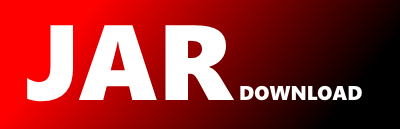
dagger.internal.MapFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dapper Show documentation
Show all versions of dapper Show documentation
dapper annotations and utils
/*
* Copyright (C) 2014 The Dagger Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package dagger.internal;
import static dagger.internal.DaggerCollections.newLinkedHashMapWithExpectedSize;
import static java.util.Collections.unmodifiableMap;
import java.util.Collections;
import java.util.Map;
import java.util.Map.Entry;
import jakarta.inject.Provider;
/**
* A {@link Factory} implementation used to implement {@link Map} bindings. This factory returns a
* {@code Map} when calling {@link #get} (as specified by {@link Factory}).
*/
public final class MapFactory extends AbstractMapFactory {
private static final Provider
© 2015 - 2025 Weber Informatics LLC | Privacy Policy