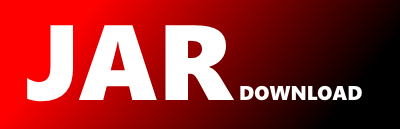
io.github.jeddict.jpa.modeler.properties.entitygraph.ExecutionGraphPanel Maven / Gradle / Ivy
/**
* Copyright 2013-2022 the original author or authors from the Jeddict project (https://jeddict.github.io/).
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package io.github.jeddict.jpa.modeler.properties.entitygraph;
import javax.swing.SwingUtilities;
import io.github.jeddict.jpa.modeler.widget.EntityWidget;
import io.github.jeddict.jpa.modeler.properties.entitygraph.nodes.EGRootNode;
import io.github.jeddict.jpa.spec.NamedEntityGraph;
import org.netbeans.modeler.properties.window.GenericDialog;
import org.openide.explorer.ExplorerManager;
import org.openide.explorer.view.OutlineView;
public class ExecutionGraphPanel extends GenericDialog implements ExplorerManager.Provider {
private final ExplorerManager manager;
private final NamedEntityGraph namedEntityGraph;
private final EntityWidget entityWidget;
private EGRootNode node;
public ExecutionGraphPanel(EntityWidget entityWidget, NamedEntityGraph namedEntityGraph) {
this.entityWidget = entityWidget;
this.namedEntityGraph = namedEntityGraph;
manager = new ExplorerManager();
initComponents();
this.setTitle("Execution graph");
loadExecutionGraph();
}
private void loadExecutionGraph() {
boolean loadGraph = "Load graph".equals(hintType_Combobox.getSelectedItem());
SwingUtilities.invokeLater(() -> {
node = new EGRootNode(entityWidget, namedEntityGraph, new ExecutionEGChildFactory(loadGraph));
manager.setRootContext(node);
node.init();
});
}
@Override
public ExplorerManager getExplorerManager() {
return manager;
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
jLayeredPane1 = new javax.swing.JLayeredPane();
hintType_LayeredPane = new javax.swing.JLayeredPane();
hintType_Label = new javax.swing.JLabel();
hintType_Combobox = new javax.swing.JComboBox();
close_Button = new javax.swing.JButton();
outlineView = new OutlineView("Execution Graph");
setDefaultCloseOperation(javax.swing.WindowConstants.DISPOSE_ON_CLOSE);
org.openide.awt.Mnemonics.setLocalizedText(hintType_Label, org.openide.util.NbBundle.getMessage(ExecutionGraphPanel.class, "ExecutionGraphPanel.hintType_Label.text")); // NOI18N
hintType_Combobox.setModel(new javax.swing.DefaultComboBoxModel(new String[] { "Load graph", "Fetch graph" }));
hintType_Combobox.setToolTipText(org.openide.util.NbBundle.getMessage(ExecutionGraphPanel.class, "ExecutionGraphPanel.hintType_Combobox.toolTipText")); // NOI18N
hintType_Combobox.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
hintType_ComboboxActionPerformed(evt);
}
});
org.openide.awt.Mnemonics.setLocalizedText(close_Button, org.openide.util.NbBundle.getMessage(ExecutionGraphPanel.class, "ExecutionGraphPanel.close_Button.text")); // NOI18N
close_Button.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
close_ButtonActionPerformed(evt);
}
});
javax.swing.GroupLayout hintType_LayeredPaneLayout = new javax.swing.GroupLayout(hintType_LayeredPane);
hintType_LayeredPane.setLayout(hintType_LayeredPaneLayout);
hintType_LayeredPaneLayout.setHorizontalGroup(
hintType_LayeredPaneLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(hintType_LayeredPaneLayout.createSequentialGroup()
.addContainerGap()
.addComponent(hintType_Label)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(hintType_Combobox, javax.swing.GroupLayout.PREFERRED_SIZE, 116, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(close_Button)
.addContainerGap())
);
hintType_LayeredPaneLayout.setVerticalGroup(
hintType_LayeredPaneLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(hintType_LayeredPaneLayout.createSequentialGroup()
.addGroup(hintType_LayeredPaneLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(hintType_Label)
.addGroup(hintType_LayeredPaneLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(hintType_Combobox, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(close_Button)))
.addGap(0, 7, Short.MAX_VALUE))
);
hintType_LayeredPane.setLayer(hintType_Label, javax.swing.JLayeredPane.DEFAULT_LAYER);
hintType_LayeredPane.setLayer(hintType_Combobox, javax.swing.JLayeredPane.DEFAULT_LAYER);
hintType_LayeredPane.setLayer(close_Button, javax.swing.JLayeredPane.DEFAULT_LAYER);
//outlineView.setDefaultActionAllowed(false);
//outlineView.setDoubleBuffered(true);
//outlineView.setDragSource(false);
//outlineView.setDropTarget(false)
javax.swing.GroupLayout jLayeredPane1Layout = new javax.swing.GroupLayout(jLayeredPane1);
jLayeredPane1.setLayout(jLayeredPane1Layout);
jLayeredPane1Layout.setHorizontalGroup(
jLayeredPane1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jLayeredPane1Layout.createSequentialGroup()
.addComponent(outlineView, javax.swing.GroupLayout.PREFERRED_SIZE, 480, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 0, Short.MAX_VALUE))
.addComponent(hintType_LayeredPane)
);
jLayeredPane1Layout.setVerticalGroup(
jLayeredPane1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jLayeredPane1Layout.createSequentialGroup()
.addComponent(outlineView, javax.swing.GroupLayout.PREFERRED_SIZE, 384, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(hintType_LayeredPane, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
);
jLayeredPane1.setLayer(hintType_LayeredPane, javax.swing.JLayeredPane.DEFAULT_LAYER);
jLayeredPane1.setLayer(outlineView, javax.swing.JLayeredPane.DEFAULT_LAYER);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLayeredPane1)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLayeredPane1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
);
pack();
}// //GEN-END:initComponents
private void hintType_ComboboxActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_hintType_ComboboxActionPerformed
loadExecutionGraph();
}//GEN-LAST:event_hintType_ComboboxActionPerformed
private void close_ButtonActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_close_ButtonActionPerformed
cancelActionPerformed(evt);
}//GEN-LAST:event_close_ButtonActionPerformed
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton close_Button;
private javax.swing.JComboBox hintType_Combobox;
private javax.swing.JLabel hintType_Label;
private javax.swing.JLayeredPane hintType_LayeredPane;
private javax.swing.JLayeredPane jLayeredPane1;
private javax.swing.JScrollPane outlineView;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy