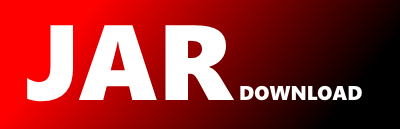
org.netbeans.modeler.core.NBModelerUtil Maven / Gradle / Ivy
/**
* Copyright [2018] Gaurav Gupta
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package org.netbeans.modeler.core;
import java.awt.Cursor;
import java.awt.Graphics2D;
import java.awt.Image;
import java.awt.Point;
import java.awt.Rectangle;
import java.awt.Toolkit;
import java.awt.geom.AffineTransform;
import java.lang.reflect.Field;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Date;
import java.util.HashSet;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import javax.lang.model.element.TypeElement;
import javax.swing.JComponent;
import javax.swing.JEditorPane;
import javax.swing.JScrollPane;
import org.netbeans.api.java.source.ClassIndex;
import org.netbeans.api.java.source.ClasspathInfo;
import org.netbeans.api.java.source.ElementHandle;
import org.netbeans.api.java.source.ui.TypeElementFinder;
import org.netbeans.api.visual.graph.GraphScene;
import org.netbeans.api.visual.widget.ConnectionWidget;
import org.netbeans.api.visual.widget.Scene;
import org.netbeans.api.visual.widget.Widget;
import org.netbeans.editor.Utilities;
import org.netbeans.modeler.resource.toolbar.ImageUtil;
import org.netbeans.modeler.specification.model.document.IModelerScene;
import org.netbeans.modeler.specification.model.document.INModelerScene;
import org.netbeans.modeler.widget.connection.relation.IRelationProxy;
import org.netbeans.modeler.widget.connection.relation.IRelationValidator;
import org.netbeans.modeler.widget.connection.relation.RelationProxy;
import org.netbeans.modeler.widget.node.INodeWidget;
import org.netbeans.modeler.widget.node.IWidget;
import org.netbeans.modeler.widget.node.NodeWidgetStatus;
import org.openide.util.Exceptions;
import org.openide.util.Pair;
public class NBModelerUtil {
private static Cursor validConnection;
private static Cursor invalidConnection;
private static int sequence = 0;
public static void loadModelerFile(ModelerFile file) throws Exception {
file.getModelerUtil().loadModelerFile(file);
}
public static void init(ModelerFile file) {
file.getModelerUtil().init();
}
/**
* BUG FIX Method : Remove all resize border
*
* @param file
*/
public static void hideAllResizeBorder(ModelerFile file) {
/* BUG : On Save if any widget is Selected with resize border then [NodeWidget + border] width is calculated as bound */
/*BUG Fix Start : Hide Resize border of all selected NodeWidget*/
IModelerScene scene = file.getModelerScene();
boolean validate = false;
for (Object o : scene.getSelectedObjects()) {
if (scene.isNode(o)) {
Widget w = scene.findWidget(o);
if (w instanceof INodeWidget) {
INodeWidget nodeWidget = (INodeWidget) w;
if (nodeWidget.getWidgetBorder() instanceof org.netbeans.modeler.border.ResizeBorder) {
nodeWidget.hideResizeBorder();
validate = true;
}
}
}
}
if (validate) {
scene.validate();
}
/*BUG Fix End*/
}
public static void saveModelerFile(ModelerFile file) {
if (file.getModelerScene() instanceof INModelerScene) {//not required for IPModelerScene because no manual resize border
NBModelerUtil.hideAllResizeBorder(file);
}
file.getModelerUtil().saveModelerFile(file);
}
public static String getAutoGeneratedStringId() {
return "_" + new Date().getTime() + ++sequence;
}
public static void hideContextPalette(IModelerScene scene) {
if (scene.getContextPaletteManager() != null) {
scene.getContextPaletteManager().cancelPalette();
}
}
public static void showContextPalette(IModelerScene scene, IWidget widget) {
if (scene.getContextPaletteManager() != null) {
scene.getContextPaletteManager().selectionChanged(widget, ((Widget) widget).convertLocalToScene(((Widget) widget).getLocation()));
}
}
public static boolean isValidRelationship(IRelationValidator relationValidator, INodeWidget from, INodeWidget to, String edgeType, Boolean changeStatus) {//gg2
IRelationProxy relationshipProxy = new RelationProxy();
relationshipProxy.setSource(from);
relationshipProxy.setTarget(to);
relationshipProxy.setEdgeType(edgeType);
boolean retVal = relationValidator.validateRelation(relationshipProxy);
if (changeStatus) {
if (retVal) {
relationshipProxy.getTarget().setStatus(NodeWidgetStatus.VALID);
} else {
relationshipProxy.getTarget().setStatus(NodeWidgetStatus.INVALID);
}
JComponent comp = (JComponent) relationshipProxy.getTarget().getModelerScene().getModelerPanelTopComponent();
if (retVal) {
if (comp.getCursor() != getValidConnectCursor()) {
comp.setCursor(getValidConnectCursor());
}
} else if (comp.getCursor() != getInvalidConnectCursor()) {
comp.setCursor(getInvalidConnectCursor());
}
}
return retVal;
}
public static Collection getAllContainedEdges(Widget widget) {
HashSet set = new HashSet<>();
Scene scene = widget.getScene();
if (scene instanceof GraphScene) {
GraphScene gs = (GraphScene) scene;
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy