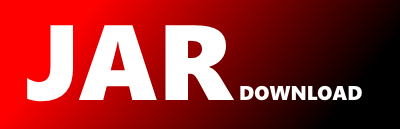
org.netbeans.modeler.properties.customattr.CustomAttributeDialog Maven / Gradle / Ivy
Go to download
Jeddict is an open source Jakarta EE application development platform that accelerates developers productivity and simplifies development tasks of creating complex entity relationship models.
/**
* Copyright 2013-2022 Gaurav Gupta
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package org.netbeans.modeler.properties.customattr;
import java.util.List;
import java.util.regex.Pattern;
import org.netbeans.modeler.locale.I18n;
/**
*
*
*/
public class CustomAttributeDialog extends javax.swing.JDialog {
GenericProperty tmpField = null;
private List properties = null;
private String originalName = null;
public CustomAttributeDialog(java.awt.Frame parent, boolean modal) {
super(parent, modal);
initComponents();
javax.swing.KeyStroke escape = javax.swing.KeyStroke.getKeyStroke(java.awt.event.KeyEvent.VK_ESCAPE, 0, false);
javax.swing.Action escapeAction = new javax.swing.AbstractAction() {
@Override
public void actionPerformed(java.awt.event.ActionEvent e) {
jButtonCancelActionPerformed(e);
}
};
getRootPane().getInputMap(javax.swing.JComponent.WHEN_IN_FOCUSED_WINDOW).put(escape, I18n.getString("Global.Pane.Escape"));
getRootPane().getActionMap().put(I18n.getString("Global.Pane.Escape"), escapeAction);
getRootPane().setDefaultButton(this.jButtonOK);
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
java.awt.GridBagConstraints gridBagConstraints;
jLabel1 = new javax.swing.JLabel();
jTextFieldName = new javax.swing.JTextField();
jLabel4 = new javax.swing.JLabel();
jPanelExpression = new javax.swing.JPanel();
jScrollPane1 = new javax.swing.JScrollPane();
jTextAreaDescription = new javax.swing.JTextArea();
jPanel1 = new javax.swing.JPanel();
jButtonOK = new javax.swing.JButton();
jButtonCancel = new javax.swing.JButton();
setTitle("Add/modify property");
setModal(true);
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent evt) {
closeDialog(evt);
}
});
getContentPane().setLayout(new java.awt.GridBagLayout());
jLabel1.setText("Attribute name");
jLabel1.setToolTipText("Custom Attribute name");
jLabel1.setMaximumSize(new java.awt.Dimension(1000, 100));
jLabel1.setMinimumSize(new java.awt.Dimension(100, 15));
jLabel1.setPreferredSize(new java.awt.Dimension(100, 15));
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.anchor = java.awt.GridBagConstraints.SOUTHWEST;
gridBagConstraints.insets = new java.awt.Insets(0, 4, 0, 4);
getContentPane().add(jLabel1, gridBagConstraints);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.anchor = java.awt.GridBagConstraints.SOUTHWEST;
gridBagConstraints.insets = new java.awt.Insets(0, 4, 0, 4);
getContentPane().add(jTextFieldName, gridBagConstraints);
jLabel4.setText("Attribute value");
jLabel4.setToolTipText("Custom Attribute value");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.insets = new java.awt.Insets(15, 4, 0, 4);
getContentPane().add(jLabel4, gridBagConstraints);
jPanelExpression.setMinimumSize(new java.awt.Dimension(10, 50));
jPanelExpression.setLayout(new java.awt.BorderLayout());
jScrollPane1.setMinimumSize(new java.awt.Dimension(200, 40));
jScrollPane1.setPreferredSize(new java.awt.Dimension(200, 160));
jTextAreaDescription.setMinimumSize(new java.awt.Dimension(0, 64));
jTextAreaDescription.setPreferredSize(new java.awt.Dimension(0, 64));
jScrollPane1.setViewportView(jTextAreaDescription);
jPanelExpression.add(jScrollPane1, java.awt.BorderLayout.CENTER);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.insets = new java.awt.Insets(0, 4, 0, 4);
getContentPane().add(jPanelExpression, gridBagConstraints);
jPanel1.setMinimumSize(new java.awt.Dimension(200, 35));
jPanel1.setPreferredSize(new java.awt.Dimension(250, 35));
jPanel1.setLayout(new java.awt.FlowLayout(java.awt.FlowLayout.RIGHT));
jButtonOK.setMnemonic('o');
jButtonOK.setText("OK");
jButtonOK.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButtonOKActionPerformed(evt);
}
});
jPanel1.add(jButtonOK);
jButtonCancel.setMnemonic('c');
jButtonCancel.setText("Cancel");
jButtonCancel.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButtonCancelActionPerformed(evt);
}
});
jPanel1.add(jButtonCancel);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.anchor = java.awt.GridBagConstraints.SOUTH;
gridBagConstraints.insets = new java.awt.Insets(10, 0, 0, 0);
getContentPane().add(jPanel1, gridBagConstraints);
setSize(new java.awt.Dimension(437, 323));
setLocationRelativeTo(null);
}// //GEN-END:initComponents
private void jButtonCancelActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButtonCancelActionPerformed
setVisible(false);
this.setDialogResult(javax.swing.JOptionPane.CANCEL_OPTION);
dispose();
}//GEN-LAST:event_jButtonCancelActionPerformed
private void jButtonOKActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButtonOKActionPerformed
if (this.jTextFieldName.getText().trim().length() <= 0 || Pattern.compile("[^\\w-]").matcher(this.jTextFieldName.getText().trim()).find()) {
javax.swing.JOptionPane.showMessageDialog(this,
I18n.getString(CustomAttributeDialog.class, "ModelerElement.AttributeDialog.Message.Warning"), I18n.getString(CustomAttributeDialog.class, "ModelerElement.AttributeDialog.Message.Warning2"),
javax.swing.JOptionPane.WARNING_MESSAGE);
return;
}
// check if the name is valid...
if (getProperties() != null) {
String name = jTextFieldName.getText().trim();
for (GenericProperty prop : getProperties()) {
if (prop.getKey().equals(name) && (originalName == null
|| !originalName.equals(name))) {
javax.swing.JOptionPane.showMessageDialog(this,
I18n.getString(CustomAttributeDialog.class, "ModelerElement.AttributeDialog.Message.warning3"), I18n.getString(CustomAttributeDialog.class, "ModelerElement.AttributeDialog.Warning4"),
javax.swing.JOptionPane.WARNING_MESSAGE);
return;
}
}
}
if (tmpField == null) {
tmpField = new GenericProperty();
}
tmpField.setKey(jTextFieldName.getText().trim());
tmpField.setValue(this.jTextAreaDescription.getText());
setVisible(false);
this.setDialogResult(javax.swing.JOptionPane.OK_OPTION);
dispose();
}//GEN-LAST:event_jButtonOKActionPerformed
/**
* Closes the dialog
*/
private void closeDialog(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_closeDialog
setVisible(false);
this.setDialogResult(javax.swing.JOptionPane.CLOSED_OPTION);
dispose();
}//GEN-LAST:event_closeDialog
/**
* Getter for property tmpParameter.
*
* @return Value of property tmpParameter.
*
*/
public GenericProperty getProperty() {
return tmpField;
}
/**
* Setter for property tmpParameter.
*
* @param tmpParameter New value of property tmpParameter.
*
*/
public void setProperty(GenericProperty tmpField) {
this.jTextFieldName.setText(tmpField.getKey());
this.jTextAreaDescription.setText("" + tmpField.getValue());
originalName = tmpField.getKey();
// updateExpressionPanel();
}
/**
* Getter for property dialogResult.
*
* @return Value of property dialogResult.
*
*/
public int getDialogResult() {
return dialogResult;
}
/**
* Setter for property dialogResult.
*
* @param dialogResult New value of property dialogResult.
*
*/
public void setDialogResult(int dialogResult) {
this.dialogResult = dialogResult;
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton jButtonCancel;
private javax.swing.JButton jButtonOK;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel4;
private javax.swing.JPanel jPanel1;
private javax.swing.JPanel jPanelExpression;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JTextArea jTextAreaDescription;
private javax.swing.JTextField jTextFieldName;
// End of variables declaration//GEN-END:variables
private int dialogResult;
public List getProperties() {
return properties;
}
public void setProperties(List properties) {
this.properties = properties;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy