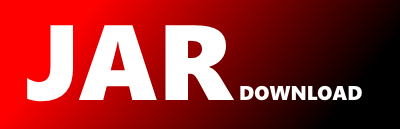
org.netbeans.modeler.properties.customattr.CustomAttributeEditor Maven / Gradle / Ivy
Go to download
Jeddict is an open source Jakarta EE application development platform that accelerates developers productivity and simplifies development tasks of creating complex entity relationship models.
/**
* Copyright 2013-2022 Gaurav Gupta
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package org.netbeans.modeler.properties.customattr;
import java.awt.event.MouseEvent;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.beans.PropertyEditor;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Vector;
import javax.swing.DefaultListSelectionModel;
import javax.swing.JPanel;
import javax.swing.table.DefaultTableModel;
import org.openide.explorer.propertysheet.PropertyEnv;
public class CustomAttributeEditor extends JPanel implements PropertyChangeListener {
private final PropertyEnv env;
private final PropertyEditor editor;
public CustomAttributeEditor(Object value, PropertyEditor editor, PropertyEnv env) {
this.env = env;
this.editor = editor;
this.env.setState(PropertyEnv.STATE_NEEDS_VALIDATION);
this.env.addPropertyChangeListener(this);
initComponents();
DefaultListSelectionModel dlsm = (DefaultListSelectionModel) this.attributeTable.getSelectionModel();
dlsm.addListSelectionListener(this::jTablePropertiesListSelectionValueChanged);
if (value != null && value instanceof Map) {
this.setPropertiesMap((Map) value);
} else if (value != null && value instanceof List) {
this.setPropertiesList((List) value);
}
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
jPanelFields = new javax.swing.JPanel();
contentPane = new javax.swing.JScrollPane();
attributeTable = new javax.swing.JTable();
actionPane = new javax.swing.JPanel();
actionLayeredPane = new javax.swing.JLayeredPane();
jButtonNewProperty = new javax.swing.JButton();
jButtonModifyProperty = new javax.swing.JButton();
jButtonDeleteProperty = new javax.swing.JButton();
jPanelFields.setLayout(new java.awt.BorderLayout());
contentPane.setPreferredSize(new java.awt.Dimension(32767, 32767));
attributeTable.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
},
new String [] {
"Name", "Value"
}
) {
Class[] types = new Class [] {
java.lang.Object.class, java.lang.String.class
};
boolean[] canEdit = new boolean [] {
false, false
};
public Class getColumnClass(int columnIndex) {
return types [columnIndex];
}
public boolean isCellEditable(int rowIndex, int columnIndex) {
return canEdit [columnIndex];
}
});
attributeTable.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
attributeTableMouseClicked(evt);
}
});
contentPane.setViewportView(attributeTable);
jPanelFields.add(contentPane, java.awt.BorderLayout.CENTER);
actionPane.setMinimumSize(new java.awt.Dimension(100, 10));
actionPane.setPreferredSize(new java.awt.Dimension(100, 32));
actionLayeredPane.setBorder(javax.swing.BorderFactory.createBevelBorder(javax.swing.border.BevelBorder.RAISED));
jButtonNewProperty.setIcon(new javax.swing.ImageIcon(getClass().getResource("/org/netbeans/modeler/properties/resource/icon_plus.png"))); // NOI18N
org.openide.awt.Mnemonics.setLocalizedText(jButtonNewProperty, org.openide.util.NbBundle.getMessage(CustomAttributeEditor.class, "CustomAttributeEditor.jButtonNewProperty.text")); // NOI18N
jButtonNewProperty.setToolTipText(org.openide.util.NbBundle.getMessage(CustomAttributeEditor.class, "CustomAttributeEditor.jButtonNewProperty.toolTipText")); // NOI18N
jButtonNewProperty.setActionCommand(org.openide.util.NbBundle.getMessage(CustomAttributeEditor.class, "CustomAttributeEditor.jButtonNewProperty.actionCommand")); // NOI18N
jButtonNewProperty.setMargin(new java.awt.Insets(2, 5, 2, 5));
jButtonNewProperty.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButtonNewPropertyActionPerformed(evt);
}
});
jButtonModifyProperty.setIcon(new javax.swing.ImageIcon(getClass().getResource("/org/netbeans/modeler/properties/resource/edit.png"))); // NOI18N
org.openide.awt.Mnemonics.setLocalizedText(jButtonModifyProperty, org.openide.util.NbBundle.getMessage(CustomAttributeEditor.class, "CustomAttributeEditor.jButtonModifyProperty.text")); // NOI18N
jButtonModifyProperty.setToolTipText(org.openide.util.NbBundle.getMessage(CustomAttributeEditor.class, "CustomAttributeEditor.jButtonModifyProperty.toolTipText")); // NOI18N
jButtonModifyProperty.setActionCommand(org.openide.util.NbBundle.getMessage(CustomAttributeEditor.class, "CustomAttributeEditor.jButtonModifyProperty.actionCommand")); // NOI18N
jButtonModifyProperty.setEnabled(false);
jButtonModifyProperty.setMargin(new java.awt.Insets(2, 5, 2, 5));
jButtonModifyProperty.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButtonModifyPropertyActionPerformed(evt);
}
});
jButtonDeleteProperty.setIcon(new javax.swing.ImageIcon(getClass().getResource("/org/netbeans/modeler/properties/resource/delete.png"))); // NOI18N
org.openide.awt.Mnemonics.setLocalizedText(jButtonDeleteProperty, org.openide.util.NbBundle.getMessage(CustomAttributeEditor.class, "CustomAttributeEditor.jButtonDeleteProperty.text")); // NOI18N
jButtonDeleteProperty.setToolTipText(org.openide.util.NbBundle.getMessage(CustomAttributeEditor.class, "CustomAttributeEditor.jButtonDeleteProperty.toolTipText")); // NOI18N
jButtonDeleteProperty.setActionCommand(org.openide.util.NbBundle.getMessage(CustomAttributeEditor.class, "CustomAttributeEditor.jButtonDeleteProperty.actionCommand")); // NOI18N
jButtonDeleteProperty.setEnabled(false);
jButtonDeleteProperty.setMargin(new java.awt.Insets(2, 5, 2, 5));
jButtonDeleteProperty.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButtonDeletePropertyActionPerformed(evt);
}
});
actionLayeredPane.setLayer(jButtonNewProperty, javax.swing.JLayeredPane.DEFAULT_LAYER);
actionLayeredPane.setLayer(jButtonModifyProperty, javax.swing.JLayeredPane.DEFAULT_LAYER);
actionLayeredPane.setLayer(jButtonDeleteProperty, javax.swing.JLayeredPane.DEFAULT_LAYER);
javax.swing.GroupLayout actionLayeredPaneLayout = new javax.swing.GroupLayout(actionLayeredPane);
actionLayeredPane.setLayout(actionLayeredPaneLayout);
actionLayeredPaneLayout.setHorizontalGroup(
actionLayeredPaneLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(actionLayeredPaneLayout.createSequentialGroup()
.addComponent(jButtonNewProperty, javax.swing.GroupLayout.PREFERRED_SIZE, 123, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jButtonModifyProperty, javax.swing.GroupLayout.PREFERRED_SIZE, 123, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jButtonDeleteProperty, javax.swing.GroupLayout.PREFERRED_SIZE, 123, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap())
);
actionLayeredPaneLayout.setVerticalGroup(
actionLayeredPaneLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(actionLayeredPaneLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jButtonNewProperty)
.addComponent(jButtonModifyProperty)
.addComponent(jButtonDeleteProperty))
);
javax.swing.GroupLayout actionPaneLayout = new javax.swing.GroupLayout(actionPane);
actionPane.setLayout(actionPaneLayout);
actionPaneLayout.setHorizontalGroup(
actionPaneLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(actionPaneLayout.createSequentialGroup()
.addComponent(actionLayeredPane, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
actionPaneLayout.setVerticalGroup(
actionPaneLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(actionPaneLayout.createSequentialGroup()
.addComponent(actionLayeredPane, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 0, Short.MAX_VALUE))
);
jPanelFields.add(actionPane, java.awt.BorderLayout.NORTH);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(4, 4, 4)
.addComponent(jPanelFields, javax.swing.GroupLayout.DEFAULT_SIZE, 392, Short.MAX_VALUE)
.addGap(4, 4, 4))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(4, 4, 4)
.addComponent(jPanelFields, javax.swing.GroupLayout.DEFAULT_SIZE, 292, Short.MAX_VALUE)
.addGap(4, 4, 4))
);
}// //GEN-END:initComponents
private void attributeTableMouseClicked(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_attributeTableMouseClicked
if (evt.getClickCount() == 2 && evt.getButton() == MouseEvent.BUTTON1 && attributeTable.getSelectedRow() >= 0) {
jButtonModifyPropertyActionPerformed(new java.awt.event.ActionEvent(jButtonModifyProperty, 0, ""));
}
}//GEN-LAST:event_attributeTableMouseClicked
private void jButtonNewPropertyActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButtonNewPropertyActionPerformed
CustomAttributeDialog jrpd = new CustomAttributeDialog(Misc.getMainFrame(), true);
jrpd.setProperties(getPropertiesList());
jrpd.setVisible(true);
DefaultTableModel dtm = (DefaultTableModel) attributeTable.getModel();
if (jrpd.getDialogResult() == javax.swing.JOptionPane.OK_OPTION) {
GenericProperty prop = jrpd.getProperty();
String val = (String) prop.getValue();
dtm.addRow(new Object[]{prop, val});
attributeTable.updateUI();
}
}//GEN-LAST:event_jButtonNewPropertyActionPerformed
private void jButtonModifyPropertyActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButtonModifyPropertyActionPerformed
int index = attributeTable.getSelectedRow();
DefaultTableModel dtm = (DefaultTableModel) attributeTable.getModel();
CustomAttributeDialog attrDialog = new CustomAttributeDialog(Misc.getMainFrame(), true);
attrDialog.setProperty((GenericProperty) dtm.getValueAt(index, 0));
attrDialog.setProperties(getPropertiesList());
attrDialog.setVisible(true);
if (attrDialog.getDialogResult() == javax.swing.JOptionPane.OK_OPTION) {
GenericProperty prop = attrDialog.getProperty();
String val = (String) prop.getValue();
dtm.setValueAt(prop, index, 0);
dtm.setValueAt(val, index, 1);
attributeTable.updateUI();
}
}//GEN-LAST:event_jButtonModifyPropertyActionPerformed
private void jButtonDeletePropertyActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButtonDeletePropertyActionPerformed
int[] rows = attributeTable.getSelectedRows();
DefaultTableModel dtm = (DefaultTableModel) attributeTable.getModel();
for (int i = rows.length - 1; i >= 0; --i) {
dtm.removeRow(rows[i]);
}
}//GEN-LAST:event_jButtonDeletePropertyActionPerformed
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JLayeredPane actionLayeredPane;
private javax.swing.JPanel actionPane;
private javax.swing.JTable attributeTable;
private javax.swing.JScrollPane contentPane;
private javax.swing.JButton jButtonDeleteProperty;
private javax.swing.JButton jButtonModifyProperty;
private javax.swing.JButton jButtonNewProperty;
private javax.swing.JPanel jPanelFields;
// End of variables declaration//GEN-END:variables
public void setPropertiesMap(Map properties) {
List list = new ArrayList<>();
Iterator> properties_Itr = properties.entrySet().iterator();
while (properties_Itr.hasNext()) {
Map.Entry entry = properties_Itr.next();
list.add(new GenericProperty(entry.getKey(), entry.getValue()));
}
setPropertiesList(list);
}
public Map getPropertiesMap() {
Map properties = new LinkedHashMap();
for (GenericProperty prop : getPropertiesList()) {
properties.put(prop.getKey(), (String) prop.getValue());
}
return properties;
}
public void setPropertiesList(List properties) {
DefaultTableModel dtm = (DefaultTableModel) attributeTable.getModel();
dtm.setRowCount(0);
for (GenericProperty prop : properties) {
String val = (String) prop.getValue();
Vector row = new Vector();
row.addElement(prop);
row.addElement(val);
dtm.addRow(row);
}
}
public List getPropertiesList() {
List props = new ArrayList();
DefaultTableModel dtm = (DefaultTableModel) attributeTable.getModel();
for (int i = 0; i < dtm.getRowCount(); ++i) {
props.add((GenericProperty) dtm.getValueAt(i, 0));
}
return props;
}
@Override
public void propertyChange(PropertyChangeEvent evt) {
if (PropertyEnv.PROP_STATE.equals(evt.getPropertyName()) && evt.getNewValue() == PropertyEnv.STATE_VALID) {
editor.setValue(getPropertiesMap());
}
}
public void jTablePropertiesListSelectionValueChanged(javax.swing.event.ListSelectionEvent e) {
if (this.attributeTable.getSelectedRowCount() <= 0) {
this.jButtonModifyProperty.setEnabled(false);
this.jButtonDeleteProperty.setEnabled(false);
} else {
this.jButtonModifyProperty.setEnabled(true);
this.jButtonDeleteProperty.setEnabled(true);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy