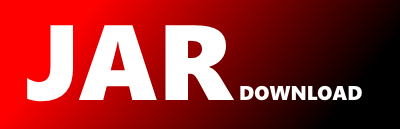
kafka.entity.changelog.topic.TopicMetadataSource Maven / Gradle / Ivy
package kafka.entity.changelog.topic;
import java.util.Collections;
import java.util.Set;
import java.util.concurrent.ExecutionException;
import kafka.entity.changelog.schema.Topic;
import org.apache.kafka.clients.admin.AdminClient;
import org.apache.kafka.clients.admin.NewTopic;
import org.apache.kafka.clients.admin.TopicDescription;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public interface TopicMetadataSource {
static TopicMetadataSource create(AdminClient adminClient) {
return new DefaultTopicMetadataSource(adminClient);
}
Topic.TopicsFound findTopics();
Topic.TopicCreated getTopicCreated(String name);
void createTopic(Topic.CreateTopic createTopic)
throws ExecutionException, InterruptedException;
class DefaultTopicMetadataSource implements TopicMetadataSource {
static final Logger LOG = LoggerFactory.getLogger(TopicMetadataSource.class);
final AdminClient adminClient;
DefaultTopicMetadataSource(AdminClient adminClient) {
this.adminClient = adminClient;
}
@Override public Topic.TopicsFound findTopics() {
try {
Set names = adminClient.listTopics().names().get();
return Topic.TopicsFound.newBuilder().addAllName(names).build();
} catch (InterruptedException | ExecutionException e) {
LOG.error("Error listing topic names", e);
return null;
}
}
@Override public Topic.TopicCreated getTopicCreated(String name) {
try {
TopicDescription description =
adminClient.describeTopics(Collections.singletonList(name))
.values()
.get(name)
.get();
return Topic.TopicCreated.newBuilder()
.setName(name)
.setPartitions(description.partitions().size())
.setReplicationFactor(description.partitions().get(0).replicas().size())
.build();
} catch (InterruptedException | ExecutionException e) {
LOG.error("Error describing topic {}", name, e);
return null;
}
}
@Override public void createTopic(Topic.CreateTopic createTopic)
throws ExecutionException, InterruptedException {
NewTopic newTopic = new NewTopic(createTopic.getName(), createTopic.getPartitions(),
(short) createTopic.getReplicationFactor());
adminClient.createTopics(Collections.singletonList(newTopic)).all().get();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy