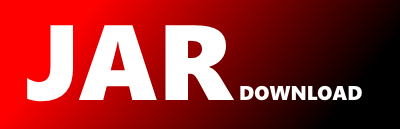
io.github.jfermat.strava.model.SummarySegment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of strava-api-client-resttemplate Show documentation
Show all versions of strava-api-client-resttemplate Show documentation
Strava's API client with RestTemplate's library.
/*
* Strava API v3
* The [Swagger Playground](https://developers.strava.com/playground) is the easiest way to familiarize yourself with the Strava API by submitting HTTP requests and observing the responses before you write any client code. It will show what a response will look like with different endpoints depending on the authorization scope you receive from your athletes. To use the Playground, go to https://www.strava.com/settings/api and change your “Authorization Callback Domain” to developers.strava.com. Please note, we only support Swagger 2.0. There is a known issue where you can only select one scope at a time. For more information, please check the section “client code” at https://developers.strava.com/docs.
*
* OpenAPI spec version: 3.0.0
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.github.jfermat.strava.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.github.jfermat.strava.model.LatLng;
import io.github.jfermat.strava.model.SummaryPRSegmentEffort;
import io.github.jfermat.strava.model.SummarySegmentEffort;
import io.swagger.v3.oas.annotations.media.Schema;
/**
* SummarySegment
*/
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.JavaClientCodegen", date = "2021-01-10T09:15:14.081+01:00[Europe/Paris]")
public class SummarySegment {
@JsonProperty("id")
private Long id = null;
@JsonProperty("name")
private String name = null;
/**
* Gets or Sets activityType
*/
public enum ActivityTypeEnum {
RIDE("Ride"),
RUN("Run");
private String value;
ActivityTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static ActivityTypeEnum fromValue(String text) {
for (ActivityTypeEnum b : ActivityTypeEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
} @JsonProperty("activity_type")
private ActivityTypeEnum activityType = null;
@JsonProperty("distance")
private Float distance = null;
@JsonProperty("average_grade")
private Float averageGrade = null;
@JsonProperty("maximum_grade")
private Float maximumGrade = null;
@JsonProperty("elevation_high")
private Float elevationHigh = null;
@JsonProperty("elevation_low")
private Float elevationLow = null;
@JsonProperty("start_latlng")
private LatLng startLatlng = null;
@JsonProperty("end_latlng")
private LatLng endLatlng = null;
@JsonProperty("climb_category")
private Integer climbCategory = null;
@JsonProperty("city")
private String city = null;
@JsonProperty("state")
private String state = null;
@JsonProperty("country")
private String country = null;
@JsonProperty("private")
private Boolean _private = null;
@JsonProperty("athlete_pr_effort")
private SummarySegmentEffort athletePrEffort = null;
@JsonProperty("athlete_segment_stats")
private SummaryPRSegmentEffort athleteSegmentStats = null;
public SummarySegment id(Long id) {
this.id = id;
return this;
}
/**
* The unique identifier of this segment
* @return id
**/
@Schema(description = "The unique identifier of this segment")
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public SummarySegment name(String name) {
this.name = name;
return this;
}
/**
* The name of this segment
* @return name
**/
@Schema(description = "The name of this segment")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public SummarySegment activityType(ActivityTypeEnum activityType) {
this.activityType = activityType;
return this;
}
/**
* Get activityType
* @return activityType
**/
@Schema(description = "")
public ActivityTypeEnum getActivityType() {
return activityType;
}
public void setActivityType(ActivityTypeEnum activityType) {
this.activityType = activityType;
}
public SummarySegment distance(Float distance) {
this.distance = distance;
return this;
}
/**
* The segment's distance, in meters
* @return distance
**/
@Schema(description = "The segment's distance, in meters")
public Float getDistance() {
return distance;
}
public void setDistance(Float distance) {
this.distance = distance;
}
public SummarySegment averageGrade(Float averageGrade) {
this.averageGrade = averageGrade;
return this;
}
/**
* The segment's average grade, in percents
* @return averageGrade
**/
@Schema(description = "The segment's average grade, in percents")
public Float getAverageGrade() {
return averageGrade;
}
public void setAverageGrade(Float averageGrade) {
this.averageGrade = averageGrade;
}
public SummarySegment maximumGrade(Float maximumGrade) {
this.maximumGrade = maximumGrade;
return this;
}
/**
* The segments's maximum grade, in percents
* @return maximumGrade
**/
@Schema(description = "The segments's maximum grade, in percents")
public Float getMaximumGrade() {
return maximumGrade;
}
public void setMaximumGrade(Float maximumGrade) {
this.maximumGrade = maximumGrade;
}
public SummarySegment elevationHigh(Float elevationHigh) {
this.elevationHigh = elevationHigh;
return this;
}
/**
* The segments's highest elevation, in meters
* @return elevationHigh
**/
@Schema(description = "The segments's highest elevation, in meters")
public Float getElevationHigh() {
return elevationHigh;
}
public void setElevationHigh(Float elevationHigh) {
this.elevationHigh = elevationHigh;
}
public SummarySegment elevationLow(Float elevationLow) {
this.elevationLow = elevationLow;
return this;
}
/**
* The segments's lowest elevation, in meters
* @return elevationLow
**/
@Schema(description = "The segments's lowest elevation, in meters")
public Float getElevationLow() {
return elevationLow;
}
public void setElevationLow(Float elevationLow) {
this.elevationLow = elevationLow;
}
public SummarySegment startLatlng(LatLng startLatlng) {
this.startLatlng = startLatlng;
return this;
}
/**
* Get startLatlng
* @return startLatlng
**/
@Schema(description = "")
public LatLng getStartLatlng() {
return startLatlng;
}
public void setStartLatlng(LatLng startLatlng) {
this.startLatlng = startLatlng;
}
public SummarySegment endLatlng(LatLng endLatlng) {
this.endLatlng = endLatlng;
return this;
}
/**
* Get endLatlng
* @return endLatlng
**/
@Schema(description = "")
public LatLng getEndLatlng() {
return endLatlng;
}
public void setEndLatlng(LatLng endLatlng) {
this.endLatlng = endLatlng;
}
public SummarySegment climbCategory(Integer climbCategory) {
this.climbCategory = climbCategory;
return this;
}
/**
* The category of the climb [0, 5]. Higher is harder ie. 5 is Hors catégorie, 0 is uncategorized in climb_category.
* @return climbCategory
**/
@Schema(description = "The category of the climb [0, 5]. Higher is harder ie. 5 is Hors catégorie, 0 is uncategorized in climb_category.")
public Integer getClimbCategory() {
return climbCategory;
}
public void setClimbCategory(Integer climbCategory) {
this.climbCategory = climbCategory;
}
public SummarySegment city(String city) {
this.city = city;
return this;
}
/**
* The segments's city.
* @return city
**/
@Schema(description = "The segments's city.")
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public SummarySegment state(String state) {
this.state = state;
return this;
}
/**
* The segments's state or geographical region.
* @return state
**/
@Schema(description = "The segments's state or geographical region.")
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public SummarySegment country(String country) {
this.country = country;
return this;
}
/**
* The segment's country.
* @return country
**/
@Schema(description = "The segment's country.")
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
public SummarySegment _private(Boolean _private) {
this._private = _private;
return this;
}
/**
* Whether this segment is private.
* @return _private
**/
@Schema(description = "Whether this segment is private.")
public Boolean isPrivate() {
return _private;
}
public void setPrivate(Boolean _private) {
this._private = _private;
}
public SummarySegment athletePrEffort(SummarySegmentEffort athletePrEffort) {
this.athletePrEffort = athletePrEffort;
return this;
}
/**
* Get athletePrEffort
* @return athletePrEffort
**/
@Schema(description = "")
public SummarySegmentEffort getAthletePrEffort() {
return athletePrEffort;
}
public void setAthletePrEffort(SummarySegmentEffort athletePrEffort) {
this.athletePrEffort = athletePrEffort;
}
public SummarySegment athleteSegmentStats(SummaryPRSegmentEffort athleteSegmentStats) {
this.athleteSegmentStats = athleteSegmentStats;
return this;
}
/**
* Get athleteSegmentStats
* @return athleteSegmentStats
**/
@Schema(description = "")
public SummaryPRSegmentEffort getAthleteSegmentStats() {
return athleteSegmentStats;
}
public void setAthleteSegmentStats(SummaryPRSegmentEffort athleteSegmentStats) {
this.athleteSegmentStats = athleteSegmentStats;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SummarySegment summarySegment = (SummarySegment) o;
return Objects.equals(this.id, summarySegment.id) &&
Objects.equals(this.name, summarySegment.name) &&
Objects.equals(this.activityType, summarySegment.activityType) &&
Objects.equals(this.distance, summarySegment.distance) &&
Objects.equals(this.averageGrade, summarySegment.averageGrade) &&
Objects.equals(this.maximumGrade, summarySegment.maximumGrade) &&
Objects.equals(this.elevationHigh, summarySegment.elevationHigh) &&
Objects.equals(this.elevationLow, summarySegment.elevationLow) &&
Objects.equals(this.startLatlng, summarySegment.startLatlng) &&
Objects.equals(this.endLatlng, summarySegment.endLatlng) &&
Objects.equals(this.climbCategory, summarySegment.climbCategory) &&
Objects.equals(this.city, summarySegment.city) &&
Objects.equals(this.state, summarySegment.state) &&
Objects.equals(this.country, summarySegment.country) &&
Objects.equals(this._private, summarySegment._private) &&
Objects.equals(this.athletePrEffort, summarySegment.athletePrEffort) &&
Objects.equals(this.athleteSegmentStats, summarySegment.athleteSegmentStats);
}
@Override
public int hashCode() {
return Objects.hash(id, name, activityType, distance, averageGrade, maximumGrade, elevationHigh, elevationLow, startLatlng, endLatlng, climbCategory, city, state, country, _private, athletePrEffort, athleteSegmentStats);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SummarySegment {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" activityType: ").append(toIndentedString(activityType)).append("\n");
sb.append(" distance: ").append(toIndentedString(distance)).append("\n");
sb.append(" averageGrade: ").append(toIndentedString(averageGrade)).append("\n");
sb.append(" maximumGrade: ").append(toIndentedString(maximumGrade)).append("\n");
sb.append(" elevationHigh: ").append(toIndentedString(elevationHigh)).append("\n");
sb.append(" elevationLow: ").append(toIndentedString(elevationLow)).append("\n");
sb.append(" startLatlng: ").append(toIndentedString(startLatlng)).append("\n");
sb.append(" endLatlng: ").append(toIndentedString(endLatlng)).append("\n");
sb.append(" climbCategory: ").append(toIndentedString(climbCategory)).append("\n");
sb.append(" city: ").append(toIndentedString(city)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" country: ").append(toIndentedString(country)).append("\n");
sb.append(" _private: ").append(toIndentedString(_private)).append("\n");
sb.append(" athletePrEffort: ").append(toIndentedString(athletePrEffort)).append("\n");
sb.append(" athleteSegmentStats: ").append(toIndentedString(athleteSegmentStats)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy