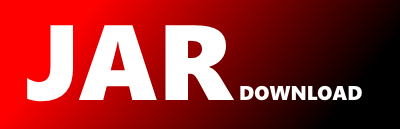
org.fluentjdbc.DatabaseSaveBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-jdbc Show documentation
Show all versions of fluent-jdbc Show documentation
A Java library used to execute JDBC statements and build SQL
package org.fluentjdbc;
import org.fluentjdbc.DatabaseTable.RowMapper;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
@ParametersAreNonnullByDefault
public abstract class DatabaseSaveBuilder extends DatabaseStatement {
protected List uniqueKeyFields = new ArrayList<>();
protected List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy