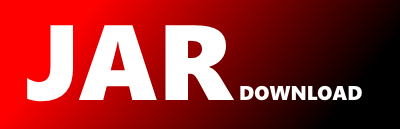
org.fluentjdbc.DatabaseStatement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-jdbc Show documentation
Show all versions of fluent-jdbc Show documentation
A Java library used to execute JDBC statements and build SQL
package org.fluentjdbc;
import org.fluentjdbc.util.ExceptionUtil;
import org.joda.time.DateTime;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.sql.Timestamp;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.UUID;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
@ParametersAreNonnullByDefault
class DatabaseStatement {
protected static Logger logger = LoggerFactory.getLogger(DatabaseStatement.class);
protected int bindParameters(PreparedStatement stmt, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy