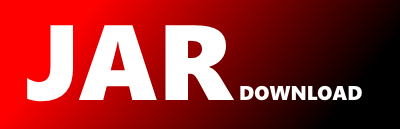
org.fluentjdbc.DatabaseTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-jdbc Show documentation
Show all versions of fluent-jdbc Show documentation
A Java library used to execute JDBC statements and build SQL
package org.fluentjdbc;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
import java.util.UUID;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
@ParametersAreNonnullByDefault
public interface DatabaseTable {
public interface RowMapper {
T mapRow(DatabaseRow row) throws SQLException;
}
String getTableName();
DatabaseSaveBuilder newSaveBuilder(String idColumn, @Nullable Long idValue);
/**
* Use instead of {@link #newSaveBuilder} if the database driver does not
* support RETURN_GENERATED_KEYS
*/
DatabaseSaveBuilder newSaveBuilderNoGeneratedKeys(String idColumn, @Nullable Long idValue);
DatabaseSaveBuilderWithUUID newSaveBuilderWithUUID(String string, @Nullable UUID uuid);
List listObjects(Connection connection, RowMapper mapper);
DatabaseSimpleQueryBuilder where(String fieldName, @Nullable Object value);
DatabaseSimpleQueryBuilder whereOptional(String fieldName, @Nullable Object value);
DatabaseSimpleQueryBuilder whereIn(String fieldName, List> parameters);
DatabaseSimpleQueryBuilder whereExpression(String expression, Object parameter);
DatabaseSimpleQueryBuilder whereAll(List uniqueKeyFields, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy