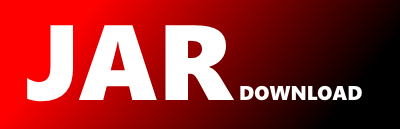
org.fluentjdbc.DatabaseBulkInsertBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-jdbc Show documentation
Show all versions of fluent-jdbc Show documentation
A Java library used to execute JDBC statements and build SQL
package org.fluentjdbc;
import org.fluentjdbc.util.ExceptionUtil;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import java.util.function.BiConsumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
import java.util.stream.Stream;
public class DatabaseBulkInsertBuilder extends DatabaseStatement implements DatabaseBulkUpdateable> {
private DatabaseTable table;
private Iterable objects;
private final List updateFields = new ArrayList<>();
private final List> updateParameters = new ArrayList<>();
DatabaseBulkInsertBuilder(DatabaseTable table, Iterable objects) {
this.table = table;
this.objects = objects;
}
DatabaseBulkInsertBuilder(DatabaseTable table, Stream objects) {
this(table, objects.collect(Collectors.toList()));
}
@Override
public DatabaseBulkInsertBuilder setField(String fieldName, Function transformer) {
updateFields.add(fieldName);
updateParameters.add(transformer);
return this;
}
public int execute(Connection connection) {
String insertStatement = createInsertSql(table.getTableName(), updateFields);
try (PreparedStatement statement = connection.prepareStatement(insertStatement)) {
addBatch(statement, objects, updateParameters);
int[] counts = statement.executeBatch();
return IntStream.of(counts).sum();
} catch (SQLException e) {
throw ExceptionUtil.softenCheckedException(e);
}
}
public DatabaseBulkInsertBuilderWithPk generatePrimaryKeys(BiConsumer consumer) {
return new DatabaseBulkInsertBuilderWithPk<>(objects, table, updateFields, updateParameters, consumer);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy