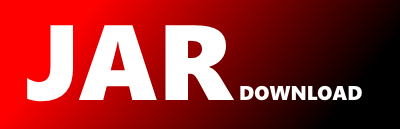
org.fluentjdbc.DatabaseInsertBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-jdbc Show documentation
Show all versions of fluent-jdbc Show documentation
A Java library used to execute JDBC statements and build SQL
package org.fluentjdbc;
import java.sql.Connection;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
@ParametersAreNonnullByDefault
public class DatabaseInsertBuilder extends DatabaseStatement implements DatabaseUpdateable {
private List fieldNames = new ArrayList<>();
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy