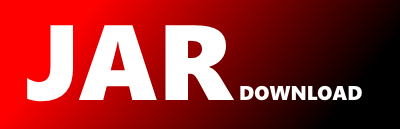
org.fluentjdbc.DbSyncBuilderContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-jdbc Show documentation
Show all versions of fluent-jdbc Show documentation
A Java library used to execute JDBC statements and build SQL
package org.fluentjdbc;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.EnumMap;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class DbSyncBuilderContext {
private final DbTableContext table;
private EnumMap status = new EnumMap<>(DatabaseSaveResult.SaveStatus.class);
private final List theirObjects;
private boolean isCached = false;
private Map, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy