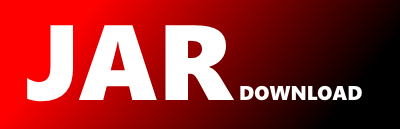
org.fluentjdbc.DbTableContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-jdbc Show documentation
Show all versions of fluent-jdbc Show documentation
A Java library used to execute JDBC statements and build SQL
package org.fluentjdbc;
import javax.annotation.Nullable;
import java.sql.Connection;
import java.util.Collection;
import java.util.List;
import java.util.Optional;
import java.util.UUID;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class DbTableContext implements DatabaseQueriable {
private DatabaseTable table;
private DbContext dbContext;
public DbTableContext(DatabaseTable databaseTable, DbContext dbContext) {
this.table = databaseTable;
this.dbContext = dbContext;
}
public DbInsertContext insert() {
return new DbInsertContext(this);
}
public DatabaseTable getTable() {
return table;
}
public DbSelectContext whereIn(String fieldName, Collection> parameters) {
return new DbSelectContext(this).whereIn(fieldName, parameters);
}
public DbSelectContext whereOptional(String fieldName, Object value) {
return new DbSelectContext(this).whereOptional(fieldName, value);
}
public DbSelectContext whereExpression(String expression) {
return new DbSelectContext(this).whereExpression(expression);
}
public DbSelectContext whereExpression(String expression, @Nullable Object value) {
return new DbSelectContext(this).whereExpression(expression, value);
}
public DbSelectContext whereExpressionWithMultipleParameters(String expression, Collection> parameters){
return new DbSelectContext(this).whereExpressionWithMultipleParameters(expression, parameters);
}
@Override
public DbSelectContext whereAll(List fields, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy