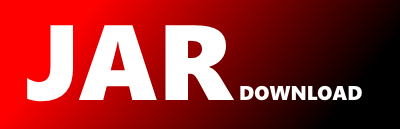
org.fluentjdbc.DatabaseInsertWithPkBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-jdbc Show documentation
Show all versions of fluent-jdbc Show documentation
A Java library used to execute JDBC statements and build SQL
package org.fluentjdbc;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nonnull;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.List;
import static org.fluentjdbc.DatabaseStatement.bindParameters;
/**
* Generate INSERT
statements with automatic primary key generation by collecting
* field names and parameters. Example:
*
*
* Long id = table.insert()
* .setPrimaryKey("id", (Long)null) // this creates {@link DatabaseInsertWithPkBuilder}
* .setField("name", "Something")
* .setField("code", 102)
* .execute(connection);
*
*/
public class DatabaseInsertWithPkBuilder {
private static final Logger logger = LoggerFactory.getLogger(DatabaseInsertWithPkBuilder.class);
private final DatabaseInsertBuilder insertBuilder;
private final String idField;
private final T idValue;
public DatabaseInsertWithPkBuilder(DatabaseInsertBuilder insertBuilder, String idField, T idValue) {
this.insertBuilder = insertBuilder;
this.idField = idField;
this.idValue = idValue;
}
/**
* Adds fieldName to the INSERT (fieldName) VALUES (?)
and parameter to the list of parameters
*/
@CheckReturnValue
public DatabaseInsertWithPkBuilder setField(String fieldName, Object parameter) {
//noinspection ResultOfMethodCallIgnored
insertBuilder.setField(fieldName, parameter);
return this;
}
/**
* Calls {@link #setField(String, Object)} for each fieldName and parameter
*/
@CheckReturnValue
public DatabaseInsertWithPkBuilder setFields(List fields, List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy