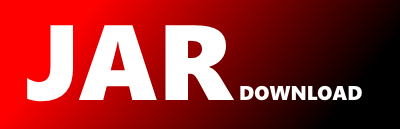
org.fluentjdbc.DatabaseQueryBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-jdbc Show documentation
Show all versions of fluent-jdbc Show documentation
A Java library used to execute JDBC statements and build SQL
package org.fluentjdbc;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nonnull;
import java.sql.Connection;
import java.time.Instant;
import java.util.Optional;
/**
* Interface for consistent query operations in a fluent way
*/
public interface DatabaseQueryBuilder> extends DatabaseQueryable {
/**
* If you haven't called {@link #orderBy}, the results of {@link DatabaseListableQueryBuilder#list}
* will be unpredictable. Call unordered()
if you are okay with this.
*/
@CheckReturnValue
T unordered();
/**
* Adds an order by
clause to the query. Needed in order to list results
* in a predictable order.
*/
@CheckReturnValue
T orderBy(String orderByClause);
/**
* If the query returns no rows, returns {@link Optional#empty()}, if exactly one row is returned, maps it and return it,
* if more than one is returned, throws `IllegalStateException`
*
* @param connection Database connection
* @param mapper Function object to map a single returned row to a object
* @return the mapped row if one row is returned, Optional.empty otherwise
* @throws IllegalStateException if more than one row was matched the the query
*/
@Nonnull
@CheckReturnValue
© 2015 - 2024 Weber Informatics LLC | Privacy Policy