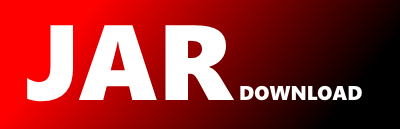
org.fluentjdbc.DatabaseWhereBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-jdbc Show documentation
Show all versions of fluent-jdbc Show documentation
A Java library used to execute JDBC statements and build SQL
package org.fluentjdbc;
import javax.annotation.CheckReturnValue;
import javax.annotation.ParametersAreNonnullByDefault;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
/**
* Used to build the WHERE ...
clause of SQL statements such as SELECT, UPDATE and DELETE.
* Add clauses with {@link #where(String, Object)}, {@link #whereIn(String, Collection)} etc. Then
* get the string for the WHERE clause with {@link #whereClause()} and the parameters with {@link #getParameters()}
*/
@ParametersAreNonnullByDefault
public class DatabaseWhereBuilder implements DatabaseQueryable {
private final List conditions = new ArrayList<>();
private final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy