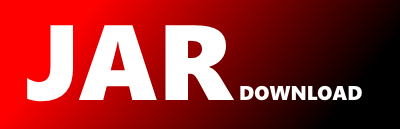
org.fluentjdbc.DbContextInsertBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-jdbc Show documentation
Show all versions of fluent-jdbc Show documentation
A Java library used to execute JDBC statements and build SQL
package org.fluentjdbc;
import org.fluentjdbc.util.ExceptionUtil;
import javax.annotation.CheckReturnValue;
import java.sql.SQLException;
import java.util.Collection;
/**
* Generate INSERT
statements by collecting field names and parameters. Support
* autogeneration of primary keys. Example:
*
*
* {@link DbContextTable} table = context.table("database_test_table");
* try (DbContextConnection ignored = context.startConnection(dataSource)) {
* Long id = table.insert()
* .setPrimaryKey("id", (Long)null)
* .setField("name", "Something")
* .setField("code", 102)
* .execute();
* }
*
*
* @see DatabaseInsertBuilder
*/
public class DbContextInsertBuilder implements DatabaseUpdatable {
public class DbContextInsertBuilderWithPk {
private final DatabaseInsertWithPkBuilder builder;
public DbContextInsertBuilderWithPk(DatabaseInsertWithPkBuilder builder) {
this.builder = builder;
}
@CheckReturnValue
public DbContextInsertBuilderWithPk setField(String fieldName, Object parameter) {
//noinspection ResultOfMethodCallIgnored
builder.setField(fieldName, parameter);
return this;
}
public T execute() {
return builder.execute(dbContextTable.getConnection());
}
}
private final DatabaseInsertBuilder builder;
private final DbContextTable dbContextTable;
public DbContextInsertBuilder(DbContextTable dbContextTable) {
this.dbContextTable = dbContextTable;
builder = dbContextTable.getTable().insert();
}
/**
* Adds primary key to the INSERT
statement if idValue is not null. If idValue is null
* this will {@link java.sql.PreparedStatement#execute(String, String[])} to generate the primary
* key using the underlying table autogeneration mechanism
*/
@CheckReturnValue
public DbContextInsertBuilderWithPk setPrimaryKey(String idField, T idValue) {
DatabaseInsertWithPkBuilder setPrimaryKey = builder.setPrimaryKey(idField, idValue);
return new DbContextInsertBuilderWithPk<>(setPrimaryKey);
}
/**
* Adds fieldName to the INSERT (fieldName) VALUES (?)
and parameter to the list of parameters
*/
@Override
public DbContextInsertBuilder setField(String fieldName, Object parameter) {
//noinspection ResultOfMethodCallIgnored
builder.setField(fieldName, parameter);
return this;
}
/**
* Calls {@link #setField(String, Object)} for each fieldName and parameter
*/
@Override
public DbContextInsertBuilder setFields(Collection fields, Collection> values) {
//noinspection ResultOfMethodCallIgnored
builder.setFields(fields, values);
return this;
}
/**
* Executes the insert statement and returns the number of rows inserted
*/
public int execute() {
return builder.execute(dbContextTable.getConnection());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy