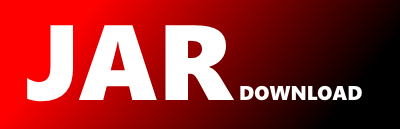
org.fluentjdbc.DbContextStatement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-jdbc Show documentation
Show all versions of fluent-jdbc Show documentation
A Java library used to execute JDBC statements and build SQL
package org.fluentjdbc;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nonnull;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* Used to execute an arbitrary statement. Create with {@link DbContext#statement(String, List)}.
*
* Example
*
*
* dbContext.statement(
* "update orders set quantity = quantity + 1 WHERE customer_id = ?",
* Arrays.asList(customerId)
* ).executeUpdate();
*
*/
public class DbContextStatement {
private final DbContext dbContext;
private final DatabaseStatement statement;
public DbContextStatement(DbContext dbContext, String statement, List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy