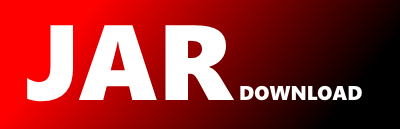
org.fluentjdbc.DbContextTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-jdbc Show documentation
Show all versions of fluent-jdbc Show documentation
A Java library used to execute JDBC statements and build SQL
package org.fluentjdbc;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nonnull;
import javax.sql.DataSource;
import java.sql.Connection;
import java.util.List;
import java.util.Optional;
import java.util.UUID;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* Provides a thread context oriented way of manipulating a table. Create from {@link DbContext}.
* All database operations must be nested in {@link DbContext#startConnection(DataSource)}.
*
*Usage examples
*
* {@link DbContextTable} table = context.table("database_test_table");
* try (DbContextConnection ignored = context.startConnection(dataSource)) {
* table.{@link #insert()}.setField("code", 102).execute();
* table.{@link #query()}.where("key", key).orderBy("value").listStrings("value");
* table.where("id", id).update().setField("value", newValue).execute();
* table.where("id", id).delete();
*
* table.newSaveBuilderWithUUID("id", o.getId()).setField("name", o.getName()).execute();
* }
*
*/
@CheckReturnValue
public class DbContextTable implements DatabaseQueryable {
private final DatabaseTable table;
private final DbContext dbContext;
public DbContextTable(DatabaseTable databaseTable, @Nonnull DbContext dbContext) {
this.table = databaseTable;
this.dbContext = dbContext;
}
/**
* Creates a {@link DbContextInsertBuilder} object to fluently generate a INSERT ...
statement. Example:
*
*
* table.insert().setField("id", id).setField("name", name).execute();
*
*
*/
public DbContextInsertBuilder insert() {
return new DbContextInsertBuilder(this);
}
/**
* Creates a {@link #query()} ready for list-operations without any ORDER BY
clause
*/
public DbContextSelectBuilder unordered() {
return query();
}
/**
* Creates a {@link #query()} ready for list-operations with ORDER BY ...
clause
*/
public DbContextSelectBuilder orderedBy(String orderByClause) {
return query().orderBy(orderByClause);
}
/**
* Creates a {@link DbContextSaveBuilder} which creates a INSERT
or UPDATE
* statement, depending on whether the row already exists in the database. If idValue is null,
* {@link DbContextSaveBuilder} will attempt to use the table's autogeneration of primary keys
* if there is no row with matching unique keys
*/
public DbContextSaveBuilder newSaveBuilder(String idColumn, Long idValue) {
return save(table.newSaveBuilder(idColumn, idValue));
}
/**
* Creates a {@link DbContextSaveBuilder} which creates a INSERT
or UPDATE
* statement, depending on whether the row already exists in the database. Throws exception if idValue is null
*/
public DbContextSaveBuilder newSaveBuilderWithString(String idColumn, String idValue) {
return save(table.newSaveBuilderWithString(idColumn, idValue));
}
/**
* Creates a {@link DbContextSaveBuilder} which creates a INSERT
or UPDATE
* statement, depending on whether the row already exists in the database.
* Generates UUID.randomUUID if idValue is null and row with matching unique keys does not already exist
*/
public DbContextSaveBuilder newSaveBuilderWithUUID(String field, UUID uuid) {
return save(table.newSaveBuilderWithUUID(field, uuid));
}
/**
* Associates the argument {@link DatabaseSaveResult} with this {@link DbContextTable}'s {@link DbContext}
*/
public DbContextSaveBuilder save(DatabaseSaveBuilder saveBuilder) {
return new DbContextSaveBuilder<>(this, saveBuilder);
}
/**
* Retrieves the underlying or cached value of the retriever argument. This cache is per
* {@link DbContextConnection} and is evicted when the connection is closed. The key is in context
* of this table, so different tables can have the same key without collision
*/
public Optional cache(KEY key, DbContext.RetrieveMethod retriever) {
return dbContext.cache(getTable().getTableName(), key, retriever);
}
/**
* Create a {@link DbContextTableAlias} associated with this {@link DbContextTable} which can
* be used to JOIN
statements with {@link DbContextTableAlias#join(DatabaseColumnReference, DatabaseColumnReference)}.
*
* @see DbContextJoinedSelectBuilder
*/
public DbContextTableAlias alias(String alias) {
return new DbContextTableAlias(this, alias);
}
/**
* Creates a {@link DbContextSelectBuilder} which allows you to query the table by
* generating "SELECT ..."
statements
*/
public DbContextSelectBuilder query() {
return new DbContextSelectBuilder(this);
}
/**
* Creates a {@link DbContextSyncBuilder} which allows you to synchronize the data in the
* table with an external source
*/
public DbContextSyncBuilder sync(List entities) {
return new DbContextSyncBuilder<>(this, entities);
}
/**
* Creates a {@link DbContextBulkInsertBuilder} object to fluently generate a INSERT ...
statement
* for a list of objects. Example:
*
*
* public void saveAll(List<TagType> tagTypes) {
* tagTypesTable.bulkInsert(tagTypes)
* .setField("name", TagType::getName)
* .generatePrimaryKeys("id", TagType::setId)
* .execute();
* }
*
*/
public DbContextBulkInsertBuilder bulkInsert(@Nonnull Stream objects) {
return bulkInsert(objects.collect(Collectors.toList()));
}
/**
* Creates a {@link DbContextBulkInsertBuilder} object to fluently generate a INSERT ...
statement
* for a list of objects. Example:
*
*
* public void saveAll(List<TagType> tagTypes) {
* tagTypesTable.bulkInsert(tagTypes)
* .setField("name", TagType::getName)
* .generatePrimaryKeys("id", TagType::setId)
* .execute();
* }
*
*/
public DbContextBulkInsertBuilder bulkInsert(Iterable objects) {
return new DbContextBulkInsertBuilder<>(this, table.bulkInsert(objects));
}
/**
* Creates a {@link DbContextBulkDeleteBuilder} object to fluently generate a DELETE ...
statement
* for a list of objects. Example:
*
* Example:
*
*
* public void deleteAll(Stream<TagType> tagTypes) {
* tagTypesTable.bulkDelete(tagTypes)
* .where("id", TagType::getId)
* .execute();
* }
*
*/
public DbContextBulkDeleteBuilder bulkDelete(@Nonnull Stream objects) {
return bulkDelete(objects.collect(Collectors.toList()));
}
/**
* Creates a {@link DbContextBulkDeleteBuilder} object to fluently generate a DELETE ...
statement
* for a list of objects. Example:
*
* Example:
*
*
* public void deleteAll(List<TagType> tagTypes) {
* tagTypesTable.bulkDelete(tagTypes)
* .where("id", TagType::getId)
* .execute();
* }
*
*/
public DbContextBulkDeleteBuilder bulkDelete(Iterable objects) {
return new DbContextBulkDeleteBuilder<>(this, table.bulkDelete(objects));
}
/**
* Creates a {@link DbContextBulkUpdateBuilder} object to fluently generate a UPDATE ...
statement
* for a list of objects. Example:
*
*
* public void updateAll(Stream<TagType> tagTypes) {
* tagTypesTable.bulkUpdate(tagTypes)
* .where("id", TagType::getId)
* .setField("name", TagType::getName)
* .execute();
* }
*
*/
public DbContextBulkUpdateBuilder bulkUpdate(@Nonnull Stream objects) {
return bulkUpdate(objects.collect(Collectors.toList()));
}
/**
* Creates a {@link DbContextBulkUpdateBuilder} object to fluently generate a UPDATE ...
statement
* for a list of objects. Example:
*
*
* public void updateAll(List<TagType> tagTypes) {
* tagTypesTable.bulkUpdate(tagTypes)
* .where("id", TagType::getId)
* .setField("name", TagType::getName)
* .execute();
* }
*
*/
private DbContextBulkUpdateBuilder bulkUpdate(List objects) {
return new DbContextBulkUpdateBuilder<>(this, table.bulkUpdate(objects));
}
public DatabaseTable getTable() {
return table;
}
public DbContext getDbContext() {
return dbContext;
}
@Nonnull
public Connection getConnection() {
return dbContext.getThreadConnection();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy