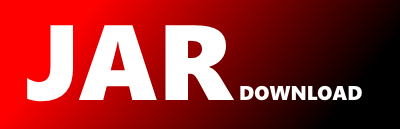
org.fluentjdbc.DbContextUpdateBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-jdbc Show documentation
Show all versions of fluent-jdbc Show documentation
A Java library used to execute JDBC statements and build SQL
package org.fluentjdbc;
import java.util.Collection;
/**
* Generate UPDATE
insert statements by collecting field names and parameters. Support
* autogeneration of primary keys. Example:
*
*
* {@link DbContextTable} table = context.table("database_test_table");
* try (DbContextConnection ignored = context.startConnection(dataSource)) {
* int count = table
* .where("id", id)
* .update()
* .setField("name", "Something")
* .setField("code", 102)
* .execute(connection);
* }
*
*/
public class DbContextUpdateBuilder implements DatabaseUpdatable {
private final DbContextTable table;
private final DatabaseUpdateBuilder updateBuilder;
public DbContextUpdateBuilder(DbContextTable table, DatabaseUpdateBuilder updateBuilder) {
this.table = table;
this.updateBuilder = updateBuilder;
}
/**
* Calls {@link #setField(String, Object)} for each fieldName and parameter
*/
@Override
public DbContextUpdateBuilder setFields(Collection fields, Collection> values) {
//noinspection ResultOfMethodCallIgnored
updateBuilder.setFields(fields, values);
return this;
}
/**
* Adds the fieldName to the SQL statement and the value to the parameter list
*/
@Override
public DbContextUpdateBuilder setField(String field, Object value) {
//noinspection ResultOfMethodCallIgnored
updateBuilder.setField(field, value);
return this;
}
/**
* Will execute the UPDATE statement to the database
*/
public int execute() {
return updateBuilder.execute(table.getConnection());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy