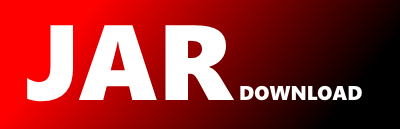
io.github.jiashunx.masker.rest.framework.MRestRequest Maven / Gradle / Ivy
package io.github.jiashunx.masker.rest.framework;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import io.github.jiashunx.masker.rest.framework.serialize.MRestSerializer;
import io.netty.handler.codec.http.HttpHeaders;
import io.netty.handler.codec.http.HttpMethod;
import io.netty.handler.codec.http.HttpRequest;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/**
* @author jiashunx
*/
public class MRestRequest {
private HttpRequest httpRequest;
private Map attributes = new HashMap<>();
private HttpMethod method;
private String url;
private String urlQuery;
private Map parameters;
private Map> originParameters;
private HttpHeaders headers;
private byte[] bodyBytes;
public MRestRequest() {}
public String bodyToString() {
return new String(bodyBytes, StandardCharsets.UTF_8);
}
public JSONObject bodyToJSONObj() {
return MRestSerializer.parseToJSONObject(bodyToString());
}
public JSONArray bodyToJSONArr() {
return MRestSerializer.parseToJSONArray(bodyToString());
}
public List parseBodyToObjList(Class klass) {
return JSON.parseArray(bodyToString(), klass);
}
public T parseBodyToObj(Class klass) {
return JSON.parseObject(bodyToString(), klass);
}
public HttpRequest getHttpRequest() {
return httpRequest;
}
public void setHttpRequest(HttpRequest httpRequest) {
this.httpRequest = httpRequest;
}
public Map getAttributes() {
return attributes;
}
public void setAttributes(Map attributes) {
this.attributes = attributes;
}
public void setAttribute(String key, String value) {
attributes.put(key, value);
}
public String getAttribute(String key) {
return attributes.get(key);
}
public HttpMethod getMethod() {
return method;
}
public void setMethod(HttpMethod method) {
this.method = method;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public String getUrlQuery() {
return urlQuery;
}
public void setUrlQuery(String urlQuery) {
this.urlQuery = urlQuery;
}
public Map getParameters() {
return parameters;
}
public String getParameter(String key) {
return getParameters().get(key);
}
public void setParameters(Map parameters) {
this.parameters = parameters;
}
public Map> getOriginParameters() {
return originParameters;
}
public void setOriginParameters(Map> originParameters) {
this.originParameters = originParameters;
}
public HttpHeaders getHeaders() {
return headers;
}
public Object getHeader(String key) {
return getHeaders().get(key);
}
public String getHeaderToStr(String key) {
Object value = getHeader(key);
return value == null ? null : value.toString();
}
public void setHeaders(HttpHeaders headers) {
this.headers = headers;
}
public byte[] getBodyBytes() {
return bodyBytes;
}
public void setBodyBytes(byte[] bodyBytes) {
this.bodyBytes = bodyBytes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy