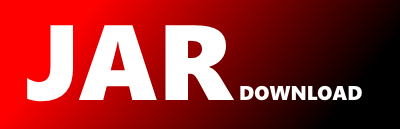
org.pixel.pipeline.PipelineContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pixel-pipeline Show documentation
Show all versions of pixel-pipeline Show documentation
Modular Java/Kotlin 2D Game Framework.
/*
* This software is available under Apache License
* Copyright (c)
*/
package org.pixel.pipeline;
import java.util.Iterator;
import java.util.concurrent.CompletableFuture;
import org.pixel.commons.logger.Logger;
import org.pixel.commons.logger.LoggerFactory;
public class PipelineContext {
private static final Logger log = LoggerFactory.getLogger(PipelineContext.class);
private final DataPipeline dataPipeline;
private final CompletableFuture future;
private Iterator> iterator;
/**
* Constructor.
*
* @param dataPipeline The data pipeline associated with this context.
*/
public PipelineContext(DataPipeline dataPipeline, CompletableFuture future) {
this.dataPipeline = dataPipeline;
this.future = future;
this.iterator = null;
}
/**
* Resolves current handler processing and pushes the output to the next handler (or the end of the pipeline).
*
* @param output The output of the current handler.
*/
public void next(T output) {
if (iterator == null) {
log.trace("Getting new handler iterator due to being null.");
iterator = dataPipeline.getHandlerIterator();
}
if (!iterator.hasNext()) {
// Pipeline has reached the end.
log.trace("Pipeline has reached the end, notifying listeners...");
iterator = null;
future.complete(output);
return;
}
iterator.next().process(this, output);
}
/**
* Resolves the current handler processing and terminates the pipeline processing with the given output.
*
* @param output The output of the pipeline.
*/
public void resolve(T output) {
iterator = null;
future.complete(output);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy