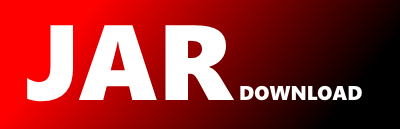
org.pixel.pipeline.ThreadPoolDataPipeline Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pixel-pipeline Show documentation
Show all versions of pixel-pipeline Show documentation
Modular Java/Kotlin 2D Game Framework.
/*
* This software is available under Apache License
* Copyright (c)
*/
package org.pixel.pipeline;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.SynchronousQueue;
import java.util.concurrent.ThreadFactory;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
import org.pixel.commons.lifecycle.Disposable;
public class ThreadPoolDataPipeline extends DataPipeline implements Disposable {
private ThreadPoolExecutor threadPoolExecutor;
private boolean disposed = false;
/**
* Constructor.
*
* @param threadPoolCoreSize The core size of the thread pool.
* @param threadPoolMaxSize The max size of the thread pool.
*/
public ThreadPoolDataPipeline(int threadPoolCoreSize, int threadPoolMaxSize) {
super();
this.initThreadPool(threadPoolCoreSize, threadPoolMaxSize, ThreadPoolDataPipeline.class.getSimpleName());
}
/**
* Constructor.
*
* @param threadPoolCoreSize The core size of the thread pool.
* @param threadPoolMaxSize The max size of the thread pool.
* @param threadPrefix The prefix of the thread name.
*/
public ThreadPoolDataPipeline(int threadPoolCoreSize, int threadPoolMaxSize, String threadPrefix) {
super();
this.initThreadPool(threadPoolCoreSize, threadPoolMaxSize, threadPrefix);
}
/**
* Constructor.
*
* @param threadPoolCoreSize The core size of the thread pool.
* @param threadPoolMaxSize The max size of the thread pool.
* @param threadPrefix The prefix of the thread name.
* @param handlers The handlers to be added to the pipeline.
*/
@SafeVarargs
public ThreadPoolDataPipeline(int threadPoolCoreSize, int threadPoolMaxSize, String threadPrefix,
PipelineHandler... handlers) {
super(handlers);
this.initThreadPool(threadPoolCoreSize, threadPoolMaxSize, threadPrefix);
}
@Override
public CompletableFuture begin(T data) {
if (disposed) {
throw new RuntimeException("Cannot process, pipeline has already been disposed.");
}
final var future = new CompletableFuture();
threadPoolExecutor.submit(() -> new PipelineContext<>(this, future).next(data));
return future;
}
@Override
public void dispose() {
disposed = true;
if (threadPoolExecutor != null) {
threadPoolExecutor.shutdownNow();
threadPoolExecutor.purge();
}
}
private void initThreadPool(int threadPoolCoreSize, int threadPoolMaxSize, String threadPrefix) {
threadPoolExecutor = new ThreadPoolExecutor(threadPoolCoreSize, threadPoolMaxSize, 1L,
TimeUnit.SECONDS, new SynchronousQueue<>(), new NamedThreadFactory(threadPrefix),
new ThreadPoolExecutor.CallerRunsPolicy());
}
private static class NamedThreadFactory implements ThreadFactory {
private long uid = 0L;
private final String threadPrefix;
private NamedThreadFactory(String threadPrefix) {
this.threadPrefix = threadPrefix;
}
@Override
public synchronized Thread newThread(Runnable runnable) {
if (++uid < 0) {
uid = 1L;
}
return new Thread(runnable, threadPrefix + "-" + uid);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy