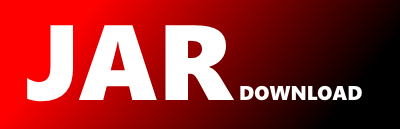
io.github.juniqlim.apicall.http.HttpRequest Maven / Gradle / Ivy
package io.github.juniqlim.apicall.http;
import java.util.HashMap;
import java.util.Map;
import org.springframework.http.HttpMethod;
/**
* interface
*/
public class HttpRequest {
private final HttpMethod httpMethod;
private final String url;
private final Map header;
private final Q request;
private final Class responseType;
private HttpRequest(HttpMethod httpMethod, String url, Map header, Q request, Class responseType) {
this.httpMethod = httpMethod;
this.url = url;
this.header = header;
this.request = request;
this.responseType = responseType;
}
public static HttpRequest of(HttpMethod httpMethod, String url, Map header, Q request,
Class responseType) {
return new HttpRequest<>(httpMethod, url, header, request, responseType);
}
public static HttpRequest of(HttpMethod httpMethod, String url, Map header,
Class responseType) {
return new HttpRequest<>(httpMethod, url, header, null, responseType);
}
public static HttpRequest of(HttpMethod httpMethod, String url, Q request,
Class responseType) {
return new HttpRequest<>(httpMethod, url, new HashMap<>(), request, responseType);
}
public static HttpRequest of(HttpMethod httpMethod, String url, Class responseType) {
return new HttpRequest<>(httpMethod, url, new HashMap<>(), null, responseType);
}
public HttpMethod httpMethod() {
return httpMethod;
}
public String url() {
return url;
}
public Map header() {
return header;
}
public Q request() {
return request;
}
public Class responseType() {
return responseType;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy