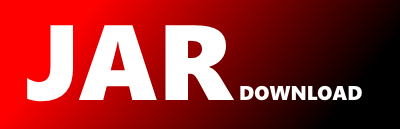
org.gnome.gdk.Display Maven / Gradle / Ivy
Show all versions of gdk Show documentation
/* Java-GI - Java language bindings for GObject-Introspection-based libraries
* Copyright (C) 2022-2023 Jan-Willem Harmannij
*
* SPDX-License-Identifier: LGPL-2.1-or-later
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, see .
*/
/* This file has been generated with Java-GI.
* Do not edit this file directly!
* Visit https://jwharm.github.io/java-gi for more information.
*/
package org.gnome.gdk;
import io.github.jwharm.javagi.gobject.*;
import io.github.jwharm.javagi.gobject.types.*;
import io.github.jwharm.javagi.base.*;
import io.github.jwharm.javagi.interop.*;
import java.lang.foreign.*;
import java.lang.invoke.*;
import org.jetbrains.annotations.*;
/**
* {@code GdkDisplay} objects are the GDK representation of a workstation.
*
* Their purpose are two-fold:
*
* - To manage and provide information about input devices (pointers, keyboards, etc)
*
- To manage and provide information about output devices (monitors, projectors, etc)
*
*
* Most of the input device handling has been factored out into separate
* {@link Seat} objects. Every display has a one or more seats, which
* can be accessed with {@link Display#getDefaultSeat} and
* {@link Display#listSeats}.
*
* Output devices are represented by {@link Monitor} objects, which can
* be accessed with {@link Display#getMonitorAtSurface} and similar APIs.
*/
public class Display extends org.gnome.gobject.GObject {
/**
* Create a Display proxy instance for the provided memory address.
* @param address the memory address of the native object
*/
public Display(MemorySegment address) {
super(address);
}
static {
Gdk.javagi$ensureInitialized();
}
/**
* Get the GType of the GdkDisplay class.
* @return the GType
*/
public static org.gnome.glib.Type getType() {
return Interop.getType("gdk_display_get_type");
}
/**
* Returns this instance as if it were its parent type. This is mostly synonymous to the Java
* {@code super} keyword, but will set the native typeclass function pointers to the parent
* type. When overriding a native virtual method in Java, "chaining up" with
* {@code super.methodName()} doesn't work, because it invokes the overridden function pointer
* again. To chain up, call {@code asParent().methodName()}. This will call the native function
* pointer of this virtual method in the typeclass of the parent type.
*/
public org.gnome.gdk.Display asParent() {
org.gnome.gdk.Display _parent = new org.gnome.gdk.Display(handle());
_parent.callParent(true);
return _parent;
}
/**
* Emits a short beep on {@code display}
*/
public void beep() {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS);
try {
Interop.downcallHandle("gdk_display_beep", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
/**
* Closes the connection to the windowing system for the given display.
*
* This cleans up associated resources.
*/
public void close() {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS);
try {
Interop.downcallHandle("gdk_display_close", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
/**
* Creates a new {@code GdkGLContext} for the {@code GdkDisplay}.
*
* The context is disconnected from any particular surface or surface
* and cannot be used to draw to any surface. It can only be used to
* draw to non-surface framebuffers like textures.
*
* If the creation of the {@code GdkGLContext} failed, {@code error} will be set.
* Before using the returned {@code GdkGLContext}, you will need to
* call {@link GLContext#makeCurrent} or {@link GLContext#realize}.
* @return the newly created {@code GdkGLContext}
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public org.gnome.gdk.GLContext createGlContext() throws GErrorException {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS);
try (Arena _arena = Arena.openConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gdk_display_create_gl_context", _fdesc, false).invokeExact(handle(),
_gerror);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return (org.gnome.gdk.GLContext) InstanceCache.getForType(_result, org.gnome.gdk.GLContext.GLContextImpl::new, true);
}
}
/**
* Returns {@code true} if there is an ongoing grab on {@code device} for {@code display}.
* @param device a {@code GdkDevice}
* @return {@code true} if there is a grab in effect for {@code device}.
*/
public boolean deviceIsGrabbed(org.gnome.gdk.Device device) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS, ValueLayout.ADDRESS);
int _result;
try {
_result = (int) Interop.downcallHandle("gdk_display_device_is_grabbed", _fdesc, false).invokeExact(handle(),
(MemorySegment) (device == null ? MemorySegment.NULL : device.handle()));
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
return _result != 0;
}
/**
* Flushes any requests queued for the windowing system.
*
* This happens automatically when the main loop blocks waiting for new events,
* but if your application is drawing without returning control to the main loop,
* you may need to call this function explicitly. A common case where this function
* needs to be called is when an application is executing drawing commands
* from a thread other than the thread where the main loop is running.
*
* This is most useful for X11. On windowing systems where requests are
* handled synchronously, this function will do nothing.
*/
public void flush() {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS);
try {
Interop.downcallHandle("gdk_display_flush", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
/**
* Returns a {@code GdkAppLaunchContext} suitable for launching
* applications on the given display.
* @return a new {@code GdkAppLaunchContext} for {@code display}
*/
public org.gnome.gdk.AppLaunchContext getAppLaunchContext() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gdk_display_get_app_launch_context", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
return (org.gnome.gdk.AppLaunchContext) InstanceCache.getForType(_result, org.gnome.gdk.AppLaunchContext::new, true);
}
/**
* Gets the clipboard used for copy/paste operations.
* @return the display's clipboard
*/
public org.gnome.gdk.Clipboard getClipboard() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gdk_display_get_clipboard", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
var _object = (org.gnome.gdk.Clipboard) InstanceCache.getForType(_result, org.gnome.gdk.Clipboard::new, true);
if (_object != null) {
GLibLogger.debug("Ref org.gnome.gdk.Clipboard %ld\n", _object == null || _object.handle() == null ? 0 : _object.handle().address());
_object.ref();
}
return _object;
}
/**
* Returns the default {@code GdkSeat} for this display.
*
* Note that a display may not have a seat. In this case,
* this function will return {@code null}.
* @return the default seat.
*/
public @Nullable org.gnome.gdk.Seat getDefaultSeat() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gdk_display_get_default_seat", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
var _object = (org.gnome.gdk.Seat) InstanceCache.getForType(_result, org.gnome.gdk.Seat.SeatImpl::new, true);
if (_object != null) {
GLibLogger.debug("Ref org.gnome.gdk.Seat %ld\n", _object == null || _object.handle() == null ? 0 : _object.handle().address());
_object.ref();
}
return _object;
}
/**
* Gets the monitor in which the largest area of {@code surface}
* resides.
* @param surface a {@code GdkSurface}
* @return the monitor with the largest
* overlap with {@code surface}
*/
public @Nullable org.gnome.gdk.Monitor getMonitorAtSurface(org.gnome.gdk.Surface surface) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gdk_display_get_monitor_at_surface", _fdesc, false).invokeExact(handle(),
(MemorySegment) (surface == null ? MemorySegment.NULL : surface.handle()));
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
var _object = (org.gnome.gdk.Monitor) InstanceCache.getForType(_result, org.gnome.gdk.Monitor::new, true);
if (_object != null) {
GLibLogger.debug("Ref org.gnome.gdk.Monitor %ld\n", _object == null || _object.handle() == null ? 0 : _object.handle().address());
_object.ref();
}
return _object;
}
/**
* Gets the list of monitors associated with this display.
*
* Subsequent calls to this function will always return the
* same list for the same display.
*
* You can listen to the GListModel::items-changed signal on
* this list to monitor changes to the monitor of this display.
* @return a {@code GListModel} of {@code GdkMonitor}
*/
public org.gnome.gio.ListModel getMonitors() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gdk_display_get_monitors", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
return (org.gnome.gio.ListModel) InstanceCache.getForType(_result, org.gnome.gio.ListModel.ListModelImpl::new, true);
}
/**
* Gets the name of the display.
* @return a string representing the display name. This string is owned
* by GDK and should not be modified or freed.
*/
public java.lang.String getName() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS.asUnbounded(), ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gdk_display_get_name", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Gets the clipboard used for the primary selection.
*
* On backends where the primary clipboard is not supported natively,
* GDK emulates this clipboard locally.
* @return the primary clipboard
*/
public org.gnome.gdk.Clipboard getPrimaryClipboard() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gdk_display_get_primary_clipboard", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
var _object = (org.gnome.gdk.Clipboard) InstanceCache.getForType(_result, org.gnome.gdk.Clipboard::new, true);
if (_object != null) {
GLibLogger.debug("Ref org.gnome.gdk.Clipboard %ld\n", _object == null || _object.handle() == null ? 0 : _object.handle().address());
_object.ref();
}
return _object;
}
/**
* Retrieves a desktop-wide setting such as double-click time
* for the {@code display}.
* @param name the name of the setting
* @param value location to store the value of the setting
* @return {@code true} if the setting existed and a value was stored
* in {@code value}, {@code false} otherwise
*/
public boolean getSetting(java.lang.String name, org.gnome.gobject.Value value) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS);
try (Arena _arena = Arena.openConfined()) {
int _result;
try {
_result = (int) Interop.downcallHandle("gdk_display_get_setting", _fdesc, false).invokeExact(handle(),
(MemorySegment) (name == null ? MemorySegment.NULL : Interop.allocateNativeString(name, _arena)),
(MemorySegment) (value == null ? MemorySegment.NULL : value.handle()));
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
return _result != 0;
}
}
/**
* Gets the startup notification ID for a Wayland display, or {@code null}
* if no ID has been defined.
* @return the startup notification ID for {@code display}
*/
@Deprecated
public @Nullable java.lang.String getStartupNotificationId() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS.asUnbounded(), ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gdk_display_get_startup_notification_id", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Finds out if the display has been closed.
* @return {@code true} if the display is closed.
*/
public boolean isClosed() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS);
int _result;
try {
_result = (int) Interop.downcallHandle("gdk_display_is_closed", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
return _result != 0;
}
/**
* Returns whether surfaces can reasonably be expected to have
* their alpha channel drawn correctly on the screen.
*
* Check {@link Display#isRgba} for whether the display
* supports an alpha channel.
*
* On X11 this function returns whether a compositing manager is
* compositing on {@code display}.
*
* On modern displays, this value is always {@code true}.
* @return Whether surfaces with RGBA visuals can reasonably
* be expected to have their alpha channels drawn correctly
* on the screen.
*/
public boolean isComposited() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS);
int _result;
try {
_result = (int) Interop.downcallHandle("gdk_display_is_composited", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
return _result != 0;
}
/**
* Returns whether surfaces on this {@code display} are created with an
* alpha channel.
*
* Even if a {@code true} is returned, it is possible that the
* surface’s alpha channel won’t be honored when displaying the
* surface on the screen: in particular, for X an appropriate
* windowing manager and compositing manager must be running to
* provide appropriate display. Use {@link Display#isComposited}
* to check if that is the case.
*
* On modern displays, this value is always {@code true}.
* @return {@code true} if surfaces are created with an alpha channel or
* {@code false} if the display does not support this functionality.
*/
public boolean isRgba() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS);
int _result;
try {
_result = (int) Interop.downcallHandle("gdk_display_is_rgba", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
return _result != 0;
}
/**
* Returns the list of seats known to {@code display}.
* @return the
* list of seats known to the {@code GdkDisplay}
*/
public org.gnome.glib.List listSeats() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gdk_display_list_seats", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
var _instance = new org.gnome.glib.List(_result);
if (_instance != null) {
MemoryCleaner.takeOwnership(_instance.handle());
}
return _instance;
}
/**
* Returns the keyvals bound to {@code keycode}.
*
* The Nth {@code GdkKeymapKey} in {@code keys} is bound to the Nth keyval in {@code keyvals}.
*
* When a keycode is pressed by the user, the keyval from
* this list of entries is selected by considering the effective
* keyboard group and level.
*
* Free the returned arrays with g_free().
* @param keycode a keycode
* @param keys return
* location for array of {@code GdkKeymapKey}
* @param keyvals return
* location for array of keyvals
* @return {@code true} if there were any entries
*/
public boolean mapKeycode(int keycode, @Nullable Out keys, Out keyvals) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS, ValueLayout.JAVA_INT, ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS);
try (Arena _arena = Arena.openConfined()) {
MemorySegment _nEntriesPointer = _arena.allocate(ValueLayout.JAVA_INT);
Out nEntries = new Out<>();
MemorySegment _keysPointer = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _keyvalsPointer = _arena.allocate(ValueLayout.ADDRESS);
int _result;
try {
_result = (int) Interop.downcallHandle("gdk_display_map_keycode", _fdesc, false).invokeExact(handle(),
keycode,
(MemorySegment) (keys == null ? MemorySegment.NULL : _keysPointer),
(MemorySegment) (keyvals == null ? MemorySegment.NULL : _keyvalsPointer),
_nEntriesPointer);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
nEntries.set(_nEntriesPointer.get(ValueLayout.JAVA_INT, 0));
if (keys != null) {
org.gnome.gdk.KeymapKey[] _keysArray = new org.gnome.gdk.KeymapKey[nEntries.get().intValue()];
for (int _idx = 0; _idx < nEntries.get().intValue(); _idx++) {
var _object = _keysPointer.get(ValueLayout.ADDRESS, _idx);
_keysArray[_idx] = new org.gnome.gdk.KeymapKey(_object);
}
keys.set(_keysArray);
}
if (keyvals != null) keyvals.set(MemorySegment.ofAddress(_keyvalsPointer.get(ValueLayout.ADDRESS, 0).address(), nEntries.get().intValue() * ValueLayout.JAVA_INT.byteSize(), _arena.scope()).toArray(ValueLayout.JAVA_INT));
return _result != 0;
}
}
/**
* Obtains a list of keycode/group/level combinations that will
* generate {@code keyval}.
*
* Groups and levels are two kinds of keyboard mode; in general, the level
* determines whether the top or bottom symbol on a key is used, and the
* group determines whether the left or right symbol is used.
*
* On US keyboards, the shift key changes the keyboard level, and there
* are no groups. A group switch key might convert a keyboard between
* Hebrew to English modes, for example.
*
* {@code GdkEventKey} contains a {@code group} field that indicates the active
* keyboard group. The level is computed from the modifier mask.
*
* The returned array should be freed with g_free().
* @param keyval a keyval, such as {@code GDK_KEY_a}, {@code GDK_KEY_Up}, {@code GDK_KEY_Return}, etc.
* @param keys return location
* for an array of {@code GdkKeymapKey}
* @return {@code true} if keys were found and returned
*/
public boolean mapKeyval(int keyval, Out keys) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS, ValueLayout.JAVA_INT, ValueLayout.ADDRESS, ValueLayout.ADDRESS);
try (Arena _arena = Arena.openConfined()) {
MemorySegment _nKeysPointer = _arena.allocate(ValueLayout.JAVA_INT);
Out nKeys = new Out<>();
MemorySegment _keysPointer = _arena.allocate(ValueLayout.ADDRESS);
int _result;
try {
_result = (int) Interop.downcallHandle("gdk_display_map_keyval", _fdesc, false).invokeExact(handle(),
keyval,
(MemorySegment) (keys == null ? MemorySegment.NULL : _keysPointer),
_nKeysPointer);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
nKeys.set(_nKeysPointer.get(ValueLayout.JAVA_INT, 0));
if (keys != null) {
org.gnome.gdk.KeymapKey[] _keysArray = new org.gnome.gdk.KeymapKey[nKeys.get().intValue()];
for (int _idx = 0; _idx < nKeys.get().intValue(); _idx++) {
var _object = _keysPointer.get(ValueLayout.ADDRESS, _idx);
_keysArray[_idx] = new org.gnome.gdk.KeymapKey(_object);
}
keys.set(_keysArray);
}
return _result != 0;
}
}
/**
* Indicates to the GUI environment that the application has
* finished loading, using a given identifier.
*
* GTK will call this function automatically for {@code org.gnome.gtk.Window}
* with custom startup-notification identifier unless
* {@code org.gnome.gtk.Window#setAutoStartupNotification}
* is called to disable that feature.
* @param startupId a startup-notification identifier, for which
* notification process should be completed
* @deprecated Using {@link Toplevel#setStartupId} is sufficient
*/
@Deprecated
public void notifyStartupComplete(java.lang.String startupId) {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS);
try (Arena _arena = Arena.openConfined()) {
try {
Interop.downcallHandle("gdk_display_notify_startup_complete", _fdesc, false).invokeExact(handle(),
(MemorySegment) (startupId == null ? MemorySegment.NULL : Interop.allocateNativeString(startupId, _arena)));
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
}
/**
* Checks that OpenGL is available for {@code self} and ensures that it is
* properly initialized.
* When this fails, an {@code error} will be set describing the error and this
* function returns {@code false}.
*
* Note that even if this function succeeds, creating a {@code GdkGLContext}
* may still fail.
*
* This function is idempotent. Calling it multiple times will just
* return the same value or error.
*
* You never need to call this function, GDK will call it automatically
* as needed. But you can use it as a check when setting up code that
* might make use of OpenGL.
* @return {@code true} if the display supports OpenGL
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public boolean prepareGl() throws GErrorException {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS, ValueLayout.ADDRESS);
try (Arena _arena = Arena.openConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
int _result;
try {
_result = (int) Interop.downcallHandle("gdk_display_prepare_gl", _fdesc, false).invokeExact(handle(),
_gerror);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return _result != 0;
}
}
/**
* Appends the given event onto the front of the event
* queue for {@code display}.
* @param event a {@code GdkEvent}
* @deprecated This function is only useful in very
* special situations and should not be used by applications.
*/
@Deprecated
public void putEvent(org.gnome.gdk.Event event) {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS);
try {
Interop.downcallHandle("gdk_display_put_event", _fdesc, false).invokeExact(handle(),
(MemorySegment) (event == null ? MemorySegment.NULL : event.handle()));
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
/**
* Returns {@code true} if the display supports input shapes.
*
* This means that {@link Surface#setInputRegion} can
* be used to modify the input shape of surfaces on {@code display}.
*
* On modern displays, this value is always {@code true}.
* @return {@code true} if surfaces with modified input shape are supported
*/
public boolean supportsInputShapes() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS);
int _result;
try {
_result = (int) Interop.downcallHandle("gdk_display_supports_input_shapes", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
return _result != 0;
}
/**
* Flushes any requests queued for the windowing system and waits until all
* requests have been handled.
*
* This is often used for making sure that the display is synchronized
* with the current state of the program. Calling {@link Display#sync}
* before {@code GdkX11.Display#errorTrapPop} makes sure that any errors
* generated from earlier requests are handled before the error trap is removed.
*
* This is most useful for X11. On windowing systems where requests are
* handled synchronously, this function will do nothing.
*/
public void sync() {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS);
try {
Interop.downcallHandle("gdk_display_sync", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
/**
* Translates the contents of a {@code GdkEventKey} into a keyval, effective group,
* and level.
*
* Modifiers that affected the translation and are thus unavailable for
* application use are returned in {@code consumed_modifiers}.
*
* The {@code effective_group} is the group that was actually used for the
* translation; some keys such as Enter are not affected by the active
* keyboard group. The {@code level} is derived from {@code state}.
*
* {@code consumed_modifiers} gives modifiers that should be masked out
* from {@code state} when comparing this key press to a keyboard shortcut.
* For instance, on a US keyboard, the {@code plus} symbol is shifted, so
* when comparing a key press to a {@code plus} accelerator {@code }
* should be masked out.
*
* This function should rarely be needed, since {@code GdkEventKey} already
* contains the translated keyval. It is exported for the benefit of
* virtualized test environments.
* @param keycode a keycode
* @param state a modifier state
* @param group active keyboard group
* @param keyval return location for keyval
* @param effectiveGroup return location for effective group
* @param level return location for level
* @param consumed return location for modifiers that were used
* to determine the group or level
* @return {@code true} if there was a keyval bound to keycode/state/group.
*/
public boolean translateKey(int keycode, org.gnome.gdk.ModifierType state, int group, Out keyval, Out effectiveGroup, Out level, @Nullable Out consumed) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS, ValueLayout.JAVA_INT, ValueLayout.JAVA_INT, ValueLayout.JAVA_INT, ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS);
try (Arena _arena = Arena.openConfined()) {
MemorySegment _keyvalPointer = _arena.allocate(ValueLayout.JAVA_INT);
MemorySegment _effectiveGroupPointer = _arena.allocate(ValueLayout.JAVA_INT);
MemorySegment _levelPointer = _arena.allocate(ValueLayout.JAVA_INT);
MemorySegment _consumedPointer = _arena.allocate(ValueLayout.JAVA_INT);
int _result;
try {
_result = (int) Interop.downcallHandle("gdk_display_translate_key", _fdesc, false).invokeExact(handle(),
keycode,
state.getValue(),
group,
(MemorySegment) (keyval == null ? MemorySegment.NULL : _keyvalPointer),
(MemorySegment) (effectiveGroup == null ? MemorySegment.NULL : _effectiveGroupPointer),
(MemorySegment) (level == null ? MemorySegment.NULL : _levelPointer),
(MemorySegment) (consumed == null ? MemorySegment.NULL : _consumedPointer));
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
if (keyval != null) keyval.set(_keyvalPointer.get(ValueLayout.JAVA_INT, 0));
if (effectiveGroup != null) effectiveGroup.set(_effectiveGroupPointer.get(ValueLayout.JAVA_INT, 0));
if (level != null) level.set(_levelPointer.get(ValueLayout.JAVA_INT, 0));
if (consumed != null) consumed.set(new org.gnome.gdk.ModifierType(_consumedPointer.get(ValueLayout.JAVA_INT, 0)));
return _result != 0;
}
}
/**
* Gets the default {@code GdkDisplay}.
*
* This is a convenience function for:
*
* gdk_display_manager_get_default_display (gdk_display_manager_get ())
* @return a {@code GdkDisplay}, or {@code null} if
* there is no default display
*/
public static @Nullable org.gnome.gdk.Display getDefault() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gdk_display_get_default", _fdesc, false).invokeExact();
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
var _object = (org.gnome.gdk.Display) InstanceCache.getForType(_result, org.gnome.gdk.Display::new, true);
if (_object != null) {
GLibLogger.debug("Ref org.gnome.gdk.Display %ld\n", _object == null || _object.handle() == null ? 0 : _object.handle().address());
_object.ref();
}
return _object;
}
/**
* Opens a display.
*
* If opening the display fails, {@code NULL} is returned.
* @param displayName the name of the display to open
* @return a {@code GdkDisplay}
*/
public static @Nullable org.gnome.gdk.Display open(@Nullable java.lang.String displayName) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS);
try (Arena _arena = Arena.openConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) Interop.downcallHandle("gdk_display_open", _fdesc, false).invokeExact((MemorySegment) (displayName == null ? MemorySegment.NULL : Interop.allocateNativeString(displayName, _arena)));
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
var _object = (org.gnome.gdk.Display) InstanceCache.getForType(_result, org.gnome.gdk.Display::new, true);
if (_object != null) {
GLibLogger.debug("Ref org.gnome.gdk.Display %ld\n", _object == null || _object.handle() == null ? 0 : _object.handle().address());
_object.ref();
}
return _object;
}
}
@FunctionalInterface
public interface Closed {
/**
* Emitted when the connection to the windowing system for {@code display} is closed.
*/
void run(boolean isError);
/**
* The {@code upcall} method is called from native code. The parameters
* are marshalled and {@link #run} is executed.
*/
@ApiStatus.Internal default void upcall(MemorySegment sourceDisplay, int isError) {
run(isError != 0);
}
/**
* Creates a callback that can be called from native code and executes the {@code run} method.
* @return the memory address of the callback function
*/
default MemorySegment toCallback() {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.JAVA_INT);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), Closed.class, _fdesc);
return Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, SegmentScope.global());
}
}
/**
* Emitted when the connection to the windowing system for {@code display} is closed.
* @param handler the signal handler
* @return a {@link SignalConnection} object to keep track of the signal connection
*/
public SignalConnection onClosed(Display.Closed handler) {
try (Arena _arena = Arena.openConfined()) {
try {
var _result = (long) Signals.g_signal_connect_data.invokeExact(
handle(), Interop.allocateNativeString("closed", _arena), handler.toCallback(), MemorySegment.NULL, MemorySegment.NULL, 0);
return new SignalConnection<>(handle(), _result);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
}
/**
* Emits the "closed" signal. See {@link #onClosed}.
*/
public void emitClosed(boolean isError) {
try (Arena _arena = Arena.openConfined()) {
Signals.g_signal_emit_by_name.invokeExact(
handle(),
Interop.allocateNativeString("closed", _arena),
new Object[] {
isError ? 1 : 0
}
);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
@FunctionalInterface
public interface Opened {
/**
* Emitted when the connection to the windowing system for {@code display} is opened.
*/
void run();
/**
* The {@code upcall} method is called from native code. The parameters
* are marshalled and {@link #run} is executed.
*/
@ApiStatus.Internal default void upcall(MemorySegment sourceDisplay) {
run();
}
/**
* Creates a callback that can be called from native code and executes the {@code run} method.
* @return the memory address of the callback function
*/
default MemorySegment toCallback() {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), Opened.class, _fdesc);
return Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, SegmentScope.global());
}
}
/**
* Emitted when the connection to the windowing system for {@code display} is opened.
* @param handler the signal handler
* @return a {@link SignalConnection} object to keep track of the signal connection
*/
public SignalConnection onOpened(Display.Opened handler) {
try (Arena _arena = Arena.openConfined()) {
try {
var _result = (long) Signals.g_signal_connect_data.invokeExact(
handle(), Interop.allocateNativeString("opened", _arena), handler.toCallback(), MemorySegment.NULL, MemorySegment.NULL, 0);
return new SignalConnection<>(handle(), _result);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
}
/**
* Emits the "opened" signal. See {@link #onOpened}.
*/
public void emitOpened() {
try (Arena _arena = Arena.openConfined()) {
Signals.g_signal_emit_by_name.invokeExact(
handle(),
Interop.allocateNativeString("opened", _arena), new Object[0]);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
@FunctionalInterface
public interface SeatAdded {
/**
* Emitted whenever a new seat is made known to the windowing system.
*/
void run(org.gnome.gdk.Seat seat);
/**
* The {@code upcall} method is called from native code. The parameters
* are marshalled and {@link #run} is executed.
*/
@ApiStatus.Internal default void upcall(MemorySegment sourceDisplay, MemorySegment seat) {
run((org.gnome.gdk.Seat) InstanceCache.getForType(seat, org.gnome.gdk.Seat.SeatImpl::new, false));
}
/**
* Creates a callback that can be called from native code and executes the {@code run} method.
* @return the memory address of the callback function
*/
default MemorySegment toCallback() {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), SeatAdded.class, _fdesc);
return Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, SegmentScope.global());
}
}
/**
* Emitted whenever a new seat is made known to the windowing system.
* @param handler the signal handler
* @return a {@link SignalConnection} object to keep track of the signal connection
*/
public SignalConnection onSeatAdded(Display.SeatAdded handler) {
try (Arena _arena = Arena.openConfined()) {
try {
var _result = (long) Signals.g_signal_connect_data.invokeExact(
handle(), Interop.allocateNativeString("seat-added", _arena), handler.toCallback(), MemorySegment.NULL, MemorySegment.NULL, 0);
return new SignalConnection<>(handle(), _result);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
}
/**
* Emits the "seat-added" signal. See {@link #onSeatAdded}.
*/
public void emitSeatAdded(org.gnome.gdk.Seat seat) {
try (Arena _arena = Arena.openConfined()) {
Signals.g_signal_emit_by_name.invokeExact(
handle(),
Interop.allocateNativeString("seat-added", _arena),
new Object[] {
(MemorySegment) (seat == null ? MemorySegment.NULL : seat.handle())
}
);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
@FunctionalInterface
public interface SeatRemoved {
/**
* Emitted whenever a seat is removed by the windowing system.
*/
void run(org.gnome.gdk.Seat seat);
/**
* The {@code upcall} method is called from native code. The parameters
* are marshalled and {@link #run} is executed.
*/
@ApiStatus.Internal default void upcall(MemorySegment sourceDisplay, MemorySegment seat) {
run((org.gnome.gdk.Seat) InstanceCache.getForType(seat, org.gnome.gdk.Seat.SeatImpl::new, false));
}
/**
* Creates a callback that can be called from native code and executes the {@code run} method.
* @return the memory address of the callback function
*/
default MemorySegment toCallback() {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), SeatRemoved.class, _fdesc);
return Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, SegmentScope.global());
}
}
/**
* Emitted whenever a seat is removed by the windowing system.
* @param handler the signal handler
* @return a {@link SignalConnection} object to keep track of the signal connection
*/
public SignalConnection onSeatRemoved(Display.SeatRemoved handler) {
try (Arena _arena = Arena.openConfined()) {
try {
var _result = (long) Signals.g_signal_connect_data.invokeExact(
handle(), Interop.allocateNativeString("seat-removed", _arena), handler.toCallback(), MemorySegment.NULL, MemorySegment.NULL, 0);
return new SignalConnection<>(handle(), _result);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
}
/**
* Emits the "seat-removed" signal. See {@link #onSeatRemoved}.
*/
public void emitSeatRemoved(org.gnome.gdk.Seat seat) {
try (Arena _arena = Arena.openConfined()) {
Signals.g_signal_emit_by_name.invokeExact(
handle(),
Interop.allocateNativeString("seat-removed", _arena),
new Object[] {
(MemorySegment) (seat == null ? MemorySegment.NULL : seat.handle())
}
);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
@FunctionalInterface
public interface SettingChanged {
/**
* Emitted whenever a setting changes its value.
*/
void run(java.lang.String setting);
/**
* The {@code upcall} method is called from native code. The parameters
* are marshalled and {@link #run} is executed.
*/
@ApiStatus.Internal default void upcall(MemorySegment sourceDisplay, MemorySegment setting) {
try (Arena _arena = Arena.openConfined()) {
run(Interop.getStringFrom(setting, false));
}
}
/**
* Creates a callback that can be called from native code and executes the {@code run} method.
* @return the memory address of the callback function
*/
default MemorySegment toCallback() {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS.asUnbounded());
MethodHandle _handle = Interop.upcallHandle(MethodHandles.lookup(), SettingChanged.class, _fdesc);
return Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, SegmentScope.global());
}
}
/**
* Emitted whenever a setting changes its value.
* @param handler the signal handler
* @return a {@link SignalConnection} object to keep track of the signal connection
*/
public SignalConnection onSettingChanged(Display.SettingChanged handler) {
try (Arena _arena = Arena.openConfined()) {
try {
var _result = (long) Signals.g_signal_connect_data.invokeExact(
handle(), Interop.allocateNativeString("setting-changed", _arena), handler.toCallback(), MemorySegment.NULL, MemorySegment.NULL, 0);
return new SignalConnection<>(handle(), _result);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
}
/**
* Emits the "setting-changed" signal. See {@link #onSettingChanged}.
*/
public void emitSettingChanged(java.lang.String setting) {
try (Arena _arena = Arena.openConfined()) {
Signals.g_signal_emit_by_name.invokeExact(
handle(),
Interop.allocateNativeString("setting-changed", _arena),
new Object[] {
(MemorySegment) (setting == null ? MemorySegment.NULL : Interop.allocateNativeString(setting, _arena))
}
);
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
}
/**
* A {@link Display.Builder} object constructs a {@code Display}
* using the builder pattern to set property values.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Display.Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
/**
* Inner class implementing a builder pattern to construct
* a GObject with properties.
* @param the type of the Builder that is returned
*/
public static class Builder> extends org.gnome.gobject.GObject.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code Display} object. This will call
* {@link org.gnome.gobject.GObject#newWithProperties} to create a new
* GObject instance, which is then cast to {@code Display}.
* @return A new instance of {@code Display} with the properties
* that were set in the Builder object.
*/
public org.gnome.gdk.Display build() {
try {
return (org.gnome.gdk.Display) org.gnome.gobject.GObject.newWithProperties(
org.gnome.gdk.Display.getType(), getNames(), getValues()
);
} finally {
for (var _value : getValues()) {
_value.unset();
}
}
}
/**
* {@code true} if the display properly composites the alpha channel.
* @param composited the value for the {@code composited} property
* @return the {@code Build} instance is returned, to allow method chaining
*/
public S composited(boolean composited) {
org.gnome.gobject.Value _value = org.gnome.gobject.Value.allocate();
_value.init(Types.BOOLEAN);
_value.setBoolean(composited);
addBuilderProperty("composited", _value);
return (S) this;
}
/**
* {@code true} if the display supports input shapes.
* @param inputShapes the value for the {@code input-shapes} property
* @return the {@code Build} instance is returned, to allow method chaining
*/
public S inputShapes(boolean inputShapes) {
org.gnome.gobject.Value _value = org.gnome.gobject.Value.allocate();
_value.init(Types.BOOLEAN);
_value.setBoolean(inputShapes);
addBuilderProperty("input-shapes", _value);
return (S) this;
}
/**
* {@code true} if the display supports an alpha channel.
* @param rgba the value for the {@code rgba} property
* @return the {@code Build} instance is returned, to allow method chaining
*/
public S rgba(boolean rgba) {
org.gnome.gobject.Value _value = org.gnome.gobject.Value.allocate();
_value.init(Types.BOOLEAN);
_value.setBoolean(rgba);
addBuilderProperty("rgba", _value);
return (S) this;
}
}
}