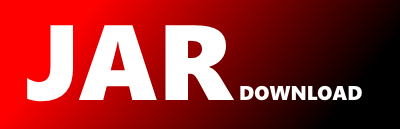
org.gnome.gdk.ScrollEvent Maven / Gradle / Ivy
Show all versions of gdk Show documentation
/* Java-GI - Java language bindings for GObject-Introspection-based libraries
* Copyright (C) 2022-2023 Jan-Willem Harmannij
*
* SPDX-License-Identifier: LGPL-2.1-or-later
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, see .
*/
/* This file has been generated with Java-GI.
* Do not edit this file directly!
* Visit https://jwharm.github.io/java-gi for more information.
*/
package org.gnome.gdk;
import io.github.jwharm.javagi.gobject.*;
import io.github.jwharm.javagi.gobject.types.*;
import io.github.jwharm.javagi.base.*;
import io.github.jwharm.javagi.interop.*;
import java.lang.foreign.*;
import java.lang.invoke.*;
import org.jetbrains.annotations.*;
/**
* An event related to a scrolling motion.
*/
public class ScrollEvent extends org.gnome.gdk.Event {
/**
* Create a ScrollEvent proxy instance for the provided memory address.
* @param address the memory address of the native object
*/
public ScrollEvent(MemorySegment address) {
super(address);
}
static {
Gdk.javagi$ensureInitialized();
}
/**
* Get the GType of the GdkScrollEvent class.
* @return the GType
*/
public static org.gnome.glib.Type getType() {
return Interop.getType("gdk_scroll_event_get_type");
}
/**
* Returns this instance as if it were its parent type. This is mostly synonymous to the Java
* {@code super} keyword, but will set the native typeclass function pointers to the parent
* type. When overriding a native virtual method in Java, "chaining up" with
* {@code super.methodName()} doesn't work, because it invokes the overridden function pointer
* again. To chain up, call {@code asParent().methodName()}. This will call the native function
* pointer of this virtual method in the typeclass of the parent type.
*/
public org.gnome.gdk.ScrollEvent asParent() {
org.gnome.gdk.ScrollEvent _parent = new org.gnome.gdk.ScrollEvent(handle());
_parent.callParent(true);
return _parent;
}
/**
* Extracts the scroll deltas of a scroll event.
*
* The deltas will be zero unless the scroll direction
* is {@link ScrollDirection#SMOOTH}.
*
* For the representation unit of these deltas, see
* {@link ScrollEvent#getUnit}.
* @param deltaX return location for x scroll delta
* @param deltaY return location for y scroll delta
*/
public void getDeltas(Out deltaX, Out deltaY) {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS);
try (Arena _arena = Arena.openConfined()) {
MemorySegment _deltaXPointer = _arena.allocate(ValueLayout.JAVA_DOUBLE);
MemorySegment _deltaYPointer = _arena.allocate(ValueLayout.JAVA_DOUBLE);
try {
Interop.downcallHandle("gdk_scroll_event_get_deltas", _fdesc, false).invokeExact(handle(),
(MemorySegment) (deltaX == null ? MemorySegment.NULL : _deltaXPointer),
(MemorySegment) (deltaY == null ? MemorySegment.NULL : _deltaYPointer));
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
if (deltaX != null) deltaX.set(_deltaXPointer.get(ValueLayout.JAVA_DOUBLE, 0));
if (deltaY != null) deltaY.set(_deltaYPointer.get(ValueLayout.JAVA_DOUBLE, 0));
}
}
/**
* Extracts the direction of a scroll event.
* @return the scroll direction of {@code event}
*/
public org.gnome.gdk.ScrollDirection getDirection() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS);
int _result;
try {
_result = (int) Interop.downcallHandle("gdk_scroll_event_get_direction", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
return org.gnome.gdk.ScrollDirection.of(_result);
}
/**
* Extracts the scroll delta unit of a scroll event.
*
* The unit will always be {@link ScrollUnit#WHEEL} if the scroll direction is not
* {@link ScrollDirection#SMOOTH}.
* @return the scroll unit.
*/
public org.gnome.gdk.ScrollUnit getUnit() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS);
int _result;
try {
_result = (int) Interop.downcallHandle("gdk_scroll_event_get_unit", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
return org.gnome.gdk.ScrollUnit.of(_result);
}
/**
* Check whether a scroll event is a stop scroll event.
*
* Scroll sequences with smooth scroll information may provide
* a stop scroll event once the interaction with the device finishes,
* e.g. by lifting a finger. This stop scroll event is the signal
* that a widget may trigger kinetic scrolling based on the current
* velocity.
*
* Stop scroll events always have a delta of 0/0.
* @return {@code true} if the event is a scroll stop event
*/
public boolean isStop() {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS);
int _result;
try {
_result = (int) Interop.downcallHandle("gdk_scroll_event_is_stop", _fdesc, false).invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError("Unexpected exception occured: ", _err);
}
return _result != 0;
}
}