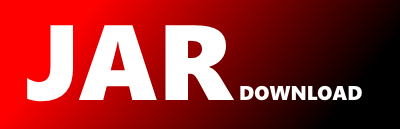
org.gnome.gio.DBusServer Maven / Gradle / Ivy
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gio;
import io.github.jwharm.javagi.base.FunctionPointer;
import io.github.jwharm.javagi.base.GErrorException;
import io.github.jwharm.javagi.base.GLibLogger;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.gobject.SignalConnection;
import io.github.jwharm.javagi.gobject.types.Signals;
import io.github.jwharm.javagi.gobject.types.Types;
import io.github.jwharm.javagi.interop.Arenas;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.FunctionalInterface;
import java.lang.String;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.Linker;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import java.util.EnumSet;
import java.util.Set;
import javax.annotation.processing.Generated;
import org.gnome.glib.Type;
import org.gnome.gobject.GObject;
import org.gnome.gobject.Value;
import org.jetbrains.annotations.Nullable;
/**
* {@code GDBusServer} is a helper for listening to and accepting D-Bus
* connections. This can be used to create a new D-Bus server, allowing two
* peers to use the D-Bus protocol for their own specialized communication.
* A server instance provided in this way will not perform message routing or
* implement the
* {@code org.freedesktop.DBus} interface.
*
* To just export an object on a well-known name on a message bus, such as the
* session or system bus, you should instead use {@link Gio#busOwnName}.
*
* An example of peer-to-peer communication with GDBus can be found
* in gdbus-example-peer.c.
*
* Note that a minimal {@code GDBusServer} will accept connections from any
* peer. In many use-cases it will be necessary to add a
* {@link DBusAuthObserver} that only accepts connections that have
* successfully authenticated as the same user that is running the
* {@code GDBusServer}. Since GLib 2.68 this can be achieved more simply by passing
* the {@code G_DBUS_SERVER_FLAGS_AUTHENTICATION_REQUIRE_SAME_USER} flag to the
* server.
*
* @version 2.26
*/
@Generated("io.github.jwharm.JavaGI")
public class DBusServer extends GObject implements Initable {
static {
Gio.javagi$ensureInitialized();
}
/**
* Create a DBusServer proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public DBusServer(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Get the GType of the DBusServer class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("g_dbus_server_get_type");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected DBusServer asParent() {
DBusServer _parent = new DBusServer(handle());
_parent.callParent(true);
return _parent;
}
/**
* Creates a new D-Bus server that listens on the first address in
* {@code address} that works.
*
* Once constructed, you can use g_dbus_server_get_client_address() to
* get a D-Bus address string that clients can use to connect.
*
* To have control over the available authentication mechanisms and
* the users that are authorized to connect, it is strongly recommended
* to provide a non-{@code null} {@code GDBusAuthObserver}.
*
* Connect to the {@code GDBusServer}::new-connection signal to handle
* incoming connections.
*
* The returned {@code GDBusServer} isn't active - you have to start it with
* g_dbus_server_start().
*
* {@code GDBusServer} is used in this [example][gdbus-peer-to-peer].
*
* This is a synchronous failable constructor. There is currently no
* asynchronous version.
*
* @param address A D-Bus address.
* @param flags Flags from the {@code GDBusServerFlags} enumeration.
* @param guid A D-Bus GUID.
* @param observer A {@code GDBusAuthObserver} or {@code null}.
* @param cancellable A {@code GCancellable} or {@code null}.
* @return A {@code GDBusServer} or {@code null} if {@code error} is set. Free with
* g_object_unref().
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public static DBusServer sync(String address, Set flags, String guid,
@Nullable DBusAuthObserver observer, @Nullable Cancellable cancellable) throws
GErrorException {
var _result = constructSync(address, flags, guid, observer, cancellable);
var _object = (DBusServer) InstanceCache.getForType(_result, DBusServer::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gio.DBusServer %ld", _gobject.handle().address());
_gobject.ref();
}
return (DBusServer) _object;
}
private static MemorySegment constructSync(String address, Set flags,
String guid, @Nullable DBusAuthObserver observer, @Nullable Cancellable cancellable)
throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_dbus_server_new_sync.invokeExact(
(MemorySegment) (address == null ? MemorySegment.NULL : Interop.allocateNativeString(address, _arena)),
Interop.enumSetToInt(flags),
(MemorySegment) (guid == null ? MemorySegment.NULL : Interop.allocateNativeString(guid, _arena)),
(MemorySegment) (observer == null ? MemorySegment.NULL : observer.handle()),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return _result;
}
}
/**
* Creates a new D-Bus server that listens on the first address in
* {@code address} that works.
*
* Once constructed, you can use g_dbus_server_get_client_address() to
* get a D-Bus address string that clients can use to connect.
*
* To have control over the available authentication mechanisms and
* the users that are authorized to connect, it is strongly recommended
* to provide a non-{@code null} {@code GDBusAuthObserver}.
*
* Connect to the {@code GDBusServer}::new-connection signal to handle
* incoming connections.
*
* The returned {@code GDBusServer} isn't active - you have to start it with
* g_dbus_server_start().
*
* {@code GDBusServer} is used in this [example][gdbus-peer-to-peer].
*
* This is a synchronous failable constructor. There is currently no
* asynchronous version.
*
* @param address A D-Bus address.
* @param flags Flags from the {@code GDBusServerFlags} enumeration.
* @param guid A D-Bus GUID.
* @param observer A {@code GDBusAuthObserver} or {@code null}.
* @param cancellable A {@code GCancellable} or {@code null}.
* @return A {@code GDBusServer} or {@code null} if {@code error} is set. Free with
* g_object_unref().
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public static DBusServer sync(String address, DBusServerFlags flags, String guid,
@Nullable DBusAuthObserver observer, @Nullable Cancellable cancellable) throws
GErrorException {
return sync(address, EnumSet.of(flags), guid, observer, cancellable);
}
/**
* Gets a
* D-Bus address
* string that can be used by clients to connect to this DBusServer.
*
* This is valid and non-empty if initializing the {@code GDBusServer} succeeded.
*
* @return A D-Bus address string. Do not free, the string is owned
* by this DBusServer.
*/
public String getClientAddress() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_dbus_server_get_client_address.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Gets the flags for this DBusServer.
*
* @return A set of flags from the {@code GDBusServerFlags} enumeration.
*/
public Set getFlags() {
int _result;
try {
_result = (int) MethodHandles.g_dbus_server_get_flags.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.intToEnumSet(DBusServerFlags.class, DBusServerFlags::of, _result);
}
/**
* Gets the GUID for this DBusServer, as provided to g_dbus_server_new_sync().
*
* @return A D-Bus GUID. Do not free this string, it is owned by this DBusServer.
*/
public String getGuid() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_dbus_server_get_guid.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Gets whether this DBusServer is active.
*
* @return {@code true} if server is active, {@code false} otherwise.
*/
public boolean isActive() {
int _result;
try {
_result = (int) MethodHandles.g_dbus_server_is_active.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
/**
* Starts this DBusServer.
*/
public void start() {
try {
MethodHandles.g_dbus_server_start.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Stops this DBusServer.
*/
public void stop() {
try {
MethodHandles.g_dbus_server_stop.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Emitted when a new authenticated connection has been made. Use
* g_dbus_connection_get_peer_credentials() to figure out what
* identity (if any), was authenticated.
*
* If you want to accept the connection, take a reference to the
* {@code connection} object and return {@code true}. When you are done with the
* connection call g_dbus_connection_close() and give up your
* reference. Note that the other peer may disconnect at any time -
* a typical thing to do when accepting a connection is to listen to
* the {@code GDBusConnection}::closed signal.
*
* If {@code GDBusServer}:flags contains {@link org.gnome.gio.DBusServerFlags#RUN_IN_THREAD}
* then the signal is emitted in a new thread dedicated to the
* connection. Otherwise the signal is emitted in the
* [thread-default main context][g-main-context-push-thread-default]
* of the thread that {@code server} was constructed in.
*
* You are guaranteed that signal handlers for this signal runs
* before incoming messages on {@code connection} are processed. This means
* that it's suitable to call g_dbus_connection_register_object() or
* similar from the signal handler.
*
* @param handler the signal handler
* @return a signal handler ID to keep track of the signal connection
* @see NewConnectionCallback#run
*/
public SignalConnection onNewConnection(NewConnectionCallback handler) {
try (Arena _arena = Arena.ofConfined()) {
try {
var _name = Interop.allocateNativeString("new-connection", _arena);
var _callbackArena = Arena.ofShared();
var _result = (int) (long) Signals.g_signal_connect_data.invokeExact(handle(),
_name, handler.toCallback(_callbackArena),
Arenas.cacheArena(_callbackArena), Arenas.CLOSE_CB_SYM, 0);
return new SignalConnection<>(handle(), _result);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Emits the "new-connection" signal. See {@link #onNewConnection}.
*/
public boolean emitNewConnection(DBusConnection connection) {
try (Arena _arena = Arena.ofConfined()) {
MemorySegment _result = _arena.allocate(ValueLayout.JAVA_INT);
MemorySegment _name = Interop.allocateNativeString("new-connection", _arena);
Object[] _args = new Object[] {
(MemorySegment) (connection == null ? MemorySegment.NULL : connection.handle()), _result};
Signals.g_signal_emit_by_name.invokeExact(handle(), _name, _args);
return _result.get(ValueLayout.JAVA_INT, 0) != 0;
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* A {@link Builder} object constructs a {@code DBusServer}
* with the specified properties.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
/**
* Functional interface declaration of the {@code NewConnectionCallback} callback.
*
* @see NewConnectionCallback#run
*/
@FunctionalInterface
public interface NewConnectionCallback extends FunctionPointer {
/**
* Emitted when a new authenticated connection has been made. Use
* g_dbus_connection_get_peer_credentials() to figure out what
* identity (if any), was authenticated.
*
* If you want to accept the connection, take a reference to the
* {@code connection} object and return {@code true}. When you are done with the
* connection call g_dbus_connection_close() and give up your
* reference. Note that the other peer may disconnect at any time -
* a typical thing to do when accepting a connection is to listen to
* the {@code GDBusConnection}::closed signal.
*
* If {@code GDBusServer}:flags contains {@link org.gnome.gio.DBusServerFlags#RUN_IN_THREAD}
* then the signal is emitted in a new thread dedicated to the
* connection. Otherwise the signal is emitted in the
* [thread-default main context][g-main-context-push-thread-default]
* of the thread that {@code server} was constructed in.
*
* You are guaranteed that signal handlers for this signal runs
* before incoming messages on {@code connection} are processed. This means
* that it's suitable to call g_dbus_connection_register_object() or
* similar from the signal handler.
*/
boolean run(DBusConnection connection);
/**
* The {@code upcall} method is called from native code. The parameters
* are marshaled and {@link #run} is executed.
*/
default int upcall(MemorySegment sourceDBusServer, MemorySegment connection) {
var _result = run((DBusConnection) InstanceCache.getForType(connection, DBusConnection::new, false));
return _result ? 1 : 0;
}
/**
* Creates a native function pointer to the {@link #upcall} method.
*
* @return the native function pointer
*/
default MemorySegment toCallback(Arena arena) {
FunctionDescriptor _fdesc = FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(java.lang.invoke.MethodHandles.lookup(), NewConnectionCallback.class, _fdesc);
return Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
}
}
/**
* Inner class implementing a builder pattern to construct a GObject with
* properties.
*
* @param the type of the Builder that is returned
*/
public static class Builder> extends GObject.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code DBusServer} object. This will call
* {@link GObject#withProperties} to create a new GObject instance,
* which is then cast to {@code DBusServer}.
*
* @return a new instance of {@code DBusServer} with the properties
* that were set in the Builder object.
*/
public DBusServer build() {
try {
var _instance = (DBusServer) GObject.withProperties(DBusServer.getType(), getNames(), getValues());
connectSignals(_instance.handle());
return _instance;
} finally {
for (Value _value : getValues()) _value.unset();
getArena().close();
}
}
/**
* The D-Bus address to listen on.
*
* @param address the value for the {@code address} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setAddress(String address) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(address);
addBuilderProperty("address", _value);
return (B) this;
}
/**
* A {@code GDBusAuthObserver} object to assist in the authentication process or {@code null}.
*
* @param authenticationObserver the value for the {@code authentication-observer} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setAuthenticationObserver(DBusAuthObserver authenticationObserver) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(DBusAuthObserver.getType());
_value.setObject((GObject) authenticationObserver);
addBuilderProperty("authentication-observer", _value);
return (B) this;
}
/**
* Flags from the {@code GDBusServerFlags} enumeration.
*
* @param flags the value for the {@code flags} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setFlags(Set flags) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(DBusServerFlags.getType());
_value.setFlags(Interop.enumSetToInt(flags));
addBuilderProperty("flags", _value);
return (B) this;
}
/**
* The GUID of the server.
*
* See {@code GDBusConnection}:guid for more details.
*
* @param guid the value for the {@code guid} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setGuid(String guid) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(guid);
addBuilderProperty("guid", _value);
return (B) this;
}
/**
* Flags from the {@code GDBusServerFlags} enumeration.
*
* @param flags the value for the {@code flags} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setFlags(DBusServerFlags... flags) {
return setFlags((flags == null ? null : (flags.length == 0) ? EnumSet.noneOf(DBusServerFlags.class) : EnumSet.of(flags[0], flags)));
}
/**
* Emitted when a new authenticated connection has been made. Use
* g_dbus_connection_get_peer_credentials() to figure out what
* identity (if any), was authenticated.
*
* If you want to accept the connection, take a reference to the
* {@code connection} object and return {@code true}. When you are done with the
* connection call g_dbus_connection_close() and give up your
* reference. Note that the other peer may disconnect at any time -
* a typical thing to do when accepting a connection is to listen to
* the {@code GDBusConnection}::closed signal.
*
* If {@code GDBusServer}:flags contains {@link org.gnome.gio.DBusServerFlags#RUN_IN_THREAD}
* then the signal is emitted in a new thread dedicated to the
* connection. Otherwise the signal is emitted in the
* [thread-default main context][g-main-context-push-thread-default]
* of the thread that {@code server} was constructed in.
*
* You are guaranteed that signal handlers for this signal runs
* before incoming messages on {@code connection} are processed. This means
* that it's suitable to call g_dbus_connection_register_object() or
* similar from the signal handler.
*
* @param handler the signal handler
* @return the {@code Builder} instance is returned, to allow method chaining
* @see NewConnectionCallback#run
*/
public B onNewConnection(NewConnectionCallback handler) {
connect("new-connection", handler);
return (B) this;
}
}
private static final class MethodHandles {
static final MethodHandle g_dbus_server_new_sync = Interop.downcallHandle(
"g_dbus_server_new_sync", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.JAVA_INT, ValueLayout.ADDRESS, ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle g_dbus_server_get_client_address = Interop.downcallHandle(
"g_dbus_server_get_client_address", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle g_dbus_server_get_flags = Interop.downcallHandle(
"g_dbus_server_get_flags", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle g_dbus_server_get_guid = Interop.downcallHandle(
"g_dbus_server_get_guid", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle g_dbus_server_is_active = Interop.downcallHandle(
"g_dbus_server_is_active", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS), false);
static final MethodHandle g_dbus_server_start = Interop.downcallHandle(
"g_dbus_server_start", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS), false);
static final MethodHandle g_dbus_server_stop = Interop.downcallHandle("g_dbus_server_stop",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS), false);
}
}