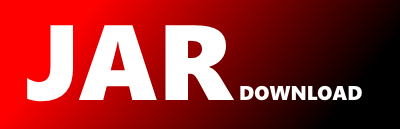
org.gnome.gio.DBusSubtreeVTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gio Show documentation
Show all versions of gio Show documentation
Java language bindings for Gio, generated with Java-GI
The newest version!
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gio;
import io.github.jwharm.javagi.base.ProxyInstance;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.foreign.Arena;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import javax.annotation.processing.Generated;
/**
* Virtual table for handling subtrees registered with g_dbus_connection_register_subtree().
*
* @version 2.26
*/
@Generated("io.github.jwharm.JavaGI")
public class DBusSubtreeVTable extends ProxyInstance {
static {
Gio.javagi$ensureInitialized();
}
/**
* Create a DBusSubtreeVTable proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public DBusSubtreeVTable(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Allocate a new DBusSubtreeVTable.
*
* @param arena to control the memory allocation scope
*/
public DBusSubtreeVTable(Arena arena) {
super(arena.allocate(getMemoryLayout()));
}
/**
* Allocate a new DBusSubtreeVTable.
* The memory is allocated with {@link Arena#ofAuto}.
*/
public DBusSubtreeVTable() {
super(Arena.ofAuto().allocate(getMemoryLayout()));
}
/**
* Allocate a new DBusSubtreeVTable with the fields set to the provided values.
*
* @param enumerate value for the field {@code enumerate}
* @param introspect value for the field {@code introspect}
* @param dispatch value for the field {@code dispatch}
* @param arena to control the memory allocation scope
*/
public DBusSubtreeVTable(DBusSubtreeEnumerateFunc enumerate,
DBusSubtreeIntrospectFunc introspect, DBusSubtreeDispatchFunc dispatch, Arena arena) {
this(arena);
writeEnumerate(enumerate, arena);
writeIntrospect(introspect, arena);
writeDispatch(dispatch, arena);
}
/**
* Allocate a new DBusSubtreeVTable with the fields set to the provided values.
* The memory is allocated with {@link Arena#ofAuto}.
*
* @param enumerate value for the field {@code enumerate}
* @param introspect value for the field {@code introspect}
* @param dispatch value for the field {@code dispatch}
*/
public DBusSubtreeVTable(DBusSubtreeEnumerateFunc enumerate,
DBusSubtreeIntrospectFunc introspect, DBusSubtreeDispatchFunc dispatch) {
this(Arena.ofAuto());
writeEnumerate(enumerate, Arena.ofAuto());
writeIntrospect(introspect, Arena.ofAuto());
writeDispatch(dispatch, Arena.ofAuto());
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
ValueLayout.ADDRESS.withName("enumerate"),
ValueLayout.ADDRESS.withName("introspect"),
ValueLayout.ADDRESS.withName("dispatch"),
MemoryLayout.sequenceLayout(8, ValueLayout.ADDRESS).withName("padding")
).withName("GDBusSubtreeVTable");
}
/**
* Read the value of the field {@code enumerate}.
*
* @return The value of the field {@code enumerate}
*/
public DBusSubtreeEnumerateFunc readEnumerate() {
Arena _arena = Arena.ofAuto();
var _result = (MemorySegment) getMemoryLayout()
.varHandle(MemoryLayout.PathElement.groupElement("enumerate")).get(handle(), 0);
return null /* Unsupported parameter type */;
}
/**
* Write a value in the field {@code enumerate}.
*
* @param enumerate The new value for the field {@code enumerate}
* @param _arena to control the memory allocation scope
*/
public void writeEnumerate(DBusSubtreeEnumerateFunc enumerate, Arena _arena) {
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("enumerate"))
.set(handle(), 0, (enumerate == null ? MemorySegment.NULL : enumerate.toCallback(_arena)));
}
/**
* Read the value of the field {@code introspect}.
*
* @return The value of the field {@code introspect}
*/
public DBusSubtreeIntrospectFunc readIntrospect() {
Arena _arena = Arena.ofAuto();
var _result = (MemorySegment) getMemoryLayout()
.varHandle(MemoryLayout.PathElement.groupElement("introspect")).get(handle(), 0);
return null /* Unsupported parameter type */;
}
/**
* Write a value in the field {@code introspect}.
*
* @param introspect The new value for the field {@code introspect}
* @param _arena to control the memory allocation scope
*/
public void writeIntrospect(DBusSubtreeIntrospectFunc introspect, Arena _arena) {
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("introspect"))
.set(handle(), 0, (introspect == null ? MemorySegment.NULL : introspect.toCallback(_arena)));
}
/**
* Read the value of the field {@code dispatch}.
*
* @return The value of the field {@code dispatch}
*/
public DBusSubtreeDispatchFunc readDispatch() {
Arena _arena = Arena.ofAuto();
var _result = (MemorySegment) getMemoryLayout()
.varHandle(MemoryLayout.PathElement.groupElement("dispatch")).get(handle(), 0);
return null /* Unsupported parameter type */;
}
/**
* Write a value in the field {@code dispatch}.
*
* @param dispatch The new value for the field {@code dispatch}
* @param _arena to control the memory allocation scope
*/
public void writeDispatch(DBusSubtreeDispatchFunc dispatch, Arena _arena) {
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("dispatch"))
.set(handle(), 0, (dispatch == null ? MemorySegment.NULL : dispatch.toCallback(_arena)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy