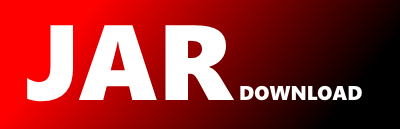
org.gnome.gio.PropertyAction Maven / Gradle / Ivy
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gio;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.gobject.types.Types;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.String;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.glib.Type;
import org.gnome.gobject.GObject;
import org.gnome.gobject.Value;
/**
* A {@code GPropertyAction} is a way to get a {@link Action} with a state value
* reflecting and controlling the value of a {@link org.gnome.gobject.GObject} property.
*
* The state of the action will correspond to the value of the property.
* Changing it will change the property (assuming the requested value
* matches the requirements as specified in the {@code GObject.ParamSpec}).
*
* Only the most common types are presently supported. Booleans are
* mapped to booleans, strings to strings, signed/unsigned integers to
* int32/uint32 and floats and doubles to doubles.
*
* If the property is an enum then the state will be string-typed and
* conversion will automatically be performed between the enum value and
* ‘nick’ string as per the {@code GObject.EnumValue} table.
*
* Flags types are not currently supported.
*
* Properties of object types, boxed types and pointer types are not
* supported and probably never will be.
*
* Properties of {@code GLib.Variant} types are not currently supported.
*
* If the property is boolean-valued then the action will have a {@code NULL}
* parameter type, and activating the action (with no parameter) will
* toggle the value of the property.
*
* In all other cases, the parameter type will correspond to the type of
* the property.
*
* The general idea here is to reduce the number of locations where a
* particular piece of state is kept (and therefore has to be synchronised
* between). {@code GPropertyAction} does not have a separate state that is kept
* in sync with the property value — its state is the property value.
*
* For example, it might be useful to create a {@link Action} corresponding
* to the {@code visible-child-name} property of a {@code GtkStack}
* so that the current page can be switched from a menu. The active radio
* indication in the menu is then directly determined from the active page of
* the {@code GtkStack}.
*
* An anti-example would be binding the {@code active-id} property on a
* {@code GtkComboBox}. This is
* because the state of the combo box itself is probably uninteresting and is
* actually being used to control something else.
*
* Another anti-example would be to bind to the {@code visible-child-name}
* property of a {@code GtkStack} if
* this value is actually stored in {@link Settings}. In that case, the
* real source of the value is* {@link Settings}. If you want
* a {@link Action} to control a setting stored in {@link Settings},
* see {@link Settings#createAction} instead, and possibly combine its
* use with {@link Settings#bind}.
*
* @version 2.38
*/
@Generated("io.github.jwharm.JavaGI")
public class PropertyAction extends GObject implements Action {
static {
Gio.javagi$ensureInitialized();
}
/**
* Create a PropertyAction proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public PropertyAction(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Creates a {@code GAction} corresponding to the value of property
* {@code propertyName} on {@code object}.
*
* The property must be existent and readable and writable (and not
* construct-only).
*
* This function takes a reference on {@code object} and doesn't release it
* until the action is destroyed.
*
* @param name the name of the action to create
* @param object the object that has the property
* to wrap
* @param propertyName the name of the property
*/
public PropertyAction(String name, GObject object, String propertyName) {
this(constructNew(name, object, propertyName));
InstanceCache.put(handle(), this);
}
/**
* Get the GType of the PropertyAction class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("g_property_action_get_type");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected PropertyAction asParent() {
PropertyAction _parent = new PropertyAction(handle());
_parent.callParent(true);
return _parent;
}
private static MemorySegment constructNew(String name, GObject object, String propertyName) {
try (var _arena = Arena.ofConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_property_action_new.invokeExact(
(MemorySegment) (name == null ? MemorySegment.NULL : Interop.allocateNativeString(name, _arena)),
(MemorySegment) (object == null ? MemorySegment.NULL : object.handle()),
(MemorySegment) (propertyName == null ? MemorySegment.NULL : Interop.allocateNativeString(propertyName, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
}
/**
* A {@link Builder} object constructs a {@code PropertyAction}
* with the specified properties.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
/**
* Inner class implementing a builder pattern to construct a GObject with
* properties.
*
* @param the type of the Builder that is returned
*/
public static class Builder> extends GObject.Builder implements Action.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code PropertyAction} object. This will call
* {@link GObject#withProperties} to create a new GObject instance,
* which is then cast to {@code PropertyAction}.
*
* @return a new instance of {@code PropertyAction} with the properties
* that were set in the Builder object.
*/
public PropertyAction build() {
try {
var _instance = (PropertyAction) GObject.withProperties(PropertyAction.getType(), getNames(), getValues());
connectSignals(_instance.handle());
return _instance;
} finally {
for (Value _value : getValues()) _value.unset();
getArena().close();
}
}
/**
* If {@code true}, the state of the action will be the negation of the
* property value, provided the property is boolean.
*
* @param invertBoolean the value for the {@code invert-boolean} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setInvertBoolean(boolean invertBoolean) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.BOOLEAN);
_value.setBoolean(invertBoolean);
addBuilderProperty("invert-boolean", _value);
return (B) this;
}
/**
* The name of the action. This is mostly meaningful for identifying
* the action once it has been added to a {@code GActionMap}.
*
* @param name the value for the {@code name} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setName(String name) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(name);
addBuilderProperty("name", _value);
return (B) this;
}
/**
* The object to wrap a property on.
*
* The object must be a non-{@code null} {@code GObject} with properties.
*
* @param object the value for the {@code object} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setObject(GObject object) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.OBJECT);
_value.setObject((GObject) object);
addBuilderProperty("object", _value);
return (B) this;
}
/**
* The name of the property to wrap on the object.
*
* The property must exist on the passed-in object and it must be
* readable and writable (and not construct-only).
*
* @param propertyName the value for the {@code property-name} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setPropertyName(String propertyName) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(propertyName);
addBuilderProperty("property-name", _value);
return (B) this;
}
}
private static final class MethodHandles {
static final MethodHandle g_property_action_new = Interop.downcallHandle(
"g_property_action_new", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
}
}