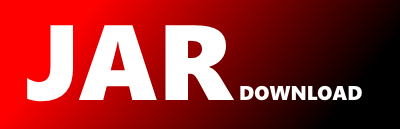
org.gnome.gio.SettingsSchema Maven / Gradle / Ivy
Show all versions of gio Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gio;
import io.github.jwharm.javagi.base.ProxyInstance;
import io.github.jwharm.javagi.interop.Interop;
import io.github.jwharm.javagi.interop.MemoryCleaner;
import java.lang.String;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.glib.Type;
/**
* The {@code Gio.SettingsSchemaSource} and {@code GSettingsSchema} APIs provide a
* mechanism for advanced control over the loading of schemas and a
* mechanism for introspecting their content.
*
* Plugin loading systems that wish to provide plugins a way to access
* settings face the problem of how to make the schemas for these
* settings visible to GSettings. Typically, a plugin will want to ship
* the schema along with itself and it won't be installed into the
* standard system directories for schemas.
*
* {@code Gio.SettingsSchemaSource} provides a mechanism for dealing with this
* by allowing the creation of a new ‘schema source’ from which schemas can
* be acquired. This schema source can then become part of the metadata
* associated with the plugin and queried whenever the plugin requires
* access to some settings.
*
* Consider the following example:
*
{@code typedef struct
* {
* …
* GSettingsSchemaSource *schema_source;
* …
* } Plugin;
*
* Plugin *
* initialise_plugin (const gchar *dir)
* {
* Plugin *plugin;
*
* …
*
* plugin->schema_source =
* g_settings_schema_source_new_from_directory (dir,
* g_settings_schema_source_get_default (), FALSE, NULL);
*
* …
*
* return plugin;
* }
*
* …
*
* GSettings *
* plugin_get_settings (Plugin *plugin,
* const gchar *schema_id)
* {
* GSettingsSchema *schema;
*
* if (schema_id == NULL)
* schema_id = plugin->identifier;
*
* schema = g_settings_schema_source_lookup (plugin->schema_source,
* schema_id, FALSE);
*
* if (schema == NULL)
* {
* … disable the plugin or abort, etc …
* }
*
* return g_settings_new_full (schema, NULL, NULL);
* }
* }
*
* The code above shows how hooks should be added to the code that
* initialises (or enables) the plugin to create the schema source and
* how an API can be added to the plugin system to provide a convenient
* way for the plugin to access its settings, using the schemas that it
* ships.
*
* From the standpoint of the plugin, it would need to ensure that it
* ships a gschemas.compiled file as part of itself, and then simply do
* the following:
*
{@code {
* GSettings *settings;
* gint some_value;
*
* settings = plugin_get_settings (self, NULL);
* some_value = g_settings_get_int (settings, "some-value");
* …
* }
* }
*
* It's also possible that the plugin system expects the schema source
* files (ie: {@code .gschema.xml} files) instead of a {@code gschemas.compiled} file.
* In that case, the plugin loading system must compile the schemas for
* itself before attempting to create the settings source.
*
* @version 2.32
*/
@Generated("io.github.jwharm.JavaGI")
public class SettingsSchema extends ProxyInstance {
static {
Gio.javagi$ensureInitialized();
}
/**
* Create a SettingsSchema proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public SettingsSchema(MemorySegment address) {
super(address);
}
/**
* Get the GType of the SettingsSchema class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("g_settings_schema_get_type");
}
/**
* Get the ID of this SettingsSchema.
*
* @return the ID
*/
public String getId() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_settings_schema_get_id.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Gets the key named {@code name} from this SettingsSchema.
*
* It is a programmer error to request a key that does not exist. See
* g_settings_schema_list_keys().
*
* @param name the name of a key
* @return the {@code GSettingsSchemaKey} for {@code name}
*/
public SettingsSchemaKey getKey(String name) {
try (var _arena = Arena.ofConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_settings_schema_get_key.invokeExact(
handle(),
(MemorySegment) (name == null ? MemorySegment.NULL : Interop.allocateNativeString(name, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _instance = MemorySegment.NULL.equals(_result) ? null : new SettingsSchemaKey(_result);
if (_instance != null) {
MemoryCleaner.takeOwnership(_instance);
MemoryCleaner.setBoxedType(_instance, SettingsSchemaKey.getType());
}
return _instance;
}
}
/**
* Gets the path associated with this SettingsSchema, or {@code null}.
*
* Schemas may be single-instance or relocatable. Single-instance
* schemas correspond to exactly one set of keys in the backend
* database: those located at the path returned by this function.
*
* Relocatable schemas can be referenced by other schemas and can
* therefore describe multiple sets of keys at different locations. For
* relocatable schemas, this function will return {@code null}.
*
* @return the path of the schema, or {@code null}
*/
public String getPath() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_settings_schema_get_path.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
/**
* Checks if this SettingsSchema has a key named {@code name}.
*
* @param name the name of a key
* @return {@code true} if such a key exists
*/
public boolean hasKey(String name) {
try (var _arena = Arena.ofConfined()) {
int _result;
try {
_result = (int) MethodHandles.g_settings_schema_has_key.invokeExact(handle(),
(MemorySegment) (name == null ? MemorySegment.NULL : Interop.allocateNativeString(name, _arena)));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result != 0;
}
}
/**
* Gets the list of children in this SettingsSchema.
*
* You should free the return value with g_strfreev() when you are done
* with it.
*
* @return a list of
* the children on {@code settings}, in no defined order
*/
public String[] listChildren() {
try (var _arena = Arena.ofConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_settings_schema_list_children.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringArrayFrom(_result, true);
}
}
/**
* Introspects the list of keys on this SettingsSchema.
*
* You should probably not be calling this function from "normal" code
* (since you should already know what keys are in your schema). This
* function is intended for introspection reasons.
*
* @return a list
* of the keys on this SettingsSchema, in no defined order
*/
public String[] listKeys() {
try (var _arena = Arena.ofConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_settings_schema_list_keys.invokeExact(
handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringArrayFrom(_result, true);
}
}
/**
* Increase the reference count of this SettingsSchema, returning a new reference.
*
* @return a new reference to this SettingsSchema
*/
public SettingsSchema ref() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_settings_schema_ref.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _instance = MemorySegment.NULL.equals(_result) ? null : new SettingsSchema(_result);
if (_instance != null) {
MemoryCleaner.takeOwnership(_instance);
MemoryCleaner.setBoxedType(_instance, SettingsSchema.getType());
}
return _instance;
}
/**
* Decrease the reference count of this SettingsSchema, possibly freeing it.
*/
public void unref() {
try {
MethodHandles.g_settings_schema_unref.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
private static final class MethodHandles {
static final MethodHandle g_settings_schema_get_id = Interop.downcallHandle(
"g_settings_schema_get_id", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle g_settings_schema_get_key = Interop.downcallHandle(
"g_settings_schema_get_key", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle g_settings_schema_get_path = Interop.downcallHandle(
"g_settings_schema_get_path", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle g_settings_schema_has_key = Interop.downcallHandle(
"g_settings_schema_has_key", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle g_settings_schema_list_children = Interop.downcallHandle(
"g_settings_schema_list_children", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle g_settings_schema_list_keys = Interop.downcallHandle(
"g_settings_schema_list_keys", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle g_settings_schema_ref = Interop.downcallHandle(
"g_settings_schema_ref", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle g_settings_schema_unref = Interop.downcallHandle(
"g_settings_schema_unref", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS), false);
}
}