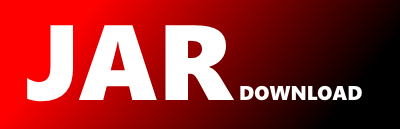
org.gnome.gio.SimpleAction Maven / Gradle / Ivy
Show all versions of gio Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gio;
import io.github.jwharm.javagi.base.FunctionPointer;
import io.github.jwharm.javagi.base.GLibLogger;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.gobject.SignalConnection;
import io.github.jwharm.javagi.gobject.types.Signals;
import io.github.jwharm.javagi.gobject.types.Types;
import io.github.jwharm.javagi.interop.Arenas;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.FunctionalInterface;
import java.lang.String;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.Linker;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.glib.Type;
import org.gnome.glib.Variant;
import org.gnome.glib.VariantType;
import org.gnome.gobject.GObject;
import org.gnome.gobject.Value;
import org.jetbrains.annotations.Nullable;
/**
* A {@code GSimpleAction} is the obvious simple implementation of the
* {@link Action} interface. This is the easiest way to create an action for
* purposes of adding it to a {@link SimpleActionGroup}.
*/
@Generated("io.github.jwharm.JavaGI")
public class SimpleAction extends GObject implements Action {
static {
Gio.javagi$ensureInitialized();
}
/**
* Create a SimpleAction proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public SimpleAction(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Creates a new action.
*
* The created action is stateless. See g_simple_action_new_stateful() to create
* an action that has state.
*
* @param name the name of the action
* @param parameterType the type of parameter that will be passed to
* handlers for the {@code GSimpleAction}::activate signal, or {@code null} for no parameter
*/
public SimpleAction(String name, @Nullable VariantType parameterType) {
this(constructNew(name, parameterType));
InstanceCache.put(handle(), this);
}
/**
* Get the GType of the SimpleAction class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("g_simple_action_get_type");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected SimpleAction asParent() {
SimpleAction _parent = new SimpleAction(handle());
_parent.callParent(true);
return _parent;
}
private static MemorySegment constructNew(String name, @Nullable VariantType parameterType) {
try (var _arena = Arena.ofConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_simple_action_new.invokeExact(
(MemorySegment) (name == null ? MemorySegment.NULL : Interop.allocateNativeString(name, _arena)),
(MemorySegment) (parameterType == null ? MemorySegment.NULL : parameterType.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
}
/**
* Creates a new stateful action.
*
* All future state values must have the same {@code GVariantType} as the initial
* {@code state}.
*
* If the {@code state} {@code GVariant} is floating, it is consumed.
*
* @param name the name of the action
* @param parameterType the type of the parameter that will be passed to
* handlers for the {@code GSimpleAction}::activate signal, or {@code null} for no parameter
* @param state the initial state of the action
* @return a new {@code GSimpleAction}
*/
public static SimpleAction stateful(String name, @Nullable VariantType parameterType,
Variant state) {
var _result = constructStateful(name, parameterType, state);
var _object = (SimpleAction) InstanceCache.getForType(_result, SimpleAction::new, true);
if (_object instanceof GObject _gobject) {
GLibLogger.debug("Ref org.gnome.gio.SimpleAction %ld", _gobject.handle().address());
_gobject.ref();
}
return (SimpleAction) _object;
}
private static MemorySegment constructStateful(String name, @Nullable VariantType parameterType,
Variant state) {
try (var _arena = Arena.ofConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_simple_action_new_stateful.invokeExact(
(MemorySegment) (name == null ? MemorySegment.NULL : Interop.allocateNativeString(name, _arena)),
(MemorySegment) (parameterType == null ? MemorySegment.NULL : parameterType.handle()),
(MemorySegment) (state == null ? MemorySegment.NULL : state.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
}
/**
* Sets the action as enabled or not.
*
* An action must be enabled in order to be activated or in order to
* have its state changed from outside callers.
*
* This should only be called by the implementor of the action. Users
* of the action should not attempt to modify its enabled flag.
*
* @param enabled whether the action is enabled
*/
public void setEnabled(boolean enabled) {
try {
MethodHandles.g_simple_action_set_enabled.invokeExact(handle(), enabled ? 1 : 0);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the state of the action.
*
* This directly updates the 'state' property to the given value.
*
* This should only be called by the implementor of the action. Users
* of the action should not attempt to directly modify the 'state'
* property. Instead, they should call g_action_change_state() to
* request the change.
*
* If the {@code value} GVariant is floating, it is consumed.
*
* @param value the new {@code GVariant} for the state
*/
public void setState(Variant value) {
try {
MethodHandles.g_simple_action_set_state.invokeExact(handle(),
(MemorySegment) (value == null ? MemorySegment.NULL : value.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Sets the state hint for the action.
*
* See g_action_get_state_hint() for more information about
* action state hints.
*
* @param stateHint a {@code GVariant} representing the state hint
*/
public void setStateHint(@Nullable Variant stateHint) {
try {
MethodHandles.g_simple_action_set_state_hint.invokeExact(handle(),
(MemorySegment) (stateHint == null ? MemorySegment.NULL : stateHint.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Indicates that the action was just activated.
*
* {@code parameter} will always be of the expected type, i.e. the parameter type
* specified when the action was created. If an incorrect type is given when
* activating the action, this signal is not emitted.
*
* Since GLib 2.40, if no handler is connected to this signal then the
* default behaviour for boolean-stated actions with a {@code null} parameter
* type is to toggle them via the {@code GSimpleAction}::change-state signal.
* For stateful actions where the state type is equal to the parameter
* type, the default is to forward them directly to
* {@code GSimpleAction}::change-state. This should allow almost all users
* of {@code GSimpleAction} to connect only one handler or the other.
*
* @param handler the signal handler
* @return a signal handler ID to keep track of the signal connection
* @see ActivateCallback#run
*/
public SignalConnection onActivate(ActivateCallback handler) {
try (Arena _arena = Arena.ofConfined()) {
try {
var _name = Interop.allocateNativeString("activate", _arena);
var _callbackArena = Arena.ofShared();
var _result = (int) (long) Signals.g_signal_connect_data.invokeExact(handle(),
_name, handler.toCallback(_callbackArena),
Arenas.cacheArena(_callbackArena), Arenas.CLOSE_CB_SYM, 0);
return new SignalConnection<>(handle(), _result);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Emits the "activate" signal. See {@link #onActivate}.
*/
public void emitActivate(@Nullable Variant parameter) {
try (Arena _arena = Arena.ofConfined()) {
MemorySegment _name = Interop.allocateNativeString("activate", _arena);
Object[] _args = new Object[] {
(MemorySegment) (parameter == null ? MemorySegment.NULL : parameter.handle())};
Signals.g_signal_emit_by_name.invokeExact(handle(), _name, _args);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Indicates that the action just received a request to change its
* state.
*
* {@code value} will always be of the correct state type, i.e. the type of the
* initial state passed to g_simple_action_new_stateful(). If an incorrect
* type is given when requesting to change the state, this signal is not
* emitted.
*
* If no handler is connected to this signal then the default
* behaviour is to call g_simple_action_set_state() to set the state
* to the requested value. If you connect a signal handler then no
* default action is taken. If the state should change then you must
* call g_simple_action_set_state() from the handler.
*
* An example of a 'change-state' handler:
*
{@code static void
* change_volume_state (GSimpleAction *action,
* GVariant *value,
* gpointer user_data)
* {
* gint requested;
*
* requested = g_variant_get_int32 (value);
*
* // Volume only goes from 0 to 10
* if (0 <= requested && requested <= 10)
* g_simple_action_set_state (action, value);
* }
* }
*
* The handler need not set the state to the requested value.
* It could set it to any value at all, or take some other action.
*
* @param handler the signal handler
* @return a signal handler ID to keep track of the signal connection
* @see ChangeStateCallback#run
*/
public SignalConnection onChangeState(ChangeStateCallback handler) {
try (Arena _arena = Arena.ofConfined()) {
try {
var _name = Interop.allocateNativeString("change-state", _arena);
var _callbackArena = Arena.ofShared();
var _result = (int) (long) Signals.g_signal_connect_data.invokeExact(handle(),
_name, handler.toCallback(_callbackArena),
Arenas.cacheArena(_callbackArena), Arenas.CLOSE_CB_SYM, 0);
return new SignalConnection<>(handle(), _result);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Emits the "change-state" signal. See {@link #onChangeState}.
*/
public void emitChangeState(@Nullable Variant value) {
try (Arena _arena = Arena.ofConfined()) {
MemorySegment _name = Interop.allocateNativeString("change-state", _arena);
Object[] _args = new Object[] {
(MemorySegment) (value == null ? MemorySegment.NULL : value.handle())};
Signals.g_signal_emit_by_name.invokeExact(handle(), _name, _args);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* A {@link Builder} object constructs a {@code SimpleAction}
* with the specified properties.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
/**
* Functional interface declaration of the {@code ActivateCallback} callback.
*
* @see ActivateCallback#run
*/
@FunctionalInterface
public interface ActivateCallback extends FunctionPointer {
/**
* Indicates that the action was just activated.
*
* {@code parameter} will always be of the expected type, i.e. the parameter type
* specified when the action was created. If an incorrect type is given when
* activating the action, this signal is not emitted.
*
* Since GLib 2.40, if no handler is connected to this signal then the
* default behaviour for boolean-stated actions with a {@code null} parameter
* type is to toggle them via the {@code GSimpleAction}::change-state signal.
* For stateful actions where the state type is equal to the parameter
* type, the default is to forward them directly to
* {@code GSimpleAction}::change-state. This should allow almost all users
* of {@code GSimpleAction} to connect only one handler or the other.
*/
void run(@Nullable Variant parameter);
/**
* The {@code upcall} method is called from native code. The parameters
* are marshaled and {@link #run} is executed.
*/
default void upcall(MemorySegment sourceSimpleAction, MemorySegment parameter) {
run(MemorySegment.NULL.equals(parameter) ? null : new Variant(parameter));
}
/**
* Creates a native function pointer to the {@link #upcall} method.
*
* @return the native function pointer
*/
default MemorySegment toCallback(Arena arena) {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(java.lang.invoke.MethodHandles.lookup(), ActivateCallback.class, _fdesc);
return Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
}
}
/**
* Functional interface declaration of the {@code ChangeStateCallback} callback.
*
* @see ChangeStateCallback#run
*/
@FunctionalInterface
public interface ChangeStateCallback extends FunctionPointer {
/**
* Indicates that the action just received a request to change its
* state.
*
* {@code value} will always be of the correct state type, i.e. the type of the
* initial state passed to g_simple_action_new_stateful(). If an incorrect
* type is given when requesting to change the state, this signal is not
* emitted.
*
* If no handler is connected to this signal then the default
* behaviour is to call g_simple_action_set_state() to set the state
* to the requested value. If you connect a signal handler then no
* default action is taken. If the state should change then you must
* call g_simple_action_set_state() from the handler.
*
* An example of a 'change-state' handler:
*
{@code static void
* change_volume_state (GSimpleAction *action,
* GVariant *value,
* gpointer user_data)
* {
* gint requested;
*
* requested = g_variant_get_int32 (value);
*
* // Volume only goes from 0 to 10
* if (0 <= requested && requested <= 10)
* g_simple_action_set_state (action, value);
* }
* }
*
* The handler need not set the state to the requested value.
* It could set it to any value at all, or take some other action.
*/
void run(@Nullable Variant value);
/**
* The {@code upcall} method is called from native code. The parameters
* are marshaled and {@link #run} is executed.
*/
default void upcall(MemorySegment sourceSimpleAction, MemorySegment value) {
run(MemorySegment.NULL.equals(value) ? null : new Variant(value));
}
/**
* Creates a native function pointer to the {@link #upcall} method.
*
* @return the native function pointer
*/
default MemorySegment toCallback(Arena arena) {
FunctionDescriptor _fdesc = FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS);
MethodHandle _handle = Interop.upcallHandle(java.lang.invoke.MethodHandles.lookup(), ChangeStateCallback.class, _fdesc);
return Linker.nativeLinker().upcallStub(_handle.bindTo(this), _fdesc, arena);
}
}
/**
* Inner class implementing a builder pattern to construct a GObject with
* properties.
*
* @param the type of the Builder that is returned
*/
public static class Builder> extends GObject.Builder implements Action.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code SimpleAction} object. This will call
* {@link GObject#withProperties} to create a new GObject instance,
* which is then cast to {@code SimpleAction}.
*
* @return a new instance of {@code SimpleAction} with the properties
* that were set in the Builder object.
*/
public SimpleAction build() {
try {
var _instance = (SimpleAction) GObject.withProperties(SimpleAction.getType(), getNames(), getValues());
connectSignals(_instance.handle());
return _instance;
} finally {
for (Value _value : getValues()) _value.unset();
getArena().close();
}
}
/**
* If {@code action} is currently enabled.
*
* If the action is disabled then calls to g_action_activate() and
* g_action_change_state() have no effect.
*
* @param enabled the value for the {@code enabled} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setEnabled(boolean enabled) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.BOOLEAN);
_value.setBoolean(enabled);
addBuilderProperty("enabled", _value);
return (B) this;
}
/**
* The name of the action. This is mostly meaningful for identifying
* the action once it has been added to a {@code GSimpleActionGroup}.
*
* @param name the value for the {@code name} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setName(String name) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.STRING);
_value.setString(name);
addBuilderProperty("name", _value);
return (B) this;
}
/**
* The type of the parameter that must be given when activating the
* action.
*
* @param parameterType the value for the {@code parameter-type} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setParameterType(VariantType parameterType) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(VariantType.getType());
_value.setBoxed(parameterType.handle());
addBuilderProperty("parameter-type", _value);
return (B) this;
}
/**
* The state of the action, or {@code null} if the action is stateless.
*
* @param state the value for the {@code state} property
* @return the {@code Builder} instance is returned, to allow method chaining
*/
public B setState(Variant state) {
Arena _arena = getArena();
Value _value = new Value(_arena);
_value.init(Types.VARIANT);
_value.setVariant(state);
addBuilderProperty("state", _value);
return (B) this;
}
/**
* Indicates that the action was just activated.
*
* {@code parameter} will always be of the expected type, i.e. the parameter type
* specified when the action was created. If an incorrect type is given when
* activating the action, this signal is not emitted.
*
* Since GLib 2.40, if no handler is connected to this signal then the
* default behaviour for boolean-stated actions with a {@code null} parameter
* type is to toggle them via the {@code GSimpleAction}::change-state signal.
* For stateful actions where the state type is equal to the parameter
* type, the default is to forward them directly to
* {@code GSimpleAction}::change-state. This should allow almost all users
* of {@code GSimpleAction} to connect only one handler or the other.
*
* @param handler the signal handler
* @return the {@code Builder} instance is returned, to allow method chaining
* @see ActivateCallback#run
*/
public B onActivate(ActivateCallback handler) {
connect("activate", handler);
return (B) this;
}
/**
* Indicates that the action just received a request to change its
* state.
*
* {@code value} will always be of the correct state type, i.e. the type of the
* initial state passed to g_simple_action_new_stateful(). If an incorrect
* type is given when requesting to change the state, this signal is not
* emitted.
*
* If no handler is connected to this signal then the default
* behaviour is to call g_simple_action_set_state() to set the state
* to the requested value. If you connect a signal handler then no
* default action is taken. If the state should change then you must
* call g_simple_action_set_state() from the handler.
*
* An example of a 'change-state' handler:
*
{@code static void
* change_volume_state (GSimpleAction *action,
* GVariant *value,
* gpointer user_data)
* {
* gint requested;
*
* requested = g_variant_get_int32 (value);
*
* // Volume only goes from 0 to 10
* if (0 <= requested && requested <= 10)
* g_simple_action_set_state (action, value);
* }
* }
*
* The handler need not set the state to the requested value.
* It could set it to any value at all, or take some other action.
*
* @param handler the signal handler
* @return the {@code Builder} instance is returned, to allow method chaining
* @see ChangeStateCallback#run
*/
public B onChangeState(ChangeStateCallback handler) {
connect("change-state", handler);
return (B) this;
}
}
private static final class MethodHandles {
static final MethodHandle g_simple_action_new = Interop.downcallHandle(
"g_simple_action_new", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle g_simple_action_new_stateful = Interop.downcallHandle(
"g_simple_action_new_stateful", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle g_simple_action_set_enabled = Interop.downcallHandle(
"g_simple_action_set_enabled", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
static final MethodHandle g_simple_action_set_state = Interop.downcallHandle(
"g_simple_action_set_state", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle g_simple_action_set_state_hint = Interop.downcallHandle(
"g_simple_action_set_state_hint", FunctionDescriptor.ofVoid(ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
}
}