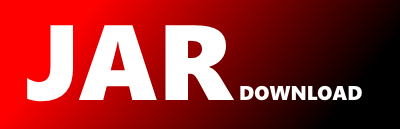
org.gnome.gio.UnixConnection Maven / Gradle / Ivy
Show all versions of gio Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gio;
import io.github.jwharm.javagi.base.GErrorException;
import io.github.jwharm.javagi.gobject.InstanceCache;
import io.github.jwharm.javagi.interop.ArenaCloseAction;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.glib.Type;
import org.gnome.gobject.GObject;
import org.gnome.gobject.Value;
import org.jetbrains.annotations.Nullable;
/**
* This is the subclass of {@link SocketConnection} that is created
* for UNIX domain sockets.
*
* It contains functions to do some of the UNIX socket specific
* functionality like passing file descriptors.
*
* Since GLib 2.72, {@code GUnixConnection} is available on all platforms. It requires
* underlying system support (such as Windows 10 with {@code AF_UNIX}) at run time.
*
* Before GLib 2.72, {@code } belonged to the UNIX-specific GIO
* interfaces, thus you had to use the {@code gio-unix-2.0.pc} pkg-config file when
* using it. This is no longer necessary since GLib 2.72.
*
* @version 2.22
*/
@Generated("io.github.jwharm.JavaGI")
public class UnixConnection extends SocketConnection {
static {
Gio.javagi$ensureInitialized();
}
/**
* Create a UnixConnection proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public UnixConnection(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Get the GType of the UnixConnection class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("g_unix_connection_get_type");
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
SocketConnection.getMemoryLayout().withName("parent_instance"),
ValueLayout.ADDRESS.withName("priv")
).withName("GUnixConnection");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected UnixConnection asParent() {
UnixConnection _parent = new UnixConnection(handle());
_parent.callParent(true);
return _parent;
}
/**
* Receives credentials from the sending end of the connection. The
* sending end has to call g_unix_connection_send_credentials() (or
* similar) for this to work.
*
* As well as reading the credentials this also reads (and discards) a
* single byte from the stream, as this is required for credentials
* passing to work on some implementations.
*
* This method can be expected to be available on the following platforms:
*
* - Linux since GLib 2.26
*
- FreeBSD since GLib 2.26
*
- GNU/kFreeBSD since GLib 2.36
*
- Solaris, Illumos and OpenSolaris since GLib 2.40
*
- GNU/Hurd since GLib 2.40
*
*
* Other ways to exchange credentials with a foreign peer includes the
* {@code GUnixCredentialsMessage} type and g_socket_get_credentials() function.
*
* @param cancellable A {@code GCancellable} or {@code null}.
* @return Received credentials on success (free with
* g_object_unref()), {@code null} if {@code error} is set.
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public Credentials receiveCredentials(@Nullable Cancellable cancellable) throws
GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_unix_connection_receive_credentials.invokeExact(
handle(),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return (Credentials) InstanceCache.getForType(_result, Credentials::new, true);
}
}
/**
* Asynchronously receive credentials.
*
* For more details, see g_unix_connection_receive_credentials() which is
* the synchronous version of this call.
*
* When the operation is finished, {@code callback} will be called. You can then call
* g_unix_connection_receive_credentials_finish() to get the result of the operation.
*
* @param cancellable optional {@code GCancellable} object, {@code null} to ignore.
* @param callback a {@code GAsyncReadyCallback}
* to call when the request is satisfied
*/
public void receiveCredentialsAsync(@Nullable Cancellable cancellable,
@Nullable AsyncReadyCallback callback) {
try (var _arena = Arena.ofConfined()) {
final Arena _callbackScope = Arena.ofConfined();
if (callback != null) ArenaCloseAction.CLEANER.register(callback, new ArenaCloseAction(_callbackScope));
try {
MethodHandles.g_unix_connection_receive_credentials_async.invokeExact(handle(),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()),
(MemorySegment) (callback == null ? MemorySegment.NULL : callback.toCallback(_callbackScope)),
MemorySegment.NULL);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Finishes an asynchronous receive credentials operation started with
* g_unix_connection_receive_credentials_async().
*
* @param result a {@code GAsyncResult}.
* @return a {@code GCredentials}, or {@code null} on error.
* Free the returned object with g_object_unref().
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public Credentials receiveCredentialsFinish(AsyncResult result) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_unix_connection_receive_credentials_finish.invokeExact(
handle(),
(MemorySegment) (result == null ? MemorySegment.NULL : result.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return (Credentials) InstanceCache.getForType(_result, Credentials::new, true);
}
}
/**
* Receives a file descriptor from the sending end of the connection.
* The sending end has to call g_unix_connection_send_fd() for this
* to work.
*
* As well as reading the fd this also reads a single byte from the
* stream, as this is required for fd passing to work on some
* implementations.
*
* @param cancellable optional {@code GCancellable} object, {@code null} to ignore
* @return a file descriptor on success, -1 on error.
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public int receiveFd(@Nullable Cancellable cancellable) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
int _result;
try {
_result = (int) MethodHandles.g_unix_connection_receive_fd.invokeExact(handle(),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return _result;
}
}
/**
* Passes the credentials of the current user the receiving side
* of the connection. The receiving end has to call
* g_unix_connection_receive_credentials() (or similar) to accept the
* credentials.
*
* As well as sending the credentials this also writes a single NUL
* byte to the stream, as this is required for credentials passing to
* work on some implementations.
*
* This method can be expected to be available on the following platforms:
*
* - Linux since GLib 2.26
*
- FreeBSD since GLib 2.26
*
- GNU/kFreeBSD since GLib 2.36
*
- Solaris, Illumos and OpenSolaris since GLib 2.40
*
- GNU/Hurd since GLib 2.40
*
*
* Other ways to exchange credentials with a foreign peer includes the
* {@code GUnixCredentialsMessage} type and g_socket_get_credentials() function.
*
* @param cancellable A {@code GCancellable} or {@code null}.
* @return {@code true} on success, {@code false} if {@code error} is set.
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public boolean sendCredentials(@Nullable Cancellable cancellable) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
int _result;
try {
_result = (int) MethodHandles.g_unix_connection_send_credentials.invokeExact(
handle(),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return _result != 0;
}
}
/**
* Asynchronously send credentials.
*
* For more details, see g_unix_connection_send_credentials() which is
* the synchronous version of this call.
*
* When the operation is finished, {@code callback} will be called. You can then call
* g_unix_connection_send_credentials_finish() to get the result of the operation.
*
* @param cancellable optional {@code GCancellable} object, {@code null} to ignore.
* @param callback a {@code GAsyncReadyCallback}
* to call when the request is satisfied
*/
public void sendCredentialsAsync(@Nullable Cancellable cancellable,
@Nullable AsyncReadyCallback callback) {
try (var _arena = Arena.ofConfined()) {
final Arena _callbackScope = Arena.ofConfined();
if (callback != null) ArenaCloseAction.CLEANER.register(callback, new ArenaCloseAction(_callbackScope));
try {
MethodHandles.g_unix_connection_send_credentials_async.invokeExact(handle(),
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()),
(MemorySegment) (callback == null ? MemorySegment.NULL : callback.toCallback(_callbackScope)),
MemorySegment.NULL);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
}
/**
* Finishes an asynchronous send credentials operation started with
* g_unix_connection_send_credentials_async().
*
* @param result a {@code GAsyncResult}.
* @return {@code true} if the operation was successful, otherwise {@code false}.
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public boolean sendCredentialsFinish(AsyncResult result) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
int _result;
try {
_result = (int) MethodHandles.g_unix_connection_send_credentials_finish.invokeExact(
handle(),
(MemorySegment) (result == null ? MemorySegment.NULL : result.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return _result != 0;
}
}
/**
* Passes a file descriptor to the receiving side of the
* connection. The receiving end has to call g_unix_connection_receive_fd()
* to accept the file descriptor.
*
* As well as sending the fd this also writes a single byte to the
* stream, as this is required for fd passing to work on some
* implementations.
*
* @param fd a file descriptor
* @param cancellable optional {@code GCancellable} object, {@code null} to ignore.
* @return a {@code true} on success, {@code null} on error.
* @throws GErrorException see {@link org.gnome.glib.GError}
*/
public boolean sendFd(int fd, @Nullable Cancellable cancellable) throws GErrorException {
try (var _arena = Arena.ofConfined()) {
MemorySegment _gerror = _arena.allocate(ValueLayout.ADDRESS);
int _result;
try {
_result = (int) MethodHandles.g_unix_connection_send_fd.invokeExact(handle(), fd,
(MemorySegment) (cancellable == null ? MemorySegment.NULL : cancellable.handle()), _gerror);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
if (GErrorException.isErrorSet(_gerror)) {
throw new GErrorException(_gerror);
}
return _result != 0;
}
}
/**
* A {@link Builder} object constructs a {@code UnixConnection}
* with the specified properties.
* Use the various {@code set...()} methods to set properties,
* and finish construction with {@link Builder#build()}.
*/
public static Builder extends Builder> builder() {
return new Builder<>();
}
public static class UnixConnectionClass extends SocketConnection.SocketConnectionClass {
/**
* Create a UnixConnectionClass proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public UnixConnectionClass(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Allocate a new UnixConnectionClass.
*
* @param arena to control the memory allocation scope
*/
public UnixConnectionClass(Arena arena) {
super(arena.allocate(getMemoryLayout()));
}
/**
* Allocate a new UnixConnectionClass.
* The memory is allocated with {@link Arena#ofAuto}.
*/
public UnixConnectionClass() {
super(Arena.ofAuto().allocate(getMemoryLayout()));
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
SocketConnection.SocketConnectionClass.getMemoryLayout().withName("parent_class")
).withName("GUnixConnectionClass");
}
}
/**
* Inner class implementing a builder pattern to construct a GObject with
* properties.
*
* @param the type of the Builder that is returned
*/
public static class Builder> extends SocketConnection.Builder {
/**
* Default constructor for a {@code Builder} object.
*/
protected Builder() {
}
/**
* Finish building the {@code UnixConnection} object. This will call
* {@link GObject#withProperties} to create a new GObject instance,
* which is then cast to {@code UnixConnection}.
*
* @return a new instance of {@code UnixConnection} with the properties
* that were set in the Builder object.
*/
public UnixConnection build() {
try {
var _instance = (UnixConnection) GObject.withProperties(UnixConnection.getType(), getNames(), getValues());
connectSignals(_instance.handle());
return _instance;
} finally {
for (Value _value : getValues()) _value.unset();
getArena().close();
}
}
}
private static final class MethodHandles {
static final MethodHandle g_unix_connection_receive_credentials = Interop.downcallHandle(
"g_unix_connection_receive_credentials", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle g_unix_connection_receive_credentials_async = Interop.downcallHandle(
"g_unix_connection_receive_credentials_async",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle g_unix_connection_receive_credentials_finish = Interop.downcallHandle(
"g_unix_connection_receive_credentials_finish",
FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
static final MethodHandle g_unix_connection_receive_fd = Interop.downcallHandle(
"g_unix_connection_receive_fd", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle g_unix_connection_send_credentials = Interop.downcallHandle(
"g_unix_connection_send_credentials", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle g_unix_connection_send_credentials_async = Interop.downcallHandle(
"g_unix_connection_send_credentials_async",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS, ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle g_unix_connection_send_credentials_finish = Interop.downcallHandle(
"g_unix_connection_send_credentials_finish",
FunctionDescriptor.of(ValueLayout.JAVA_INT, ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle g_unix_connection_send_fd = Interop.downcallHandle(
"g_unix_connection_send_fd", FunctionDescriptor.of(ValueLayout.JAVA_INT,
ValueLayout.ADDRESS, ValueLayout.JAVA_INT, ValueLayout.ADDRESS,
ValueLayout.ADDRESS), false);
}
}