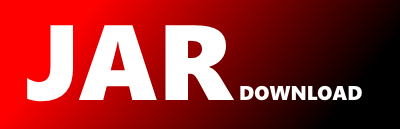
org.gnome.gobject.ClosureNotifyData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gobject Show documentation
Show all versions of gobject Show documentation
Java language bindings for Gobject, generated with Java-GI
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gobject;
import io.github.jwharm.javagi.base.ProxyInstance;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.foreign.Arena;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import javax.annotation.processing.Generated;
@Generated("io.github.jwharm.JavaGI")
public class ClosureNotifyData extends ProxyInstance {
static {
GObjects.javagi$ensureInitialized();
}
/**
* Create a ClosureNotifyData proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public ClosureNotifyData(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Allocate a new ClosureNotifyData.
*
* @param arena to control the memory allocation scope
*/
public ClosureNotifyData(Arena arena) {
super(arena.allocate(getMemoryLayout()));
}
/**
* Allocate a new ClosureNotifyData.
* The memory is allocated with {@link Arena#ofAuto}.
*/
public ClosureNotifyData() {
super(Arena.ofAuto().allocate(getMemoryLayout()));
}
/**
* Allocate a new ClosureNotifyData with the fields set to the provided values.
*
* @param data value for the field {@code data}
* @param notify value for the field {@code notify}
* @param arena to control the memory allocation scope
*/
public ClosureNotifyData(MemorySegment data, ClosureNotify notify, Arena arena) {
this(arena);
writeData(data);
writeNotify(notify, arena);
}
/**
* Allocate a new ClosureNotifyData with the fields set to the provided values.
* The memory is allocated with {@link Arena#ofAuto}.
*
* @param data value for the field {@code data}
* @param notify value for the field {@code notify}
*/
public ClosureNotifyData(MemorySegment data, ClosureNotify notify) {
this(Arena.ofAuto());
writeData(data);
writeNotify(notify, Arena.ofAuto());
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
ValueLayout.ADDRESS.withName("data"),
ValueLayout.ADDRESS.withName("notify")
).withName("GClosureNotifyData");
}
/**
* Read the value of the field {@code data}.
*
* @return The value of the field {@code data}
*/
public MemorySegment readData() {
var _result = (MemorySegment) getMemoryLayout()
.varHandle(MemoryLayout.PathElement.groupElement("data")).get(handle(), 0);
return _result;
}
/**
* Write a value in the field {@code data}.
*
* @param data The new value for the field {@code data}
*/
public void writeData(MemorySegment data) {
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("data"))
.set(handle(), 0, (data == null ? MemorySegment.NULL : data));
}
/**
* Read the value of the field {@code notify}.
*
* @return The value of the field {@code notify}
*/
public ClosureNotify readNotify() {
Arena _arena = Arena.ofAuto();
var _result = (MemorySegment) getMemoryLayout()
.varHandle(MemoryLayout.PathElement.groupElement("notify")).get(handle(), 0);
return null /* Unsupported parameter type */;
}
/**
* Write a value in the field {@code notify}.
*
* @param notify The new value for the field {@code notify}
* @param _arena to control the memory allocation scope
*/
public void writeNotify(ClosureNotify notify, Arena _arena) {
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("notify"))
.set(handle(), 0, (notify == null ? MemorySegment.NULL : notify.toCallback(_arena)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy