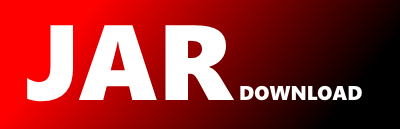
org.gnome.gobject.ParamSpecOverride Maven / Gradle / Ivy
Show all versions of gobject Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gobject;
import io.github.jwharm.javagi.gobject.types.Types;
import io.github.jwharm.javagi.interop.Interop;
import io.github.jwharm.javagi.interop.MemoryCleaner;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import javax.annotation.processing.Generated;
import org.gnome.glib.Type;
/**
* A {@code GParamSpec} derived structure that redirects operations to
* other types of {@code GParamSpec}.
*
* All operations other than getting or setting the value are redirected,
* including accessing the nick and blurb, validating a value, and so
* forth.
*
* See g_param_spec_get_redirect_target() for retrieving the overridden
* property. {@code GParamSpecOverride} is used in implementing
* g_object_class_override_property(), and will not be directly useful
* unless you are implementing a new base type similar to GObject.
*
* @version 2.4
*/
@Generated("io.github.jwharm.JavaGI")
public class ParamSpecOverride extends ParamSpec {
static {
GObjects.javagi$ensureInitialized();
}
/**
* Create a ParamSpecOverride proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public ParamSpecOverride(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
MemoryCleaner.setFreeFunc(this, "g_param_spec_unref");
}
/**
* Get the GType of the GParamSpecOverride class
*
* @return always {@link Types#PARAM}
*/
public static Type getType() {
return Types.PARAM;
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
ParamSpec.getMemoryLayout().withName("parent_instance"),
ValueLayout.ADDRESS.withName("overridden")
).withName("GParamSpecOverride");
}
/**
* Returns this instance as if it were its parent type. This is mostly
* synonymous to the Java {@code super} keyword, but will set the native
* typeclass function pointers to the parent type. When overriding a native
* virtual method in Java, "chaining up" with {@code super.methodName()}
* doesn't work, because it invokes the overridden function pointer again.
* To chain up, call {@code asParent().methodName()}. This will call the
* native function pointer of this virtual method in the typeclass of the
* parent type.
*/
protected ParamSpecOverride asParent() {
ParamSpecOverride _parent = new ParamSpecOverride(handle());
_parent.callParent(true);
return _parent;
}
}