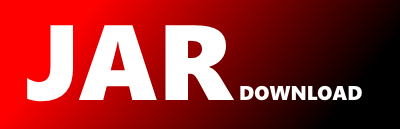
org.gnome.gobject.ValueArray Maven / Gradle / Ivy
Show all versions of gobject Show documentation
// This file was automatically generated by Java-GI. Do not edit this file
// directly! Visit for more information.
//
// The API documentation in this file was derived from GObject-Introspection
// metadata and may include text or comments from the original C sources.
//
// Copyright (c), upstream authors as identified in the GObject-Introspection
// metadata.
//
// This generated file is distributed under the same license as the original
// GObject-Introspection data, unless otherwise specified. Users of this file
// are responsible for complying with any licenses or terms required by the
// original authors.
//
// THIS FILE IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE, AND NONINFRINGEMENT.
//
package org.gnome.gobject;
import io.github.jwharm.javagi.base.ProxyInstance;
import io.github.jwharm.javagi.interop.Interop;
import io.github.jwharm.javagi.interop.MemoryCleaner;
import java.lang.Deprecated;
import java.lang.foreign.Arena;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.glib.CompareDataFunc;
import org.gnome.glib.Type;
import org.jetbrains.annotations.Nullable;
/**
* A {@code GValueArray} is a container structure to hold an array of generic values.
*
* The prime purpose of a {@code GValueArray} is for it to be used as an
* object property that holds an array of values. A {@code GValueArray} wraps
* an array of {@code GValue} elements in order for it to be used as a boxed
* type through {@code G_TYPE_VALUE_ARRAY}.
*
* {@code GValueArray} is deprecated in favour of {@code GArray} since GLib 2.32.
* It is possible to create a {@code GArray} that behaves like a {@code GValueArray}
* by using the size of {@code GValue} as the element size, and by setting
* {@link Value#unset} as the clear function using
* {@link org.gnome.glib.Array#setClearFunc}, for instance, the following code:
*
{@code GValueArray *array = g_value_array_new (10);
* }
*
* can be replaced by:
*
{@code GArray *array = g_array_sized_new (FALSE, TRUE, sizeof (GValue), 10);
* g_array_set_clear_func (array, (GDestroyNotify) g_value_unset);
* }
*/
@Generated("io.github.jwharm.JavaGI")
@Deprecated
public class ValueArray extends ProxyInstance {
static {
GObjects.javagi$ensureInitialized();
}
/**
* Create a ValueArray proxy instance for the provided memory address.
*
* @param address the memory address of the native object
*/
public ValueArray(MemorySegment address) {
super(Interop.reinterpret(address, getMemoryLayout().byteSize()));
}
/**
* Allocate and initialize a new {@code GValueArray}, optionally preserve space
* for {@code nPrealloced} elements. New arrays always contain 0 elements,
* regardless of the value of {@code nPrealloced}.
*
* @param nPrealloced number of values to preallocate space for
* @deprecated Use {@code GArray} and g_array_sized_new() instead.
*/
@Deprecated
public ValueArray(int nPrealloced) {
this(constructNew(nPrealloced));
MemoryCleaner.takeOwnership(this);
MemoryCleaner.setBoxedType(this, ValueArray.getType());
}
/**
* Get the GType of the ValueArray class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("g_value_array_get_type");
}
/**
* The memory layout of the native struct.
* @return the memory layout
*/
public static MemoryLayout getMemoryLayout() {
return MemoryLayout.structLayout(
ValueLayout.JAVA_INT.withName("n_values"),
MemoryLayout.paddingLayout(4),
ValueLayout.ADDRESS.withName("values"),
ValueLayout.JAVA_INT.withName("n_prealloced")
).withName("GValueArray");
}
/**
* Read the value of the field {@code n_values}.
*
* @return The value of the field {@code n_values}
*/
public int readNValues() {
var _result = (int) getMemoryLayout()
.varHandle(MemoryLayout.PathElement.groupElement("n_values")).get(handle(), 0);
return _result;
}
/**
* Write a value in the field {@code n_values}.
*
* @param nValues The new value for the field {@code n_values}
*/
public void writeNValues(int nValues) {
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("n_values"))
.set(handle(), 0, nValues);
}
/**
* Read the value of the field {@code values}.
*
* @return The value of the field {@code values}
*/
public Value readValues() {
var _result = (MemorySegment) getMemoryLayout()
.varHandle(MemoryLayout.PathElement.groupElement("values")).get(handle(), 0);
return MemorySegment.NULL.equals(_result) ? null : new Value(_result);
}
/**
* Write a value in the field {@code values}.
*
* @param values The new value for the field {@code values}
*/
public void writeValues(Value values) {
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("values"))
.set(handle(), 0, (values == null ? MemorySegment.NULL : values.handle()));
}
/**
* Read the value of the field {@code n_prealloced}.
*
* @return The value of the field {@code n_prealloced}
*/
public int readNPrealloced() {
var _result = (int) getMemoryLayout()
.varHandle(MemoryLayout.PathElement.groupElement("n_prealloced")).get(handle(), 0);
return _result;
}
/**
* Write a value in the field {@code n_prealloced}.
*
* @param nPrealloced The new value for the field {@code n_prealloced}
*/
public void writeNPrealloced(int nPrealloced) {
getMemoryLayout().varHandle(MemoryLayout.PathElement.groupElement("n_prealloced"))
.set(handle(), 0, nPrealloced);
}
@Deprecated
private static MemorySegment constructNew(int nPrealloced) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_value_array_new.invokeExact(nPrealloced);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return _result;
}
/**
* Insert a copy of {@code value} as last element of this ValueArray. If {@code value} is
* {@code null}, an uninitialized value is appended.
*
* @param value {@code GValue} to copy into {@code GValueArray}, or {@code null}
* @return the {@code GValueArray} passed in as this ValueArray
* @deprecated Use {@code GArray} and g_array_append_val() instead.
*/
@Deprecated
public ValueArray append(@Nullable Value value) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_value_array_append.invokeExact(handle(),
(MemorySegment) (value == null ? MemorySegment.NULL : value.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
_result = GObjects.boxedCopy(ValueArray.getType(), _result);
var _instance = MemorySegment.NULL.equals(_result) ? null : new ValueArray(_result);
MemoryCleaner.takeOwnership(_instance);
MemoryCleaner.setBoxedType(_instance, ValueArray.getType());
return _instance;
}
/**
* Construct an exact copy of a {@code GValueArray} by duplicating all its
* contents.
*
* @return Newly allocated copy of {@code GValueArray}
* @deprecated Use {@code GArray} and g_array_ref() instead.
*/
@Deprecated
public ValueArray copy() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_value_array_copy.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
var _instance = MemorySegment.NULL.equals(_result) ? null : new ValueArray(_result);
if (_instance != null) {
MemoryCleaner.takeOwnership(_instance);
MemoryCleaner.setBoxedType(_instance, ValueArray.getType());
}
return _instance;
}
/**
* Free a {@code GValueArray} including its contents.
*
* @deprecated Use {@code GArray} and g_array_unref() instead.
*/
@Deprecated
public void free() {
try {
MethodHandles.g_value_array_free.invokeExact(handle());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
}
/**
* Return a pointer to the value at {@code index} contained in this ValueArray.
*
* @param index index of the value of interest
* @return pointer to a value at {@code index} in this ValueArray
* @deprecated Use g_array_index() instead.
*/
@Deprecated
public Value getNth(int index) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_value_array_get_nth.invokeExact(handle(),
index);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
_result = GObjects.boxedCopy(Value.getType(), _result);
var _instance = MemorySegment.NULL.equals(_result) ? null : new Value(_result);
MemoryCleaner.takeOwnership(_instance);
MemoryCleaner.setBoxedType(_instance, Value.getType());
return _instance;
}
/**
* Insert a copy of {@code value} at specified position into this ValueArray. If {@code value}
* is {@code null}, an uninitialized value is inserted.
*
* @param index insertion position, must be <= value_array->;n_values
* @param value {@code GValue} to copy into {@code GValueArray}, or {@code null}
* @return the {@code GValueArray} passed in as this ValueArray
* @deprecated Use {@code GArray} and g_array_insert_val() instead.
*/
@Deprecated
public ValueArray insert(int index, @Nullable Value value) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_value_array_insert.invokeExact(handle(),
index, (MemorySegment) (value == null ? MemorySegment.NULL : value.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
_result = GObjects.boxedCopy(ValueArray.getType(), _result);
var _instance = MemorySegment.NULL.equals(_result) ? null : new ValueArray(_result);
MemoryCleaner.takeOwnership(_instance);
MemoryCleaner.setBoxedType(_instance, ValueArray.getType());
return _instance;
}
/**
* Insert a copy of {@code value} as first element of this ValueArray. If {@code value} is
* {@code null}, an uninitialized value is prepended.
*
* @param value {@code GValue} to copy into {@code GValueArray}, or {@code null}
* @return the {@code GValueArray} passed in as this ValueArray
* @deprecated Use {@code GArray} and g_array_prepend_val() instead.
*/
@Deprecated
public ValueArray prepend(@Nullable Value value) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_value_array_prepend.invokeExact(handle(),
(MemorySegment) (value == null ? MemorySegment.NULL : value.handle()));
} catch (Throwable _err) {
throw new AssertionError(_err);
}
_result = GObjects.boxedCopy(ValueArray.getType(), _result);
var _instance = MemorySegment.NULL.equals(_result) ? null : new ValueArray(_result);
MemoryCleaner.takeOwnership(_instance);
MemoryCleaner.setBoxedType(_instance, ValueArray.getType());
return _instance;
}
/**
* Remove the value at position {@code index} from this ValueArray.
*
* @param index position of value to remove, which must be less than
* this ValueArray->n_values
* @return the {@code GValueArray} passed in as this ValueArray
* @deprecated Use {@code GArray} and g_array_remove_index() instead.
*/
@Deprecated
public ValueArray remove(int index) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_value_array_remove.invokeExact(handle(),
index);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
_result = GObjects.boxedCopy(ValueArray.getType(), _result);
var _instance = MemorySegment.NULL.equals(_result) ? null : new ValueArray(_result);
MemoryCleaner.takeOwnership(_instance);
MemoryCleaner.setBoxedType(_instance, ValueArray.getType());
return _instance;
}
/**
* Sort this ValueArray using {@code compareFunc} to compare the elements according
* to the semantics of {@code GCompareDataFunc}.
*
* The current implementation uses the same sorting algorithm as standard
* C qsort() function.
*
* @param compareFunc function to compare elements
* @return the {@code GValueArray} passed in as this ValueArray
* @deprecated Use {@code GArray} and g_array_sort_with_data().
*/
@Deprecated
public ValueArray sort(CompareDataFunc compareFunc) {
try (var _arena = Arena.ofConfined()) {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.g_value_array_sort_with_data.invokeExact(
handle(),
(MemorySegment) (compareFunc == null ? MemorySegment.NULL : compareFunc.toCallback(_arena)),
MemorySegment.NULL);
} catch (Throwable _err) {
throw new AssertionError(_err);
}
_result = GObjects.boxedCopy(ValueArray.getType(), _result);
var _instance = MemorySegment.NULL.equals(_result) ? null : new ValueArray(_result);
MemoryCleaner.takeOwnership(_instance);
MemoryCleaner.setBoxedType(_instance, ValueArray.getType());
return _instance;
}
}
private static final class MethodHandles {
static final MethodHandle g_value_array_new = Interop.downcallHandle("g_value_array_new",
FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.JAVA_INT), false);
static final MethodHandle g_value_array_append = Interop.downcallHandle(
"g_value_array_append", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle g_value_array_copy = Interop.downcallHandle("g_value_array_copy",
FunctionDescriptor.of(ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle g_value_array_free = Interop.downcallHandle("g_value_array_free",
FunctionDescriptor.ofVoid(ValueLayout.ADDRESS), false);
static final MethodHandle g_value_array_get_nth = Interop.downcallHandle(
"g_value_array_get_nth", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.JAVA_INT), false);
static final MethodHandle g_value_array_insert = Interop.downcallHandle(
"g_value_array_insert", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.JAVA_INT, ValueLayout.ADDRESS), false);
static final MethodHandle g_value_array_prepend = Interop.downcallHandle(
"g_value_array_prepend", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
static final MethodHandle g_value_array_remove = Interop.downcallHandle(
"g_value_array_remove", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.JAVA_INT), false);
static final MethodHandle g_value_array_sort_with_data = Interop.downcallHandle(
"g_value_array_sort_with_data", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.ADDRESS, ValueLayout.ADDRESS, ValueLayout.ADDRESS), false);
}
}