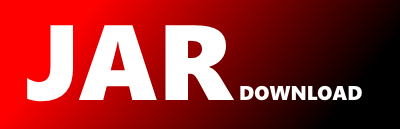
org.gnome.gobject.BindingFlags Maven / Gradle / Ivy
Show all versions of gobject Show documentation
/* Java-GI - Java language bindings for GObject-Introspection-based libraries
* Copyright (C) 2022-2023 Jan-Willem Harmannij
*
* SPDX-License-Identifier: LGPL-2.1-or-later
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, see .
*/
/* This file has been generated with Java-GI.
* Do not edit this file directly!
* Visit https://jwharm.github.io/java-gi for more information.
*/
package org.gnome.gobject;
import io.github.jwharm.javagi.gobject.*;
import io.github.jwharm.javagi.gobject.types.*;
import io.github.jwharm.javagi.base.*;
import io.github.jwharm.javagi.interop.*;
import java.lang.foreign.*;
import java.lang.invoke.*;
import org.jetbrains.annotations.*;
/**
* Flags to be passed to g_object_bind_property() or
* g_object_bind_property_full().
*
* This enumeration can be extended at later date.
* @version 2.26
*/
public class BindingFlags extends io.github.jwharm.javagi.base.Bitfield {
/**
* Get the GType of the GBindingFlags class.
* @return the GType
*/
public static org.gnome.glib.Type getType() {
return Interop.getType("g_binding_flags_get_type");
}
/**
* The default binding; if the source property
* changes, the target property is updated with its value.
*/
public static final BindingFlags DEFAULT = new BindingFlags(0);
/**
* Bidirectional binding; if either the
* property of the source or the property of the target changes,
* the other is updated.
*/
public static final BindingFlags BIDIRECTIONAL = new BindingFlags(1);
/**
* Synchronize the values of the source and
* target properties when creating the binding; the direction of
* the synchronization is always from the source to the target.
*/
public static final BindingFlags SYNC_CREATE = new BindingFlags(2);
/**
* If the two properties being bound are
* booleans, setting one to {@code true} will result in the other being
* set to {@code false} and vice versa. This flag will only work for
* boolean properties, and cannot be used when passing custom
* transformation functions to g_object_bind_property_full().
*/
public static final BindingFlags INVERT_BOOLEAN = new BindingFlags(4);
/**
* Create a new BindingFlags with the provided value
*/
public BindingFlags(int value) {
super(value);
}
/**
* Combine (bitwise OR) operation
* @param masks one or more values to combine with
* @return the combined value by calculating {@code this | mask}
*/
public BindingFlags or(BindingFlags... masks) {
int value = this.getValue();
for (BindingFlags arg : masks) {
value |= arg.getValue();
}
return new BindingFlags(value);
}
/**
* Combine (bitwise OR) operation
* @param mask the first value to combine
* @param masks the other values to combine
* @return the combined value by calculating {@code mask | masks[0] | masks[1] | ...}
*/
public static BindingFlags combined(BindingFlags mask, BindingFlags... masks) {
int value = mask.getValue();
for (BindingFlags arg : masks) {
value |= arg.getValue();
}
return new BindingFlags(value);
}
}