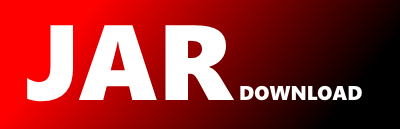
org.gnome.gobject.package-info Maven / Gradle / Ivy
Show all versions of gobject Show documentation
/**
* This package contains the generated bindings for GObject.
*
* The following native libraries are required and will be loaded: {@code libgobject-2.0.0}
*
* For namespace-global declarations, refer to the {@link GObjects} class documentation.
*
*
Enumerations Flags
* The GLib type system provides fundamental types for enumeration and
* flags types. (Flags types are like enumerations, but allow their
* values to be combined by bitwise or). A registered enumeration or
* flags type associates a name and a nickname with each allowed
* value, and the methods g_enum_get_value_by_name(),
* g_enum_get_value_by_nick(), g_flags_get_value_by_name() and
* g_flags_get_value_by_nick() can look up values by their name or
* nickname. When an enumeration or flags type is registered with the
* GLib type system, it can be used as value type for object
* properties, using g_param_spec_enum() or g_param_spec_flags().
*
* GObject ships with a utility called [glib-mkenums][glib-mkenums],
* that can construct suitable type registration functions from C enumeration
* definitions.
*
* Example of how to get a string representation of an enum value:
*
{@code
* GEnumClass *enum_class;
* GEnumValue *enum_value;
*
* enum_class = g_type_class_ref (MAMAN_TYPE_MY_ENUM);
* enum_value = g_enum_get_value (enum_class, MAMAN_MY_ENUM_FOO);
*
* g_print ("Name: %s\\n", enum_value->value_name);
*
* g_type_class_unref (enum_class);
* }
*
* Gboxed
* {@code GBoxed} is a generic wrapper mechanism for arbitrary C structures.
*
* The only thing the type system needs to know about the structures is how to
* copy them (a {@code GBoxedCopyFunc}) and how to free them (a {@code GBoxedFreeFunc});
* beyond that, they are treated as opaque chunks of memory.
*
* Boxed types are useful for simple value-holder structures like rectangles or
* points. They can also be used for wrapping structures defined in non-{@code GObject}
* based libraries. They allow arbitrary structures to be handled in a uniform
* way, allowing uniform copying (or referencing) and freeing (or unreferencing)
* of them, and uniform representation of the type of the contained structure.
* In turn, this allows any type which can be boxed to be set as the data in a
* {@code GValue}, which allows for polymorphic handling of a much wider range of data
* types, and hence usage of such types as {@code GObject} property values.
*
* {@code GBoxed} is designed so that reference counted types can be boxed. Use the
* type’s ‘ref’ function as the {@code GBoxedCopyFunc}, and its ‘unref’ function as the
* {@code GBoxedFreeFunc}. For example, for {@code GBytes}, the {@code GBoxedCopyFunc} is
* g_bytes_ref(), and the {@code GBoxedFreeFunc} is g_bytes_unref().
*
*
Generic Values
* The {@code GValue} structure is basically a variable container that consists
* of a type identifier and a specific value of that type.
*
* The type identifier within a {@code GValue} structure always determines the
* type of the associated value.
*
* To create an undefined {@code GValue} structure, simply create a zero-filled
* {@code GValue} structure. To initialize the {@code GValue}, use the g_value_init()
* function. A {@code GValue} cannot be used until it is initialized. Before
* destruction you must always use g_value_unset() to make sure allocated
* memory is freed.
*
* The basic type operations (such as freeing and copying) are determined
* by the {@code GTypeValueTable} associated with the type ID stored in the {@code GValue}.
* Other {@code GValue} operations (such as converting values between types) are
* provided by this interface.
*
* The code in the example program below demonstrates {@code GValue}'s
* features.
*
{@code
* #include
*
* static void
* int2string (const GValue *src_value,
* GValue *dest_value)
* {
* if (g_value_get_int (src_value) == 42)
* g_value_set_static_string (dest_value, "An important number");
* else
* g_value_set_static_string (dest_value, "What's that?");
* }
*
* int
* main (int argc,
* char *argv[])
* {
* // GValues must be initialized
* GValue a = G_VALUE_INIT;
* GValue b = G_VALUE_INIT;
* const gchar *message;
*
* // The GValue starts empty
* g_assert (!G_VALUE_HOLDS_STRING (&a));
*
* // Put a string in it
* g_value_init (&a, G_TYPE_STRING);
* g_assert (G_VALUE_HOLDS_STRING (&a));
* g_value_set_static_string (&a, "Hello, world!");
* g_printf ("%s\\n", g_value_get_string (&a));
*
* // Reset it to its pristine state
* g_value_unset (&a);
*
* // It can then be reused for another type
* g_value_init (&a, G_TYPE_INT);
* g_value_set_int (&a, 42);
*
* // Attempt to transform it into a GValue of type STRING
* g_value_init (&b, G_TYPE_STRING);
*
* // An INT is transformable to a STRING
* g_assert (g_value_type_transformable (G_TYPE_INT, G_TYPE_STRING));
*
* g_value_transform (&a, &b);
* g_printf ("%s\\n", g_value_get_string (&b));
*
* // Attempt to transform it again using a custom transform function
* g_value_register_transform_func (G_TYPE_INT, G_TYPE_STRING, int2string);
* g_value_transform (&a, &b);
* g_printf ("%s\\n", g_value_get_string (&b));
* return 0;
* }
* }
*
* See also [gobject-Standard-Parameter-and-Value-Types] for more information on
* validation of {@code GValue}.
*
* For letting a {@code GValue} own (and memory manage) arbitrary types or pointers,
* they need to become a [boxed type][gboxed]. The example below shows how
* the pointer {@code mystruct} of type {@code MyStruct} is used as a [boxed type][gboxed].
*
{@code
* typedef struct { ... } MyStruct;
* G_DEFINE_BOXED_TYPE (MyStruct, my_struct, my_struct_copy, my_struct_free)
*
* // These two lines normally go in a public header. By GObject convention,
* // the naming scheme is NAMESPACE_TYPE_NAME:
* #define MY_TYPE_STRUCT (my_struct_get_type ())
* GType my_struct_get_type (void);
*
* void
* foo ()
* {
* GValue *value = g_new0 (GValue, 1);
* g_value_init (value, MY_TYPE_STRUCT);
* g_value_set_boxed (value, mystruct);
* // [... your code ....]
* g_value_unset (value);
* g_free (value);
* }
* }
*
* Gtype
* The GType API is the foundation of the GObject system. It provides the
* facilities for registering and managing all fundamental data types,
* user-defined object and interface types.
*
* For type creation and registration purposes, all types fall into one of
* two categories: static or dynamic. Static types are never loaded or
* unloaded at run-time as dynamic types may be. Static types are created
* with g_type_register_static() that gets type specific information passed
* in via a {@code GTypeInfo} structure.
*
* Dynamic types are created with g_type_register_dynamic() which takes a
* {@code GTypePlugin} structure instead. The remaining type information (the
* {@code GTypeInfo} structure) is retrieved during runtime through {@code GTypePlugin}
* and the g_type_plugin_*() API.
*
* These registration functions are usually called only once from a
* function whose only purpose is to return the type identifier for a
* specific class. Once the type (or class or interface) is registered,
* it may be instantiated, inherited, or implemented depending on exactly
* what sort of type it is.
*
* There is also a third registration function for registering fundamental
* types called g_type_register_fundamental() which requires both a {@code GTypeInfo}
* structure and a {@code GTypeFundamentalInfo} structure but it is seldom used
* since most fundamental types are predefined rather than user-defined.
*
* Type instance and class structs are limited to a total of 64 KiB,
* including all parent types. Similarly, type instances' private data
* (as created by G_ADD_PRIVATE()) are limited to a total of
* 64 KiB. If a type instance needs a large static buffer, allocate it
* separately (typically by using {@code GArray} or {@code GPtrArray}) and put a pointer
* to the buffer in the structure.
*
* As mentioned in the [GType conventions][gtype-conventions], type names must
* be at least three characters long. There is no upper length limit. The first
* character must be a letter (a–z or A–Z) or an underscore (‘_’). Subsequent
* characters can be letters, numbers or any of ‘-_+’.
*
* Runtime Debugging
* When {@code G_ENABLE_DEBUG} is defined during compilation, the GObject library
* supports an environment variable {@code GOBJECT_DEBUG} that can be set to a
* combination of flags to trigger debugging messages about
* object bookkeeping and signal emissions during runtime.
*
* The currently supported flags are:
*
* - {@code objects}: Tracks all {@code GObject} instances in a global hash table called
* {@code debug_objects_ht}, and prints the still-alive objects on exit.
*
- {@code instance-count}: Tracks the number of instances of every {@code GType} and makes
* it available via the g_type_get_instance_count() function.
*
- {@code signals}: Currently unused.
*
*
* Objects
* GObject is the fundamental type providing the common attributes and
* methods for all object types in GTK, Pango and other libraries
* based on GObject. The GObject class provides methods for object
* construction and destruction, property access methods, and signal
* support. Signals are described in detail [here][gobject-Signals].
*
* For a tutorial on implementing a new GObject class, see [How to define and
* implement a new GObject][howto-gobject]. For a list of naming conventions for
* GObjects and their methods, see the [GType conventions][gtype-conventions].
* For the high-level concepts behind GObject, read [Instantiatable classed types:
* Objects][gtype-instantiatable-classed].
*
* Floating references # {#floating-ref}
* Note: Floating references are a C convenience API and should not be
* used in modern GObject code. Language bindings in particular find the
* concept highly problematic, as floating references are not identifiable
* through annotations, and neither are deviations from the floating reference
* behavior, like types that inherit from {@code GInitiallyUnowned} and still return
* a full reference from g_object_new().
*
* GInitiallyUnowned is derived from GObject. The only difference between
* the two is that the initial reference of a GInitiallyUnowned is flagged
* as a "floating" reference. This means that it is not specifically
* claimed to be "owned" by any code portion. The main motivation for
* providing floating references is C convenience. In particular, it
* allows code to be written as:
*
{@code
* container = create_container ();
* container_add_child (container, create_child());
* }
*
* If container_add_child() calls g_object_ref_sink() on the passed-in child,
* no reference of the newly created child is leaked. Without floating
* references, container_add_child() can only g_object_ref() the new child,
* so to implement this code without reference leaks, it would have to be
* written as:
*
{@code
* Child *child;
* container = create_container ();
* child = create_child ();
* container_add_child (container, child);
* g_object_unref (child);
* }
*
* The floating reference can be converted into an ordinary reference by
* calling g_object_ref_sink(). For already sunken objects (objects that
* don't have a floating reference anymore), g_object_ref_sink() is equivalent
* to g_object_ref() and returns a new reference.
*
* Since floating references are useful almost exclusively for C convenience,
* language bindings that provide automated reference and memory ownership
* maintenance (such as smart pointers or garbage collection) should not
* expose floating references in their API. The best practice for handling
* types that have initially floating references is to immediately sink those
* references after g_object_new() returns, by checking if the {@code GType}
* inherits from {@code GInitiallyUnowned}. For instance:
*
{@code
* GObject *res = g_object_new_with_properties (gtype,
* n_props,
* prop_names,
* prop_values);
*
* // or: if (g_type_is_a (gtype, G_TYPE_INITIALLY_UNOWNED))
* if (G_IS_INITIALLY_UNOWNED (res))
* g_object_ref_sink (res);
*
* return res;
* }
*
* Some object implementations may need to save an objects floating state
* across certain code portions (an example is {@code GtkMenu}), to achieve this,
* the following sequence can be used:
*
{@code
* // save floating state
* gboolean was_floating = g_object_is_floating (object);
* g_object_ref_sink (object);
* // protected code portion
*
* ...
*
* // restore floating state
* if (was_floating)
* g_object_force_floating (object);
* else
* g_object_unref (object); // release previously acquired reference
* }
*
* Param Value Types
* {@code GValue} provides an abstract container structure which can be
* copied, transformed and compared while holding a value of any
* (derived) type, which is registered as a {@code GType} with a
* {@code GTypeValueTable} in its {@code GTypeInfo} structure. Parameter
* specifications for most value types can be created as {@code GParamSpec}
* derived instances, to implement e.g. {@code GObject} properties which
* operate on {@code GValue} containers.
*
* Parameter names need to start with a letter (a-z or A-Z). Subsequent
* characters can be letters, numbers or a '-'.
* All other characters are replaced by a '-' during construction.
*
* See also {@code GValue} for more information.
*
*
Signals
* The basic concept of the signal system is that of the emission
* of a signal. Signals are introduced per-type and are identified
* through strings. Signals introduced for a parent type are available
* in derived types as well, so basically they are a per-type facility
* that is inherited.
*
* A signal emission mainly involves invocation of a certain set of
* callbacks in precisely defined manner. There are two main categories
* of such callbacks, per-object ones and user provided ones.
* (Although signals can deal with any kind of instantiatable type, I'm
* referring to those types as "object types" in the following, simply
* because that is the context most users will encounter signals in.)
* The per-object callbacks are most often referred to as "object method
* handler" or "default (signal) handler", while user provided callbacks are
* usually just called "signal handler".
*
* The object method handler is provided at signal creation time (this most
* frequently happens at the end of an object class' creation), while user
* provided handlers are frequently connected and disconnected to/from a
* certain signal on certain object instances.
*
* A signal emission consists of five stages, unless prematurely stopped:
*
* 1. Invocation of the object method handler for {@link org.gnome.gobject.SignalFlags#RUN_FIRST} signals
*
* 2. Invocation of normal user-provided signal handlers (where the {@code after}
* flag is not set)
*
* 3. Invocation of the object method handler for {@link org.gnome.gobject.SignalFlags#RUN_LAST} signals
*
* 4. Invocation of user provided signal handlers (where the {@code after} flag is set)
*
* 5. Invocation of the object method handler for {@link org.gnome.gobject.SignalFlags#RUN_CLEANUP} signals
*
* The user-provided signal handlers are called in the order they were
* connected in.
*
* All handlers may prematurely stop a signal emission, and any number of
* handlers may be connected, disconnected, blocked or unblocked during
* a signal emission.
*
* There are certain criteria for skipping user handlers in stages 2 and 4
* of a signal emission.
*
* First, user handlers may be blocked. Blocked handlers are omitted during
* callback invocation, to return from the blocked state, a handler has to
* get unblocked exactly the same amount of times it has been blocked before.
*
* Second, upon emission of a {@link org.gnome.gobject.SignalFlags#DETAILED} signal, an additional
* {@code detail} argument passed in to g_signal_emit() has to match the detail
* argument of the signal handler currently subject to invocation.
* Specification of no detail argument for signal handlers (omission of the
* detail part of the signal specification upon connection) serves as a
* wildcard and matches any detail argument passed in to emission.
*
* While the {@code detail} argument is typically used to pass an object property name
* (as with {@code GObject}::notify), no specific format is mandated for the detail
* string, other than that it must be non-empty.
*
* Memory management of signal handlers # {#signal-memory-management}
* If you are connecting handlers to signals and using a {@code GObject} instance as
* your signal handler user data, you should remember to pair calls to
* g_signal_connect() with calls to g_signal_handler_disconnect() or
* g_signal_handlers_disconnect_by_func(). While signal handlers are
* automatically disconnected when the object emitting the signal is finalised,
* they are not automatically disconnected when the signal handler user data is
* destroyed. If this user data is a {@code GObject} instance, using it from a
* signal handler after it has been finalised is an error.
*
* There are two strategies for managing such user data. The first is to
* disconnect the signal handler (using g_signal_handler_disconnect() or
* g_signal_handlers_disconnect_by_func()) when the user data (object) is
* finalised; this has to be implemented manually. For non-threaded programs,
* g_signal_connect_object() can be used to implement this automatically.
* Currently, however, it is unsafe to use in threaded programs.
*
* The second is to hold a strong reference on the user data until after the
* signal is disconnected for other reasons. This can be implemented
* automatically using g_signal_connect_data().
*
* The first approach is recommended, as the second approach can result in
* effective memory leaks of the user data if the signal handler is never
* disconnected for some reason.
*
*
Value Arrays
* The prime purpose of a {@code GValueArray} is for it to be used as an
* object property that holds an array of values. A {@code GValueArray} wraps
* an array of {@code GValue} elements in order for it to be used as a boxed
* type through {@code G_TYPE_VALUE_ARRAY}.
*
* {@code GValueArray} is deprecated in favour of {@code GArray} since GLib 2.32. It
* is possible to create a {@code GArray} that behaves like a {@code GValueArray} by
* using the size of {@code GValue} as the element size, and by setting
* g_value_unset() as the clear function using g_array_set_clear_func(),
* for instance, the following code:
*
{@code
* GValueArray *array = g_value_array_new (10);
* }
*
* can be replaced by:
*
{@code
* GArray *array = g_array_sized_new (FALSE, TRUE, sizeof (GValue), 10);
* g_array_set_clear_func (array, (GDestroyNotify) g_value_unset);
* }
*/
package org.gnome.gobject;