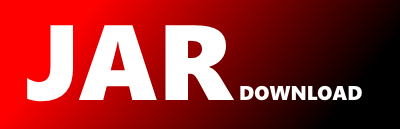
org.freedesktop.gstreamer.gst.DebugLevel Maven / Gradle / Ivy
// Java-GI - Java language bindings for GObject-Introspection-based libraries
// Copyright (C) 2022-2024 Jan-Willem Harmannij
//
// SPDX-License-Identifier: LGPL-2.1-or-later
//
// This library is free software; you can redistribute it and/or
// modify it under the terms of the GNU Lesser General Public
// License as published by the Free Software Foundation; either
// version 2.1 of the License, or (at your option) any later version.
//
// This library is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
// Lesser General Public License for more details.
//
// You should have received a copy of the GNU Lesser General Public
// License along with this library; if not, see .
//
// This file has been generated with Java-GI.
// Do not edit this file directly!
// Visit for more information.
//
package org.freedesktop.gstreamer.gst;
import io.github.jwharm.javagi.base.Enumeration;
import io.github.jwharm.javagi.interop.Interop;
import java.lang.IllegalStateException;
import java.lang.Override;
import java.lang.String;
import java.lang.foreign.FunctionDescriptor;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.ValueLayout;
import java.lang.invoke.MethodHandle;
import javax.annotation.processing.Generated;
import org.gnome.glib.Type;
/**
* The level defines the importance of a debugging message. The more important a
* message is, the greater the probability that the debugging system outputs it.
*/
@Generated("io.github.jwharm.JavaGI")
public enum DebugLevel implements Enumeration {
/**
* No debugging level specified or desired. Used to deactivate
* debugging output.
*/
NONE(0),
/**
* Error messages are to be used only when an error occurred
* that stops the application from keeping working correctly.
* An examples is gst_element_error, which outputs a message with this priority.
* It does not mean that the application is terminating as with g_error.
*/
ERROR(1),
/**
* Warning messages are to inform about abnormal behaviour
* that could lead to problems or weird behaviour later on. An example of this
* would be clocking issues ("your computer is pretty slow") or broken input
* data ("Can't synchronize to stream.")
*/
WARNING(2),
/**
* Fixme messages are messages that indicate that something
* in the executed code path is not fully implemented or handled yet. Note
* that this does not replace proper error handling in any way, the purpose
* of this message is to make it easier to spot incomplete/unfinished pieces
* of code when reading the debug log.
*/
FIXME(3),
/**
* Informational messages should be used to keep the developer
* updated about what is happening.
* Examples where this should be used are when a typefind function has
* successfully determined the type of the stream or when an mp3 plugin detects
* the format to be used. ("This file has mono sound.")
*/
INFO(4),
/**
* Debugging messages should be used when something common
* happens that is not the expected default behavior, or something that's
* useful to know but doesn't happen all the time (ie. per loop iteration or
* buffer processed or event handled).
* An example would be notifications about state changes or receiving/sending
* of events.
*/
DEBUG(5),
/**
* Log messages are messages that are very common but might be
* useful to know. As a rule of thumb a pipeline that is running as expected
* should never output anything else but LOG messages whilst processing data.
* Use this log level to log recurring information in chain functions and
* loop functions, for example.
*/
LOG(6),
/**
* Tracing-related messages.
* Examples for this are referencing/dereferencing of objects.
*/
TRACE(7),
/**
* memory dump messages are used to log (small) chunks of
* data as memory dumps in the log. They will be displayed as hexdump with
* ASCII characters.
*/
MEMDUMP(9),
/**
* The number of defined debugging levels.
*/
COUNT(10);
static {
Gst.javagi$ensureInitialized();
}
private final int value;
/**
* Create a new DebugLevel for the provided value
*
* @param value the enum value
*/
private DebugLevel(int value) {
this.value = value;
}
/**
* Create a new DebugLevel for the provided value
*
* @param value the enum value
* @return the enum for the provided value
*/
public static DebugLevel of(int value) {
return switch(value) {
case 0 -> NONE;
case 1 -> ERROR;
case 2 -> WARNING;
case 3 -> FIXME;
case 4 -> INFO;
case 5 -> DEBUG;
case 6 -> LOG;
case 7 -> TRACE;
case 9 -> MEMDUMP;
case 10 -> COUNT;
default -> throw new IllegalStateException("Unexpected value: " + value);
} ;
}
/**
* Get the numeric value of this enum
*
* @return the enum value
*/
@Override
public int getValue() {
return value;
}
/**
* Get the GType of the DebugLevel class
*
* @return the GType
*/
public static Type getType() {
return Interop.getType("gst_debug_level_get_type");
}
/**
* Get the string representation of a debugging level
*
* @return the name
*/
public String getName() {
MemorySegment _result;
try {
_result = (MemorySegment) MethodHandles.gst_debug_level_get_name.invokeExact(
getValue());
} catch (Throwable _err) {
throw new AssertionError(_err);
}
return Interop.getStringFrom(_result, false);
}
private static final class MethodHandles {
static final MethodHandle gst_debug_level_get_name = Interop.downcallHandle(
"gst_debug_level_get_name", FunctionDescriptor.of(ValueLayout.ADDRESS,
ValueLayout.JAVA_INT), false);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy